C++ || Snippet – How To Read & Write Data From A File
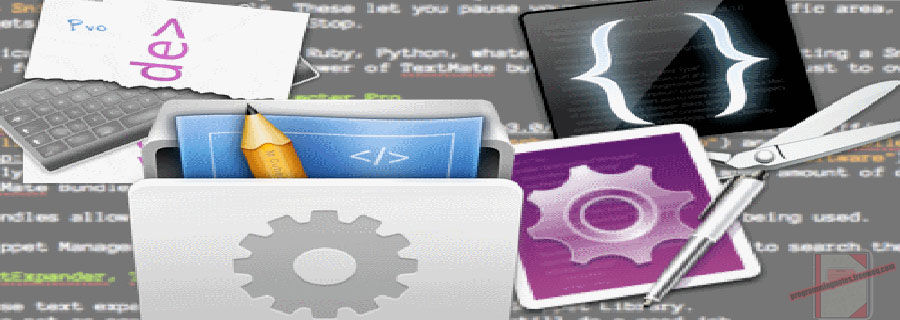
This page will consist of a demonstration of a simple quadratic formula program, which highlights the use of the input/output mechanisms of manipulating a text file. This program will read in data from a file (numbers), manipulate that data, and output new data into a different text file.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
NOTE: The data file that is used in this example can be downloaded here.
Also, in order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio > Projects > [Your project name] > [Your project name]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 12, 2012 // Taken From: http://programmingnotes.org/ // File: FileInput.cpp // Description: Demonstrates how to read data from a file // ============================================================================ #include <iostream> #include <fstream> #include <cstdlib> // used for the 'exit' command #include <cmath> using namespace std; int main () { // declare variables ifstream infile; ofstream outfile; double a=0,b=0,c=0; double root1=0, root2=0; // this opens the input file // YOUR INPUT FILE NAME GOES BELOW infile.open("INPUT_Quadratic_programmingnotes_freeweq_com.txt"); // check to see if the file even exists, & if not then EXIT if(infile.fail()) { cout<<"\nError, the input file could not be found!\n"; exit(1); // exits the program } // this opens the output file // if the file doesnt already exist, it will be created outfile.open("OUTPUT_Quadratic_programmingnotes_freeweq_com.txt"); // this loop reads in data until there is no more // data contained in the file while(infile.good()) { // this assigns the incoming data to the // variables 'a', 'b' and 'c' // NOTE: it is just like a cin >> statement infile >> a >> b >> c; // NOTE: if you want to read in data into an array // your declaration would be like this // ------------------------------------ // infile >> a[counter] >> b[counter] >> c[counter]; // ++counter; } // this does the quadratic formula calculations root1 = ((-b) + sqrt(pow(b,2) - (4*a*c)))/(2*a); root2 = ((-b) - sqrt(pow(b,2) - (4*a*c)))/(2*a); // this displays the numbers to screen via cout cout <<"For the numbers\na = "<<a<<"\nb = "<<b<<"\nc = "<<c<<endl; cout <<"\nroot 1 = "<<root1<<"\nroot 2 = "<<root2<<endl; // this saves the data to the output file // NOTE: its almost exactly the same as the cout statement outfile <<"For the numbers\na = "<<a<<"\nb = "<<b<<"\nc = "<<c<<endl; outfile <<"\nroot 1 = "<<root1<<"\nroot 2 = "<<root2<<endl; // close the input/output files once you are done using them infile.close(); outfile.close(); // stops the program from automatically closing cin.get(); return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
For the numbers
a = 2
b = 4
c = -16root 1 = 2
root 2 = -4
Leave a Reply