C++ || Snippet – Custom “For Each” Iterator Function For Arrays
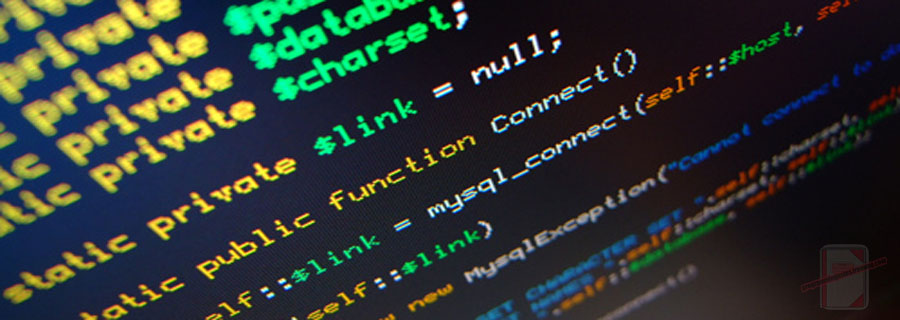
A “For Each” algorithm is a function that iterates over all the elements contained in a storage collection, and calls a separate user defined function to manipulate each element within that collection.
The code demonstrated on this page is different from the STL for_each function in that this implementation is meant to work mainly on standard arrays.
The example below illustrates the “For Each” concept in a fully compilable and working demonstration. This example is trivial in that all it does is call a separate user defined function to display all of the contents within each array. This implementation revolves around using a simple function pointer and a series of void pointers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
// ============================================================================ // Author: K Perkins // Date: July 12, 2013 // Taken From: http://programmingnotes.org/ // File: ForEach.cpp // Description: Demonstrates the use of a custom "For Each" function // implementation. // ============================================================================ #include <iostream> #include <cstring> #include <string> using namespace std; // function prototypes void ForEach(const void* arry, int arrySize, size_t sizeType, void(*function)(void*)); void PrintChar(void* c); // user defined functions void PrintInt(void* c); void PrintCString(void* c); void PrintString(void* c); int main() { // declare variables char charArry[] = "This is a simple C-String"; char* twoD_CharArry[] = {"This","is","a","2-D","C-String","array"}; int intArry[] = {1,2,3,4,5,6,7,8,9,10}; string str = "This is a simple std::string"; string strArry[] = {"This","is","a","std::string","array"}; // char array ForEach(charArry, strlen(charArry), sizeof(char), PrintChar); cout<<endl<<endl; // int array ForEach(intArry, 10, sizeof(int), PrintInt); cout<<endl<<endl; // 2-D char array ForEach(twoD_CharArry, 6, sizeof(char*), PrintCString); cout<<endl<<endl; // std::string ForEach(str.c_str(), str.length(), sizeof(char), PrintChar); cout<<endl<<endl; // std::string array ForEach(strArry, 5, sizeof(string), PrintString); cout<<endl<<endl; return 0; }// end of main /** * FUNCTION: ForEach * USE: Applies the "void(*function)" to each of the elements in the array * @param arry - the array being iterated * @param arrySize - the total number of items in the array (the array length) * @param sizeType - the number of bytes required to represent the data-type * of the individual items in the array * @param *function - function pointer that accepts an element in the array as argument * @return - none */ void ForEach(const void* arry, int arrySize, size_t sizeType, void(*function)(void*)) { for(unsigned x = 0; x < (unsigned)arrySize; ++x) { function((char*)arry + (x*sizeType)); } }// end of ForEach void PrintChar(void* c) { cout<<*(char*)c <<" "; }// end of PrintChar void PrintInt(void* c) { cout<<*(int*)c <<" "; }// end of PrintInt void PrintCString(void* c) { cout<<*(char**)c <<" "; }// end of PrintCString void PrintString(void* c) { cout<<*(string*)c <<" "; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
1 2 3 4 5 6 7 8 9 |
T h i s i s a s i m p l e C - S t r i n g 1 2 3 4 5 6 7 8 9 10 This is a 2-D C-String array T h i s i s a s i m p l e s t d : : s t r i n g This is a std::string array |
Leave a Reply