JavaScript/CSS/HTML || Modal.js – Simple Modal Dialog Prompt Using Vanilla JavaScript
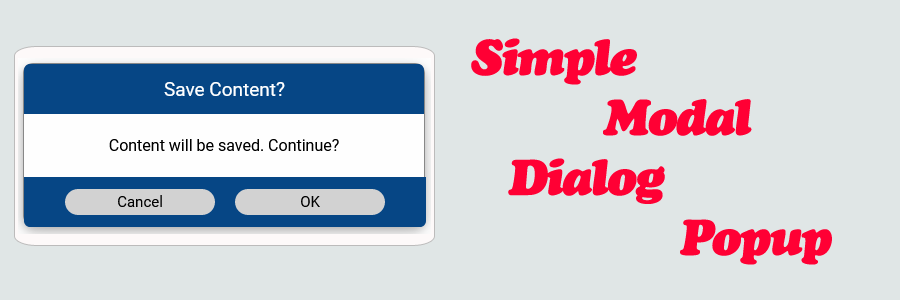
The following is a module which allows for a simple modal popup dialog panel in vanilla JavaScript. This module allows for custom Yes/No prompts.
Options include allowing the modal to be dragged, resized, and closed on outer area click. Modal drag and resize supports touch (mobile) input.
Contents
1. Basic Usage
2. Available Options
3. Alert Dialog
4. Yes/No Dialog
5. Custom Buttons
6. Dialog Instance
7. Modal.js & CSS Source
8. More Examples
1. Basic Usage
Syntax is very straightforward. The following demonstrates adding a simple dialog to the page.
1 2 3 4 5 6 7 8 9 10 11 |
// Add simple dialog. <script> (() => { // Adds a simple dialog to the page Modal.showDialog({ title: 'Save Content?', body: 'Content will be saved. Continue?', }); })(); </script> |
The following example demonstrates how to add click events to the default dialog buttons. The ordering of the buttons on initialization defines its placement on the dialog.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Add click events. <script> (() => { // Add click events to the buttons Modal.showDialog({ title: 'Save Content?', body: 'Content will be saved. Continue?', buttons: { cancel: { onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } }, ok: { onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } } } }); })(); </script> |
2. Available Options
The options supplied to the ‘Modal.showDialog‘ function is an object that is made up of the following properties.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// Available options Modal.showDialog({ title: 'Save Content?', // The title of the dialog body: 'Content will be saved. Continue?', // The content body of the dialog onShow: () => { // Optional. Function that allows to do something before show. Return false to stop showing process console.log(`Dialog is showing`); }, onHide: () => { // Optional. Function that allows to do something before hide. Return false to stop hidding process console.log(`Dialog is hiding`); }, onDestroy: () => { // Optional. Function that allows to do something before destroy. Return false to stop destroying process console.log(`Dialog is destroying`); }, closeOnOuterClick: false, // Optional. Determines if the dialog should close on outer dialog click. Default is False draggable: false, // Optional. Determines if the dialog is draggable. Default is False resizable: false, // Optional. Determines if the dialog is resizable. Default is False autoOpen: true, // Optional. Determines if the dialog should auto open. Default is True destroyOnHide: true, // Optional. Determines if the dialog should be removed from DOM after hiding. Default is True buttons: { // Optional. Allows to add custom buttons to the dialog custom1: { text: 'Custom Button', cssClass: '', visible: true, onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } }, // ..... Additional buttons }, }); |
Supplying different options to ‘Modal.showDialog‘ can change its appearance. The following examples below demonstrate this.
3. Alert Dialog
The following example demonstrates an alert dialog.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
// Alert dialog. <script> (() => { // Alert Modal.showDialog({ title: 'Content Saved!', body: 'Content has been saved to your account', buttons: { ok: {} } }); })(); </script> |
4. Yes/No Dialog
The following example demonstrates a yes/no dialog.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Yes/No dialog. <script> (() => { // Yes/No Modal.showDialog({ title: 'Continue?', body: 'Do you want to continue?', buttons: { no: {text: '😢 No'}, yes: {text: '😊 Yes'} } }); })(); </script> |
5. Custom Buttons
The following example demonstrates multiple custom buttons.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// Custom buttons. <script> (() => { // Custom buttons Modal.showDialog({ title: 'Save Content?', body: 'Content will be saved. Continue?', draggable: true, buttons: { custom1: { text: 'No Way!', cssClass: '', visible: true, onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } }, custom2: { text: 'Maybe?', cssClass: '', visible: true, onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } }, custom3: { text: 'Sure', cssClass: '', visible: true, onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } }, }, }); })(); </script> |
6. Dialog Instance
The following example demonstrates how to use the dialog instance to show and hide when needed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Dialog Instance. <script> (() => { // Create dialog instance and show/hide when needed let dialog = Modal.showDialog({ title: 'Save Content?', body: 'Content will be saved. Continue?', autoOpen: false, }); //dialog.modal // The Javascript modal element dialog.show(); // Shows the modal popup //dialog.hide(); // Hides the modal popup //dialog.destroy(); // Hides the modal popup & removes it from the DOM })(); </script> |
7. Modal.js & CSS Source
8. More Examples
Below are more examples demonstrating the use of ‘Modal.js‘. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 |
<!-- // ============================================================================ // Author: Kenneth Perkins // Date: Sept 23, 2020 // Taken From: http://programmingnotes.org/ // File: modalDialog.html // Description: Demonstrates the use of Modal.js // ============================================================================ --> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>My Programming Notes Modal.js Demo</title> <style> .main { text-align:center; margin-left:auto; margin-right:auto; } .button { padding: 5px; background-color: #d2d2d2; height:100%; text-align:center; text-decoration:none; color:black; display: flex; justify-content: center; align-items: center; flex-direction: column; border-radius: 15px; cursor: pointer; width: 120px; border: none; font-size: 15px; font-family: "Roboto",sans-serif, Marmelad,"Lucida Grande",Arial,"Hiragino Sans GB",Georgia,"Helvetica Neue",Helvetica; } .button:hover { background-color:#bdbdbd; } .inline { display:inline-block; } </style> <!-- // Include module --> <link type="text/css" rel="stylesheet" href="./Modal.css"> <script type="text/javascript" src="./Modal.js"></script> </head> <body> <div class="main"> My Programming Notes Modal.js Demo <div style="margin-top: 20px;"> <div id="btnShow" class="inline button"> Show </div> </div> </div> <script> document.addEventListener("DOMContentLoaded", function(eventLoaded) { // Custom buttons let dialog = Modal.showDialog({ title: 'Save Content?', // The title of the dialog body: 'Content will be saved. Continue?', // The content body of the dialog onShow: () => { // Optional. Function that allows to do something before show. Return false to stop showing process console.log(`Dialog ${dialog.modal.id} is showing`); }, onHide: () => { // Optional. Function that allows to do something before hide. Return false to stop hidding process console.log(`Dialog ${dialog.modal.id} is hiding`); }, onDestroy: () => { // Optional. Function that allows to do something before destroy. Return false to stop destroying process console.log(`Dialog ${dialog.modal.id} is destroying`); }, closeOnOuterClick: false, // Optional. Determines if the dialog should close on outer dialog click. Default is False draggable: true, // Optional. Determines if the dialog is draggable. Default is False resizable: false, // Optional. Determines if the dialog is resizable. Default is False autoOpen: false, // Optional. Determines if the dialog should auto open. Default is True destroyOnHide: false, // Optional. Determines if the dialog should be removed from DOM after hiding. Default is True buttons: { // Optional. Allows to add custom buttons to the dialog custom1: { text: 'No Way!', cssClass: '', visible: true, onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } }, custom2: { text: 'Maybe?', cssClass: '', visible: true, onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } }, custom3: { text: 'Sure', cssClass: '', visible: true, onClick: (event) => { console.log(`${event.target.innerText} Button Clicked`); } }, }, }); setTimeout(() => { //dialog.modal // The Javascript modal element dialog.show(); // Shows the modal popup setTimeout(() => { dialog.hide(); // Hides the modal popup //dialog.destroy(); // Hides the modal popup & removes it from the DOM }, 2000); }, 200); // Show the modal document.querySelector('#btnShow').addEventListener('click', (event) => { dialog.show() }); }); </script> </body> </html><!-- // http://programmingnotes.org/ --> |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply