C++ || How To Shuffle & Randomize An Array/Vector/Container Using C++
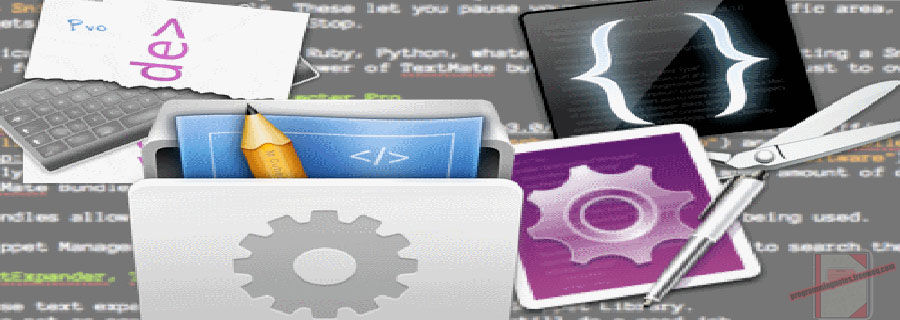
The following is a module with functions which demonstrates how to randomize and shuffle the contents of an array/vector/container using C++.
The following template function is a wrapper for the std::shuffle function.
1. Shuffle – Integer Array
The example below demonstrates the use of ‘Utils::shuffle‘ to randomize an integer array.
123456789101112131415161718192021222324252627
// Shuffle - Integer Array // Declare numbersint numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array sizeint size = sizeof(numbers) / sizeof(numbers[0]); // Get resultsUtils::shuffle(numbers, numbers + size); // Display resultsfor (const auto& item : numbers) { std::cout << item << std::endl;} // example output:/* 28 1991 22 1987 19 2019 2009 31*/
2. Shuffle – String Vector
The example below demonstrates the use of ‘Utils::shuffle‘ to randomize a string vector.
1234567891011121314151617181920
// Shuffle - String Vector // Declare namesstd::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Get resultsUtils::shuffle(names.begin(), names.end()); // Display resultsfor (const auto& item : names) { std::cout << item << std::endl;} // example output:/* Jennifer Sole Kenneth Lynn*/
3. Shuffle – Custom Object Vector
The example below demonstrates the use of ‘Utils::shuffle‘ to randomize a custom object vector.
12345678910111213141516171819202122232425262728293031
// Shuffle - Custom Object Vector // Declare objectstruct Person { int id; std::string name;}; // Declare names std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"}}; // Get resultsUtils::shuffle(people.begin(), people.end()); // Display resultsfor (const auto& item : people) { std::cout << item.id << " - " << item.name << std::endl;} // example output:/* 28 - Jennifer 19 - Jennifer 22 - Kenneth 87 - Lynn 91 - Sole 31 - Kenneth*/
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1234567891011121314151617181920212223242526272829
// ============================================================================// Author: Kenneth Perkins// Date: Feb 9, 2021// Taken From: http://programmingnotes.org/// File: Utils.h// Description: Handles general utility functions// ============================================================================#pragma once#include <random>#include <chrono>#include <algorithm> namespace Utils { /** * FUNCTION: shuffle * USE: Shuffles a collection in the given range [first, last) * @param first: The first position of the sequence to be sorted * @param last: The last position of the sequence to be sorted * @return: N/A */ template<typename RandomIt> void shuffle(RandomIt first, RandomIt last) { auto seed = static_cast<std::mt19937::result_type> ( std::chrono::system_clock::now().time_since_epoch().count() ); std::mt19937 generator(seed); std::shuffle(first, last, generator); }}// http://programmingnotes.org/
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
12345678910111213141516171819202122232425262728293031323334353637383940414243444546474849505152535455565758596061626364656667686970717273747576
// ============================================================================// Author: Kenneth Perkins// Date: Feb 9, 2021// Taken From: http://programmingnotes.org/// File: program.cpp// Description: The following demonstrates the use of the Utils Namespace// ============================================================================#include <iostream>#include <string>#include <exception>#include <vector>#include "Utils.h" void display(const std::string& message); // Declare objectstruct Person { int id; std::string name;}; int main() { try { // Declare numbers int numbers[] = { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Get array size int size = sizeof(numbers) / sizeof(numbers[0]); // Get results Utils::shuffle(numbers, numbers + size); // Display results for (const auto& item : numbers) { std::cout << item << std::endl; } display(""); // Declare names std::vector<std::string> names = { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Get results Utils::shuffle(names.begin(), names.end()); // Display results for (const auto& item : names) { std::cout << item << std::endl; } display(""); // Declare names std::vector<Person> people = { {31, "Kenneth"}, {28, "Jennifer"}, {87, "Lynn"}, {91, "Sole"}, {22, "Kenneth"}, {19, "Jennifer"} }; // Get results Utils::shuffle(people.begin(), people.end()); // Display results for (const auto& item : people) { std::cout << item.id << " - " << item.name << std::endl; } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0;} void display(const std::string& message) { std::cout << message << std::endl;}// http://programmingnotes.org/
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply