C++ || How To Get The Next & Previous Multiple Of A Number Using C++
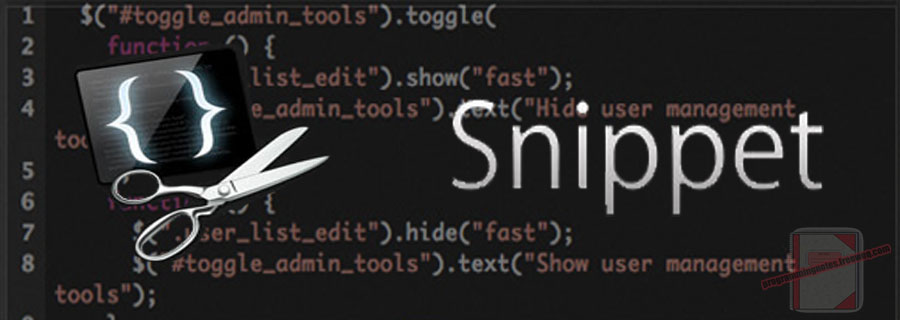
The following is a module with functions which demonstrates how to get the next and previous multiple of a number using C++.
If a number is already a multiple, there is a parameter that allows you to specify if it should be rounded or not.
Click here for sample code demonstrating how to round a number by a specific amount.
1. Get Next Multiple – Include Existing
The example below demonstrates how to round up a number to the next multiple. In this example, if a number is already a multiple, it will not be rounded up to the next multiple.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Get next multiple - include if already a multiple. int multiple = 5; for (int index = 1; index <= 10; ++index) { std::cout << index << " => " << Utils::getNextMultiple(index, multiple) << std::endl; } // expected output: /* 1 => 5 2 => 5 3 => 5 4 => 5 5 => 5 6 => 10 7 => 10 8 => 10 9 => 10 10 => 10 */ |
2. Get Next Multiple – Skip Existing
The example below demonstrates how to round up a number to the next multiple. In this example, if a number is already a multiple, it will be rounded up to the next multiple.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Get next multiple - skip if already a multiple. int multiple = 5; // Set 3rd parameter to true to skip numbers that are already a // multiple to the next value for (int index = 1; index <= 10; ++index) { std::cout << index << " => " << Utils::getNextMultiple(index, multiple, true) << std::endl; } // expected output: /* 1 => 5 2 => 5 3 => 5 4 => 5 5 => 10 6 => 10 7 => 10 8 => 10 9 => 10 10 => 15 */ |
3. Get Previous Multiple – Include Existing
The example below demonstrates how to round down a number to the previous multiple. In this example, if a number is already a multiple, it will not be rounded down to the previous multiple.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Get previous multiple - include if already a multiple. int multiple = 5; for (int index = 10; index >= 1; --index) { std::cout << index << " => " << Utils::getPreviousMultiple(index, multiple) << std::endl; } // expected output: /* 10 => 10 9 => 5 8 => 5 7 => 5 6 => 5 5 => 5 4 => 0 3 => 0 2 => 0 1 => 0 */ |
4. Get Previous Multiple – Skip Existing
The example below demonstrates how to round down a number to the previous multiple. In this example, if a number is already a multiple, it will be rounded down to the previous multiple.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Get previous multiple - skip if already a multiple. int multiple = 5; // Set 3rd parameter to true to skip numbers that are already a // multiple to the previous value for (int index = 10; index >= 1; --index) { std::cout << index << " => " << Utils::getPreviousMultiple(index, multiple, true) << std::endl; } // expected output: /* 10 => 5 9 => 5 8 => 5 7 => 5 6 => 5 5 => 0 4 => 0 3 => 0 2 => 0 1 => 0 */ |
5. Utils Namespace
The following is the Utils.js Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
// ============================================================================ // Author: Kenneth Perkins // Date: Mar 3, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <cmath> namespace Utils { /** * FUNCTION: getNextMultiple * USE: Returns the next multiple of a given number. Unless specified, * it will not round up numbers which are already multiples * @param number: The given number to find the next multiple * @param multiple: The multiple number * @param skipAlreadyMultiple: Boolean that specifies if input numbers which * are already multiples should be skipped to the next multiple or not * @return: The next multiple of the given number */ int getNextMultiple(int number, int multiple, bool skipAlreadyMultiple = false) { int result = 0; if (multiple != 0) { int remainder = (std::abs(number) % multiple); if (!skipAlreadyMultiple && remainder == 0) { result = number; } else if (number < 0 && remainder != 0) { result = -(std::abs(number) - remainder); } else { result = number + (multiple - remainder); } } return result; } /** * FUNCTION: getPreviousMultiple * USE: Returns the previous multiple of a given number. Unless specified, * it will not round down numbers which are already multiples * @param number: The given number to find the previous multiple * @param multiple: The multiple number * @param skipAlreadyMultiple: Boolean that specifies if input numbers which * are already multiples should be skipped to the previous multiple or not * @return: The previous multiple of the given number */ int getPreviousMultiple(int number, int multiple, bool skipAlreadyMultiple = false) { return getNextMultiple(number, multiple, !skipAlreadyMultiple) - multiple; } }// http://programmingnotes.org/ |
6. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
// ============================================================================ // Author: Kenneth Perkins // Date: Mar 3, 2021 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { int multiple = 5; for (int index = 1; index <= 10; ++index) { std::cout << index << " => " << Utils::getNextMultiple(index, multiple) << std::endl; } display(""); // Set 3rd parameter to true to skip numbers that are already a // multiple to the next value for (int index = 1; index <= 10; ++index) { std::cout << index << " => " << Utils::getNextMultiple(index, multiple, true) << std::endl; } display(""); for (int index = 10; index >= 1; --index) { std::cout << index << " => " << Utils::getPreviousMultiple(index, multiple) << std::endl; } display(""); // Set 3rd parameter to true to skip numbers that are already a // multiple to the previous value for (int index = 10; index >= 1; --index) { std::cout << index << " => " << Utils::getPreviousMultiple(index, multiple, true) << std::endl; } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply