Java || Snippet – Custom Setw & Setfill Sample Code For Java
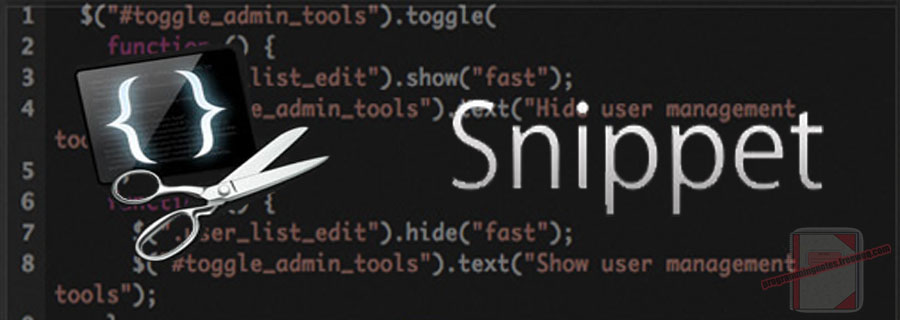
This page will consist of a brief implementation of setw/ios::width and setfill/ios::fill in Java.
If you are a C++ programmer, no doubt you have used the setw and setfill commands many times. It makes formatting output like this very clean and simple (see below).
Ending balance:................. $ 6433.47
Amount of deposits:............. $ 1750.00
Amount of withdrawals:.......... $ 420.00
Amount of interest earned:...... $ 103.47
But to my amazement, I could not find a very suitable replacement for the setw/setfill functions in Java. Using simple for loops, the methods provided on this page has the ability to mimic both functions which are available in C++.
Included in the sample code are the following:
== SETW ==
(1) right - Justifies string data of size "width," filling the width to the start of the string with whitespace
(2) left - Justifies string data of size "width," filling the width to the end of the string with whitespace
== SETFILL ==
(3) right - Justifies string data of size "width," filling the width to the start of the string with a filler character
(4) left - Justifies string data of size "width," filling the width to the end of the string with a filler character
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jun 6, 2012 // Taken From: http://programmingnotes.org/ // File: Setw.java // Description: Demonstrate the use of custom setw/ios::width and // setfill/ios::fill functions for text formatting in Java. // ============================================================================ public class Setw { // ==== right ====================================================================== // // - Set field width right align - // // Justifies string data of size "width," filling the width to the start of // the string with whitespace (' '). // // - USAGE - // right(<String> the text you wish to format, <int> size of width to be formated); // // ================================================================================= public static void right(String str, int width) { right(str, width, ' '); }// end of right // ==== left ====================================================================== // // - Set field width left align - // // Justifies string data of size "width," filling the width to the end of // the string with whitespace (' '). // // - USAGE - // left(<String> the text you wish to format, <int> size of width to be formated); // // ================================================================================= public static void left(String str, int width) { left(str, width, ' '); }// end of left // ==== right ====================================================================== // // - Set field width right align fill - // // Justifies string data of size "width," filling the width to the start of // the string with a filler character. // // Use this method (instead of using 'left/right') when you want so specify the // type of filler you want to use // // - USAGE - // right(<String> the text you wish to format, // <int> size of width to be formated, <char> the type of filler to be displayed); // // ================================================================================= public static void right(String str, int width, char fill) { for (int x = str.length(); x < width; ++x) { System.out.print(fill); } System.out.print(str); }// end of right // ==== left ====================================================================== // // - Set field width left align fill - // // Justifies string data of size "width," filling the width to the end of // the string with a filler character. // // Use this method (instead of using 'left/right') when you want so specify the // type of filler you want to use // // - USAGE - // left(<String> the text you wish to format, // <int> size of width to be formated, <char> the type of filler to be displayed); // // ================================================================================= public static void left(String str, int width, char fill) { System.out.print(str); for (int x = str.length(); x < width; ++x) { System.out.print(fill); } }// end of left }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Also, you must understand minimal object oriented programming to use this code!
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
===== DEMONSTRATION HOW TO USE =====
Use of the above snippet is similar to its C++ counterpart. Here is a sample program demonstrating its use.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jun 6, 2012 // Taken From: http://programmingnotes.org/ // File: Main.java // Description: Demonstrate the use of the Setw class // ============================================================================ public class Main { public static void main(String[] args) { // this formats text, printng whitespace to the right of the string System.out.print("1)"); Setw.right("This is text right aligned", 45); System.out.println(""); // this formats text, printng whitespace to the left of the string System.out.print("2)"); Setw.left("This is text left aligned", 45); System.out.print("!"); System.out.println(""); // this formats text, but this time, instead of printng whitespace // to the left of the string, it prints a "filler," which can be anything // you want. Currently, the filler is a exclaimation ('!') System.out.print("3)"); Setw.right("This is text right aligned with filler", 45, '!'); System.out.println(""); // this formats text, but this time, instead of printng whitespace // to the right of the string, it prints a "filler," which can be anything // you want. Currently, the filler is a dollar sign ('$') System.out.print("4)"); Setw.left("This is text left aligned with filler", 45, '$'); System.out.println(""); // you can also send numbers to any of the functions, // provided you first convert them to strings System.out.print("5)"); Setw.left("This is a string with a number " + Integer.toString(1987), 45, '.'); System.out.println("\n"); // you can also use this method to print the famous "triangle" shapes System.out.println("6) This is a triangle printed using the right method"); for(int width = 0; width <= 10; ++width) { Setw.right("", width, '*'); System.out.println(""); } System.out.println(""); // this prints an upside down triangle System.out.println("7) This is an upside down triangle printed " + "using the left method\n"); for(int width = 10; width > 0; --width) { Setw.left("", width, '*'); System.out.println(""); } System.out.println(""); // this is the example receipt System.out.println("8) This is the example receipt\n"); Setw.left("Ending balance:", 32, '.'); System.out.println(" $ " + Double.toString(6433.47)); Setw.left("Amount of deposits:", 32, '.'); System.out.println(" $ " + Double.toString(1750.00)); Setw.left("Amount of withdrawals:", 32, '.'); System.out.println(" $ " + Double.toString(420.00)); Setw.left("Amount of interest earned:", 32, '.'); System.out.println(" $ " + Double.toString(103.47)); }// end of main }// http://programmingnotes.org/ |
Once compiled, you should get this as your output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
1) This is text right aligned 2)This is text left aligned ! 3)!!!!!!!This is text right aligned with filler 4)This is text left aligned with filler$$$$$$$$ 5)This is a string with a number 1987.......... 6) This is a triangle printed using the right method * ** *** **** ***** ****** ******* ******** ********* ********** 7) This is an upside down triangle printed using the left method ********** ********* ******** ******* ****** ***** **** *** ** * 8) This is the example receipt Ending balance:................. $ 6433.47 Amount of deposits:............. $ 1750.0 Amount of withdrawals:.......... $ 420.0 Amount of interest earned:...... $ 103.47 |
Leave a Reply