C++ || How To Replace A Letter With Its Alphabet Position Using C++
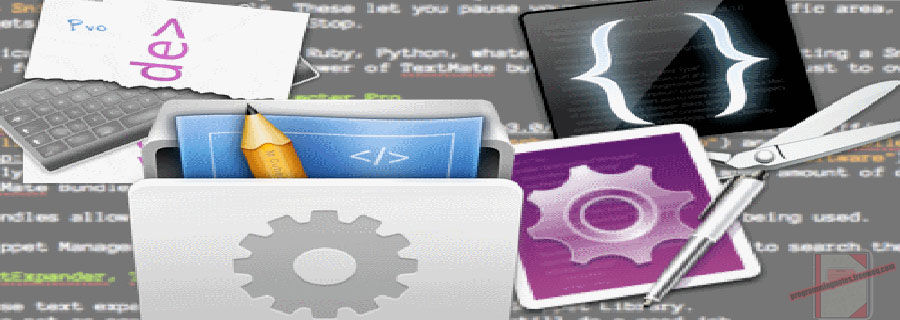
The following is a module with functions which demonstrates how to replace a letter with its alphabet position using C++.
1. Replace With Alphabet Position
The example below demonstrates the use of ‘Utils::getAlphabetPosition‘ to replace a letter with its alphabet position.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
// Replace With Alphabet Position // Get alphabet position auto result = Utils::getAlphabetPosition("The sunset sets at twelve o' clock."); // Display the results for (const auto& position : result) { std::cout << position << " "; } // expected output: /* 20 8 5 19 21 14 19 5 20 19 5 20 19 1 20 20 23 5 12 22 5 15 3 12 15 3 11 */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jun 3, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <cctype> #include <vector> #include <string> namespace Utils { /** * FUNCTION: getAlphabetPosition * USE: Gets the alphabet position of each character in a string * @param text: The text to get the position * @return: The alphabet position of each character */ std::vector<std::size_t> getAlphabetPosition(std::string text) { std::string alphabet = "abcdefghijklmnopqrstuvwxyz"; std::vector<std::size_t> result; for (const auto& letter : text) { auto index = alphabet.find(static_cast<unsigned char>(std::tolower(letter))); if (index != std::string::npos) { result.push_back(index + 1); } } return result; } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jun 3, 2021 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Get alphabet position auto result = Utils::getAlphabetPosition("The sunset sets at twelve o' clock."); // Display the results for (const auto& position : result) { std::cout << position << " "; } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply