C# || How To Determine When A Fresh Orange Becomes Rotten Using C#
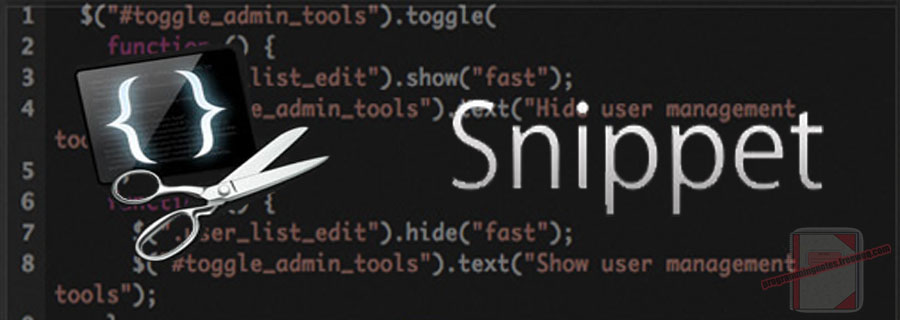
The following is a module with functions which demonstrates how to determine when a fresh orange becomes rotten using C#.
1. Oranges Rotting – Problem Statement
You are given an m x n grid where each cell can have one of three values:
- 0 representing an empty cell,
- 1 representing a fresh orange, or
- 2 representing a rotten orange.
Every minute, any fresh orange that is 4-directionally adjacent to a rotten orange becomes rotten.
Return the minimum number of minutes that must elapse until no cell has a fresh orange. If this is impossible, return -1.
Example 1:
Input: grid = [[2,1,1],[1,1,0],[0,1,1]]
Output: 4
Example 2:
Input: grid = [[2,1,1],[0,1,1],[1,0,1]]
Output: -1
Explanation: The orange in the bottom left corner (row 2, column 0) is never rotten, because rotting only happens 4-directionally.
Example 3:
Input: grid = [[0,2]]
Output: 0
Explanation: Since there are already no fresh oranges at minute 0, the answer is just 0.
2. Oranges Rotting – Solution
The following is a solution which demonstrates how to determine when a fresh orange becomes rotten.
This solution uses Breadth First Search when looking for fresh cells to turn rotten.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
// ============================================================================ // Author: Kenneth Perkins // Date: Oct 29, 2021 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to determine rotten oranges // ============================================================================ public class Solution { public int OrangesRotting(int[][] grid) { var EMPTY = 0; var FRESH = 1; var ROTTEN = 2; var elapsed = 0; var freshCount = 0; var queue = new Queue<KeyValuePair<int, int>>(); // Get fresh cell count and rotten cell coordinates for (int row = 0; row < grid.Length; ++row) { for (int col = 0; col < grid[row].Length; ++col) { if (grid[row][col] == FRESH) { ++freshCount; } else if (grid[row][col] == ROTTEN) { queue.Enqueue(new KeyValuePair<int, int>(row, col)); } } } // Search directions var directions = new List<KeyValuePair<int, int>>() { // Right new KeyValuePair<int, int>(0, 1), // Left new KeyValuePair<int, int>(0, -1), // Top new KeyValuePair<int, int>(-1, 0), // Bottom new KeyValuePair<int, int>(1, 0), }; // Look for fresh cells to turn rotten while (queue.Count > 0) { for (var itemCount = queue.Count; itemCount > 0; --itemCount) { var current = queue.Peek(); queue.Dequeue(); // Get the coordinates of the rotten cell var row = current.Key; var col = current.Value; // Search right, left, top, bottom to see if a fresh cell can become rotten foreach (var direction in directions) { var newRow = row + direction.Key; var newCol = col + direction.Value; // If the cell is fresh in this direction, make it rotten if (IsValidCell(grid, newRow, newCol) && grid[newRow][newCol] == FRESH) { grid[newRow][newCol] = ROTTEN; queue.Enqueue(new KeyValuePair<int, int>(newRow, newCol)); --freshCount; } } } // Increment elapsed time if (queue.Count > 0) { ++elapsed; } } return freshCount > 0 ? -1 : elapsed; } private bool IsValidCell(int[][] grid, int row, int col) { return row >= 0 && row < grid.Length && col >= 0 && col < grid[row].Length; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
4
-1
0
Leave a Reply