Java || Snippet – How To Send Text Over A Network Using Socket
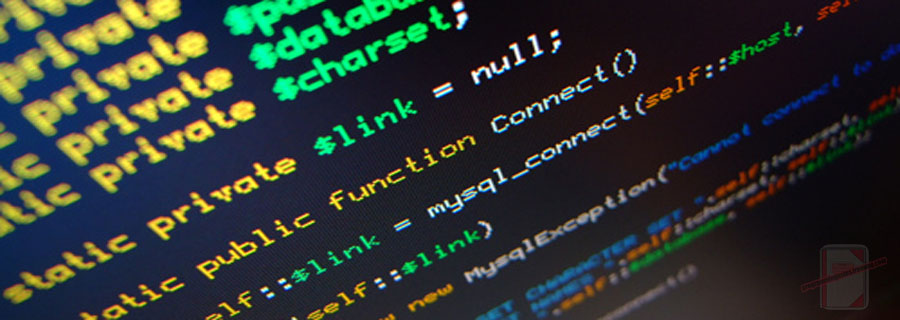
The following is sample code which demonstrates the use of the “socket” function call for interprocess communication over a network.
The following example demonstrates a simple network application in which a client sends text to a server, and the server replies (sends text) back to the client.
Note: The server program must be ran before the client program!
=== 1. SERVER ===
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 |
// ============================================================================ // Author: K Perkins // Date: Jan 8, 2014 // Taken From: http://programmingnotes.org/ // File: Server.java // Description: This program implements a simple network application in // which a client sends text to a server and the server replies back to the // client. This is the server program, and has the following functionality: // 1. Listen for incoming connections on a specified port. // 2. When a client tries to connect, the connection is accepted. // 3. When a connection is created, text is received from the client. // 4. Text is sent back to the client from the server. // 5. Close the connection and go back to waiting for more connections. // ============================================================================ import java.io.*; import java.net.*; import java.util.Date; // Compile & Run // javac Server.java // java Server 1234 class Server { // the port on which to listen protected int listenPort; // the initialization constructor public Server(int port) { // save the listening port listenPort = port; }// end of Server // executes the main server loop public void mainServerLoop() throws Exception { // create a listening socket in the specified port. ServerSocket listenSock = new ServerSocket(this.listenPort); // keep servicing the clients while(true) { System.out.println("nWaiting for someone to connect.."); // accept the incoming connection from the client Socket communicationSocket = listenSock.accept(); // the client-to-server stream BufferedReader inFromClient = new BufferedReader(new InputStreamReader( communicationSocket.getInputStream())); // the server-to-client stream DataOutputStream outToClient = new DataOutputStream(communicationSocket.getOutputStream()); // display misc. IPC information System.out.println("n(SRCIP=" + communicationSocket.getInetAddress()+ ", SRCPORT="+communicationSocket.getPort() + ") "+"(DESTIP="+ communicationSocket.getLocalAddress()+", DESTPORT="+ communicationSocket.getLocalPort()+")"); // receive a string from the client String clientMsg = inFromClient.readLine(); System.out.println("nRECEIVED: ""+clientMsg+"" from the client"); // instantiate a date class: used for obtaining the current time Date date = new Date(); // send the current time and date to the client System.out.println("nSENDING: ""+date.toString()+"" to the client"); outToClient.writeBytes(date.toString()); // close the connection to the client communicationSocket.close(); } }// end of mainServerLoop public static void main(String[] args) { // make sure the port number is provided if(args.length != 1) { System.out.println("USAGE: java Server <SERVER PORT #>"); } else { // create an instance of the server class Server server = new Server(Integer.parseInt(args[0])); try { // start receiving client connections System.out.println("nServer started!"); server.mainServerLoop(); } catch(Exception e) { e.printStackTrace(); } } }// end of main }// http://programmingnotes.org/ |
=== 2. CLIENT ===
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 |
// ============================================================================ // Author: K Perkins // Date: Jan 8, 2014 // Taken From: http://programmingnotes.org/ // File: Client.java // Description: This program implements a simple network application in // which a client sends text to a server and the server replies back to the // client. This is the client program, and has the following functionality: // 1. Establish a connection to the server. // 2. Send text to the server. // 3. Recieve text from the server. // 4. Close the connection and exit. // ============================================================================ import java.io.*; import java.net.*; // Compile & Run // javac Client.java // java Client localhost 1234 class Client { // the server IP address protected String svrIpAddr; // the server port number protected int svrPort; /* the initialization constructor * @param ipAddr - the IP address of the server * @param port - the port on which the server is listening */ public Client(String ipAddr, int port) { // save the server's IP address and port number svrIpAddr = ipAddr; svrPort = port; }// end of Client // connects to the server public void connectToServer() throws Exception { // the message to send to the server String msgForServer = "Server, what time is it?"; // the string to store the date and time to be received from the server String dateAndTime = null; // create a socket and use it for connecting to the server Socket clientSocket = new Socket(svrIpAddr, svrPort); // the client-to-server stream DataOutputStream outToServer = new DataOutputStream(clientSocket.getOutputStream()); // the server-to-client stream BufferedReader inFromServer = new BufferedReader(new InputStreamReader(clientSocket.getInputStream())); // write the string to the server System.out.println("nSENDING: ""+msgForServer+"" to the servern"); outToServer.writeBytes(msgForServer+"n"); // get the date and time from the server dateAndTime = inFromServer.readLine(); // print the date and time received from the server System.out.println("RECEIVED: ""+dateAndTime+"" from the servern"); // close the socket clientSocket.close(); }// end of connectToServer public static void main(String args[]) { // check the command line arguments if(args.length < 2) { System.err.println("n** ERROR NOT ENOUGH ARGS!n" +"USAGE: Client <SERVER IP> <SERVER PORT #>n"); } else { // instantiate the client class Client client = new Client(args[0], Integer.parseInt(args[1])); try { // connect to the server client.connectToServer(); } catch(Exception e) { e.printStackTrace(); } } }// end of main }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
The following is sample output.
SERVER OUTPUT:
Server started!
Waiting for someone to connect..
(SRCIP=/127.0.0.1, SRCPORT=46608) (DESTIP=/127.0.0.1, DESTPORT=1234)
RECEIVED: "Server, what time is it?" from the client
SENDING: "Wed Jan 08 18:07:15 PST 2014" to the client
CLIENT OUTPUT:
SENDING: "Server, what time is it?" to the server
RECEIVED: "Wed Jan 08 18:07:15 PST 2014" from the server
Leave a Reply