C# || How To Resize & Rotate Image, Convert Image To Byte Array, Change Image Format, & Fix Image Orientation Using C#
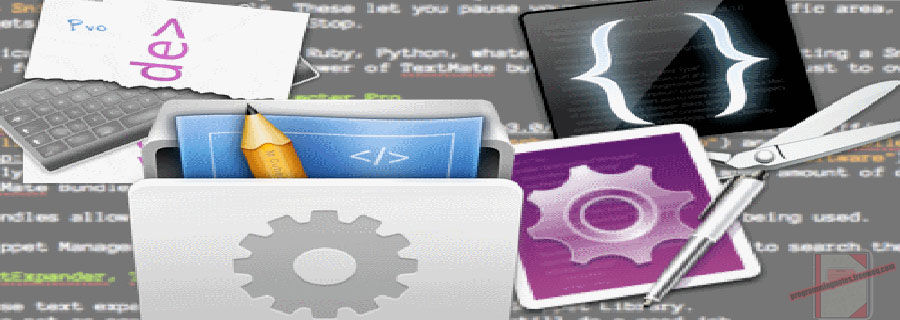
The following is a module with functions which demonstrates how to resize an image, rotate an image to a specific angle, convert an image to a byte array, change an image format, and fix an image orientation Using C#.
Contents
1. Overview
2. Resize & Rotate - Image
3. Resize & Rotate - Byte Array
4. Resize & Rotate - Memory Stream
5. Convert Image To Byte Array
6. Change Image Format
7. Fix Image Orientation
8. Utils Namespace
9. More Examples
1. Overview
To use the functions in this module, make sure you have a reference to ‘System.Drawing‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add References‘, then type ‘System.Drawing‘ in the search box, and add a reference to System.Drawing in the results Tab.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
2. Resize & Rotate – Image
The example below demonstrates the use of ‘Utils.Image.Extensions.Resize‘ and ‘Utils.Image.Extensions.Rotate‘ to resize and rotate an image object.
Resizing and rotating an image returns a newly created image object.
1 2 3 4 5 6 7 8 9 10 11 12 |
// Resize & Rotate - Image using Utils.Image; var path = $@"path-to-file\file.extension"; var image = System.Drawing.Image.FromFile(path); // Resize image 500x500 var resized = image.Resize(new System.Drawing.Size(500, 500)); // Rotate 90 degrees counter clockwise var rotated = image.Rotate(-90); |
3. Resize & Rotate – Byte Array
The example below demonstrates the use of ‘Utils.Image.Extensions.Resize‘ and ‘Utils.Image.Extensions.Rotate‘ to resize and rotate a byte array.
Resizing and rotating an image returns a newly created image object.
1 2 3 4 5 6 7 8 9 10 |
// Resize & Rotate - Byte Array // Read file to byte array byte[] bytes; // Resize image 800x800 var resized = Utils.Image.Extensions.Resize(bytes, new System.Drawing.Size(800, 800)); // Rotate 180 degrees clockwise var rotated = Utils.Image.Extensions.Rotate(bytes, 180); |
4. Resize & Rotate – Memory Stream
The example below demonstrates the use of ‘Utils.Image.Resize‘ and ‘Utils.Image.Rotate‘ to resize and rotate a memory stream.
Resizing and rotating an image returns a newly created image object.
1 2 3 4 5 6 7 8 9 10 |
// Resize & Rotate - Memory Stream // Read file to memory stream var stream = new System.IO.MemoryStream(); // Resize image 2000x2000 var resized = Utils.Image.Extensions.Resize(stream, new System.Drawing.Size(2000, 2000)); // Rotate 360 degrees var rotated = Utils.Image.Extensions.Rotate(stream, 360); |
5. Convert Image To Byte Array
The example below demonstrates the use of ‘Utils.Image.Extensions.ToArray‘ to convert an image object to a byte array.
The optional function parameter allows you to specify the image format.
1 2 3 4 5 6 7 8 9 |
// Convert Image To Byte Array using Utils.Image; var path = $@"path-to-file\file.extension" var image = System.Drawing.Image.FromFile(path); // Convert image to byte array var bytes = image.ToArray(); |
6. Change Image Format
The example below demonstrates the use of ‘Utils.Image.Extensions.ConvertFormat‘ to convert the image object raw format to another format.
Converting an images format returns a newly created image object.
1 2 3 4 5 6 7 8 9 |
// Change Image Format using Utils.Image; var path = $@"path-to-file\file.extension"; var image = System.Drawing.Image.FromFile(path); // Convert image to another format var newImage = image.ConvertFormat(System.Drawing.Imaging.ImageFormat.Png); |
7. Fix Image Orientation
The example below demonstrates the use of ‘Utils.Image.Extensions.FixOrientation‘ to update the image object to reflect its Exif orientation.
When you view an image in a Photo Viewer, it automatically corrects image orientation if it has Exif orientation data.
‘Utils.Image.Extensions.FixOrientation‘ makes it so the loaded image object reflects the correct orientation when rendered according to its Exif orientation data.
1 2 3 4 5 6 7 8 9 |
// Fix Image Orientation using Utils.Image; var path = $@"path-to-file\file.extension"; var image = System.Drawing.Image.FromFile(path); // Correct the image object to reflect its Exif orientation image.FixOrientation(); |
8. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 9, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Collections.Generic; using System.Linq; namespace Utils { namespace Image { public static class Extensions { /// <summary> /// Resizes an image to a specified size /// </summary> /// <param name="source">The image to resize</param> /// <param name="desiredSize">The size to resize the image to</param> /// <param name="preserveAspectRatio">Determines if the aspect ratio should be preserved</param> /// <returns>A new image resized to the specified size</returns> public static System.Drawing.Image Resize(this System.Drawing.Image source, System.Drawing.Size desiredSize, bool preserveAspectRatio = true) { if (desiredSize.IsEmpty) { throw new ArgumentException("Image size cannot be empty", nameof(desiredSize)); } var resizedWidth = desiredSize.Width; var resizedHeight = desiredSize.Height; var sourceFormat = source.RawFormat; source.FixOrientation(); if (preserveAspectRatio) { var sourceWidth = Math.Max(source.Width, 1); var sourceHeight = Math.Max(source.Height, 1); var resizedPercentWidth = desiredSize.Width / (double)sourceWidth; var resizedPercentHeight = desiredSize.Height / (double)sourceHeight; var resizedPercentRatio = Math.Min(resizedPercentHeight, resizedPercentWidth); resizedWidth = System.Convert.ToInt32(sourceWidth * resizedPercentRatio); resizedHeight = System.Convert.ToInt32(sourceHeight * resizedPercentRatio); } var resizedImage = new System.Drawing.Bitmap(resizedWidth, resizedHeight); resizedImage.SetResolution(source.HorizontalResolution, source.VerticalResolution); using (var graphicsHandle = System.Drawing.Graphics.FromImage(resizedImage)) { graphicsHandle.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic; graphicsHandle.CompositingQuality = System.Drawing.Drawing2D.CompositingQuality.HighQuality; graphicsHandle.PixelOffsetMode = System.Drawing.Drawing2D.PixelOffsetMode.HighQuality; graphicsHandle.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality; graphicsHandle.DrawImage(source, 0, 0, resizedWidth, resizedHeight); } if (!sourceFormat.Equals(resizedImage.RawFormat)) { resizedImage = (System.Drawing.Bitmap)resizedImage.ConvertFormat(sourceFormat); } return resizedImage; } /// <summary> /// Resizes an image to a specified size /// </summary> /// <param name="stream">The stream of the image to resize</param> /// <param name="desiredSize">The size to resize the image to</param> /// <param name="preserveAspectRatio">Determines if the aspect ratio should be preserved</param> /// <returns>A new image resized to the specified size</returns> public static System.Drawing.Image Resize(System.IO.Stream stream, System.Drawing.Size desiredSize, bool preserveAspectRatio = true) { System.Drawing.Image image; using (var original = StreamToImage(stream)) { image = Resize(original, desiredSize, preserveAspectRatio: preserveAspectRatio); } return image; } /// <summary> /// Resizes an image to a specified size /// </summary> /// <param name="bytes">The byte array of the image to resize</param> /// <param name="desiredSize">The size to resize the image to</param> /// <param name="preserveAspectRatio">Determines if the aspect ratio should be preserved</param> /// <returns>A new image resized to the specified size</returns> public static System.Drawing.Image Resize(byte[] bytes, System.Drawing.Size desiredSize, bool preserveAspectRatio = true) { System.Drawing.Image image; using (var original = BytesToImage(bytes)) { image = Resize(original, desiredSize, preserveAspectRatio: preserveAspectRatio); } return image; } /// <summary> /// Rotates an image to a specified angle /// </summary> /// <param name="source">The image to resize</param> /// <param name="angle">The angle to rotate the image</param> /// <returns>A new image rotated to the specified angle</returns> public static System.Drawing.Image Rotate(this System.Drawing.Image source, decimal angle) { var sourceFormat = source.RawFormat; source.FixOrientation(); var alpha = angle; while (alpha < 0) { alpha += 360; } var gamma = 90M; var beta = 180 - angle - gamma; var c1 = source.Height; var a1 = Math.Abs((c1 * Math.Sin((double)alpha * Math.PI / 180))); var b1 = Math.Abs((c1 * Math.Sin((double)beta * Math.PI / 180))); var c2 = source.Width; var a2 = Math.Abs((c2 * Math.Sin((double)alpha * Math.PI / 180))); var b2 = Math.Abs((c2 * Math.Sin((double)beta * Math.PI / 180))); var width = System.Convert.ToInt32(b2 + a1); var height = System.Convert.ToInt32(b1 + a2); var rotatedImage = new System.Drawing.Bitmap(width, height); rotatedImage.SetResolution(source.HorizontalResolution, source.VerticalResolution); using (var graphicsHandle = System.Drawing.Graphics.FromImage(rotatedImage)) { graphicsHandle.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic; graphicsHandle.CompositingQuality = System.Drawing.Drawing2D.CompositingQuality.HighQuality; graphicsHandle.PixelOffsetMode = System.Drawing.Drawing2D.PixelOffsetMode.HighQuality; graphicsHandle.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality; graphicsHandle.TranslateTransform(rotatedImage.Width / 2, rotatedImage.Height / 2); graphicsHandle.RotateTransform((float)angle); graphicsHandle.TranslateTransform(-rotatedImage.Width / 2, -rotatedImage.Height / 2); graphicsHandle.DrawImage(source, new System.Drawing.Point((width - source.Width) / 2, (height - source.Height) / 2)); } if (!sourceFormat.Equals(rotatedImage.RawFormat)) { rotatedImage = (System.Drawing.Bitmap)rotatedImage.ConvertFormat(sourceFormat); } return rotatedImage; } /// <summary> /// Rotates an image to a specified angle /// </summary> /// <param name="stream">The stream of the image to resize</param> /// <param name="angle">The angle to rotate the image</param> /// <returns>A new image rotated to the specified angle</returns> public static System.Drawing.Image Rotate(System.IO.Stream stream, decimal angle) { System.Drawing.Image image; using (var original = StreamToImage(stream)) { image = Rotate(original, angle); } return image; } /// <summary> /// Rotates an image to a specified angle /// </summary> /// <param name="bytes">The byte array of the image to rotate</param> /// <param name="angle">The angle to rotate the image</param> /// <returns>A new image rotated to the specified angle</returns> public static System.Drawing.Image Rotate(byte[] bytes, decimal angle) { System.Drawing.Image image; using (var original = BytesToImage(bytes)) { image = Rotate(original, angle); } return image; } /// <summary> /// Converts an image to a byte array /// </summary> /// <param name="source">The image to convert</param> /// <param name="format">The format the byte array image should be converted to</param> /// <returns>The image as a byte array</returns> public static byte[] ToArray(this System.Drawing.Image source, System.Drawing.Imaging.ImageFormat format = null) { if (format == null) { format = source.ResolvedFormat(); if (format.Equals(System.Drawing.Imaging.ImageFormat.MemoryBmp)) { format = System.Drawing.Imaging.ImageFormat.Png; } } byte[] bytes; using (var stream = new System.IO.MemoryStream()) { source.Save(stream, format); bytes = stream.ToArray(); } return bytes; } /// <summary> /// Converts an image to a different ImageFormat /// </summary> /// <param name="source">The image to convert</param> /// <param name="format">The format the image should be changed to</param> /// <returns>The new image converted to the specified format</returns> public static System.Drawing.Image ConvertFormat(this System.Drawing.Image source, System.Drawing.Imaging.ImageFormat format) { var bytes = source.ToArray(format); var image = BytesToImage(bytes); return image; } /// <summary> /// Returns the resolved static image format from an images RawFormat /// </summary> /// <param name="source">The image to get the format from</param> /// <returns>The resolved image format</returns> public static System.Drawing.Imaging.ImageFormat ResolvedFormat(this System.Drawing.Image source) { var format = FindImageFormat(source.RawFormat); return format; } /// <summary> /// Returns all the ImageFormats as an IEnumerable /// </summary> /// <returns>All the ImageFormats as an IEnumerable</returns> public static IEnumerable<System.Drawing.Imaging.ImageFormat> GetImageFormats() { var formats = typeof(System.Drawing.Imaging.ImageFormat) .GetProperties(System.Reflection.BindingFlags.Static | System.Reflection.BindingFlags.Public) .Select(p => (System.Drawing.Imaging.ImageFormat)p.GetValue(null, null)); return formats; } /// <summary> /// Returns the static image format from a RawFormat /// </summary> /// <param name="raw">The ImageFormat to get info from</param> /// <returns>The resolved image format</returns> public static System.Drawing.Imaging.ImageFormat FindImageFormat(System.Drawing.Imaging.ImageFormat raw) { var format = GetImageFormats().FirstOrDefault(x => raw.Equals(x)); if (format == null) { format = System.Drawing.Imaging.ImageFormat.Png; } return format; } /// <summary> /// Update the image to reflect its exif orientation /// </summary> /// <param name="image">The image to update</param> public static void FixOrientation(this System.Drawing.Image image) { var orientationId = 274; if (!image.PropertyIdList.Contains(orientationId)) { return; } var orientation = BitConverter.ToInt16(image.GetPropertyItem(orientationId).Value, 0); var rotateFlip = System.Drawing.RotateFlipType.RotateNoneFlipNone; switch (orientation) { case 1: // Image is correctly oriented rotateFlip = System.Drawing.RotateFlipType.RotateNoneFlipNone; break; case 2: // Image has been mirrored horizontally rotateFlip = System.Drawing.RotateFlipType.RotateNoneFlipX; break; case 3: // Image has been rotated 180 degrees rotateFlip = System.Drawing.RotateFlipType.Rotate180FlipNone; break; case 4: // Image has been mirrored horizontally and rotated 180 degrees rotateFlip = System.Drawing.RotateFlipType.Rotate180FlipX; break; case 5: // Image has been mirrored horizontally and rotated 90 degrees clockwise rotateFlip = System.Drawing.RotateFlipType.Rotate90FlipX; break; case 6: // Image has been rotated 90 degrees clockwise rotateFlip = System.Drawing.RotateFlipType.Rotate90FlipNone; break; case 7: // Image has been mirrored horizontally and rotated 270 degrees clockwise rotateFlip = System.Drawing.RotateFlipType.Rotate270FlipX; break; case 8: // Image has been rotated 270 degrees clockwise rotateFlip = System.Drawing.RotateFlipType.Rotate270FlipNone; break; } if (rotateFlip != System.Drawing.RotateFlipType.RotateNoneFlipNone) { image.RotateFlip(rotateFlip); } } /// <summary> /// Returns an image from a byte array /// </summary> /// <param name="bytes">The bytes representing the image</param> /// <returns>The image from the byte array</returns> public static System.Drawing.Image BytesToImage(byte[] bytes) { return StreamToImage(new System.IO.MemoryStream(bytes)); } /// <summary> /// Returns an image from a stream /// </summary> /// <param name="stream">The stream representing the image</param> /// <returns>The image from the stream</returns> public static System.Drawing.Image StreamToImage(System.IO.Stream stream) { return System.Drawing.Image.FromStream(stream); } } } }// http://programmingnotes.org/ |
9. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 9, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils.Image; public class Program { static void Main(string[] args) { try { var path = ""; // Image var image = System.Drawing.Image.FromFile(path); var resized = image.Resize(new System.Drawing.Size(500, 500)); //var rotated = image.Rotate(-90); // Byte //byte[] bytes; //var resized = Utils.Image.Extensions.Resize(bytes, new System.Drawing.Size(800, 800)); //var rotated = Utils.Image.Extensions.Rotate(bytes, 180); // Stream //var stream = new System.IO.MemoryStream(); //var resized = Utils.Image.Extensions.Resize(stream, new System.Drawing.Size(2000, 2000)); //var rotated = Utils.Image.Extensions.Rotate(stream, 360); var newPath = ""; resized.Save(newPath); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply