C++ || “One Size Fits All” – BubbleSort Which Works For Integer, Float, & Char Arrays
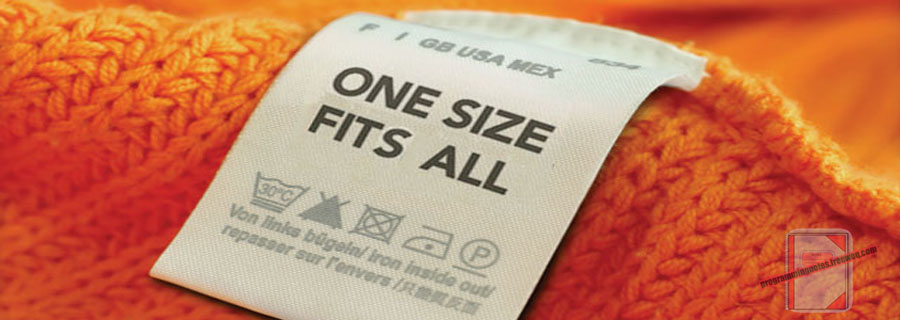
Here is another sorting algorithm, which sorts data that is contained in an array. The difference between this method, and the previous methods of sorting data is that this method works with multiple data types, all in one function. So for example, if you wanted to sort an integer and a character array within the same program, the code demonstrated on this page has the ability to sort both data types, eliminating the need to make two separate sorting functions for the two different data types.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Integer Arrays
Character Arrays
BubbleSort
Pointers
Function Pointers - What Are They?
Memcpy
Sizeof
Sizet
Malloc
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 |
#include <iostream> #include <cstdlib> #include <cctype> #include <iomanip> #include <cstring> #include <ctime> using namespace std; // function prototypes void BubbleSort(void* list, size_t nelm, size_t size, int (*cmp) (const void*, const void*)); void Swap(void* a, void* b, size_t width); int IntCompare (const void * a, const void * b); int StrCompare(const void *a, const void *b); int FloatCompare (const void * a, const void * b); // constant variable for int arrays const int NUM_INTS=14; int main() { // seed random number generator srand(time(NULL)); // =================== INT EXAMPLE =================== // int intNumbers[100]; int numInts=0; // place random numbers into the array for(int x = 0; x < NUM_INTS; ++x) { intNumbers[x] =rand()%100+1; } cout<<"Original values in the int array:n"; // show original data to the user for(int x=0; x<NUM_INTS; ++x) { cout << setw(4) << intNumbers[x]; } // this is the function call, which takes in an // integer array for sorting purposes // NOTICE: the function "IntCompare" is being used as a parameter BubbleSort(intNumbers,NUM_INTS,sizeof(int),IntCompare); cout<<"nnThe sorted values in the int array:n"; // display sorted array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(4) << intNumbers[x]; } // creates a line seperator cout << "n-----------------------------------------------" <<"----------------------------------------n"; // =================== FLOAT EXAMPLE =================== // float floatNumbers[100]; // place random numbers into the array for(int x = 0; x < NUM_INTS; ++x) { floatNumbers[x] =(rand()%100+1)+(0.5); } cout<<"Original values in the float array:n"; // show original array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(6) << floatNumbers[x]; } // this is the function call, which takes in a // float array for sorting purposes // NOTICE: the function "FloatCompare" is being used as a parameter BubbleSort(floatNumbers,NUM_INTS,sizeof(float),FloatCompare); cout<<"nnThe sorted values in the float array:n"; // show sorted array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(6) << floatNumbers[x]; } // creates a line seperator cout << "n-----------------------------------------------" <<"----------------------------------------n"; // =================== CHAR EXAMPLE =================== // char* names[100]={"This", "Is","Random","Text","Brought","To", "You","By","My","Programming","Notes"}; int numNames=11; cout<<"Original values in the char array:n"; // show original array to user for(int x=0; x<numNames; ++x) { cout << " " << names[x]; } // this is the function call, which takes in a // char array for sorting purposes // NOTICE: the function "StrCompare" is being used as a parameter BubbleSort(names,numNames,sizeof(char*),StrCompare); cout<<"nnThe sorted values in the char array:n"; // show sorted array to user for(int x=0; x<numNames; ++x) { cout << " " << names[x]; } cout<<endl; return 0; }// end of main void BubbleSort(void* arry, size_t nelm, size_t size, int (*cmp) (const void*, const void*)) {// this function sorts the received array bool sorted = false; do{ sorted = true; for(unsigned int x=0; x < nelm-1; ++x) { if(cmp((((char*)arry)+(x*size)), (((char*)arry)+((x+1)*size))) > 0) { Swap((((char*)arry)+((x)*size)), (((char*)arry)+((x+1)*size)),size); sorted=false; } } --nelm; }while(!sorted); }// end of BubbleSort void Swap(void* a, void* b, size_t width) {// this function swaps the values in the array void *tmp = malloc(width); memcpy(tmp, a, width); memcpy(a, b, width); memcpy(b, tmp, width); free(tmp); }// end of Swap int FloatCompare (const void * a, const void * b) {// this function compares two float values together return (*(float*)a - *(float*)b); }// end of FloatCompare int IntCompare (const void * a, const void * b) {// this function compares two int values together return (*(int*)a - *(int*)b); }// end of IntCompare int StrCompare(const void *a, const void *b) {// this function compares two char values together return(strcmp(*(const char **)a, *(const char **)b)); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Notice, the same function declaration is being used for all 3 different data types, with the only difference between each function call are the parameters which are being sent out.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: The function works for all three data types)
Original values in the int array:
44 91 43 22 20 100 77 80 84 60 47 91 51 81The sorted values in the int array:
20 22 43 44 47 51 60 77 80 81 84 91 91 100
---------------------------------------------------------------------------------------
Original values in the float array:
49.5 30.5 67.5 50.5 29.5 89.5 78.5 80.5 54.5 7.5 54.5 38.5 56.5 70.5The sorted values in the float array:
7.5 29.5 30.5 38.5 49.5 50.5 54.5 54.5 56.5 67.5 70.5 78.5 80.5 89.5
---------------------------------------------------------------------------------------
Original values in the char array:
This Is Random Text Brought To You By My Programming NotesThe sorted values in the char array:
Brought By Is My Notes Programming Random Text This To You
Leave a Reply