C++ || Input/Output – Find The Average of The Numbers Contained In a Text File Using an Array/Bubble Sort
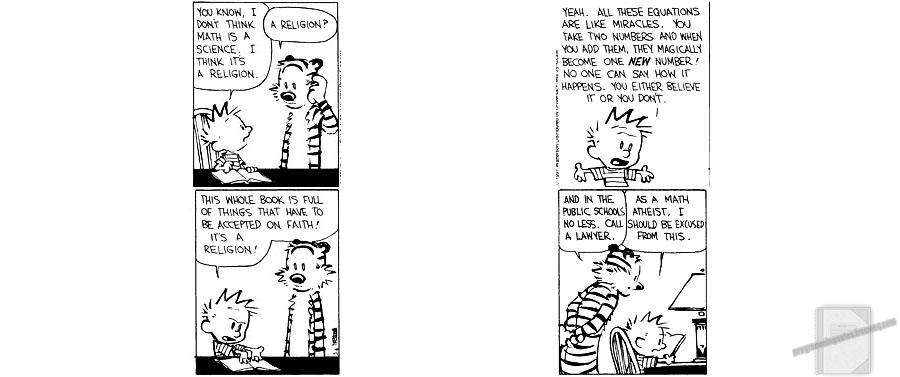
This program highlights more practice using text files and arrays. This program is very similar to one which was previously discussed on this site, but unlike that program, this implementation omits the highest/lowest values which are found within the array via a sort.
The previously discussed program works almost as well as the current implementation, but where it fails is when the data which is being entered into the program contains multiple values of the same type. For example, using the previously discussed method to obtain the average by omitting the highest/lowest entries found within an array, if the array contained the numbers:
1, 2, 3, 3, 3, 2, 2, 1
The previous implementation would mark 1 as being the lowest number (which is correct) and it would mark 3 as being the highest number (which is also correct). The area where it fails is when it omits the highest and lowest scores found within the array. The program will skip over ALL of the numbers contained within the array which equal to 1 and 3, thus resulting in the program obtaining the wrong answer.
To illustrate, before the previous program computes its adjusted average scores, it will not only omit just 1 and 3 from the array, but it will also omit all of the 1’s and 3’s from the list, resulting in our array looking like this:
2, 2, 2
When you are finding the average of a list of numbers by omitting the highest/lowest scores, you don’t want to omit ALL of the values which may equal said numbers, but merely just the highest (last element in the array) and lowest (first element in the array) scores.
So if the previous implementation has subtle issues, why is it on this site? The previous program illustrates very well the process of finding the highest/lowest integers found within an array. It also works flawlessly for data in which there is non repeating values found within a list (i.e 1,2,3,4,5,23,6). So if you know you are reading in from a file in which there are non repeating values, the previous implementation works well. Often times though, developers do not know what type of data the incoming files will contain, so this current implementation is a better way to go, especially if it is not known exactly how many numbers are contained within a file.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Fstream
Ifstream
Ofstream
Working With Files
While Loops
For Loops
Bubble Sort
Basic Math - Finding The Average
The data file that is used in this example can be downloaded here.
Note: In order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 |
#include <iostream> #include <fstream> using namespace std; // function prototype float FindOmittedAverage(int numbers[], int counter); int main() { // declare variables ifstream infile; ofstream outfile; float average=0; float sum =0; int highestNumber = -999999; int lowestNumber = 99999999; int numbers[100]; // open the input file in which you will read data from infile.open("INPUT_Numbers_ programmingnotes_freeweq_com.txt"); if(infile.fail()) { cout<<"nInput file not found!n"; exit(1); // there was an error, program exits } // open the output file in which the compiled data will be saved to outfile.open("OUTPUT_Averages_ programmingnotes_freeweq_com.txt"); if(outfile.fail()) { cout <<"There was an error creating the output file, press enter to terminate program."; exit(1); // there was an error, program exits } // display data to screen via cout cout <<"The numbers countained in the input file are: "; // saves data to the outfile. Notice the declaration to // save data to the outfile is the same as the ^ above cout // statment outfile <<"The numbers countained in the input file are: "; // incoming data from the file will be stored in an int array // so this counter will increment the array index every time the // program finds a new value inside the file to store inside the array int counter =0; // this while loop will read in data from the file until it reaches the end of the file // (i.e until there are no more number found within the file) // this process also stored the numbers inside the "numbers" array while(infile >> numbers[counter]) { // 'sum' variable adds the incoming numbers together // computing the sum sum += numbers[counter]; // finds the highest number contained within the incoming file if( numbers[counter] > highestNumber) { highestNumber = numbers[counter]; } // finds the lowest number contained within the incoming file if (numbers[counter] < lowestNumber) { lowestNumber = numbers[counter]; } // displays the currently found number from the file to stdout cout << numbers[counter] << ", "; // saves the currently found number from the file to the output file outfile << numbers[counter]<< ", "; ++counter; } // always close your file after your done using them infile.close(); // display the highest found number to stdout cout<< "nnThe highest and lowest numbers contained in the file are: nHighest: " << highestNumber<<"nLowest: "<<lowestNumber<<endl; // saves the highest found number to the output file outfile<< "nnThe highest and lowest numbers contained in the file are: nHighest: " << highestNumber<<"nLowest: "<<lowestNumber<<endl; // using the sum found from the above while loop, we compute the average average = sum/(counter); // display/save the average of the numbers which were contained in the // file to stdout and the output file cout<<"nThe average of the "<<counter<<" numbers contained in the file is: "<<average<<endl; outfile<<"nThe average of the "<<counter<<" numbers contained in the file is: "<<average<<endl; // function declaration which finds the omitted average of the found numbers // from the file average = FindOmittedAverage(numbers,counter); // display/save the omitted average of the numbers which were contained in the // file to stdout and the output file cout<<"nThe average of the "<<counter<<" numbers contained in the file omitting the highest and lowest scores is: "<<average<<endl; outfile<<"nThe average of the "<<counter<<" numbers contained in the file omitting the highest and lowest scores is: "<<average<<endl; // closes the outfile once we are done using it outfile.close(); return 0; } // function takes in the 'numbers' array, and 'counter' variable from // the main function as parameters, and computes the average, omitting // the highest and lowest numbers found within the array float FindOmittedAverage(int numbers[], int counter) { float sum = 0; float avg = 0; // this is a 'bubble sort' which will sort the numbers // contained within the array, from lowest to the highest // i.e (1,2,3,4,5,6,7,8) for(int iteration = 1; iteration < counter; iteration++) { for(int index = 0; index < counter - iteration; index++) { // if the previous value in the array is bigger than the next, then swap them if(numbers[index]> numbers[index+1]) { int temp = numbers[index]; numbers[index] = numbers[index+1]; numbers[index+1]= temp; } } }// end of sort // after the sorting is complete, set the highest // and lowest elements in the array to zero numbers[0]=0; numbers[counter-1]=0; // find the sum of the newly sorted array // with the highest and lowest entries being deleted (set to zero) for(int i = 0; i < counter; i++) { sum += numbers[i]; } // compute the average avg = sum / (counter-2); // return the average back to main return avg; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Remember to include the input file)
The numbers countained in the input file are: 12, 45, 23, 46, 11, -5, 23, 33, 50, 17, 13, 25, 15, 50,
The highest and lowest numbers contained in the file are:
Highest: 50
Lowest: -5The average of the 14 numbers contained in the file is: 25.5714
The average of the 14 numbers contained in the file omitting the highest and lowest scores is: 26.0833
Leave a Reply