VB.NET || How To Generate, Create & Read A QR Code Using VB.NET
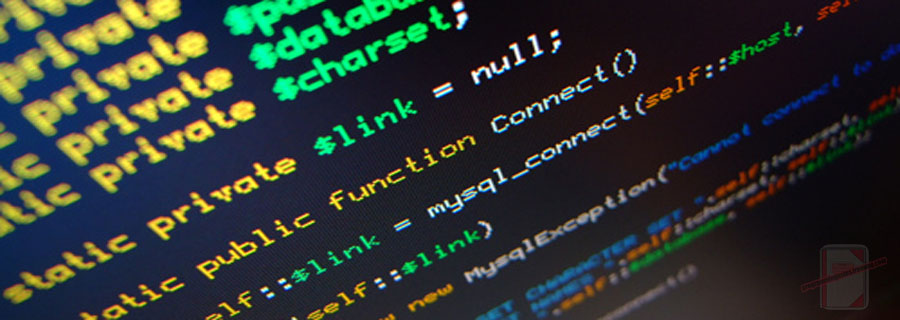
The following is a module with functions which demonstrates how to generate, create and read a QR code using VB.NET.
The functions demonstrated on this page has the ability to create and encode a string to a QR code byte array, and another function to read and decode a byte array QR code to a string.
The following functions use ZXing.Net to create and read QR codes.
Note: To use the functions in this module, make sure you have the ‘ZXing.Net‘ package installed in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Manage NuGet Packages….‘, then type ‘ZXing.Net‘ in the search box, and install the package titled ZXing.Net in the results Tab.
1. Create QR Code – Encode
The example below demonstrates the use of ‘Utils.QRCode.Create‘ to create a QR code byte array from string data.
The optional function parameters allows you to specify the QR code height, width and margin.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Create QR Code - Encode ' Data to encode Dim data = "https://www.programmingnotes.org/" ' Encode data to a QR code byte array Dim bytes = Utils.QRCode.Create(data) ' Display bytes length Debug.Print($"Length: {bytes.Length}") ' expected output: ' Length: 1959 |
2. Read QR Code – Decode
The example below demonstrates the use of ‘Utils.QRCode.Read‘ to read a QR code byte array and decode its contents to a string.
1 2 3 4 5 6 7 8 9 |
' Read QR Code - Decode ' Read QR as byte array Dim qrBytes As Byte() ' Decode QR code to a string Dim data = Utils.QRCode.Read(qrBytes) ' ... Do something with the result string |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 28, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace QRCode Public Module modQRCode ''' <summary> ''' Converts a string and encodes it to a QR code byte array ''' </summary> ''' <param name="data">The data to encode</param> ''' <param name="height">The height of the QR code</param> ''' <param name="width">The width of the QR code</param> ''' <param name="margin">The margin around the QR code</param> ''' <returns>The byte array of the data encoded into a QR code</returns> Public Function Create(data As String, Optional height As Integer = 100 _ , Optional width As Integer = 100, Optional margin As Integer = 0) As Byte() Dim bytes As Byte() = Nothing Dim barcodeWriter = New ZXing.BarcodeWriter With { .Format = ZXing.BarcodeFormat.QR_CODE, .Options = New ZXing.QrCode.QrCodeEncodingOptions With { .Height = height, .Width = width, .Margin = margin } } Using image = barcodeWriter.Write(data) Using stream = New System.IO.MemoryStream image.Save(stream, System.Drawing.Imaging.ImageFormat.Png) bytes = stream.ToArray End Using End Using Return bytes End Function ''' <summary> ''' Converts a QR code and decodes it to its string data ''' </summary> ''' <param name="bytes">The QR code byte array</param> ''' <returns>The string data decoded from the QR code</returns> Public Function Read(bytes As Byte()) As String Dim result = String.Empty Using stream = New System.IO.MemoryStream(bytes) Using image = System.Drawing.Image.FromStream(stream) Dim barcodeReader = New ZXing.BarcodeReader With { .AutoRotate = True, .TryInverted = True, .Options = New ZXing.Common.DecodingOptions With { .TryHarder = True, .PossibleFormats = New ZXing.BarcodeFormat() { ZXing.BarcodeFormat.QR_CODE } } } Dim decoded = barcodeReader.Decode(CType(image, System.Drawing.Bitmap)) If decoded IsNot Nothing Then result = decoded.Text End If End Using End Using Return result End Function End Module End Namespace End Namespace ' http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 28, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Sub Main(args As String()) Try ' Data to encode Dim data = "https://www.programmingnotes.org/" ' Encode data to a QR code byte array Dim bytes = Utils.QRCode.Create(data) Display($"Length: {bytes.Length}") ' Decode QR code to a string Dim result = Utils.QRCode.Read(bytes) Display($"Result: {result}") Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply