Monthly Archives: May 2021
C# || How To Get The Date Value & Time Value From A Date Using C#
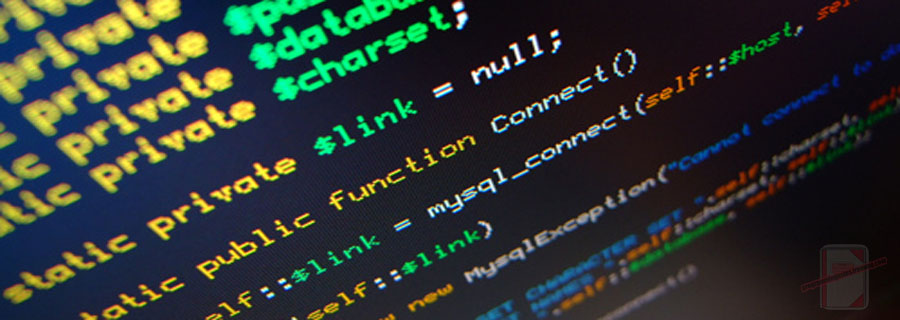
The following is a module with functions which demonstrates how to get the date value and time value from a DateTime using C#.
The functions listed on this page demonstrates how to isolate the date portion of a date, as well as the time portion of a date. For example, given the date “5/23/2019 7:28:31 PM”, the date value would be 5/23/2019, and time value 7:28:31 PM.
1. Date Value
The example below demonstrates the use of ‘Utils.Methods.DateValue‘ to get the date value of a date.
The DateTime object value contains the date information, with the time portion set to midnight (00:00:00)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Date Value // Declare date value var original = "5/23/2019 7:28:31 PM"; // Get the date portion var dateOnly = Utils.Methods.DateValue(original); // Display results Console.WriteLine($@" Original: {original} Date Value: {dateOnly} "); // example output: /* Original: 5/23/2019 7:28:31 PM Date Value: 5/23/2019 12:00:00 AM */ |
2. Time Value
The example below demonstrates the use of ‘Utils.Methods.TimeValue‘ to get the date value of a date.
The DateTime object contains the time information, with the date portion set to January 1 of the year 1
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Time Value // Declare date value var original = "5/23/2019 7:28:31 PM"; // Get the time portion var timeOnly = Utils.Methods.TimeValue(original); // Display results Console.WriteLine($@" Original: {original} Time Value: {timeOnly} "); // example output: /* Original: 5/23/2019 7:28:31 PM Time Value: 1/1/0001 7:28:31 PM */ |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 23, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Methods { /// <summary> /// Returns a DateTime value containing the date information, with /// the time information set to midnight (00:00:00) /// </summary> /// <param name="value">A string expression representing a date/time /// value from 00:00:00 on January 1 of the year 1 through 23:59:59 /// on December 31, 9999</param> /// <returns>A DateTime value containing the date information, with /// the time information set to midnight (00:00:00)</returns> public static DateTime DateValue(string value) { return DateValue(System.Convert.ToDateTime(value)); } /// <summary> /// Returns a DateTime value containing the date information, with the /// time information set to midnight (00:00:00) /// </summary> /// <param name="value">A DateTime value</param> /// <returns>A DateTime value containing the date information, with the /// time information set to midnight (00:00:00)</returns> public static DateTime DateValue(DateTime value) { return value.Date; } /// <summary> /// Returns a DateTime value containing the time information, with the /// date information set to January 1 of the year 1 /// </summary> /// <param name="value">A string expression representing a date/time /// value from 00:00:00 on January 1 of the year 1 through 23:59:59 /// on December 31, 9999</param> /// <returns>A DateTime value containing the time information, with the /// date information set to January 1 of the year 1</returns> public static DateTime TimeValue(string value) { return TimeValue(System.Convert.ToDateTime(value)); } /// <summary> /// Returns a DateTime value containing the time information, with /// the date information set to January 1 of the year 1 /// </summary> /// <param name="value">A DateTime value</param> /// <returns>A DateTime value containing the time information, with /// the date information set to January 1 of the year 1</returns> public static DateTime TimeValue(DateTime value) { return (new DateTime()).Add(value.TimeOfDay); } } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 23, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; public class Program { static void Main(string[] args) { try { // Declare date value var original = "5/23/2019 7:28:31 PM"; // Get the date portion var dateOnly = Utils.Methods.DateValue(original); // Get the time portion var timeOnly = Utils.Methods.TimeValue(original); // Display results Display($@" Original: {original} Date Value: {dateOnly} Time Value: {timeOnly} "); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Create Multiple Tasks With Maximum Concurrency Using C#
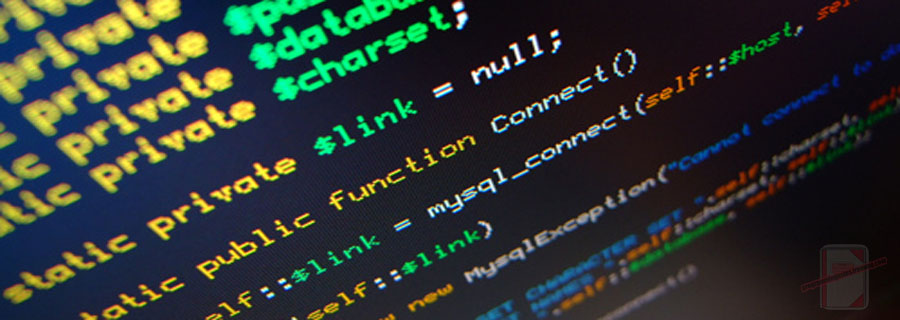
The following is a module with functions which demonstrates how to create multiple tasks with maximum concurrency using C#.
The examples demonstrated on this page uses System.Threading.Tasks.Task to start and run tasks. They also use System.Threading.SemaphoreSlim to limit the number of tasks that can run concurrently.
The examples on this page demonstrates how to start and run multiple tasks with a maximum concurrency. It also demonstrates how to start and run multiple tasks with a return value.
1. Task – Maximum Concurrency
The example below demonstrates how to start and run multiple tasks with a maximum concurrency. For example purposes, the tasks do not return a value.
The functions shown in the example below are called asynchronously, but they can also be called synchronously.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
// Task - Maximum Concurrency var concurrentTasks = 5; var semaphore = new System.Threading.SemaphoreSlim(concurrentTasks, concurrentTasks); var tasks = new List<System.Threading.Tasks.Task>(); for (var count = 1; count <= 20; ++count) { var taskNumber = count; // Blocks execution until another task can be started. // This function also accepts a timeout in milliseconds await semaphore.WaitAsync(); // Start a task var task = System.Threading.Tasks.Task.Run(async () => { // Execute long running code try { Console.WriteLine($"Task #{taskNumber} starting..."); await System.Threading.Tasks.Task.Delay(5000); Console.WriteLine($" - Task #{taskNumber} completed!"); } catch { throw; } finally { // Signal that the task is completed // so another task can start semaphore.Release(); } }); tasks.Add(task); } Console.WriteLine($"Waiting for tasks to complete"); // Wait for all tasks to complete await System.Threading.Tasks.Task.WhenAll(tasks.ToArray()); Console.WriteLine($"All tasks completed!"); // example output: /* Task #2 starting... Task #1 starting... Task #3 starting... Task #5 starting... Task #4 starting... - Task #3 completed! - Task #1 completed! Task #6 starting... - Task #4 completed! - Task #2 completed! Task #8 starting... Task #9 starting... - Task #5 completed! Task #7 starting... Task #10 starting... - Task #6 completed! Task #11 starting... - Task #8 completed! Task #12 starting... - Task #9 completed! Task #13 starting... - Task #7 completed! - Task #10 completed! Task #14 starting... Task #15 starting... - Task #11 completed! Task #16 starting... - Task #12 completed! Task #17 starting... - Task #13 completed! Task #18 starting... - Task #14 completed! - Task #15 completed! Task #19 starting... Waiting for tasks to complete Task #20 starting... - Task #16 completed! - Task #17 completed! - Task #18 completed! - Task #20 completed! - Task #19 completed! All tasks completed! */ |
2. Task – Maximum Concurrency – Return Value
The example below demonstrates how to start and run multiple tasks with a maximum concurrency. In this example, a value is returned and retrieved from the tasks
The functions shown in the example below are called asynchronously, but they can also be called synchronously.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 |
// Task - Maximum Concurrency - Return Value public class Part { public string PartName { get; set; } public int PartId { get; set; } } var concurrentTasks = 5; var semaphore = new System.Threading.SemaphoreSlim(concurrentTasks, concurrentTasks); var tasks = new List<System.Threading.Tasks.Task<Part>>(); for (var count = 1; count <= 20; ++count) { var taskNumber = count; // Blocks execution until another task can be started. // This function also accepts a timeout in milliseconds await semaphore.WaitAsync(); // Start a task var task = System.Threading.Tasks.Task.Run(async () => { // Execute long running code try { Console.WriteLine($"Task #{taskNumber} starting..."); await System.Threading.Tasks.Task.Delay(5000); Console.WriteLine($" - Task #{taskNumber} completed!"); // Return result return new Part() { PartId = taskNumber, PartName = $"Part #{taskNumber}" }; } catch { throw; } finally { // Signal that the task is completed // so another task can start semaphore.Release(); } }); tasks.Add(task); } Console.WriteLine($"Waiting for tasks to complete"); // Wait for all tasks to complete await System.Threading.Tasks.Task.WhenAll(tasks.ToArray()); Console.WriteLine($"All tasks completed!"); Console.WriteLine(""); // Get the results foreach (var task in tasks) { var part = task.Result; Console.WriteLine($"Id: {part.PartId}, Name: {part.PartName}"); } // example output: /* Task #1 starting... Task #2 starting... Task #3 starting... Task #4 starting... Task #5 starting... - Task #3 completed! - Task #1 completed! Task #6 starting... - Task #5 completed! - Task #2 completed! Task #8 starting... Task #7 starting... Task #9 starting... - Task #4 completed! Task #10 starting... - Task #6 completed! Task #11 starting... - Task #8 completed! Task #12 starting... - Task #7 completed! Task #13 starting... - Task #10 completed! - Task #9 completed! Task #14 starting... Task #15 starting... - Task #11 completed! Task #16 starting... - Task #12 completed! Task #17 starting... - Task #13 completed! Task #18 starting... - Task #15 completed! - Task #14 completed! Task #19 starting... Waiting for tasks to complete Task #20 starting... - Task #17 completed! - Task #16 completed! - Task #18 completed! - Task #19 completed! - Task #20 completed! All tasks completed! Id: 1, Name: Part #1 Id: 2, Name: Part #2 Id: 3, Name: Part #3 Id: 4, Name: Part #4 Id: 5, Name: Part #5 Id: 6, Name: Part #6 Id: 7, Name: Part #7 Id: 8, Name: Part #8 Id: 9, Name: Part #9 Id: 10, Name: Part #10 Id: 11, Name: Part #11 Id: 12, Name: Part #12 Id: 13, Name: Part #13 Id: 14, Name: Part #14 Id: 15, Name: Part #15 Id: 16, Name: Part #16 Id: 17, Name: Part #17 Id: 18, Name: Part #18 Id: 19, Name: Part #19 Id: 20, Name: Part #20 */ |
3. More Examples
Below is a full example of the process demonstrated on this page!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 15, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates creating a task pool // ============================================================================ using System; using System.Diagnostics; using System.Linq; using System.Collections.Generic; using System.Threading.Tasks; public class Program { public class Part { public string PartName { get; set; } public int? PartId { get; set; } } static void Main(string[] args) { try { //TaskVoid().Wait(); TaskFunction().Wait(); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } public static async Task<List<Part>> TaskFunction() { var concurrentTasks = 5; var semaphore = new System.Threading.SemaphoreSlim(concurrentTasks, concurrentTasks); var tasks = new List<System.Threading.Tasks.Task<Part>>(); for (var count = 1; count <= 20; ++count) { var taskNumber = count; // Blocks execution until another task can be started. // This function also accepts a timeout in milliseconds await semaphore.WaitAsync(); // Start a task var task = System.Threading.Tasks.Task.Run(async () => { // Execute long running code try { Display($"Task #{taskNumber} starting..."); await System.Threading.Tasks.Task.Delay(5000); Display($" - Task #{taskNumber} completed!"); // Return result return new Part() { PartId = taskNumber, PartName = $"Part #{taskNumber}" }; } catch { throw; } finally { // Signal that the task is completed // so another task can start semaphore.Release(); } }); tasks.Add(task); } Display($"Waiting for tasks to complete"); // Wait for all tasks to complete await System.Threading.Tasks.Task.WhenAll(tasks.ToArray()); Display($"All tasks completed!"); Display(""); // Get the results foreach (var task in tasks) { var part = task.Result; Display($"Id: {part.PartId}, Name: {part.PartName}"); } return tasks.Select(task => task.Result).ToList(); } public static async Task TaskVoid() { var concurrentTasks = 5; var semaphore = new System.Threading.SemaphoreSlim(concurrentTasks, concurrentTasks); var tasks = new List<System.Threading.Tasks.Task>(); for (var count = 1; count <= 20; ++count) { var taskNumber = count; // Blocks execution until another task can be started. // This function also accepts a timeout in milliseconds await semaphore.WaitAsync(); // Start a task var task = System.Threading.Tasks.Task.Run(async () => { // Execute long running code try { Display($"Task #{taskNumber} starting..."); await System.Threading.Tasks.Task.Delay(5000); Display($" - Task #{taskNumber} completed!"); } catch { throw; } finally { // Signal that the task is completed // so another task can start semaphore.Release(); } }); tasks.Add(task); } Display($"Waiting for tasks to complete"); // Wait for all tasks to complete await System.Threading.Tasks.Task.WhenAll(tasks.ToArray()); Display($"All tasks completed!"); } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Convert Bytes To Kilobytes, Megabytes, Gigabytes, Terabytes Using C#
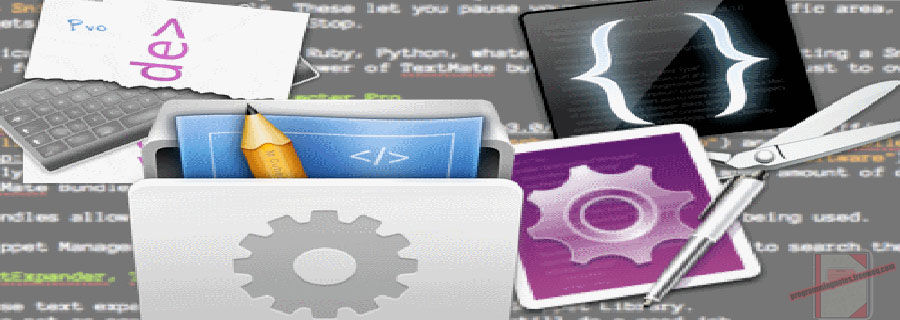
The following is a module with functions which demonstrates how to convert bytes to decimal formats like kilobytes, megabytes, gigabytes, terabytes, petabytes, exabytes, zettabytes, and yottabytes, as well as binary formats like kibibytes, mebibytes, gibibytes, tebibytes, pebibytes, exbibytes, zebibytes, and yobibytes using C#.
The function demonstrated on this page follows the IEC standard, which means:
• 1 kilobyte = 1000 bytes (Decimal)
• 1 kibibyte = 1024 bytes (Binary)
This function allows you to convert bytes to a measurement unit, a measurement unit to bytes, and allows to convert from one measurement unit to another measurement unit.
1. Convert Bytes To Measurement Unit
The example below demonstrates the use of ‘Utils.Bytes.FromTo‘ to convert bytes to a measurement unit.
The optional function parameter allows you to specify the decimal places.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Convert Bytes To Measurement Unit // Declare values to convert var values = new[] { 2287879, 536870912, 1073741824 }; // Convert values foreach (var value in values) { // Convert bytes to megabyte var mb = Utils.Bytes.FromTo(Utils.Bytes.Unit.Byte, value, Utils.Bytes.Unit.Megabyte); // Convert bytes to mebibyte var mib = Utils.Bytes.FromTo(Utils.Bytes.Unit.Byte, value, Utils.Bytes.Unit.Mebibyte); // Display the converted values Console.WriteLine($"Bytes: {value}, Megabyte: {mb}, Mebibyte: {mib}"); } // expected output: /* Bytes: 2287879, Megabyte: 2.287879, Mebibyte: 2.18189144134521484375 Bytes: 536870912, Megabyte: 536.870912, Mebibyte: 512 Bytes: 1073741824, Megabyte: 1073.741824, Mebibyte: 1024 */ |
2. Convert Measurement Unit To Bytes
The example below demonstrates the use of ‘Utils.Bytes.FromTo‘ to convert a measurement unit to bytes.
The optional function parameter allows you to specify the decimal places.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
// Convert Measurement Unit To Bytes // Declare values to convert var values = new[] { 1, 0.5M, 10.75M }; // Convert values foreach (var value in values) { // Convert gibibyte to byte var bytes = Utils.Bytes.FromTo(Utils.Bytes.Unit.Gibibyte, value, Utils.Bytes.Unit.Byte); // Display the converted values Console.WriteLine($"Gibibyte: {value}, Bytes: {bytes}"); } // expected output: /* Gibibyte: 1, Bytes: 1073741824 Gibibyte: 0.5, Bytes: 536870912.0 Gibibyte: 10.75, Bytes: 11542724608.00 */ |
3. Convert Measurement Unit To Measurement Unit
The example below demonstrates the use of ‘Utils.Bytes.FromTo‘ to convert a measurement unit to another measurement unit.
The optional function parameter allows you to specify the decimal places.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Convert Measurement Unit To Measurement Unit // Declare values to convert var values = new[] { 1991, 1987, 28.31M, 19.22M }; // Convert values foreach (var value in values) { // Convert value from one unit to another var size = Utils.Bytes.FromTo(Utils.Bytes.Unit.Gigabyte, value, Utils.Bytes.Unit.Terabyte); // Display the converted values Display($"Gigabyte: {value}, Terabyte: {size}"); } // expected output: /* Gigabyte: 1991, Terabyte: 1.991 Gigabyte: 1987, Terabyte: 1.987 Gigabyte: 28.31, Terabyte: 0.02831 Gigabyte: 19.22, Terabyte: 0.01922 */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Collections.Generic; namespace Utils { public static class Bytes { public enum Unit { Byte, // Decimal Kilobyte, Megabyte, Gigabyte, Terabyte, Petabyte, Exabyte, Zettabyte, Yottabyte, // Binary Kibibyte, Mebibyte, Gibibyte, Tebibyte, Pebibyte, Exbibyte, Zebibyte, Yobibyte } /// <summary> /// Converts a measurement 'Unit' to another measurement 'Unit' /// </summary> /// <param name="unitFrom">The measurement unit converting from</param> /// <param name="sizeFrom">The size of the 'from' measurement unit</param> /// <param name="unitTo">The measurement unit to convert to</param> /// <param name="decimalPlaces">The decimal places to round to</param> /// <returns>The value converted to the specified measurement unit</returns> public static decimal FromTo(Unit unitFrom, decimal sizeFrom, Unit unitTo , int? decimalPlaces = null) { var result = sizeFrom; if (unitFrom != unitTo) { if (unitFrom == Unit.Byte) { result = ConvertTo(unitTo, sizeFrom, decimalPlaces); } else if (unitTo == Unit.Byte) { result = ConvertFrom(unitFrom, sizeFrom, decimalPlaces); } else { result = ConvertTo(unitTo, ConvertFrom(unitFrom, sizeFrom), decimalPlaces); } } return result; } private enum Conversion { From, To } // Converts bytes to a measurement unit private static decimal ConvertTo(Unit unit, decimal bytes , int? decimalPlaces = null) { return Convert(Conversion.To, bytes, unit, decimalPlaces); } // Converts a measurement unit to bytes private static decimal ConvertFrom(Unit unit, decimal bytes , int? decimalPlaces = null) { return Convert(Conversion.From, bytes, unit, decimalPlaces); } private static decimal Convert(Conversion operation, decimal bytes, Unit unit , int? decimalPlaces) { // Get the unit type definition var definition = GetDefinition(unit); if (definition == null) { throw new ArgumentException($"Unknown unit type: {unit}", nameof(unit)); } // Get the unit value var value = definition.Value; // Calculate the result var result = operation == Conversion.To ? bytes / value : bytes * value; if (decimalPlaces.HasValue) { result = Math.Round(result, decimalPlaces.Value, MidpointRounding.AwayFromZero); } return result; } public enum Prefix { Decimal, Binary } public class Definition { public Prefix Prefix { get; set; } public int OrderOfMagnitude { get; set; } public decimal Multiple { get { return Prefix == Prefix.Decimal ? 1000 : 1024; } } public decimal Value { get { return System.Convert.ToDecimal(Math.Pow((double)Multiple, OrderOfMagnitude)); } } } public static Definition GetDefinition(Unit unit) { var definitions = GetDefinitions(); return definitions.ContainsKey(unit) ? definitions[unit] : null; } public static Dictionary<Unit, Definition> GetDefinitions() { if (definitions == null) { definitions = new Dictionary<Unit, Definition>(); // Place units in order of magnitude // Decimal units var decimals = new[] { Unit.Kilobyte, Unit.Megabyte, Unit.Gigabyte, Unit.Terabyte, Unit.Petabyte, Unit.Exabyte, Unit.Zettabyte, Unit.Yottabyte }; // Binary units var binary = new[] { Unit.Kibibyte, Unit.Mebibyte, Unit.Gibibyte, Unit.Tebibyte, Unit.Pebibyte, Unit.Exbibyte, Unit.Zebibyte, Unit.Yobibyte }; AddDefinitions(definitions, Prefix.Decimal, decimals); AddDefinitions(definitions, Prefix.Binary, binary); } return definitions; } private static Dictionary<Unit, Definition> definitions = null; private static void AddDefinitions(Dictionary<Unit, Definition> definitions , Prefix prefix, IEnumerable<Unit> units) { int index = 1; foreach (var unit in units) { if (!definitions.ContainsKey(unit)) { definitions.Add(unit, new Definition() { Prefix = prefix, OrderOfMagnitude = index }); } ++index; } } } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; public class Program { static void Main(string[] args) { try { // Declare values to convert var values = new[] { 2287879, 536870912, 1073741824 }; // Convert values foreach (var value in values) { // Convert bytes to megabyte var mb = Utils.Bytes.FromTo(Utils.Bytes.Unit.Byte, value, Utils.Bytes.Unit.Megabyte); // Convert bytes to mebibyte var mib = Utils.Bytes.FromTo(Utils.Bytes.Unit.Byte, value, Utils.Bytes.Unit.Mebibyte); // Display the converted values Display($"Bytes: {value}, Megabyte: {mb}, Mebibyte: {mib}"); } Display(""); // Declare values to convert var values2 = new[] { 1, 0.5M, 10.75M }; // Convert values foreach (var value in values2) { // Convert gibibyte to byte var bytes = Utils.Bytes.FromTo(Utils.Bytes.Unit.Gibibyte, value, Utils.Bytes.Unit.Byte); // Display the converted values Display($"Gibibyte: {value}, Bytes: {bytes}"); } Display(""); // Declare values to convert var values3 = new[] { 1991, 1987, 28.31M, 19.22M }; // Convert values foreach (var value in values3) { // Convert value from one unit to another var size = Utils.Bytes.FromTo(Utils.Bytes.Unit.Gigabyte, value, Utils.Bytes.Unit.Terabyte); // Display the converted values Display($"Gigabyte: {value}, Terabyte: {size}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Parse A Delimited CSV File Using C#
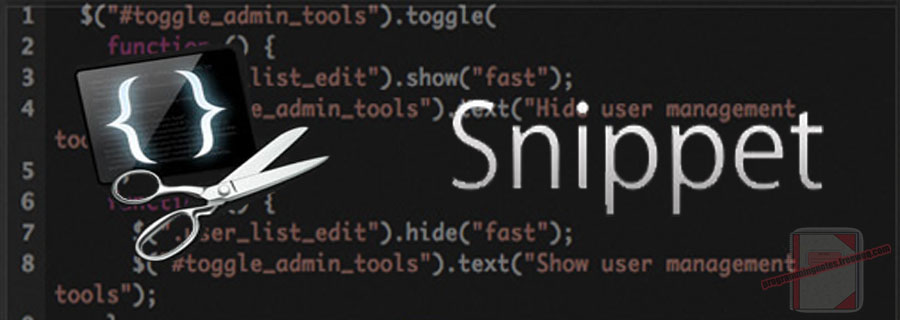
The following is a module with functions which demonstrates how to parse a delimited CSV file using VB.NET.
This function parses a CSV file and returns its results as a List. Each List index represents a line in the CSV file, with each item in the list representing a record contained on that line.
The function demonstrated on this page uses FileIO.TextFieldParser to parse values in a CSV file.
Note: To use the function in this module, make sure you have a reference to ‘Microsoft.VisualBasic‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add Reference‘, then type ‘Microsoft.VisualBasic‘ in the search box, and add the reference titled Microsoft.VisualBasic in the results Tab.
1. Parse CSV File
The example below demonstrates the use of ‘Utils.Methods.ParseCsv‘ to parse a CSV file and return its results as a List.
The optional function parameter allows you to specify the delimiters. Default delimiter is a comma (,).
Sample CSV used in this example is the following:
1 2 3 4 5 6 |
Kenneth,Perkins,120 jefferson st.,Riverside, NJ, 08075 Jack,McGinnis,220 hobo Av.,Phila, PA,09119 "Jennifer ""Da Man""",Repici,120 Jefferson St.,Riverside, NJ,08075 Stephen,Tyler,"7452 Terrace ""At the Plaza"" road",SomeTown,SD, 91234 ,Blankman,,SomeTown, SD, 00298 "Joan ""the bone"", Anne",Jet,"9th, at Terrace plc",Desert City,CO,00123 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
// Parse CSV File // Read file into byte array byte[] fileBytes; // Parse the contents of the file into a list var fileContents = Utils.Methods.ParseCsv(fileBytes); // Display the contents of the file for (var lineIndex = 0; lineIndex < fileContents.Count; ++lineIndex) { var line = fileContents[lineIndex]; Console.WriteLine($"Line #{lineIndex + 1}"); foreach (var item in line) { Console.WriteLine($" Item: {item}"); } } // expected output: /* Line #1 Item: Kenneth Item: Perkins Item: 120 jefferson st. Item: Riverside Item: NJ Item: 08075 Line #2 Item: Jack Item: McGinnis Item: 220 hobo Av. Item: Phila Item: PA Item: 09119 Line #3 Item: Jennifer "Da Man" Item: Repici Item: 120 Jefferson St. Item: Riverside Item: NJ Item: 08075 Line #4 Item: Stephen Item: Tyler Item: 7452 Terrace "At the Plaza" road Item: SomeTown Item: SD Item: 91234 Line #5 Item: Item: Blankman Item: Item: SomeTown Item: SD Item: 00298 Line #6 Item: Joan "the bone", Anne Item: Jet Item: 9th, at Terrace plc Item: Desert City Item: CO Item: 00123 */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; using System.Collections.Generic; namespace Utils { public static class Methods { /// <summary> /// Parses a Csv file and returns its results as a List. /// Each List index represents a line in the Csv file, with each /// item in the list representing a record contained on that line. /// </summary> /// <param name="fileBytes">The Csv file as a byte array</param> /// <param name="delimiters">The Csv data delimiter</param> /// <returns>The the file contents as a List</returns> public static List<List<string>> ParseCsv(byte[] fileBytes , string delimiters = ",") { var results = new List<List<string>>(); using (var stream = new System.IO.MemoryStream(fileBytes)) { using (var parser = new Microsoft.VisualBasic.FileIO.TextFieldParser(stream)) { parser.TextFieldType = Microsoft.VisualBasic.FileIO.FieldType.Delimited; parser.Delimiters = delimiters.Select(letter => letter.ToString()).ToArray(); parser.HasFieldsEnclosedInQuotes = true; // Parse each line in the file while (!parser.EndOfData) { var currentLine = parser.ReadFields(); results.Add(currentLine.ToList()); } } } return results; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { static void Main(string[] args) { try { // Read file into byte array byte[] fileBytes; // Parse the contents of the file into a list var fileContents = Utils.Methods.ParseCsv(fileBytes); // Display the contents of the file for (var lineIndex = 0; lineIndex < fileContents.Count; ++lineIndex) { var line = fileContents[lineIndex]; Display($"Line #{lineIndex + 1}"); foreach (var item in line) { Display($" Item: {item}"); } } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Round A Number To The Nearest X Using C#
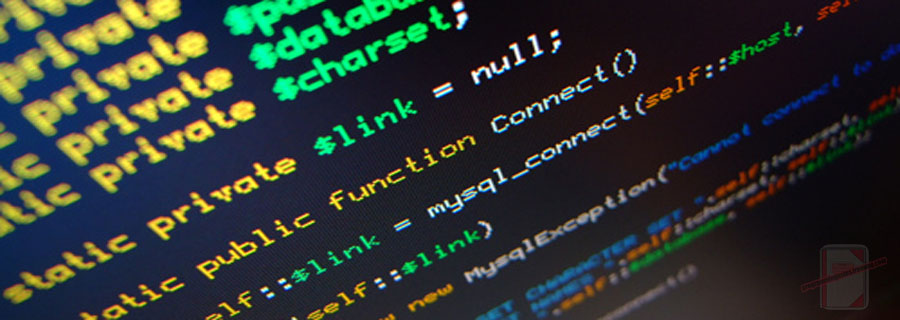
The following is a module with functions which demonstrates how to round a number to the nearest X using C#.
This function has the ability to either round a number to the nearest amount, always round up, or always round down. For example, when dealing with money, this is good for rounding a dollar amount to the nearest 5 cents.
1. Round – Nearest
The example below demonstrates the use of ‘Utils.Round.Amount‘ to round a number to the nearest 5 cents.
The optional function parameter determines they type of rounding to perform.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Round - Nearest // Declare values to round var values = new decimal[] { 19.28M, 31.22M, 19.91M, 19.87M, 0.05M }; // Declare step amount var stepAmount = 0.05M; // Round to nearest amount foreach (var value in values) { Console.WriteLine($"Value: {value}, Rounded: {Utils.Round.Amount(value, stepAmount)}"); } // expected output: /* Value: 19.28, Rounded: 19.3 Value: 31.22, Rounded: 31.2 Value: 19.91, Rounded: 19.9 Value: 19.87, Rounded: 19.85 Value: 0.05, Rounded: 0.05 */ |
2. Round – Up
The example below demonstrates the use of ‘Utils.Round.Amount‘ to always round a number up to the nearest 5 cents.
The optional function parameter determines they type of rounding to perform.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Round - Up // Declare values to round var values = new decimal[] { 19.28M, 31.22M, 19.91M, 19.87M, 0.05M }; // Declare step amount var stepAmount = 0.05M; // Round up to nearest amount foreach (var value in values) { Console.WriteLine($"Value: {value}, Rounded: {Utils.Round.Amount(value, stepAmount, Utils.Round.Type.Up)}"); } // expected output: /* Value: 19.28, Rounded: 19.3 Value: 31.22, Rounded: 31.25 Value: 19.91, Rounded: 19.95 Value: 19.87, Rounded: 19.9 Value: 0.05, Rounded: 0.05 */ |
3. Round – Down
The example below demonstrates the use of ‘Utils.Round.Amount‘ to always round a number down to the nearest 5 cents.
The optional function parameter determines they type of rounding to perform.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Round - Down // Declare values to round var values = new decimal[] { 19.28M, 31.22M, 19.91M, 19.87M, 0.05M }; // Declare step amount var stepAmount = 0.05M; // Round down to nearest amount foreach (var value in values) { Console.WriteLine($"Value: {value}, Rounded: {Utils.Round.Amount(value, stepAmount, Utils.Round.Type.Down)}"); } // expected output: /* Value: 19.28, Rounded: 19.25 Value: 31.22, Rounded: 31.2 Value: 19.91, Rounded: 19.9 Value: 19.87, Rounded: 19.85 Value: 0.05, Rounded: 0.05 */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Round { public enum Type { Nearest, Up, Down } /// <summary> /// Rounds a number to the nearest X /// </summary> /// <param name="value">The value to round</param> /// <param name="stepAmount">The amount to round the value by</param> /// <param name="type">The type of rounding to perform</param> /// <returns>The value rounded by the step amount and type</returns> public static decimal Amount(decimal value, decimal stepAmount , Round.Type type = Round.Type.Nearest) { var inverse = 1 / stepAmount; var dividend = value * inverse; switch (type) { case Round.Type.Nearest: dividend = Math.Round(dividend); break; case Round.Type.Up: dividend = Math.Ceiling(dividend); break; case Round.Type.Down: dividend = Math.Floor(dividend); break; default: throw new ArgumentException($"Unknown type: {type}", nameof(type)); } var result = dividend / inverse; return result; } } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; public class Program { static void Main(string[] args) { try { // Declare values to round var values = new decimal[] { 19.28M, 31.22M, 19.91M, 19.87M, 0.05M }; // Declare step amount var stepAmount = 0.05M; // Round to nearest amount foreach (var value in values) { Display($"Value: {value}, Rounded: {Utils.Round.Amount(value, stepAmount)}"); } Display(""); // Round up to nearest amount foreach (var value in values) { Display($"Value: {value}, Rounded: {Utils.Round.Amount(value, stepAmount, Utils.Round.Type.Up)}"); } Display(""); // Round down to nearest amount foreach (var value in values) { Display($"Value: {value}, Rounded: {Utils.Round.Amount(value, stepAmount, Utils.Round.Type.Down)}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Replace A Letter With Its Alphabet Position Using C#
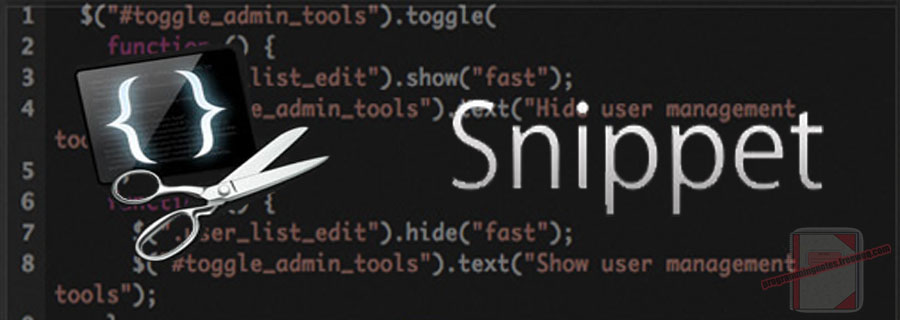
The following is a module with functions which demonstrates how to replace a letter with its alphabet position using C#.
1. Replace With Alphabet Position
The example below demonstrates the use of ‘Utils.Methods.GetAlphabetPosition‘ to replace a letter with its alphabet position.
1 2 3 4 5 6 7 8 9 10 11 12 |
// Replace With Alphabet Position // Get alphabet position var result = Utils.Methods.GetAlphabetPosition("The sunset sets at twelve o' clock."); // Display the results Console.WriteLine(string.Join(" ", result)); // expected output: /* 20 8 5 19 21 14 19 5 20 19 5 20 19 1 20 20 23 5 12 22 5 15 3 12 15 3 11 */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Collections.Generic; namespace Utils { public static class Methods { /// <summary> /// Gets the alphabet position of each character in a string /// </summary> /// <param name="text">The text to get the position</param> /// <returns>The alphabet position of each character</returns> public static List<int> GetAlphabetPosition(string text) { var alphabet = "abcdefghijklmnopqrstuvwxyz"; var result = new List<int>(); foreach (var letter in text.ToLower()) { var index = alphabet.IndexOf(letter); if (index > -1) { result.Add(index + 1); } } return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { static void Main(string[] args) { try { var result = Utils.Methods.GetAlphabetPosition("The sunset sets at twelve o' clock."); Display(string.Join(" ", result)); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Generate A Random String Of A Specified Length Using C#
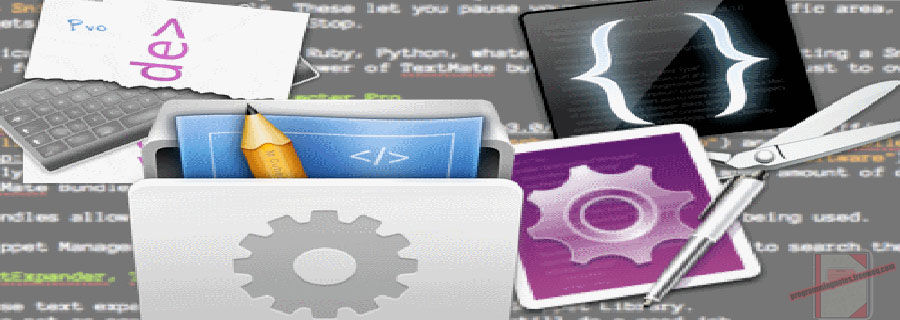
The following is a module with functions which demonstrates how to generate a random code of a specified length using C#.
The function demonstrated on this page has the ability to generate random strings that contains only letters, only numerical digits, or alphanumeric strings.
1. Random Code – Alphabetical
The example below demonstrates the use of ‘Utils.Code.GetRandom‘ to generate a code of a specified length that contains only letters.
The optional function parameter determines the type of code that is generated.
1 2 3 4 5 6 7 8 9 10 11 12 |
// Random Code - Alphabetical // Generate code containing only letters var letters = Utils.Code.GetRandom(5); // Display the code Console.WriteLine($"Code: {letters}"); // example output: /* Code: PBduQ */ |
2. Random Code – Numeric
The example below demonstrates the use of ‘Utils.Code.GetRandom‘ to generate a code of a specified length that contains only digits.
The optional function parameter determines the type of code that is generated.
1 2 3 4 5 6 7 8 9 10 11 12 |
// Random Code - Numeric // Generate code containing only digits var numeric = Utils.Code.GetRandom(7, Utils.Code.Type.Numeric); // Display the code Console.WriteLine($"Code: {numeric}"); // example output: /* Code: 1768033 */ |
3. Random Code – Alphanumeric
The example below demonstrates the use of ‘Utils.Code.GetRandom‘ to generate a code of a specified length that is alphanumeric.
The optional function parameter determines the type of code that is generated.
1 2 3 4 5 6 7 8 9 10 11 12 |
// Random Code - Alphanumeric // Generate alphanumeric code var alphaNumeric = Utils.Code.GetRandom(10, Utils.Code.Type.AlphaNumeric); // Display the code Console.WriteLine($"Code: {alphaNumeric}"); // example output: /* Code: iSL3bwu09O */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Code { public enum Type { /// <summary> /// Code contains only letters /// </summary> Alphabetical, /// <summary> /// Code contains only digits /// </summary> Numeric, /// <summary> /// Code contains letters and digits /// </summary> AlphaNumeric } /// <summary> /// Generates a random string of a specified length according to the type /// </summary> /// <param name="length">The length of the random string</param> /// <param name="type">The type of string to generate</param> /// <returns>The random string according to the type</returns> public static string GetRandom(int length , Code.Type type = Code.Type.Alphabetical) { var digits = "0123456789"; var alphabet = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"; var source = string.Empty; switch (type) { case Code.Type.Alphabetical: source = alphabet; break; case Code.Type.Numeric: source = digits; break; case Code.Type.AlphaNumeric: source = alphabet + digits; break; default: throw new ArgumentException($"Unknown type: {type}", nameof(type)); } var r = new Random(); var result = new System.Text.StringBuilder(); while (result.Length < length) { result.Append(source[r.Next(0, source.Length)]); } return result.ToString(); } } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 14, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; public class Program { static void Main(string[] args) { try { // Generate code containing only letters var letters = Utils.Code.GetRandom(5); // Display the code Display($"Code: {letters}"); Display(""); // Generate code containing only digits var numeric = Utils.Code.GetRandom(7, Utils.Code.Type.Numeric); // Display the code Display($"Code: {numeric}"); Display(""); // Generate alphanumeric code var alphaNumeric = Utils.Code.GetRandom(10, Utils.Code.Type.AlphaNumeric); // Display the code Display($"Code: {alphaNumeric}"); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Get A List Of Files At A Given Path Directory Using C#
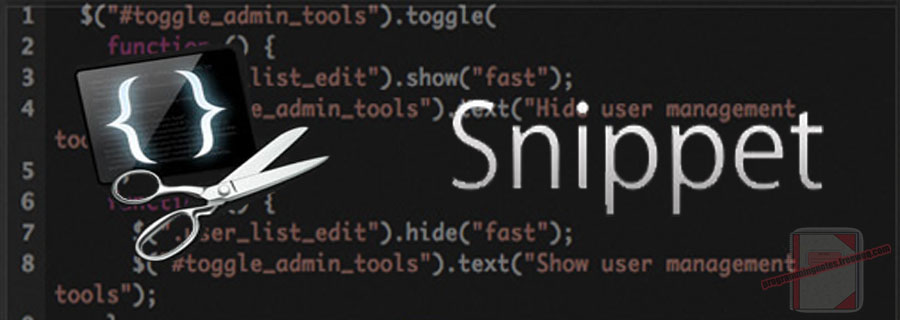
The following is a module with functions which demonstrates how to get a list of files at a given directory path using C#.
The function demonstrated on this page returns a list of System.IO.FileInfo, which contains information about the files in the given directory.
1. Get Files In Directory
The example below demonstrates the use of ‘Utils.Methods.GetFilesInDirectory‘ to get a list of files at a given path directory.
The optional function parameter lets you specify the search option. This lets you specify whether to limit the search to just the current directory, or expand the search to the current directory and all subdirectories when searching for files.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Get Files In Directory // Declare directory path var directory = $@"C:\Users\Name\Desktop"; // Get files at the specified directory var files = Utils.Methods.GetFilesInDirectory(directory); // Display info about the files foreach (var file in files) { Console.WriteLine($"File: {file.FullName} - Last Modified: {file.LastWriteTime}"); } // example output: /* File: C:\Users\Name\Desktop\text.txt - Last Modified: 10/7/2020 12:47:56 PM File: C:\Users\Name\Desktop\image.png - Last Modified: 9/4/2020 8:36:25 PM File: C:\Users\Name\Desktop\document.docx - Last Modified: 3/7/2018 9:26:19 PM */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; using System.Collections.Generic; namespace Utils { public static class Methods { /// <summary> /// Returns a list of <see cref="System.IO.FileInfo"/> of the files /// in the given directory /// </summary> /// <param name="directory">The relative or absolute path to the directory to search</param> /// <param name="searchOption">The file search option</param> /// <returns>A list of <see cref="System.IO.FileInfo"/> in the given directory</returns> public static List<System.IO.FileInfo> GetFilesInDirectory(string directory , System.IO.SearchOption searchOption = System.IO.SearchOption.TopDirectoryOnly) { return System.IO.Directory.GetFiles(directory, "*", searchOption) .Select(fileName => new System.IO.FileInfo(fileName)).ToList(); } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { static void Main(string[] args) { try { // Declare directory path var directory = $@"C:\path\to\directory"; // Get files at the specified directory var files = Utils.Methods.GetFilesInDirectory(directory); // Display info about the files foreach (var file in files) { Display($"File: {file.FullName} - Last Modified: {file.LastWriteTime}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Get The Computer & User Client IP Address Using C#
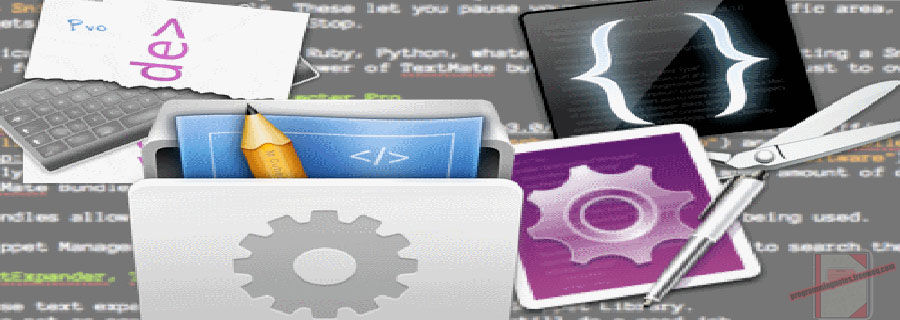
The following is a module with functions which demonstrates how to get the computers and user client request IPv4 IP address using C#.
The function demonstrated on this page returns the IPv4 address of the calling user. When under a web environment, it returns the clients System.Web.HttpContext.Current.Request IP address, otherwise it returns the IP address of the local machine (i.e: server) if there is no request.
The following function uses System.Web to determine the IP address of the client.
Note: To use the function in this module, make sure you have a reference to ‘System.Web‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add Reference‘, then type ‘System.Web‘ in the search box, and add the reference titled System.Web in the results Tab.
1. Get IP Address
The example below demonstrates the use of ‘Utils.Methods.GetIPv4Address‘ to get the IPv4 address of the calling user.
1 2 3 4 5 6 7 8 9 10 11 |
// Get IP Address // Get IP address of the current user var ipAddress = Utils.Methods.GetIPv4Address(); Console.WriteLine($"IP Address: {ipAddress}"); // example output: /* IP Address: 192.168.0.5 */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; namespace Utils { public static class Methods { /// <summary> /// Returns the IPv4 address of the calling user. When under a web /// environment, returns the <see cref="System.Web.HttpRequest"/> /// IP address, otherwise returns the IP address of the local machine /// </summary> /// <returns>The IPv4 address of the calling user</returns> public static string GetIPv4Address() { var ipAddress = string.Empty; // Get client ip address if (System.Web.HttpContext.Current != null && System.Web.HttpContext.Current.Request != null) { // Get client ip address using ServerVariables var request = System.Web.HttpContext.Current.Request; ipAddress = request.ServerVariables["HTTP_X_FORWARDED_FOR"]; if (!string.IsNullOrEmpty(ipAddress)) { var addresses = ipAddress.Split(",".ToCharArray(), StringSplitOptions.RemoveEmptyEntries); if (addresses.Length > 0) { ipAddress = addresses[0]; } } if (string.IsNullOrEmpty(ipAddress)) { ipAddress = request.ServerVariables["REMOTE_ADDR"]; } // Get client ip address using UserHostAddress if (string.IsNullOrEmpty(ipAddress)) { var clientIPA = GetNetworkAddress(request.UserHostAddress); if (clientIPA != null) { ipAddress = clientIPA.ToString(); } } } // Get local machine ip address if (string.IsNullOrEmpty(ipAddress)) { var loacalIPA = GetNetworkAddress(System.Net.Dns.GetHostName()); if (loacalIPA != null) { ipAddress = loacalIPA.ToString(); } } return ipAddress; } private static System.Net.IPAddress GetNetworkAddress(string addresses) { return System.Net.Dns.GetHostAddresses(addresses) .FirstOrDefault(x => x.AddressFamily == System.Net.Sockets.AddressFamily.InterNetwork); } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; public class Program { static void Main(string[] args) { try { // Get IP address of the current user var ipAddress = Utils.Methods.GetIPv4Address(); Display($"IP Address: {ipAddress}"); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Copy DataTable DataRow From One DataRow To Another Using C#
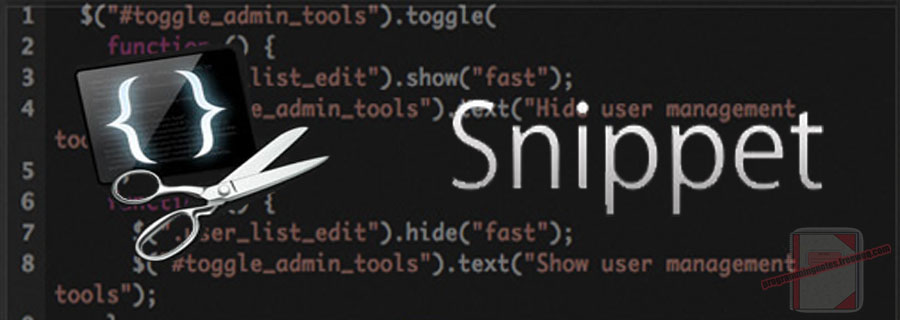
The following is a module with functions which demonstrates how to copy a DataTable DataRow from one DataRow to another using C#.
The function demonstrated on this page is an extension method, which copies all matching columns from the source DataRow to the destination DataRow. If no matching column exists between the two rows, the data at that column is skipped. This allows to safely copy a single row from one DataTable to other.
1. Copy DataRow
The example below demonstrates the use of ‘Utils.Collections.Extensions.CopyTo‘ to copy all matching columns from a source row to a destination row.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
// Copy DataRow using Utils.Collections; // Declare the source datatable var table1 = new DataTable(); table1.Columns.Add("Name", typeof(string)); table1.Rows.Add("Kenneth"); table1.Rows.Add("Jennifer"); table1.Rows.Add("Lynn"); table1.Rows.Add("Sole"); table1.Rows.Add(System.DBNull.Value); // Declare the destination datatable var table2 = new DataTable(); table2.Columns.Add("Id", typeof(int)); table2.Columns.Add("Name", typeof(string)); for (var index = 0; index < table1.Rows.Count; ++index) { // Get the source row var rowSource = table1.Rows[index]; // Get the destination row var rowDestination = table2.NewRow(); // Do something with the destination row rowDestination["Id"] = index + 1; // Copy contents from source to destination rowSource.CopyTo(rowDestination); table2.Rows.Add(rowDestination); } // Display information from destination table foreach (DataRow row in table2.Rows) { Console.WriteLine($"Id: {row["Id"]}, Name: {row["Name"]}"); } // expected output: /* Id: 1, Name: Kenneth Id: 2, Name: Jennifer Id: 3, Name: Lynn Id: 4, Name: Sole Id: 5, Name: */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Data; namespace Utils { namespace Collections { public static class Extensions { /// <summary> /// Copies all matching columns from the source row to the destination row /// </summary> /// <param name="source">The DataRow source</param> /// <param name="destination">The DataRow destination</param> public static void CopyTo(this DataRow source, DataRow destination) { foreach (DataColumn col in source.Table.Columns) { if (destination.Table.Columns.Contains(col.ColumnName)) { destination[col.ColumnName] = source[col.ColumnName]; } } } } } } |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils.Collections; using System.Data; public class Program { static void Main(string[] args) { try { // Declare the source datatable var table1 = new DataTable(); table1.Columns.Add("Name", typeof(string)); table1.Rows.Add("Kenneth"); table1.Rows.Add("Jennifer"); table1.Rows.Add("Lynn"); table1.Rows.Add("Sole"); table1.Rows.Add(System.DBNull.Value); // Declare the destination datatable var table2 = new DataTable(); table2.Columns.Add("Id", typeof(int)); table2.Columns.Add("Name", typeof(string)); for (var index = 0; index < table1.Rows.Count; ++index) { // Get the source row var rowSource = table1.Rows[index]; // Get the destination row var rowDestination = table2.NewRow(); // Do something with the destination row rowDestination["Id"] = index + 1; // Copy contents from source to destination rowSource.CopyTo(rowDestination); table2.Rows.Add(rowDestination); } // Display information from destination table foreach (DataRow row in table2.Rows) { Display($"Id: {row["Id"]}, Name: {row["Name"]}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Generate, Create & Read A QR Code Using C#
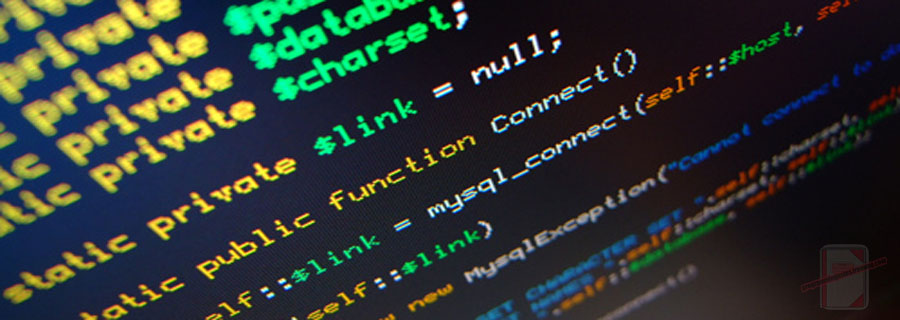
The following is a module with functions which demonstrates how to generate, create and read a QR code using C#.
The functions demonstrated on this page has the ability to create and encode a string to a QR code byte array, and another function to read and decode a byte array QR code to a string.
The following functions use ZXing.Net to create and read QR codes.
Note: To use the functions in this module, make sure you have the ‘ZXing.Net‘ package installed in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Manage NuGet Packages….‘, then type ‘ZXing.Net‘ in the search box, and install the package titled ZXing.Net in the results Tab.
1. Create QR Code – Encode
The example below demonstrates the use of ‘Utils.QRCode.Create‘ to create a QR code byte array from string data.
The optional function parameters allows you to specify the QR code height, width and margin.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Create QR Code - Encode // Data to encode var data = "https://www.programmingnotes.org/"; // Encode data to a QR code byte array var qrBytes = Utils.QRCode.Create(data); // Display bytes length Console.WriteLine($"Length: {qrBytes.Length}"); // expected output: /* Length: 1959 */ |
2. Read QR Code – Decode
The example below demonstrates the use of ‘Utils.QRCode.Read‘ to read a QR code byte array and decode its contents to a string.
1 2 3 4 5 6 7 8 9 |
// Read QR Code - Decode // Read QR as byte array byte[] qrBytes; // Decode QR code to a string var data = Utils.QRCode.Read(qrBytes); // ... Do something with the result string |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class QRCode { /// <summary> /// Converts a string and encodes it to a QR code byte array /// </summary> /// <param name="data">The data to encode</param> /// <param name="height">The height of the QR code</param> /// <param name="width">The width of the QR code</param> /// <param name="margin">The margin around the QR code</param> /// <returns>The byte array of the data encoded into a QR code</returns> public static byte[] Create(string data, int height = 100 , int width = 100, int margin = 0) { byte[] bytes = null; var barcodeWriter = new ZXing.BarcodeWriter() { Format = ZXing.BarcodeFormat.QR_CODE, Options = new ZXing.QrCode.QrCodeEncodingOptions() { Height = height, Width = width, Margin = margin } }; using (var image = barcodeWriter.Write(data)) { using (var stream = new System.IO.MemoryStream()) { image.Save(stream, System.Drawing.Imaging.ImageFormat.Png); bytes = stream.ToArray(); } } return bytes; } /// <summary> /// Converts a QR code and decodes it to its string data /// </summary> /// <param name="bytes">The QR code byte array</param> /// <returns>The string data decoded from the QR code</returns> public static string Read(byte[] bytes) { var result = string.Empty; using (var stream = new System.IO.MemoryStream(bytes)) { using (var image = System.Drawing.Image.FromStream(stream)) { var barcodeReader = new ZXing.BarcodeReader() { AutoRotate = true, TryInverted = true, Options = new ZXing.Common.DecodingOptions() { TryHarder = true, PossibleFormats = new ZXing.BarcodeFormat[] { ZXing.BarcodeFormat.QR_CODE } } }; var decoded = barcodeReader.Decode((System.Drawing.Bitmap)image); if (decoded != null) { result = decoded.Text; } } } return result; } } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; public class Program { static void Main(string[] args) { try { // Data to encode var data = "https://www.programmingnotes.org/"; // Encode data to a QR code byte array var bytes = Utils.QRCode.Create(data); Display($"Length: {bytes.Length}"); // Decode QR code to a string var result = Utils.QRCode.Read(bytes); Display($"Result: {result}"); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Append & Join A Date & Time Value Together Using C#
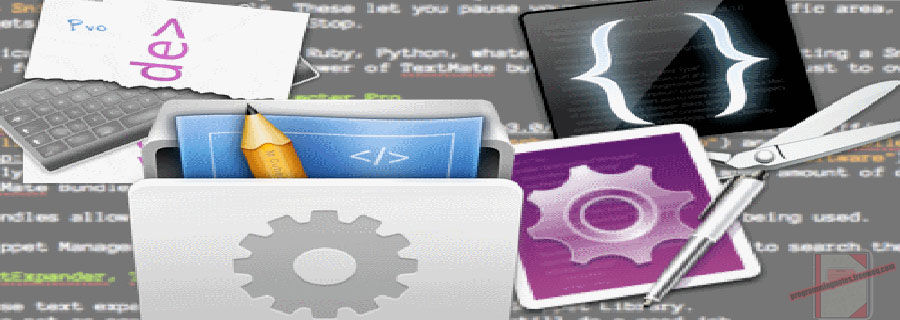
The following is a module with functions which demonstrates how to append and join a date and time value together using C#.
1. Append Date & Time
The example below demonstrates the use of ‘Utils.Extensions.SetTime‘ to append a date and time value together.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Append Date & Time using Utils var dteDate = Convert.ToDateTime("1/27/1991"); var time = Convert.ToDateTime("7:28:33 PM"); Console.WriteLine($"dteDate: {dteDate}"); // Append time to the date var result = dteDate.SetTime(time); Console.WriteLine($"Result: {result}"); // expected output: /* dteDate: 1/27/1991 12:00:00 AM Result: 1/27/1991 7:28:33 PM */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Extensions { /// <summary> /// Appends a date and time value together /// </summary> /// <param name="date">The date portion</param> /// <param name="time">The time portion</param> /// <returns>The appended date and time value</returns> public static DateTime SetTime(this DateTime date, DateTime time) { return date.Date.Add(time.TimeOfDay); } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { static void Main(string[] args) { try { var dteDate = Convert.ToDateTime("1/27/1991"); var time = Convert.ToDateTime("7:28:33 PM"); Display($"dteDate: {dteDate}"); // Append time to the date var result = dteDate.SetTime(time); Display($"Result: {result}"); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Generate Hourly Time Range With Minute Interval Using C#
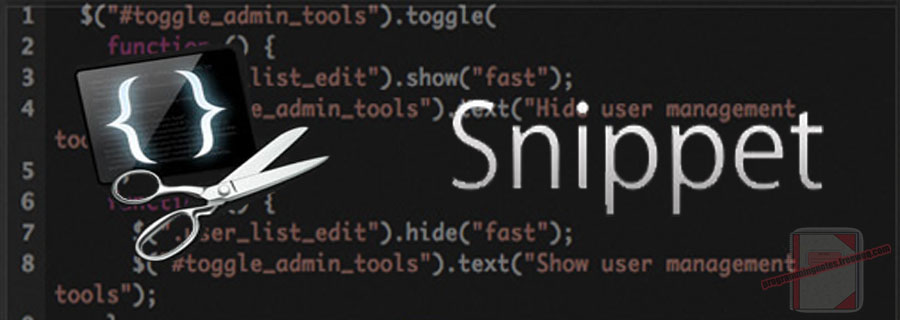
The following is a module with functions which demonstrates how to generate an hourly time range with minute interval between a start and end time using C#.
The function demonstrated in this page generates a time value list containing the time from a starting hour to an ending hour, separated by a minute step. The starting and ending hour is an integer from 0 through 23 representing the 24 hour period of the day, and the minute step can be any minute interval, including decimal values.
The starting and ending hours can also be flipped, indicating that the results should be returned in descending order.
An optional parameter also exists which allows to tune the results. When the range is in ascending order, the parameter indicates that the results should exclude/include the entire ending hour. When the range is in descending order, the parameter indicates that the results should exclude/include the entire starting hour.
1. Ascending Time Range – Minute Interval – Default
The example below demonstrates the use of ‘Utils.DateRange.GetTimeRange‘ to get the time range with a minute interval in ascending order.
In this example, the default options are used, which excludes the full ending hour from the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
// Ascending Time Range - Minute Interval - Default // Get an hour range from 12AM - 11PM, with a 15 and half minute interval var result = Utils.DateRange.GetTimeRange(0, 23, 15.5); foreach (var value in result) { Console.WriteLine($"Time: {value.Time}, String: {value.ToString()}"); } // expected output: /* Time: 1/1/0001 12:00:00 AM, String: 12:00:00 AM Time: 1/1/0001 12:15:30 AM, String: 12:15:30 AM Time: 1/1/0001 12:31:00 AM, String: 12:31:00 AM Time: 1/1/0001 12:46:30 AM, String: 12:46:30 AM Time: 1/1/0001 1:02:00 AM, String: 1:02:00 AM Time: 1/1/0001 1:17:30 AM, String: 1:17:30 AM Time: 1/1/0001 1:33:00 AM, String: 1:33:00 AM Time: 1/1/0001 1:48:30 AM, String: 1:48:30 AM .... .... .... .... Time: 1/1/0001 9:11:00 PM, String: 9:11:00 PM Time: 1/1/0001 9:26:30 PM, String: 9:26:30 PM Time: 1/1/0001 9:42:00 PM, String: 9:42:00 PM Time: 1/1/0001 9:57:30 PM, String: 9:57:30 PM Time: 1/1/0001 10:13:00 PM, String: 10:13:00 PM Time: 1/1/0001 10:28:30 PM, String: 10:28:30 PM Time: 1/1/0001 10:44:00 PM, String: 10:44:00 PM Time: 1/1/0001 10:59:30 PM, String: 10:59:30 PM */ |
2. Descending Time Range – Hourly Interval – Default
The example below demonstrates the use of ‘Utils.DateRange.GetTimeRange‘ to get the time range with a hourly interval in descending order.
In this example, the default options are used, which excludes the full starting hour from the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Descending Time Range - Hourly Interval - Default // Get an hour range from 10PM - 10AM, with a 2 hour interval var result = Utils.DateRange.GetTimeRange(22, 10, 120); foreach (var value in result) { Console.WriteLine($"Time: {value.Time}, String: {value.ToString(true)}"); } // expected output: /* Time: 1/1/0001 10:00:00 PM, String: 10 PM Time: 1/1/0001 8:00:00 PM, String: 8 PM Time: 1/1/0001 6:00:00 PM, String: 6 PM Time: 1/1/0001 4:00:00 PM, String: 4 PM Time: 1/1/0001 2:00:00 PM, String: 2 PM Time: 1/1/0001 12:00:00 PM, String: 12 PM Time: 1/1/0001 10:00:00 AM, String: 10 AM */ |
3. Ascending Time Range – Minute Interval – Include Full Hour
The example below demonstrates the use of ‘Utils.DateRange.GetTimeRange‘ to get the time range with a minute interval in ascending order.
In this example, the optional parameter is used, which includes the full ending hour from the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// Ascending Time Range - Minute Interval - Include Full Hour // Get an hour range from 12AM - 11PM, with a 15 and half minute interval var result = Utils.DateRange.GetTimeRange(0, 23, 15.5, true); foreach (var value in result) { Console.WriteLine($"Time: {value.Time}, String: {value.ToString()}"); } // expected output: /* Time: 1/1/0001 12:00:00 AM, String: 12:00:00 AM Time: 1/1/0001 12:15:30 AM, String: 12:15:30 AM Time: 1/1/0001 12:31:00 AM, String: 12:31:00 AM Time: 1/1/0001 12:46:30 AM, String: 12:46:30 AM Time: 1/1/0001 1:02:00 AM, String: 1:02:00 AM Time: 1/1/0001 1:17:30 AM, String: 1:17:30 AM Time: 1/1/0001 1:33:00 AM, String: 1:33:00 AM Time: 1/1/0001 1:48:30 AM, String: 1:48:30 AM .... .... .... .... Time: 1/1/0001 10:13:00 PM, String: 10:13:00 PM Time: 1/1/0001 10:28:30 PM, String: 10:28:30 PM Time: 1/1/0001 10:44:00 PM, String: 10:44:00 PM Time: 1/1/0001 10:59:30 PM, String: 10:59:30 PM Time: 1/1/0001 11:15:00 PM, String: 11:15:00 PM Time: 1/1/0001 11:30:30 PM, String: 11:30:30 PM Time: 1/1/0001 11:46:00 PM, String: 11:46:00 PM */ |
4. Descending Time Range – Hourly Interval – Include Full Hour
The example below demonstrates the use of ‘Utils.DateRange.GetTimeRange‘ to get the time range with a hourly interval in descending order.
In this example, the optional parameter is used, which includes the full starting hour from the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Descending Time Range - Hourly Interval - Include Full Hour // Get an hour range from 10PM - 10AM, with a 2 hour interval var result = Utils.DateRange.GetTimeRange(22, 10, 120, true); foreach (var value in result) { Console.WriteLine($"Time: {value.Time}, String: {value.ToString(true)}"); } // expected output: /* Time: 1/1/0001 10:59:00 PM, String: 10:59 PM Time: 1/1/0001 8:59:00 PM, String: 8:59 PM Time: 1/1/0001 6:59:00 PM, String: 6:59 PM Time: 1/1/0001 4:59:00 PM, String: 4:59 PM Time: 1/1/0001 2:59:00 PM, String: 2:59 PM Time: 1/1/0001 12:59:00 PM, String: 12:59 PM Time: 1/1/0001 10:59:00 AM, String: 10:59 AM */ |
5. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; using System.Collections.Generic; namespace Utils { public class DateRange { public DateTime Time { get; set; } protected bool showSeconds = false; public string ToString(bool condensed = false) { var str = Time.ToShortTimeString(); if (condensed) { str = str.Replace(":00", string.Empty); } else if (showSeconds) { str = Time.ToString("h:mm:ss tt"); } return str; } /// <summary> /// Generates a time value list containing the time from 'Starting Hour' /// to 'Ending Hour' separated by 'Minute Step'. 'Starting Hour' and 'Ending Hour' /// should be an integer from 0 through 23 representing the hour of the day. /// </summary> /// <param name="startingHour">The starting hour from 0 through 23</param> /// <param name="endingHour">The ending hour from 0 through 23</param> /// <param name="minuteStep">The minute step</param> /// <param name="includeFullHour">If going in ascending direction, indicates /// the results should include the entire 'Ending Hour'. If going in descending /// direction, indicates the results should include the entire 'Starting Hour'</param> /// <returns>The time value list from 'Starting Hour' to 'Ending Hour'</returns> public static List<DateRange> GetTimeRange(int startingHour, int endingHour , double minuteStep, bool includeFullHour = false) { var result = new List<DateRange>(); var maxHour = 23; var minHour = 0; var maxMinute = 59; var minMinute = 0; var startingMinute = minMinute; var endingMinute = maxMinute; var showSeconds = (int)minuteStep != minuteStep; if (minuteStep < 0) { minuteStep = 1; } // Flip results if going in opposite direction if (startingHour > endingHour) { minuteStep *= -1; startingMinute = maxMinute; endingMinute = minMinute; startingHour = Math.Min(startingHour, maxHour); endingHour = Math.Max(endingHour, minHour); } else { startingHour = Math.Max(startingHour, minHour); endingHour = Math.Min(endingHour, maxHour); } if (!includeFullHour) { startingMinute = 0; endingMinute = 0; } var today = DateTime.Today; var startTime = today.SetTime(Convert.ToDateTime($"{startingHour.ToString("00")}:{startingMinute.ToString("00")}")); var endTime = today.SetTime(Convert.ToDateTime($"{endingHour.ToString("00")}:{endingMinute.ToString("00")}")); var currentTime = startTime; while (startingHour < endingHour ? currentTime <= endTime : currentTime >= endTime) { result.Add(new DateRange() { Time = new DateTime(1, 1, 1).SetTime(currentTime), showSeconds = showSeconds }); currentTime = currentTime.AddMinutes(minuteStep); } return result; } } public static class Extensions { /// <summary> /// Appends a date and time value together /// </summary> /// <param name="date">The date portion</param> /// <param name="time">The time portion</param> /// <returns>The appended date and time value</returns> public static DateTime SetTime(this DateTime date, DateTime time) { return date.Date.Add(time.TimeOfDay); } } }// http://programmingnotes.org/ |
6. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { static void Main(string[] args) { try { // Get an hour range from 12AM - 11PM, with a 15 and half minute interval var result = Utils.DateRange.GetTimeRange(0, 23, 15.5); foreach (var value in result) { Display($"Time: {value.Time}, String: {value.ToString()}"); } Display(""); // Get an hour range from 10PM - 10AM, with a 2 hour interval var result2 = Utils.DateRange.GetTimeRange(22, 10, 120); foreach (var value in result2) { Display($"Time: {value.Time}, String: {value.ToString(true)}"); } Display(""); // Get an hour range from 12AM - 11PM, with a 15 and half minute interval var result3 = Utils.DateRange.GetTimeRange(0, 23, 15.5, true); foreach (var value in result3) { Display($"Time: {value.Time}, String: {value.ToString()}"); } Display(""); // Get an hour range from 10PM - 10AM, with a 2 hour interval var result4 = Utils.DateRange.GetTimeRange(22, 10, 120, true); foreach (var value in result4) { Display($"Time: {value.Time}, String: {value.ToString(true)}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Add & Use Custom Attributes To Class Properties Using C#
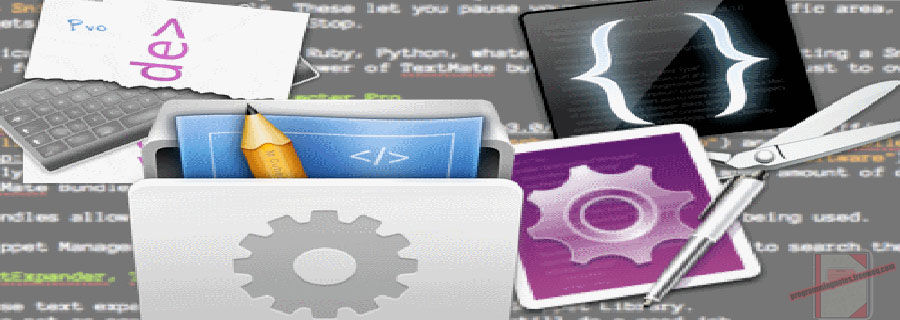
The following is a module with functions which demonstrates how to add and use custom attributes for class properties using C#.
The function demonstrated on this page is a generic extension method which uses reflection to get object property data transformed according to its custom attribute.
1. Custom Attribute
The example below demonstrates the use of ‘Utils.Extensions.GetPropertyData‘ to get object property data transformed according to its custom attribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// Custom Attribute using Utils // Create custom attribute class public class DateFormatAttribute : System.Attribute { public DateFormat Format { get; set; } } // An enum to describe the custom attribute options public enum DateFormat { Default, ToLongDateString, ToLongTimeString, ToShortDateString, ToShortTimeString } // Class that has a property that uses the custom attribute public class User { [DateFormatAttribute(Format = DateFormat.ToShortDateString)] public DateTime registered { get; set; } public string name { get; set; } } // Declare user var user = new User() { name = "Kenneth", registered = DateTime.Now }; // Get the properties with the processed attribute var result = user.GetPropertyData(); // Display Info foreach (var prop in result) { Console.WriteLine($"Property: {prop.Key}, Value: {prop.Value}"); } // expected output: /* Property: registered, Value: 5/12/2021 Property: name, Value: Kenneth */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; using System.Collections.Generic; namespace Utils { public class DateFormatAttribute : System.Attribute { public DateFormat Format { get; set; } } public enum DateFormat { Default, ToLongDateString, ToLongTimeString, ToShortDateString, ToShortTimeString } public static class Extensions { /// <summary> /// Returns an objects property data with the data transformed /// according to its attributes /// </summary> /// <returns>The objects property data</returns> public static Dictionary<string, object> GetPropertyData<T>(this T source) where T : class { var result = new Dictionary<string, object>(); var flags = System.Reflection.BindingFlags.Instance | System.Reflection.BindingFlags.Public; var properties = source.GetType().GetProperties(flags); foreach (var prop in properties) { var key = prop.Name; var value = prop.CanRead ? prop.GetValue(source , prop.GetIndexParameters().Count() == 1 ? new object[] { null } : null) : null; // Get custom attributes for the property var customAttributes = Attribute.GetCustomAttributes(prop); foreach (var attribute in customAttributes) { if (attribute.GetType().Equals(typeof(DateFormatAttribute))) { var dateValue = System.Convert.ToDateTime(value); switch (((DateFormatAttribute)attribute).Format) { case DateFormat.ToLongDateString: value = dateValue.ToLongDateString(); break; case DateFormat.ToLongTimeString: value = dateValue.ToLongTimeString(); break; case DateFormat.ToShortDateString: value = dateValue.ToShortDateString(); break; case DateFormat.ToShortTimeString: value = dateValue.ToShortTimeString(); break; default: value = dateValue.ToString(); break; } } } result.Add(key, value); } return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { public class User { [DateFormatAttribute(Format = DateFormat.ToShortDateString)] public DateTime registered { get; set; } public string name { get; set; } } static void Main(string[] args) { try { var user = new User() { name = "Kenneth", registered = DateTime.Now }; // Get the properties with the processed attribute var result = user.GetPropertyData(); foreach (var prop in result) { Display($"Property: {prop.Key}, Value: {prop.Value}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.