JavaScript || How To Calculate Nth Catalan Number Using Vanilla JavaScript
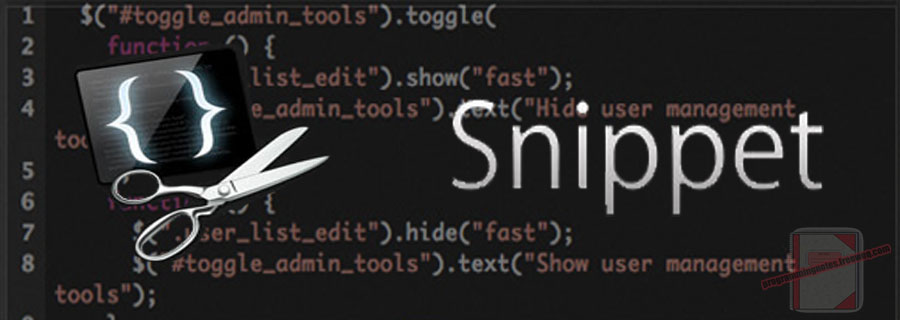
In combinatorial mathematics, a Catalan number forms a sequence of natural numbers that occur in various counting problems. It can be used to determine how many different ways to split something up.
The following is a module with functions which demonstrates how to calculate nth Catalan number.
1. Calculate Nth Catalan Number
The example below demonstrates the use of ‘Utils.catalan‘ to calculate nth Catalan number.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Calculate Nth Catalan Number <script> (() => { for (let number = 0; number <= 10; ++number) { console.log(`Number: ${number}, Catalan: ${Utils.catalan(number)}`); } })(); </script> // expected output: /* Number: 0, Catalan: 1 Number: 1, Catalan: 1 Number: 2, Catalan: 2 Number: 3, Catalan: 5 Number: 4, Catalan: 14 Number: 5, Catalan: 42 Number: 6, Catalan: 132 Number: 7, Catalan: 429 Number: 8, Catalan: 1430 Number: 9, Catalan: 4862 Number: 10, Catalan: 16796 */ |
2. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 6, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: catalan * USE: Calculates the catalan number for the number in question * @param n: The number to calculate the catalan number. * @return: The catalan number for the number in question. */ exposed.catalan = (n) => { n = Math.max(0, n); let result = Array(n + 1); result[0] = result[1] = 1; for (let x = 2; x <= n; ++x) { result[x] = 0; for (let y = 0; y < x; ++y) { result[x] += result[y] * result[x - y - 1]; } } return result[n]; } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply