VB.NET || How To Save, Open & Read File As A Byte Array & Memory Stream Using VB.NET
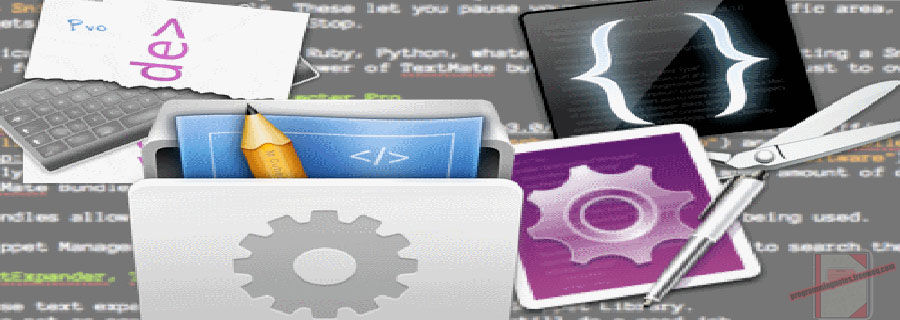
The following is a module with functions which demonstrates how to save, open and read a file as a byte array and memory stream using VB.NET.
1. Read File – Byte Array
The example below demonstrates the use of ‘Utils.ReadFile‘ to read a file as a byte array.
1 2 3 4 5 6 |
' Byte Array Dim path = $"path-to-file\file.extension" Dim fileByteArray = Utils.ReadFile(path) ' Do something with the byte array |
2. Read File – Memory Stream
The example below demonstrates the use of ‘Utils.ReadFile‘ to read a file as a memory stream.
1 2 3 4 5 6 |
' Memory Stream Dim path = $"path-to-file\file.extension" Using fileMS = New System.IO.MemoryStream(Utils.ReadFile(path)) ' Do something with the stream End Using |
3. Save File – Byte Array
The example below demonstrates the use of ‘Utils.SaveFile‘ to save the contents of a byte array to a file.
1 2 3 4 5 6 |
' Byte Array Dim path = $"path-to-file\file.extension" Dim bytes As Byte() Utils.SaveFile(path, bytes) |
4. Save File – Memory Stream
The example below demonstrates the use of ‘Utils.SaveFile‘ to save the contents of a memory stream to a file.
1 2 3 4 5 6 |
' Memory Stream Dim path = $"path-to-file\file.extension" Dim fileMS As System.IO.MemoryStream Utils.SaveFile(path, fileMS.ToArray) |
5. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 5, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Reads a file at the given path into a byte array ''' </summary> ''' <param name="filePath">The path of the file to be read</param> ''' <param name="shareOption">Determines how the file will be shared by processes</param> ''' <returns>The contents of the file as a byte array</returns> Public Function ReadFile(filePath As String, Optional shareOption As System.IO.FileShare = System.IO.FileShare.None) As Byte() Dim fileBytes() As Byte Using fs = New System.IO.FileStream(filePath, System.IO.FileMode.Open, System.IO.FileAccess.Read, shareOption) ReDim fileBytes(CInt(fs.Length - 1)) fs.Read(fileBytes, 0, fileBytes.Length) End Using Return fileBytes End Function ''' <summary> ''' Saves a file at the given path with the contents of the byte array ''' </summary> ''' <param name="filePath">The path of the file to be saved</param> ''' <param name="fileBytes">The byte array containing the file contents</param> ''' <param name="shareOption">Determines how the file will be shared by processes</param> Public Sub SaveFile(filePath As String, fileBytes As Byte(), Optional shareOption As System.IO.FileShare = System.IO.FileShare.None) Using fs = New System.IO.FileStream(filePath, System.IO.FileMode.Create, System.IO.FileAccess.Write, shareOption) fs.Write(fileBytes, 0, fileBytes.Length) End Using End Sub End Module End Namespace ' http://programmingnotes.org/ |
6. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 5, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Module Program Sub Main(args As String()) Try Dim path = $"" Dim fileByteArray = Utils.ReadFile(path, System.IO.FileShare.None) Display("") Using fileMS = New System.IO.MemoryStream(Utils.ReadFile(path, System.IO.FileShare.None)) ' Do something with the stream End Using Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply