Python || Custom Random Number Generator Class MyRNG
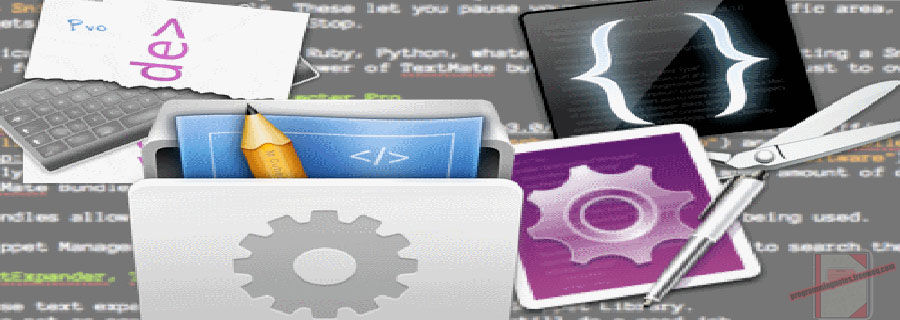
The following is sample code for a simple random number generator class. This random number generator is based on the Park & Miller paper “Random Number Generators: Good Ones Are Hard To Find.”
This class has three functions. The constructor initializes data members “m_min” and “m_max” which stores the minimum and maximum range of values in which the random numbers will generate.
The “Seed()” function stores the value of the current seed within the class, and the “Next()” function returns a random number to the caller using an algorithm based on the Park & Miller paper listed in the paragraph above. Each time the “Next()” function is called, a new random number is generated and returned to the caller.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
# ============================================================================= # Author: K Perkins # Date: Aug 6, 2013 # Taken From: http://programmingnotes.org/ # File: MyRNG.py # Description: This is the MyRNG.py module which contains the class # declaration for the random number generator. This module recieves a # seed, minimum, and maximum number and generates a random number using # the "Next()" function. Each time the "Next()" function is called, a new # random number is generated and returned to the caller # ============================================================================= import math, time class MyRNG: # MyRNG class. This is the class declaration for the random number # generator. The constructor initializes data members "m_min" and # "m_max" which stores the minimum and maximum range of values in which # the random numbers will generate. There is another variable named "m_seed" # which is initialized using the method Seed(), and stores the value of the # current seed within the class. Using the obtained values from above, the # "Next()" method returns a random number to the caller using an algorithm # based on the Park & Miller paper "RANDOM NUMBER GENERATORS: GOOD ONES ARE # HARD TO FIND" def __init__(self, low = 0, high = 0): # The constructor initializes data members "m_min" and "m_max" if(low < 2): low = 2 if(high < 2): high = 9223372036854775807 self.m_min = low self.m_max = high self.m_seed = time.time() def Seed(self, seed): # Seed the generator with 'seed' self.m_seed = seed def Next(self): # Return the next random number using an algorithm based on the # Park & Miller paper "RANDOM NUMBER GENERATORS: GOOD ONES ARE # HARD TO FIND" a = self.m_min m = self.m_max q = math.trunc(m / a) r = m % a hi = self.m_seed / q lo = self.m_seed % q x = (a * lo) - (r * hi) if(x < a): x += a self.m_seed = x self.m_seed %= m # ensure that the random number is not less # than the minimum number within the user specified range if(self.m_seed < a): self.m_seed += a return int(self.m_seed) def test(): # Simple test function to see if the functionality of my class # is there and works random = MyRNG(7, 1987) random.Seed(806189064) for x in range(15): print("%d, " %(random.Next()), end = "") if __name__ == '__main__': test() # http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
===== DEMONSTRATION HOW TO USE =====
Use of the above class is very simple to use. A sample function demonstrating its use is provided on line #64 in the above code snippet.
After running the code, the following is sample output:
SAMPLE OUTPUT:
1037, 1296, 1123, 1900, 1375, 1676, 1798, 662, 664, 674, 746, 1249, 793, 1578, 1112,
what is the .m_ means?