C++ || Find The Day Of The Week You Were Born Using Functions, String, Modulus, If/Else, & Switch
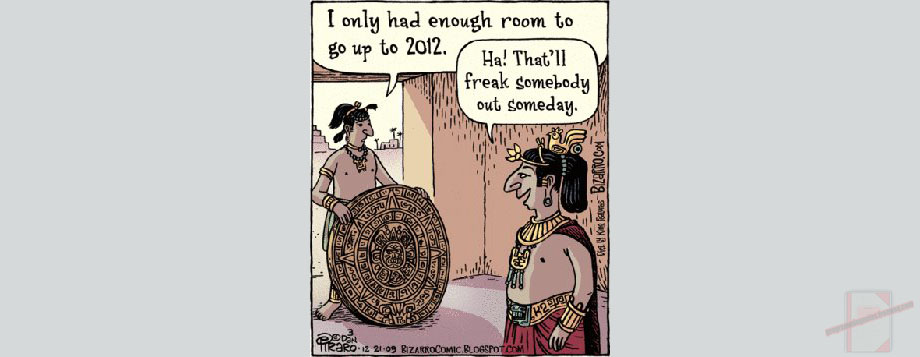
This program displays more practice using functions, modulus, if and switch statements.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Functions
Strings
Modulus
If/Else Statements
Switch Statements
Knowledge of Leap Years
A Calendar
This program prompts the user for their name, date of birth (month, day, year), and then displays information back to them via cout. Once the program obtains selected information from the user, it will use simple math to determine the day of the week in which the user was born, and determine the day of the week their current birthday will be for the current calendar year. The program will also display to the user their current age, along with re-displaying their name back to them.
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 |
#include <iostream> #include <string> using namespace std; // a const variable containing the current year const int CURRENT_YEAR = 2012; // function prototypes string GetNameOfMonth(int numOfMonth); int GetLastTwoDigits(int fourDigitYear); string GetDayOfWeek(int numOfMonth, int lastTwoDigits, int numOfDay, int fourDigitYear); int main () { // declare variables string nameOfMonth = " ", dayOfWeek = " ", userName = " ", dayOfWeekCurrentYear = " " ; int numOfMonth=0, numOfDay=0, fourDigitYear=0, lastTwoDigits=0, lastTwoDigitsCurrentYear; // user inputs data cout << "Please enter your name: "; cin >> userName; cout << "nPlease enter the month in which you were born (between 1 and 12): "; cin >> numOfMonth; // converts the number the user inputted from above ^ // into a literal month name (i.e month #1 = January) nameOfMonth = GetNameOfMonth(numOfMonth); // user inputs data cout << "nPlease enter the day you were born (between 1 and 31): "; cin >> numOfDay; // checks to see if user entered valid data if (numOfDay < 1 || numOfDay > 31) { cout << "nYou have entered an invalid number of day. " << "Please enter a valid number." << endl << endl; exit(1); } // user inputs data cout << "nPlease enter the year you were born (between 1900 and 2099): "; cin >> fourDigitYear; cout << endl << endl; // checks to see if user entered valid data if (fourDigitYear < 1900 || fourDigitYear > 2099) { cout << "You have entered an invalid year. " << "Please enter a valid year." << endl << endl; exit(1); } // gets the las two digits in which the user was born // (i.e 1999: last two digits = 99) lastTwoDigits = GetLastTwoDigits(fourDigitYear); // gets the las two digits of the current year // (i.e 2012: last two digits = 12) lastTwoDigitsCurrentYear = GetLastTwoDigits(CURRENT_YEAR); // finds the day of the week in which the user was born dayOfWeek = GetDayOfWeek(numOfMonth, lastTwoDigits, numOfDay, fourDigitYear); // finds the day of the week the users current birthday will be this year dayOfWeekCurrentYear = GetDayOfWeek(numOfMonth, lastTwoDigitsCurrentYear, numOfDay, CURRENT_YEAR); // display data to user cout << "Hello " << userName <<". Here are some facts about you!" <<endl; cout << "You were born "<< nameOfMonth << numOfDay << " "<< fourDigitYear << endl; cout << "Your birth took place on a " << dayOfWeek << endl; cout << "This year your birthday will take place on a " << dayOfWeekCurrentYear << endl; cout << "You currently are, or will be " << (CURRENT_YEAR - fourDigitYear) << " years old this year!"<<endl; return 0; }// end of main // converts the number the user inputted from above ^ // into a literal month name (i.e month #1 = January) string GetNameOfMonth(int numOfMonth) { string nameOfMonth = " "; // a switch determining what month the user was born switch(numOfMonth) { case 1: nameOfMonth = "January "; break; case 2: nameOfMonth = "February "; break; case 3: nameOfMonth = "March "; break; case 4: nameOfMonth = "April "; break; case 5: nameOfMonth = "May "; break; case 6: nameOfMonth = "June "; break; case 7: nameOfMonth = "July "; break; case 8: nameOfMonth = "August "; break; case 9: nameOfMonth = "September "; break; case 10: nameOfMonth = "October "; break; case 11: nameOfMonth = "November "; break; case 12: nameOfMonth = "December "; break; default: cout << "You have entered an invalid number of month. nPlease enter a valid number.n" << "Program Terminating.."; exit(1); break; }// end of switch return nameOfMonth ; }// end of GetNamOfMonth // gets the las two digits in which the user was born/current year int GetLastTwoDigits(int fourDigitYear) { if (fourDigitYear >= 1900 && fourDigitYear <= 1999) { return (fourDigitYear - 1900); } else { return (fourDigitYear - 2000); } }// end of GetLastTwoDigits // finds the day of the week in which the user was born/current year string GetDayOfWeek(int numOfMonth, int lastTwoDigits, int numOfDay, int fourDigitYear) { string dayOfWeek = " "; int Total = 0, valueOfMonth = 0; if (numOfMonth == 1) valueOfMonth = 1; else if (numOfMonth == 2) valueOfMonth = 4; else if (numOfMonth == 3) valueOfMonth = 4; else if (numOfMonth == 4) valueOfMonth = 0; else if (numOfMonth == 5) valueOfMonth = 2; else if (numOfMonth == 6) valueOfMonth = 5; else if (numOfMonth == 7) valueOfMonth = 0; else if (numOfMonth == 8) valueOfMonth = 3; else if (numOfMonth == 9) valueOfMonth = 6; else if (numOfMonth == 10) valueOfMonth = 1; else if (numOfMonth == 11) valueOfMonth = 4; else if (numOfMonth == 12) valueOfMonth = 6; else cout <<"nError"; Total = lastTwoDigits / 4; Total += lastTwoDigits; Total += numOfDay; Total += valueOfMonth; // uses the 'Total' variable from above to determine the day // of the week in which you were born if ((fourDigitYear > 2000)&&((numOfMonth != 1)&&(numOfMonth != 2))&&(fourDigitYear != 2004)) { Total = Total + 6; } else if ((fourDigitYear < 2000) && ((numOfMonth == 1) || (numOfMonth == 2))) { Total = Total + 7; } else if ((fourDigitYear == 2000) && ((numOfMonth == 1) || (numOfMonth == 2))) { Total = Total -2; } else if ((fourDigitYear == 2004||fourDigitYear == 2000)&&((numOfMonth != 1)&&(numOfMonth != 2))) { Total = Total -1; } else if ((fourDigitYear % 4 == 0) && (fourDigitYear % 100 == lastTwoDigits) && (fourDigitYear % 400 == lastTwoDigits)) { Total = Total-2; } else if ((fourDigitYear > 2000)&&((numOfMonth == 1)||(numOfMonth == 2))&&(fourDigitYear != 2004)) { Total = Total + 6; } // uses the 'Total' variable from above to determine the day // of the week in which you were born/when you birthday will be this year if (Total % 7 == 1) dayOfWeek = "Sunday"; else if (Total % 7 == 2) dayOfWeek = "Monday"; else if (Total % 7 == 3) dayOfWeek = "Tuesday"; else if (Total % 7 == 4) dayOfWeek = "Wednesday"; else if (Total % 7 == 5) dayOfWeek = "Thursday"; else if (Total % 7 == 6) dayOfWeek = "Friday"; else if (Total % 7 == 0) dayOfWeek = "Saturday"; else cout<< "nError"; return dayOfWeek; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled five separate times to display the different outputs its able to produce
Please enter your name: MyProgrammingNotes
Please enter the month in which you were born (between 1 and 12): 1
Please enter the day you were born (between 1 and 31): 1
Please enter the year you were born (between 1900 and 2099): 2012Hello MyProgrammingNotes. Here are some facts about you!
You were born January 1 2012
Your birth took place on a Sunday
This year your birthday will take place on a Sunday
You currently are, or will be 0 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 4
Please enter the day you were born (between 1 and 31): 2
Please enter the year you were born (between 1900 and 2099): 1957Hello Name. Here are some facts about you!
You were born April 2 1957
Your birth took place on a Tuesday
This year your birthday will take place on a Monday
You currently are, or will be 55 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 5
Please enter the day you were born (between 1 and 31): 7
Please enter the year you were born (between 1900 and 2099): 1999Hello Name. Here are some facts about you!
You were born May 7 1999
Your birth took place on a Friday
This year your birthday will take place on a Monday
You currently are, or will be 13 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 8
Please enter the day you were born (between 1 and 31): 4
Please enter the year you were born (between 1900 and 2099): 1983Hello Name. Here are some facts about you!
You were born August 4 1983
Your birth took place on a Thursday
This year your birthday will take place on a Saturday
You currently are, or will be 29 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 6
Please enter the day you were born (between 1 and 31): 7
Please enter the year you were born (between 1900 and 2099): 2987You have entered an invalid year. Please enter a valid year.
Press any key to continue . . .
Leave a Reply