C++ || How To Find All Combinations Of Well-Formed Brackets Using C++
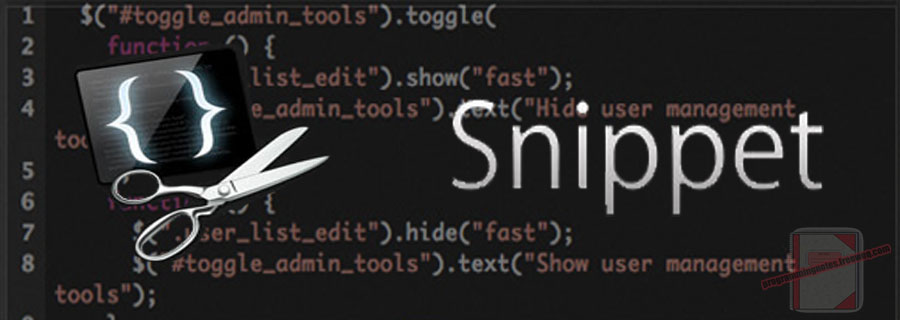
The following is a program with functions which demonstrates how to find all combinations of well-formed brackets.
The task is to write a function Brackets(int n) that prints all combinations of well-formed brackets from 1…n. For example, Brackets(3), the output would be:
()
(()) ()()
((())) (()()) (())() ()(()) ()()()
The number of possible combinations is the Catalan number of N pairs C(n).
1. Find All Well-Formed Brackets
The example below demonstrates the use of the ‘brackets‘ function to find all the well-formed bracket combinations.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 7, 2020 // Taken From: http://programmingnotes.org/ // File: generateParenthesis.cpp // Description: Generate all combinations of well-formed brackets from 1…n // ============================================================================ #include <iostream> #include <vector> #include <string> struct result { int pair; std::string combination; }; struct symbols { std::string open; std::string close; }; std::string buildBrackets(std::string output, int open, int close, int pair, symbols symbols) { if ((open == pair) && (close == pair)) { return output; } std::string result = ""; if (open < pair) { std::string openCombo = buildBrackets(output + symbols.open, open + 1, close, pair, symbols); if (openCombo.length() > 0) { result += (result.length() > 0 ? ", " : "") + openCombo; } } if (close < open) { std::string closeCombo = buildBrackets(output + symbols.close, open, close + 1, pair, symbols); if (closeCombo.length() > 0) { result += (result.length() > 0 ? ", " : "") + closeCombo; } } return result; } /** * FUNCTION: brackets * USE: Returns all combinations of well-formed brackets from 1...n * @param pairs: The number of bracket combinations to generate. * @param open: Optional. The 'open bracket' symbol. * @param close: Optional. The 'close bracket' symbol. * @return: An array of bracket combination info. */ std::vector<result> brackets(int pairs, std::string open = "(", std::string close = ")") { std::vector<result> results; symbols symbols{open, close}; for (int pair = 1; pair <= pairs; ++pair) { result result{pair, buildBrackets("", 0, 0, pair, symbols)}; results.push_back(result); } return results; } int main() { std::vector<result> results = brackets(4); for (auto const &result : results) { std::cout << "Pair: " << result.pair << ", Combination: " << result.combination << std::endl; } std::cin.get(); return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Pair: 1, Combination: ()
Pair: 2, Combination: (()), ()()
Pair: 3, Combination: ((())), (()()), (())(), ()(()), ()()()
Pair: 4, Combination: (((()))), ((()())), ((())()), ((()))(), (()(())), (()()()), (()())(), (())(()), (())()(), ()((())), ()(()()), ()(())(), ()()(()), ()()()()
Leave a Reply