C# || How To Add & Use Custom Attributes To Class Properties Using C#
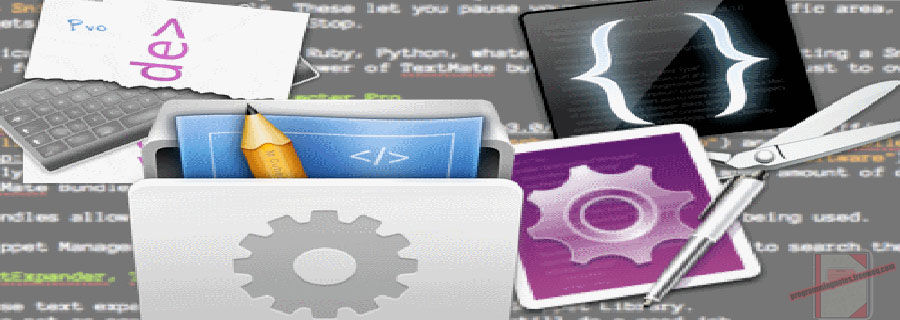
The following is a module with functions which demonstrates how to add and use custom attributes for class properties using C#.
The function demonstrated on this page is a generic extension method which uses reflection to get object property data transformed according to its custom attribute.
1. Custom Attribute
The example below demonstrates the use of ‘Utils.Extensions.GetPropertyData‘ to get object property data transformed according to its custom attribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// Custom Attribute using Utils // Create custom attribute class public class DateFormatAttribute : System.Attribute { public DateFormat Format { get; set; } } // An enum to describe the custom attribute options public enum DateFormat { Default, ToLongDateString, ToLongTimeString, ToShortDateString, ToShortTimeString } // Class that has a property that uses the custom attribute public class User { [DateFormatAttribute(Format = DateFormat.ToShortDateString)] public DateTime registered { get; set; } public string name { get; set; } } // Declare user var user = new User() { name = "Kenneth", registered = DateTime.Now }; // Get the properties with the processed attribute var result = user.GetPropertyData(); // Display Info foreach (var prop in result) { Console.WriteLine($"Property: {prop.Key}, Value: {prop.Value}"); } // expected output: /* Property: registered, Value: 5/12/2021 Property: name, Value: Kenneth */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; using System.Collections.Generic; namespace Utils { public class DateFormatAttribute : System.Attribute { public DateFormat Format { get; set; } } public enum DateFormat { Default, ToLongDateString, ToLongTimeString, ToShortDateString, ToShortTimeString } public static class Extensions { /// <summary> /// Returns an objects property data with the data transformed /// according to its attributes /// </summary> /// <returns>The objects property data</returns> public static Dictionary<string, object> GetPropertyData<T>(this T source) where T : class { var result = new Dictionary<string, object>(); var flags = System.Reflection.BindingFlags.Instance | System.Reflection.BindingFlags.Public; var properties = source.GetType().GetProperties(flags); foreach (var prop in properties) { var key = prop.Name; var value = prop.CanRead ? prop.GetValue(source , prop.GetIndexParameters().Count() == 1 ? new object[] { null } : null) : null; // Get custom attributes for the property var customAttributes = Attribute.GetCustomAttributes(prop); foreach (var attribute in customAttributes) { if (attribute.GetType().Equals(typeof(DateFormatAttribute))) { var dateValue = System.Convert.ToDateTime(value); switch (((DateFormatAttribute)attribute).Format) { case DateFormat.ToLongDateString: value = dateValue.ToLongDateString(); break; case DateFormat.ToLongTimeString: value = dateValue.ToLongTimeString(); break; case DateFormat.ToShortDateString: value = dateValue.ToShortDateString(); break; case DateFormat.ToShortTimeString: value = dateValue.ToShortTimeString(); break; default: value = dateValue.ToString(); break; } } } result.Add(key, value); } return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { public class User { [DateFormatAttribute(Format = DateFormat.ToShortDateString)] public DateTime registered { get; set; } public string name { get; set; } } static void Main(string[] args) { try { var user = new User() { name = "Kenneth", registered = DateTime.Now }; // Get the properties with the processed attribute var result = user.GetPropertyData(); foreach (var prop in result) { Display($"Property: {prop.Key}, Value: {prop.Value}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply