C# || How To Serialize & Deserialize XML Using C#
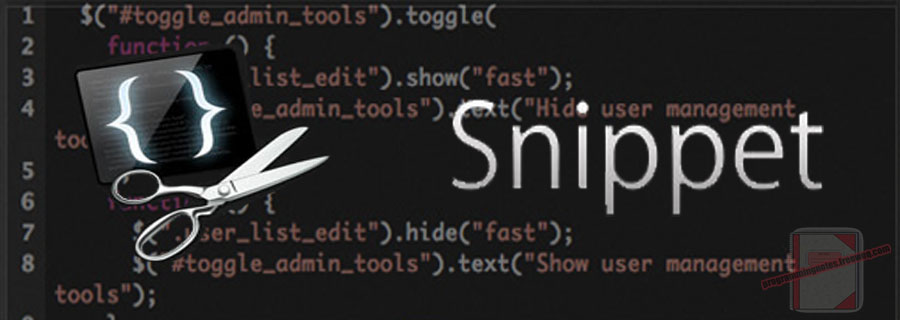
The following is a module with functions which demonstrates how to serialize and deserialize XML using C#.
The following generic functions use System.Xml.Serialization to serialize and deserialize an object.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
1. Serialize – Integer Array
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize an integer array to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// Serialize - Integer Array // Declare array of integers var numbers = new int[] { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Optional: Create an XmlRootAttribute overloaded constructor and set its namespace. var myXmlRootAttribute = new System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") { Namespace = "http://www.microsoft.com" }; // Serialize to XML var numbersXml = Utils.Xml.Serialize(numbers); // Display XML Console.WriteLine(numbersXml); // expected output: /* <?xml version="1.0"?> <ArrayOfInt xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <int>1987</int> <int>19</int> <int>22</int> <int>2009</int> <int>2019</int> <int>1991</int> <int>28</int> <int>31</int> </ArrayOfInt> */ |
2. Serialize – String List
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize a list of strings to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// Serialize - String List // Declare list of strings var names = new List<string>() { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Serialize to XML var namesXml = Utils.Xml.Serialize(names); // Display XML Console.WriteLine(namesXml); // expected output: /* <?xml version="1.0"?> <ArrayOfString xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <string>Kenneth</string> <string>Jennifer</string> <string>Lynn</string> <string>Sole</string> </ArrayOfString> */ |
3. Serialize – Custom Object List
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize a list of custom objects to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 |
// Serialize - Custom Object List [System.Xml.Serialization.XmlRoot(ElementName = "Inventory")] // Optional public class Inventory { [System.Xml.Serialization.XmlArray(ElementName = "Parts")] // Optional public List<Part> Parts { get; set; } } public class Part { [System.Xml.Serialization.XmlElement(ElementName = "PartName")] // Optional public string PartName { get; set; } [System.Xml.Serialization.XmlElement(ElementName = "PartId")] // Optional public int PartId { get; set; } } // Declare list of objects var inventory = new Inventory() { Parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } } }; // Serialize to XML var inventoryXml = Utils.Xml.Serialize(inventory); // Display XML Console.WriteLine(inventoryXml); // expected output: /* <?xml version="1.0"?> <Inventory xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <Parts> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </Parts> </Inventory> */ |
4. Deserialize – Integer Array
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to an integer array.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// Deserialize - Integer Array // Declare Xml array of integers var numbersXml = $@"<?xml version=""1.0""?> <ArrayOfInt xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <int>1987</int> <int>19</int> <int>22</int> <int>2009</int> <int>2019</int> <int>1991</int> <int>28</int> <int>31</int> </ArrayOfInt> "; // Optional: Create an XmlRootAttribute overloaded constructor and set its namespace. var myXmlRootAttribute = new System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") { Namespace = "http://www.microsoft.com" }; // Deserialize from XML to specified type var numbers = Utils.Xml.Deserialize<int[]>(numbersXml); // Display the items foreach (var item in numbers) { Console.WriteLine($"{item}"); } // expected output: /* 1987 19 22 2009 2019 1991 28 31 */ |
5. Deserialize – String List
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to a list of strings.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Deserialize - String List // Declare Xml array of strings var namesXml = $@"<?xml version=""1.0""?> <ArrayOfString xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <string>Kenneth</string> <string>Jennifer</string> <string>Lynn</string> <string>Sole</string> </ArrayOfString> "; // Deserialize from XML to specified type var names = Utils.Xml.Deserialize<List<string>>(namesXml); // Display the items foreach (var item in names) { Console.WriteLine($"{item}"); } // expected output: /* Kenneth Jennifer Lynn Sole */ |
6. Deserialize – Custom Object List
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to a list of objects.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
// Deserialize - Custom Object List [System.Xml.Serialization.XmlRoot(ElementName = "Inventory")] // Optional public class Inventory { [System.Xml.Serialization.XmlArray(ElementName = "Parts")] // Optional public List<Part> Parts { get; set; } } public class Part { [System.Xml.Serialization.XmlElement(ElementName = "PartName")] // Optional public string PartName { get; set; } [System.Xml.Serialization.XmlElement(ElementName = "PartId")] // Optional public int PartId { get; set; } } // Declare Xml array of objects var inventoryXml = $@"<?xml version=""1.0""?> <Inventory xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance""> <Parts> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </Parts> </Inventory> "; // Deserialize from XML to specified type var inventory = Utils.Xml.Deserialize<Inventory>(inventoryXml); // Display the items foreach (var item in inventory.Parts) { Console.WriteLine($"{item.PartId} - {item.PartName}"); } // expected output: /* 1234 - crank arm 1334 - chain ring 1434 - regular seat 1444 - banana seat 1534 - cassette 1634 - shift lever */ |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Xml { /// <summary> /// Serializes the specified value to XML /// </summary> /// <param name="value">The value to serialize</param> /// <param name="root">The XmlRootAttribute that represents the XML root element</param> /// <returns>The value serialized to XML</returns> public static string Serialize<T>(T value , System.Xml.Serialization.XmlRootAttribute root = null) { var xml = string.Empty; var type = value != null ? value.GetType() : typeof(T); var serializer = new System.Xml.Serialization.XmlSerializer(type: type, root: root); using (var stream = new System.IO.MemoryStream()) { serializer.Serialize(stream, value); xml = GetStreamText(stream); } return xml; } /// <summary> /// Deserializes the specified value from XML to Object T /// </summary> /// <param name="value">The value to deserialize</param> /// <param name="root">The XmlRootAttribute that represents the XML root element</param> /// <returns>The value deserialized to Object T</returns> public static T Deserialize<T>(string value , System.Xml.Serialization.XmlRootAttribute root = null) { T obj = default; var serializer = new System.Xml.Serialization.XmlSerializer(type: typeof(T), root: root); using (var stringReader = new System.IO.StringReader(value)) { using (var xmlReader = System.Xml.XmlReader.Create(stringReader)) { obj = (T)serializer.Deserialize(xmlReader); } } return obj; } public static string GetStreamText(System.IO.Stream stream) { try { TryResetPosition(stream); var stmReader = new System.IO.StreamReader(stream); var text = stmReader.ReadToEnd(); return text; } catch { throw; } finally { TryResetPosition(stream); } } private static void TryResetPosition(System.IO.Stream stream) { if (stream.CanSeek) { stream.Position = 0; } } } }// http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { [System.Xml.Serialization.XmlRoot(ElementName = "Inventory")] // Optional public class Inventory { [System.Xml.Serialization.XmlArray(ElementName = "Parts")] // Optional public List<Part> Parts { get; set; } } public class Part { [System.Xml.Serialization.XmlElement(ElementName = "PartName")] // Optional public string PartName { get; set; } [System.Xml.Serialization.XmlElement(ElementName = "PartId")] // Optional public int PartId { get; set; } } static void Main(string[] args) { try { // Declare array of integers var numbers = new int[] { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Create an XmlRootAttribute overloaded constructor and set its namespace. var myXmlRootAttribute = new System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") { Namespace = "http://www.microsoft.com" }; // Serialize to XML var numbersXml = Utils.Xml.Serialize(numbers); // Display XML Display(numbersXml); Display(""); // Declare list of strings var names = new List<string>() { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Serialize to XML var namesXml = Utils.Xml.Serialize(names); // Display XML Display(namesXml); Display(""); // Declare list of objects var inventory = new Inventory() { Parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } } }; // Serialize to XML var inventoryXml = Utils.Xml.Serialize(inventory); // Display XML Display(inventoryXml); Display(""); // Declare Xml array of integers var numbersXmlTest = $@"<?xml version=""1.0""?> <ArrayOfInt xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <int>1987</int> <int>19</int> <int>22</int> <int>2009</int> <int>2019</int> <int>1991</int> <int>28</int> <int>31</int> </ArrayOfInt> "; // Deserialize from XML to specified type var numbersTest = Utils.Xml.Deserialize<int[]>(numbersXmlTest); // Display the items foreach (var item in numbersTest) { Display($"{item}"); } Display(""); // Declare Xml array of strings var namesXmlTest = $@"<?xml version=""1.0""?> <ArrayOfString xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <string>Kenneth</string> <string>Jennifer</string> <string>Lynn</string> <string>Sole</string> </ArrayOfString> "; // Deserialize from XML to specified type var namesTest = Utils.Xml.Deserialize<List<string>>(namesXmlTest); // Display the items foreach (var item in namesTest) { Display($"{item}"); } Display(""); // Declare Xml array of objects var inventoryXmlTest = $@"<?xml version=""1.0""?> <Inventory xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance""> <Parts> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </Parts> </Inventory> "; // Deserialize from XML to specified type var inventoryTest = Utils.Xml.Deserialize<Inventory>(inventoryXmlTest); // Display the items foreach (var item in inventoryTest.Parts) { Display($"{item.PartId} - {item.PartName}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply