JavaScript || How To Get A Variable Name As A String Using Vanilla JavaScript
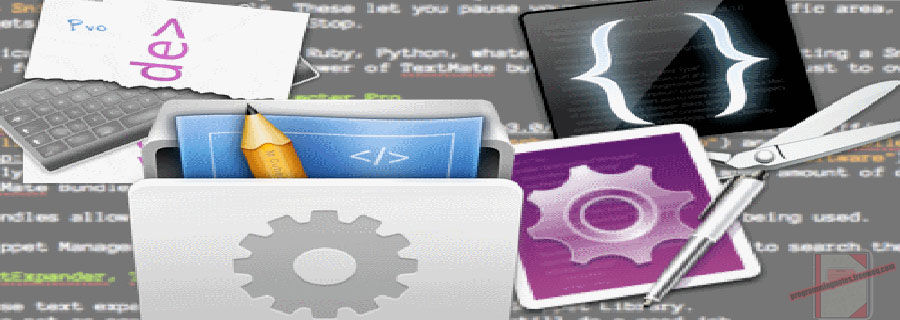
The following is a module with functions which demonstrates how to get the name of a variable as a string using vanilla JavaScript.
1. Simple Variable
The example below demonstrates the use of ‘Utils.nameOf‘ to get name of a simple variable.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Simple Variable <script> (() => { // Get the name of the variable let simpleString = 'My Programming Notes'; console.log( Utils.nameOf({ simpleString }) ); })(); </script> // expected output: /* simpleString */ |
2. Array
The example below demonstrates the use of ‘Utils.nameOf‘ to get the name of an array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// Array <script> (() => { // Mixed array let mixedArray = [ [0, 'Aluminium', 0, 'Francis'], [1, 'Argon', 1, 'Ada'], [2, 'Brom', 2, 'John'], [3, 'Cadmium', 9, 'Marie'], [4, 'Fluor', 12, 'Marie'], [5, 'Gold', 1, 'Ada'], [6, 'Kupfer', 4, 'Ines'], [7, 'Krypton', 4, 'Joe'], [8, 'Sauerstoff', 0, 'Marie'], [9, 'Zink', 5, 'Max'] ]; console.log( Utils.nameOf({ mixedArray }) ); })(); </script> // expected output: /* mixedArray */ |
3. Function
The example below demonstrates the use of ‘Utils.nameOf‘ to get the name of a function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Function <script> (() => { // Function function multiplyFunction(x, y) { return x * y; } console.log( Utils.nameOf({ multiplyFunction }) ); })(); </script> // expected output: /* multiplyFunction */ |
4. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 31, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: nameOf * USE: Returns the name of a variable. * @param expression: An object containing the variable to get the name of. * @return: The name of a variable. */ exposed.nameOf = (expression) => { if (expression !== Object(expression) || typeof expression === 'function' || Array.isArray(expression)) { throw new Error(`Unable to determine name. '${expression}' is not an object`); } return Object.keys(expression)[0]; } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply