JavaScript || How To Add & Populate A Dropdown List With An Array Using Vanilla JavaScript
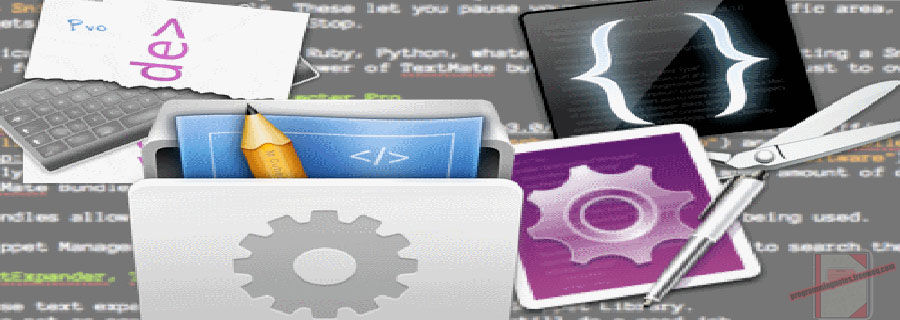
The following is a module with functions which demonstrates how to add and populate a dropdown select list with an array of items using vanilla JavaScript.
The function demonstrated on this page allows to add items from an array into a dropdown list, as well as adding option groups and custom data attributes.
1. Basic Usage
The example below demonstrates the use of ‘Utils.bindDropdown‘ to bind the data in a simple array to a dropdown list.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<!-- Basic Usage --> <!-- Declare dropdown --> <select id="dropdown"></select> <script> (() => { // Declare dropdown items let items = ['Kenneth', 'Jennifer', 'Lynn', 'Sole']; // Get dropdown let dropdown = document.querySelector('#dropdown'); // Add dropdown items Utils.bindDropdown(dropdown, items); })(); </script> |
Sample:
2. Additional Options
The example below demonstrates the use of ‘Utils.bindDropdown‘ to bind the data in an options initialization array to a dropdown list.
In this example, the text and values are specified, as well as custom option groups, the selected option value, and custom data attributes.
You can add any properties you wish to the object, and it will be added to the dropdown list option!
Note: Option groups are not required using this notation, as indicated by the ‘Ungrouped Items’.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<!-- Additional Options --> <!-- Declare dropdown --> <select id="dropdown"></select> <script> (() => { // Declare dropdown items let items = [ {text: 'Kenneth', value: 31, group: 'Parents'}, {text: 'Jennifer', value: 28, group: 'Parents', selected: true}, {text: 'Lynn', value: 87, group: 'Children'}, {text: 'Sole', value: 91, group: 'Children', 'data-test': 'test attribute'}, {text: 'Ungrouped Item #1', value: 1019}, {text: 'Ungrouped Item #2', value: 8791, disabled: false}, ]; // Get dropdown let dropdown = document.querySelector('#dropdown'); // Add dropdown items Utils.bindDropdown(dropdown, items); })(); </script> |
Sample:
3. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 30, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.js // Description: Javascript that handles general utility functions // ============================================================================ /** * NAMESPACE: Utils * USE: Handles general utility functions. */ var Utils = Utils || {}; (function(namespace) { 'use strict'; // Property to hold public variables and functions let exposed = namespace; /** * FUNCTION: bindDropdown * USE: Binds data to a dropdown list * @param dropdown: Javascript element to add dropdown options * @param items: An array of items to bind to the dropdown list * @return: N/A */ exposed.bindDropdown = (dropdown, items) => { // Clear existing entries while (dropdown.hasChildNodes()) { dropdown.removeChild(dropdown.firstChild); } // Set up option groups for the dropdown const groupKey = 'group'; let groups = {}; for (let item of items) { if (typeof item !== 'object' || !(groupKey in item) || (item[groupKey] in groups)) { continue; } let groupName = item[groupKey]; let optGroup = document.createElement('optgroup'); optGroup.label = groupName; groups[groupName] = { element: optGroup, added: false }; } // Add new entries for (let item of items) { if (typeof item !== 'object') { let text = String(item); item = {}; item.text = text; item.value = text; } let isEmptyOption = true; let isGroupOption = false; let option = document.createElement('option'); for (let prop in item) { if (prop === groupKey) { isGroupOption = true; continue; } let value = item[prop]; if (prop in option) { option[prop] = value; } else { option.setAttribute(prop, value); } isEmptyOption = false; } // Add option to the dropdown if (isGroupOption) { let groupInfo = groups[item[groupKey]]; let optGroup = groupInfo.element; if (!groupInfo.added) { dropdown.appendChild(optGroup); groupInfo.added = true; } if (!isEmptyOption) { optGroup.appendChild(option); } } else { dropdown.appendChild(option); } } } (function (factory) { if (typeof define === 'function' && define.amd) { define([], factory); } else if (typeof exports === 'object') { module.exports = factory(); } }(function() { return namespace; })); }(Utils)); // http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of ‘Utils.js‘. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
<!-- // ============================================================================ // Author: Kenneth Perkins // Date: Jan 30, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.html // Description: Demonstrates the use of Utils.js // ============================================================================ --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>My Programming Notes Utils.js Demo</title> <style> .main { text-align:center; margin-left:auto; margin-right:auto; } </style> <!-- // Include module --> <script type="text/javascript" src="./Utils.js"></script> </head> <body> <main class="main"> <div>My Programming Notes Utils.js Demo</div> <!-- Declare dropdown --> <select id="dropdown"></select> </main> <script> document.addEventListener('DOMContentLoaded', function(eventLoaded) { // Get dropdown let dropdown = document.querySelector('#dropdown'); dropdown.addEventListener('change', (e) => { let text = dropdown.options[dropdown.selectedIndex].text; let value = dropdown.value; alert(`Text: ${text} - Value: ${value}`); }); // Declare dropdown items let items = [ {text: 'Kenneth', value: 31, group: 'Parents'}, {text: 'Jennifer', value: 28, group: 'Parents', selected: true}, {text: 'Lynn', value: 87, group: 'Children'}, {text: 'Sole', value: 91, group: 'Children', 'data-test': 'test attribute'}, {text: 'Ungrouped Item #1', value: 1019}, {text: 'Ungrouped Item #2', value: 8791, disabled: false}, ]; //items = ['Kenneth', 'Jennifer', 'Lynn', 'Sole']; //items = [31, 28, 87, 91, 1019, 8791]; // Add dropdown items Utils.bindDropdown(dropdown, items); }); </script> </body> </html><!-- // http://programmingnotes.org/ --> |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply