JavaScript || Swap Two HTML Element Locations In Dom Tree Using Vanilla JavaScript
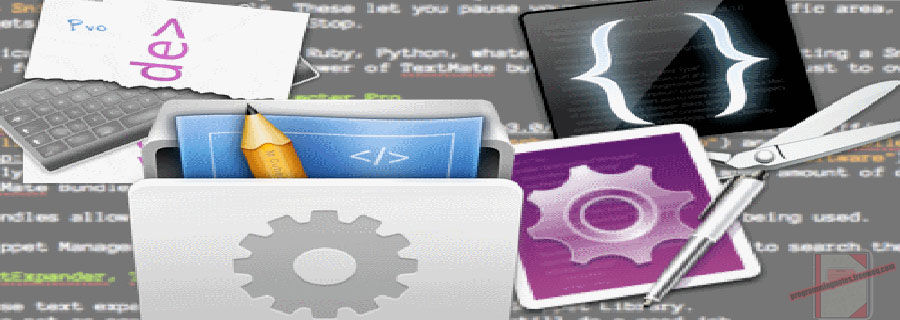
The following is a module with functions that demonstrates a simple way how to swap two HTML element locations in the Dom tree using JavaScript.
This method retains any existing event listeners.
1. Swap Element Locations
The example below demonstrates how to swap two HTML element locations.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
<!-- // Swap Element Locations. --> <div class="container"> <p>some irrelevant text 1</p> <p>some irrelevant text 2</p> <p class="first">I'm first</p> <div> <p class="second">I'm second</p> <p>some irrelevant text outside 1</p> <p>some irrelevant text outside 2</p> </div> </div> <script> (() => { let first = document.querySelector('.first'); let second = document.querySelector('.second'); let container = document.querySelector('.container'); console.log('=== Before Swap ==='); console.log(container.innerHTML); // Swap the two locations Utils.swapLocations(first, second); console.log('=== After Swap ==='); console.log(container.innerHTML); })(); </script> <!-- // expected output: /* === Before Swap === <p>some irrelevant text 1</p> <p>some irrelevant text 2</p> <p class="first">I'm first</p> <div> <p class="second">I'm second</p> <p>some irrelevant text outside 1</p> <p>some irrelevant text outside 2</p> </div> === After Swap === <p>some irrelevant text 1</p> <p>some irrelevant text 2</p> <p class="second">I'm second</p> <div> <p class="first">I'm first</p> <p>some irrelevant text outside 1</p> <p>some irrelevant text outside 2</p> </div> */ --> |
2. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 28, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: swapLocations * USE: Swaps two Javascript element locations in the DOM tree. * @param elementX: The first Javascript element to swap. * @param elementY: The second Javascript element to swap. * @return: N/A. */ exposed.swapLocations = (elementX, elementY) => { let parentY = elementY.parentNode; let nextY = elementY.nextSibling; if (nextY === elementX) { parentY.insertBefore(elementX, elementY); } else { elementX.parentNode.insertBefore(elementY, elementX); if (nextY) { parentY.insertBefore(elementX, nextY); } else { parentY.appendChild(elementX); } } } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply