Monthly Archives: May 2021
C# || How To Remove Excess Whitespace From A String Using C#
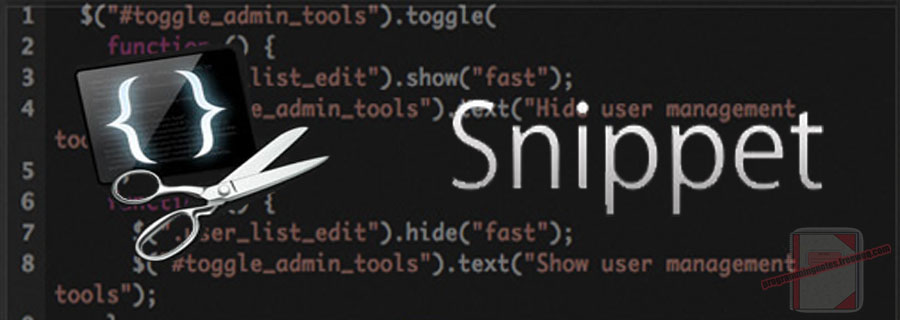
The following is a module with functions which demonstrates how to remove excess whitespace from a string, replacing multiple spaces into just one using C#.
1. Remove Excess Whitespace
The example below demonstrates the use of ‘Utils.Extensions.ToSingleSpace‘ to remove excess whitespace from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Remove Excess Whitespace using Utils; var word = @" This document uses 3 other documents "; // Creates single space string var result = word.ToSingleSpace(); Console.WriteLine(result); // expected output: /* This document uses 3 other documents */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Extensions { /// <summary> /// Removes excess whitespace from a string /// </summary> /// <param name="source">The source string</param> /// <returns>The modified source string</returns> public static string ToSingleSpace(this string source) { var pattern = @"\s\s+"; var result = System.Text.RegularExpressions.Regex.Replace(source, pattern, " ").Trim(); return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { static void Main(string[] args) { try { var word = @" This document uses 3 other documents "; // Creates single space string var result = word.ToSingleSpace(); Display(result); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Remove All Whitespace From A String Using C#
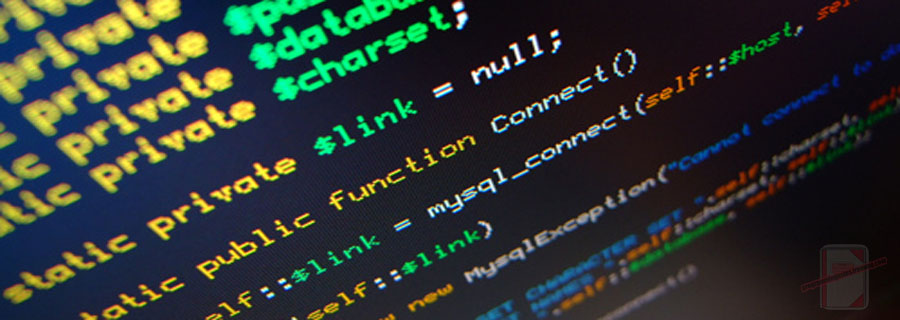
The following is a module with functions which demonstrates how to remove all whitespace from a string using C#.
1. Remove All Whitespace
The example below demonstrates the use of ‘Utils.Extensions.RemoveWhitespace‘ to remove all whitespace from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Remove All Whitespace using Utils; var word = $@" This document uses 3 other documents "; // Remove whitespace from the string var result = word.RemoveWhitespace(); Console.WriteLine(result); // expected output: /* Thisdocumentuses3otherdocuments */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Extensions { /// <summary> /// Removes all whitespace from a string /// </summary> /// <param name="source">The source string</param> /// <returns>The modified source string</returns> public static string RemoveWhitespace(this string source) { var pattern = @"\s"; var result = System.Text.RegularExpressions.Regex.Replace(source, pattern, string.Empty); return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { static void Main(string[] args) { try { var word = $@" This document uses 3 other documents "; // Remove whitespace from the string var result = word.RemoveWhitespace(); Display(result); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Remove Non Alphanumeric Characters From A String Using C#
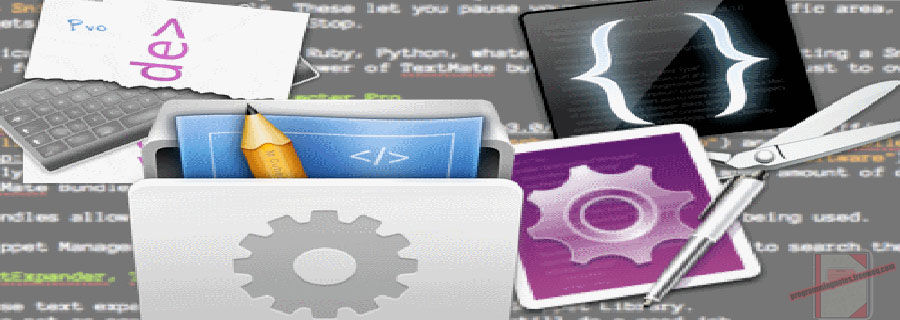
The following is a module with functions which demonstrates how to remove and replace non alphanumeric characters from a string using C#.
1. Alphanumeric Characters
The example below demonstrates the use of ‘Utils.Extensions.ToAlphaNumeric‘ to create an alphanumeric string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Alphanumeric Characters using Utils; var word = @"This-document_uses-3#other@documents!!!"; // Creates single space string var result = word.ToAlphaNumeric(); Console.WriteLine(result); // expected output: /* This document uses 3 other documents */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Extensions { /// <summary> /// Returns the string as alphanumeric /// </summary> /// <param name="source">The source string</param> /// <param name="replacement">The string replacement for invalid characters</param> /// <returns>The modified source string</returns> public static string ToAlphaNumeric(this string source, string replacement = " ") { var pattern = "[^a-zA-Z0-9]+"; var result = System.Text.RegularExpressions.Regex.Replace(source, pattern, replacement).Trim(); return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { static void Main(string[] args) { try { var word = @"This-document_uses-3#other@documents!!!"; // Creates single space string var result = word.ToAlphaNumeric(); Display(result); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Replace Entire Words In A String Using C#
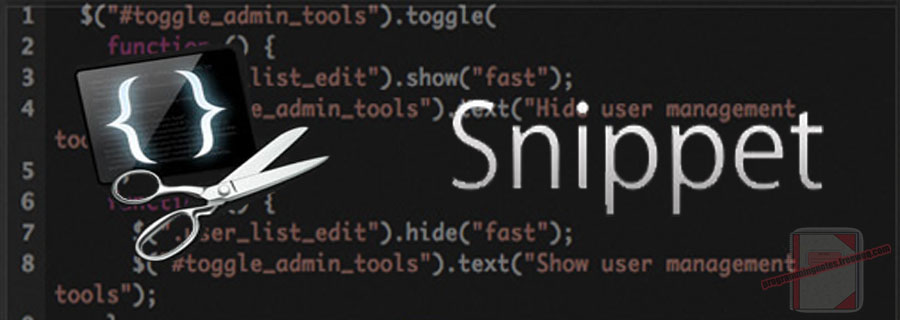
The following is a module with functions which demonstrates how to replace entire words in a string using C#.
This function is different from String.Replace in that instead of replacing all substring instances in a word, it replaces all instances of matching entire words in a string.
1. Replace Entire Word
The example below demonstrates the use of ‘Utils.Extensions.ReplaceWord‘ to replace an entire word.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Replace Entire Word using Utils; var word = "This docment uses 3 other docments to docment the docmentation"; // Replace the entire word in the string var result = word.ReplaceWord("docment", "document"); Console.WriteLine(result); // expected output: /* This document uses 3 other docments to document the docmentation */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Extensions { /// <summary> /// Replaces the entire word in the string /// </summary> /// <param name="source">The source string</param> /// <param name="wordToReplace">The word to replace</param> /// <param name="replacementWord">The replacement word</param> /// <param name="regexOptions">The search options</param> /// <returns>The modified source string</returns> public static string ReplaceWord(this string source, string wordToReplace, string replacementWord , System.Text.RegularExpressions.RegexOptions regexOptions = System.Text.RegularExpressions.RegexOptions.None) { var pattern = $@"\b{System.Text.RegularExpressions.Regex.Escape(wordToReplace)}\b"; var result = System.Text.RegularExpressions.Regex.Replace(source, pattern, replacementWord, regexOptions); return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { static void Main(string[] args) { try { var word = "This docment uses 3 other docments to docment the docmentation"; // Replace the entire word in the string var result = word.ReplaceWord("docment", "document"); Display(result); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Manually Copy Two Streams From One To Another Using C#
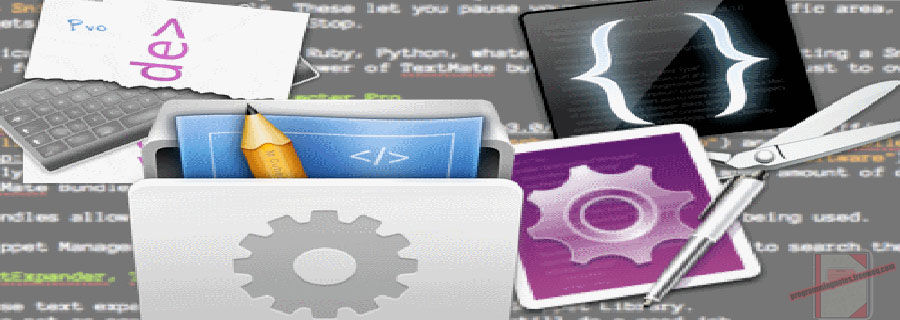
The following is a module with functions which demonstrates how to manually copy two steams from one to another using C#.
1. Manually Copy Stream
The example below demonstrates the use of ‘Utils.Extensions.CopyStream‘ to copy two streams.
1 2 3 4 5 6 7 8 9 |
// Manually Copy Stream using Utils; System.IO.Stream stream1; System.IO.Stream stream2; // Copy stream1 to stream2 stream1.CopyStream(stream2); |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Extensions { /// <summary> /// Reads bytes from the 'streamFrom' and writes them to 'streamTo' /// </summary> /// <param name="streamFrom">The source stream</param> /// <param name="streamTo">The destination stram</param> /// <returns>The total bytes written</returns> public static int CopyStream(this System.IO.Stream streamFrom, System.IO.Stream streamTo, int bufferSize = 4096) { int bytesWritten = 0; byte[] bytBuffer = new byte[bufferSize]; int bytesRead = 0; var tempPos = streamFrom.CanSeek ? (int?)streamFrom.Position : null; if (streamFrom.CanSeek) { streamFrom.Position = 0; } do { bytesRead = streamFrom.Read(bytBuffer, 0, bytBuffer.Length); if (bytesRead > 0) { streamTo.Write(bytBuffer, 0, bytesRead); bytesWritten += bytesRead; } } while (bytesRead > 0); if (tempPos.HasValue) { streamFrom.Position = tempPos.Value; } return bytesWritten; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 12, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using Utils; public class Program { static void Main(string[] args) { try { System.IO.Stream stream1; System.IO.Stream stream2; // Copy stream1 to stream2 stream1.CopyStream(stream2); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Copy All Properties & Fields From One Object To Another Using C#
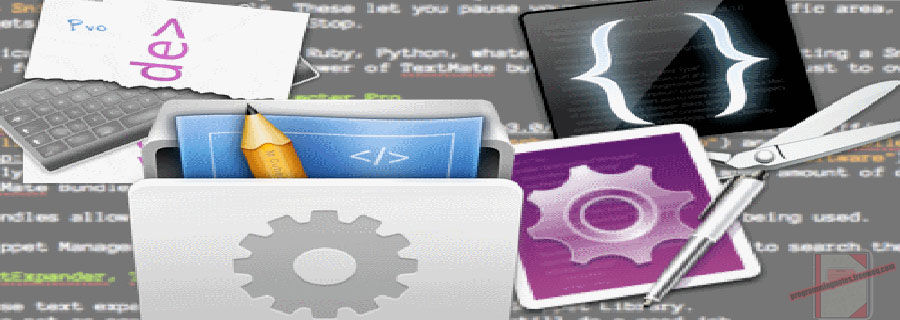
The following is a module with functions which demonstrates how to copy all properties and fields from one object to another using C#.
The function demonstrated on this page is a generic extension method which uses reflection to copy all the matching properties and fields from one object to another.
This function works on both structure and class object fields and properties.
The two objects do not have to be the same type. Only the matching properties and fields are copied.
1. Copy Properties & Fields
The example below demonstrates the use of ‘Utils.Objects.CopyPropsTo‘ to copy all the matching properties and fields from the source object to the destination object.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
// Copy Properties & Fields using Utils; public class Part { public string PartName { get; set; } public int PartId { get; set; } } public struct Part2 { public string PartName { get; set; } public int PartId; } // Declare source object var part1 = new Part() { PartName = "crank arm", PartId = 1234 }; // Declare destination object var part2 = new Part2(); // Copy matching properties and fields to destination part1.CopyPropsTo(ref part2); // Display information Console.WriteLine($"{part2.PartId} - {part2.PartName}"); // expected output: /* 1234 - crank arm */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 11, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Collections.Generic; using System.Linq; namespace Utils { public static class Objects { /// <summary> /// Copies all the matching properties and fields from 'source' to 'destination' /// </summary> /// <param name="source">The source object to copy from</param> /// <param name="destination">The destination object to copy to</param> public static void CopyPropsTo<T1, T2>(this T1 source, ref T2 destination) { var sourceMembers = GetMembers(source.GetType()); var destinationMembers = GetMembers(destination.GetType()); // Copy data from source to destination foreach (var sourceMember in sourceMembers) { if (!CanRead(sourceMember)) { continue; } var destinationMember = destinationMembers.FirstOrDefault(x => x.Name.ToLower() == sourceMember.Name.ToLower()); if (destinationMember == null || !CanWrite(destinationMember)) { continue; } SetObjectValue(ref destination, destinationMember, GetMemberValue(source, sourceMember)); } } private static void SetObjectValue<T>(ref T obj, System.Reflection.MemberInfo member, object value) { // Boxing method used for modifying structures var boxed = obj.GetType().IsValueType ? (object)obj : obj; SetMemberValue(ref boxed, member, value); obj = (T)boxed; } private static void SetMemberValue<T>(ref T obj, System.Reflection.MemberInfo member, object value) { if (IsProperty(member)) { var prop = (System.Reflection.PropertyInfo)member; if (prop.SetMethod != null) { prop.SetValue(obj, value); } } else if (IsField(member)) { var field = (System.Reflection.FieldInfo)member; field.SetValue(obj, value); } } private static object GetMemberValue(object obj, System.Reflection.MemberInfo member) { object result = null; if (IsProperty(member)) { var prop = (System.Reflection.PropertyInfo)member; result = prop.GetValue(obj, prop.GetIndexParameters().Count() == 1 ? new object[] { null } : null); } else if (IsField(member)) { var field = (System.Reflection.FieldInfo)member; result = field.GetValue(obj); } return result; } private static bool CanWrite(System.Reflection.MemberInfo member) { return IsProperty(member) ? ((System.Reflection.PropertyInfo)member).CanWrite : IsField(member); } private static bool CanRead(System.Reflection.MemberInfo member) { return IsProperty(member) ? ((System.Reflection.PropertyInfo)member).CanRead : IsField(member); } private static bool IsProperty(System.Reflection.MemberInfo member) { return IsType(member.GetType(), typeof(System.Reflection.PropertyInfo)); } private static bool IsField(System.Reflection.MemberInfo member) { return IsType(member.GetType(), typeof(System.Reflection.FieldInfo)); } private static bool IsType(System.Type type, System.Type targetType) { return type.Equals(targetType) || type.IsSubclassOf(targetType); } private static List<System.Reflection.MemberInfo> GetMembers(System.Type type) { var flags = System.Reflection.BindingFlags.Instance | System.Reflection.BindingFlags.Public | System.Reflection.BindingFlags.NonPublic; var members = new List<System.Reflection.MemberInfo>(); members.AddRange(type.GetProperties(flags)); members.AddRange(type.GetFields(flags)); return members; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 11, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; using Utils; public class Program { public class Part { public string PartName { get; set; } public int PartId { get; set; } } public struct Part2 { public string PartName { get; set; } public int PartId; } static void Main(string[] args) { try { // Declare source object var part1 = new Part() { PartName = "crank arm", PartId = 1234 }; // Declare destination object var part2 = new Part2(); // Copy matching properties and fields to destination part1.CopyPropsTo(ref part2); // Display information Console.WriteLine($"{part2.PartId} - {part2.PartName}"); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || Universal Object Serializer and Deserializer Using C#
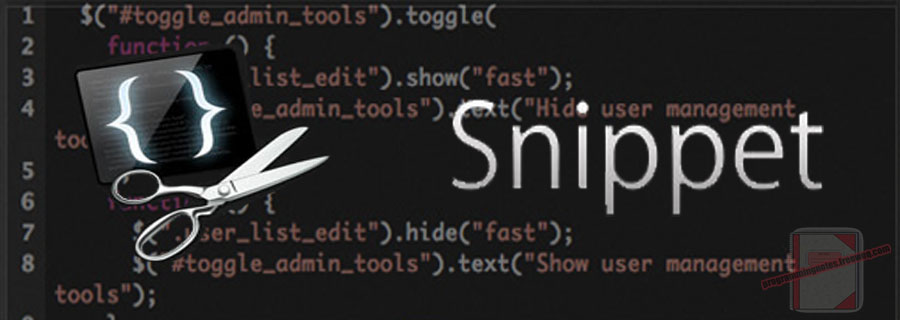
The following is a module with functions which demonstrates how to create a simple universal object serializer and deserializer using C#.
The following functions take in a instance of the abstract classes ‘ObjectSerializer‘, and ‘ObjectDeserializer‘. Those classes defines the functionality that is common to all the classes derived from it. They are what carries out the actual serialization and deserialization.
Breaking things up like so makes it easy to have different serializations with only calling one function.
Note: The functions in this module uses code from previous articles which explains how to serialize and deserialize objects.
For those examples of how to serialize and deserialize objects using Json and XML, see below:
1. JsonSerializer
The example below demonstrates the use of ‘Utils.Objects.JsonSerializer‘ to serialize an object to Json.
By changing the second parameter, calling the same function will change its behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
// JsonSerializer public class Part { public string PartName { get; set; } public int PartId { get; set; } } // Declare list of objects var parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } }; // Serialize to json var serialized = Utils.Objects.Serialize(parts, new Utils.Objects.JsonSerializer()); // Display json Console.WriteLine(serialized); // expected output: /* [ { "PartName": "crank arm", "PartId": 1234 }, { "PartName": "chain ring", "PartId": 1334 }, { "PartName": "regular seat", "PartId": 1434 }, { "PartName": "banana seat", "PartId": 1444 }, { "PartName": "cassette", "PartId": 1534 }, { "PartName": "shift lever", "PartId": 1634 } ] */ |
2. JsonDeserializer
The example below demonstrates the use of ‘Utils.Objects.JsonDeserializer‘ to deserialize an object from Json.
By changing the second parameter, calling the same function will change its behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
// JsonDeserializer // Declare Json array of objects var partsJson = @" [ { ""PartName"": ""crank arm"", ""PartId"": 1234 }, { ""PartName"": ""chain ring"", ""PartId"": 1334 }, { ""PartName"": ""regular seat"", ""PartId"": 1434 }, { ""PartName"": ""banana seat"", ""PartId"": 1444 }, { ""PartName"": ""cassette"", ""PartId"": 1534 }, { ""PartName"": ""shift lever"", ""PartId"": 1634 } ] "; // Deserialize to object var deserialized = Utils.Objects.Deserialize<List<Part>>(partsJson, new Utils.Objects.JsonDeserializer()); // Display the items foreach (var item in deserialized) { Console.WriteLine($"{item.PartId} - {item.PartName}"); } // expected output: /* 1234 - crank arm 1334 - chain ring 1434 - regular seat 1444 - banana seat 1534 - cassette 1634 - shift lever */ |
3. XmlSerializer
The example below demonstrates the use of ‘Utils.Objects.XmlSerializer‘ to serialize an object to Xml.
By changing the second parameter, calling the same function will change its behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
// XmlSerializer public class Part { public string PartName { get; set; } public int PartId { get; set; } } // Declare list of objects var parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } }; // Serialize to xml var serialized2 = Utils.Objects.Serialize(parts, new Utils.Objects.XmlSerializer()); // Display xml Console.WriteLine(serialized2); // expected output: /* <?xml version="1.0"?> <ArrayOfPart xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </ArrayOfPart> */ |
4. XmlDeserializer
The example below demonstrates the use of ‘Utils.Objects.XmlDeserializer‘ to deserialize an object from xml.
By changing the second parameter, calling the same function will change its behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
// XmlDeserializer // Declare Xml array of objects var partsXml = $@"<?xml version=""1.0""?> <ArrayOfPart xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </ArrayOfPart> "; // Deserialize to object var deserialized2 = Utils.Objects.Deserialize<List<Part>>(partsXml, new Utils.Objects.XmlDeserializer()); // Display the items foreach (var item in deserialized2) { Console.WriteLine($"{item.PartId} - {item.PartName}"); } // expected output: /* 1234 - crank arm 1334 - chain ring 1434 - regular seat 1444 - banana seat 1534 - cassette 1634 - shift lever */ |
5. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 11, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Objects { /// <summary> /// Serializes the specified value according to the ObjectSerializer /// </summary> /// <param name="value">The value to serialize</param> /// <param name="serializer">Determines how the value should be serialized</param> /// <returns>The value serialized according to the ObjectSerializer</returns> public static string Serialize<T>(T value, ObjectSerializer serializer) { return serializer.Serialize(value); } /// <summary> /// Deserializes the specified value according to the ObjectDeserializer /// </summary> /// <param name="value">The value to deserialize</param> /// <param name="deserializer">Determines how the value should be deserialized</param> /// <returns>The value deserialized according to the ObjectSerializer</returns> public static T Deserialize<T>(string value, ObjectDeserializer deserializer) { return deserializer.Deserialize<T>(value); } #region "ObjectSerializer" /// <summary> /// Defines the object serializer behavior /// </summary> public abstract class ObjectSerializer { public abstract string Serialize<T>(T value); } public class JsonSerializer : ObjectSerializer { public Newtonsoft.Json.JsonSerializerSettings settings { get; set; } = new Newtonsoft.Json.JsonSerializerSettings(); public JsonSerializer() { settings.NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore; settings.Formatting = Newtonsoft.Json.Formatting.Indented; } public override string Serialize<T>(T value) { return Utils.Json.Serialize(value, settings); } } public class XmlSerializer : ObjectSerializer { public System.Xml.Serialization.XmlRootAttribute root { get; set; } = new System.Xml.Serialization.XmlRootAttribute(); public override string Serialize<T>(T value) { return Utils.Xml.Serialize(value, root); } } #endregion #region "ObjectDeserializer" /// <summary> /// Defines the object deserializer behavior /// </summary> public abstract class ObjectDeserializer { public abstract T Deserialize<T>(string value); } public class JsonDeserializer : ObjectDeserializer { public Newtonsoft.Json.JsonSerializerSettings settings { get; set; } = new Newtonsoft.Json.JsonSerializerSettings(); public JsonDeserializer() { settings.NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore; } public override T Deserialize<T>(string value) { T obj = default; if (!string.IsNullOrEmpty(value)) { obj = Utils.Json.Deserialize<T>(value, settings); } return obj; } } public class XmlDeserializer : ObjectDeserializer { public System.Xml.Serialization.XmlRootAttribute root { get; set; } = new System.Xml.Serialization.XmlRootAttribute(); public override T Deserialize<T>(string value) { T obj = default; if (!string.IsNullOrEmpty(value)) { obj = Utils.Xml.Deserialize<T>(value, root); } return obj; } } #endregion } }// http://programmingnotes.org/ |
6. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 11, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { public class Part { public string PartName { get; set; } public int PartId { get; set; } } static void Main(string[] args) { try { // Declare list of objects var parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } }; // Serialize to json var serialized = Utils.Objects.Serialize(parts, new Utils.Objects.JsonSerializer()); // Display json Display(serialized); // Declare Json array of objects var partsJson = @" [ { ""PartName"": ""crank arm"", ""PartId"": 1234 }, { ""PartName"": ""chain ring"", ""PartId"": 1334 }, { ""PartName"": ""regular seat"", ""PartId"": 1434 }, { ""PartName"": ""banana seat"", ""PartId"": 1444 }, { ""PartName"": ""cassette"", ""PartId"": 1534 }, { ""PartName"": ""shift lever"", ""PartId"": 1634 } ] "; // Deserialize to object var deserialized = Utils.Objects.Deserialize<List<Part>>(partsJson, new Utils.Objects.JsonDeserializer()); // Display the items foreach (var item in deserialized) { Display($"{item.PartId} - {item.PartName}"); } Display(""); // Serialize to xml var serialized2 = Utils.Objects.Serialize(parts, new Utils.Objects.XmlSerializer()); // Display xml Display(serialized2); // Declare Xml array of objects var partsXml = $@"<?xml version=""1.0""?> <ArrayOfPart xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </ArrayOfPart> "; // Deserialize to object var deserialized2 = Utils.Objects.Deserialize<List<Part>>(partsXml, new Utils.Objects.XmlDeserializer()); // Display the items foreach (var item in deserialized2) { Display($"{item.PartId} - {item.PartName}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Send, Post & Process A REST API Web Request Using C#
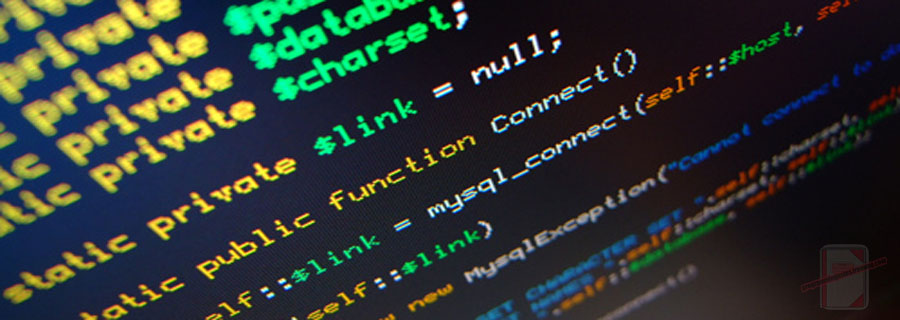
The following is a module with functions which demonstrates how to send and receive a RESTful web request using C#.
Contents
1. Overview
2. WebRequest - GET
3. WebRequest - POST
4. WebRequest - PUT
5. WebRequest - PATCH
6. WebRequest - DELETE
7. Utils Namespace
8. More Examples
1. Overview
The following functions use System.Net.HttpWebRequest and System.Net.HttpWebResponse to send and process requests. They can be called synchronously or asynchronously. This page will demonstrate using the asynchronous function calls.
The examples on this page will call a test API, and the resulting calls will return Json results.
The Json objects we are sending to the API are hard coded in the examples below. In a real world application, the objects would be serialized first before they are sent over the network, and then deserialized once a response is received. For simplicity, those operations are hard coded.
For examples of how to serialize and deserialize objects using Json and XML, see below:
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
2. WebRequest – GET
The example below demonstrates the use of ‘Utils.WebRequest.Get‘ to execute a GET request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 |
// WebRequest - GET // Declare url var url = "https://jsonplaceholder.typicode.com/users"; // Optional: Specify request options var options = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Execute a get request at the following url var response = await Utils.WebRequest.GetAsync(url); // Display the status code and response body Console.WriteLine($@" Status: {(int)response.Result.StatusCode} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} "); // .... Do something with response.Body like deserialize it to an object // expected output: /* Status: 200 - OK Bytes Received: 5645 Body: [ { "id": 1, "name": "Leanne Graham", "username": "Bret", "email": "Sincere@april.biz", "address": { "street": "Kulas Light", "suite": "Apt. 556", "city": "Gwenborough", "zipcode": "92998-3874", "geo": { "lat": "-37.3159", "lng": "81.1496" } }, "phone": "1-770-736-8031 x56442", "website": "hildegard.org", "company": { "name": "Romaguera-Crona", "catchPhrase": "Multi-layered client-server neural-net", "bs": "harness real-time e-markets" } }, { "id": 2, "name": "Ervin Howell", "username": "Antonette", "email": "Shanna@melissa.tv", "address": { "street": "Victor Plains", "suite": "Suite 879", "city": "Wisokyburgh", "zipcode": "90566-7771", "geo": { "lat": "-43.9509", "lng": "-34.4618" } }, "phone": "010-692-6593 x09125", "website": "anastasia.net", "company": { "name": "Deckow-Crist", "catchPhrase": "Proactive didactic contingency", "bs": "synergize scalable supply-chains" } }, { "id": 3, "name": "Clementine Bauch", "username": "Samantha", "email": "Nathan@yesenia.net", "address": { "street": "Douglas Extension", "suite": "Suite 847", "city": "McKenziehaven", "zipcode": "59590-4157", "geo": { "lat": "-68.6102", "lng": "-47.0653" } }, "phone": "1-463-123-4447", "website": "ramiro.info", "company": { "name": "Romaguera-Jacobson", "catchPhrase": "Face to face bifurcated interface", "bs": "e-enable strategic applications" } }, { "id": 4, "name": "Patricia Lebsack", "username": "Karianne", "email": "Julianne.OConner@kory.org", "address": { "street": "Hoeger Mall", "suite": "Apt. 692", "city": "South Elvis", "zipcode": "53919-4257", "geo": { "lat": "29.4572", "lng": "-164.2990" } }, "phone": "493-170-9623 x156", "website": "kale.biz", "company": { "name": "Robel-Corkery", "catchPhrase": "Multi-tiered zero tolerance productivity", "bs": "transition cutting-edge web services" } }, { "id": 5, "name": "Chelsey Dietrich", "username": "Kamren", "email": "Lucio_Hettinger@annie.ca", "address": { "street": "Skiles Walks", "suite": "Suite 351", "city": "Roscoeview", "zipcode": "33263", "geo": { "lat": "-31.8129", "lng": "62.5342" } }, "phone": "(254)954-1289", "website": "demarco.info", "company": { "name": "Keebler LLC", "catchPhrase": "User-centric fault-tolerant solution", "bs": "revolutionize end-to-end systems" } }, { "id": 6, "name": "Mrs. Dennis Schulist", "username": "Leopoldo_Corkery", "email": "Karley_Dach@jasper.info", "address": { "street": "Norberto Crossing", "suite": "Apt. 950", "city": "South Christy", "zipcode": "23505-1337", "geo": { "lat": "-71.4197", "lng": "71.7478" } }, "phone": "1-477-935-8478 x6430", "website": "ola.org", "company": { "name": "Considine-Lockman", "catchPhrase": "Synchronised bottom-line interface", "bs": "e-enable innovative applications" } }, { "id": 7, "name": "Kurtis Weissnat", "username": "Elwyn.Skiles", "email": "Telly.Hoeger@billy.biz", "address": { "street": "Rex Trail", "suite": "Suite 280", "city": "Howemouth", "zipcode": "58804-1099", "geo": { "lat": "24.8918", "lng": "21.8984" } }, "phone": "210.067.6132", "website": "elvis.io", "company": { "name": "Johns Group", "catchPhrase": "Configurable multimedia task-force", "bs": "generate enterprise e-tailers" } }, { "id": 8, "name": "Nicholas Runolfsdottir V", "username": "Maxime_Nienow", "email": "Sherwood@rosamond.me", "address": { "street": "Ellsworth Summit", "suite": "Suite 729", "city": "Aliyaview", "zipcode": "45169", "geo": { "lat": "-14.3990", "lng": "-120.7677" } }, "phone": "586.493.6943 x140", "website": "jacynthe.com", "company": { "name": "Abernathy Group", "catchPhrase": "Implemented secondary concept", "bs": "e-enable extensible e-tailers" } }, { "id": 9, "name": "Glenna Reichert", "username": "Delphine", "email": "Chaim_McDermott@dana.io", "address": { "street": "Dayna Park", "suite": "Suite 449", "city": "Bartholomebury", "zipcode": "76495-3109", "geo": { "lat": "24.6463", "lng": "-168.8889" } }, "phone": "(775)976-6794 x41206", "website": "conrad.com", "company": { "name": "Yost and Sons", "catchPhrase": "Switchable contextually-based project", "bs": "aggregate real-time technologies" } }, { "id": 10, "name": "Clementina DuBuque", "username": "Moriah.Stanton", "email": "Rey.Padberg@karina.biz", "address": { "street": "Kattie Turnpike", "suite": "Suite 198", "city": "Lebsackbury", "zipcode": "31428-2261", "geo": { "lat": "-38.2386", "lng": "57.2232" } }, "phone": "024-648-3804", "website": "ambrose.net", "company": { "name": "Hoeger LLC", "catchPhrase": "Centralized empowering task-force", "bs": "target end-to-end models" } } ] */ |
3. WebRequest – POST
The example below demonstrates the use of ‘Utils.WebRequest.Post‘ to execute a POST request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
// WebRequest - POST // Declare url var url = $"https://jsonplaceholder.typicode.com/users"; // Optional: Specify request options var options = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", ContentType = Utils.WebRequest.ContentType.ApplicationJson, Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Serialize object to get the Json to create a new employee var payload = @" { ""id"": 0, ""name"": ""Kenneth Perkins"", ""username"": null, ""email"": null, ""address"": null, ""phone"": null, ""website"": null, ""company"": null } "; // Execute a post request at the following url var response = await Utils.WebRequest.PostAsync(url, payload, options); // Display the status code and response body Console.WriteLine($@" Status: {(int)response.Result.StatusCode} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} "); // .... Do something with response.Body like deserialize it to an object // expected output: /* Status: 201 - Created Bytes Received: 154 Body: { "id": 11, "name": "Kenneth Perkins", "username": null, "email": null, "address": null, "phone": null, "website": null, "company": null } */ |
4. WebRequest – PUT
The example below demonstrates the use of ‘Utils.WebRequest.Put‘ to execute a PUT request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// WebRequest - PUT // Declare url var url = $"https://jsonplaceholder.typicode.com/users/1"; // Optional: Specify request options var options = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", ContentType = Utils.WebRequest.ContentType.ApplicationJson, Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Serialize object to get the Json to update an existing employee var payload = @" { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } "; // Execute a put request at the following url var response = await Utils.WebRequest.PutAsync(url, payload, options); // Display the status code and response body Console.WriteLine($@" Status: {(int)response.Result.StatusCode} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} "); // .... Do something with response.Body like deserialize it to an object // expected output: /* Status: 200 - OK Bytes Received: 87 Body: { "name": "Kenneth Perkins", "website": "https://www.programmingnotes.org/", "id": 1 } */ |
5. WebRequest – PATCH
The example below demonstrates the use of ‘Utils.WebRequest.Patch‘ to execute a PATCH request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
// WebRequest - PATCH // Declare url var url = $"https://jsonplaceholder.typicode.com/users/1"; // Optional: Specify request options var options = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", ContentType = Utils.WebRequest.ContentType.ApplicationJson, Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Serialize object to get the Json to update an existing employee var payload = @" { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } "; // Execute a put request at the following url var response = await Utils.WebRequest.PatchAsync(url, payload, options); // Display the status code and response body Console.WriteLine($@" Status: {(int)response.Result.StatusCode} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} "); // .... Do something with response.Body like deserialize it to an object // expected output: /* Status: 200 - OK Bytes Received: 526 Body: { "id": 1, "name": "Kenneth Perkins", "username": "Bret", "email": "Sincere@april.biz", "address": { "street": "Kulas Light", "suite": "Apt. 556", "city": "Gwenborough", "zipcode": "92998-3874", "geo": { "lat": "-37.3159", "lng": "81.1496" } }, "phone": "1-770-736-8031 x56442", "website": "https://www.programmingnotes.org/", "company": { "name": "Romaguera-Crona", "catchPhrase": "Multi-layered client-server neural-net", "bs": "harness real-time e-markets" } } */ |
6. WebRequest – DELETE
The example below demonstrates the use of ‘Utils.WebRequest.Delete‘ to execute a DELETE request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// WebRequest - DELETE // Declare url var url = $"https://jsonplaceholder.typicode.com/users/1"; // Optional: Specify request options var options = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Execute a delete request at the following url var response = await Utils.WebRequest.DeleteAsync(url, options); // Display the status code and response body Console.WriteLine($@" Status: {(int)response.Result.StatusCode} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} "); // .... Do something with response.Body like deserialize it to an object // expected output: /* Status: 200 - OK Bytes Received: 2 Body: {} */ |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 11, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; using System.Threading.Tasks; namespace Utils { public static class WebRequest { /// <summary> /// Executes a GET request on the given url /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Response Get(string url, Options options = null) { return GetAsync(url, options: options).Result; } /// <summary> /// Executes a GET request on the given url as an asynchronous operation /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> GetAsync(string url, Options options = null) { return ExecuteAsync(Method.Get, url, payload: (byte[])null, options: options); } /// <summary> /// Executes a POST request on the given url /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to post to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Response Post(string url, string payload, Options options = null) { return Post(url, payload: payload.GetBytes(), options: options); } /// <summary> /// Executes a POST request on the given url /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to post to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Response Post(string url, byte[] payload, Options options = null) { return PostAsync(url, payload: payload, options: options).Result; } /// <summary> /// Executes a POST request on the given url as an asynchronous operation /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to post to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> PostAsync(string url, string payload, Options options = null) { return PostAsync(url, payload: payload.GetBytes(), options: options); } /// <summary> /// Executes a POST request on the given url as an asynchronous operation /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to post to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> PostAsync(string url, byte[] payload, Options options = null) { return ExecuteAsync(Method.Post, url, payload: payload, options: options); } /// <summary> /// Executes a PUT request on the given url /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to put to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Response Put(string url, string payload, Options options = null) { return Put(url, payload: payload.GetBytes(), options: options); } /// <summary> /// Executes a PUT request on the given url /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to put to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Response Put(string url, byte[] payload, Options options = null) { return PutAsync(url, payload: payload, options: options).Result; } /// <summary> /// Executes a PUT request on the given url as an asynchronous operation /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to put to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> PutAsync(string url, string payload, Options options = null) { return PutAsync(url, payload: payload.GetBytes(), options: options); } /// <summary> /// Executes a PUT request on the given url as an asynchronous operation /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to put to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> PutAsync(string url, byte[] payload, Options options = null) { return ExecuteAsync(Method.Put, url, payload: payload, options: options); } /// <summary> /// Executes a PATCH request on the given url /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to patch to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Response Patch(string url, string payload, Options options = null) { return Patch(url, payload: payload.GetBytes(), options: options); } /// <summary> /// Executes a PATCH request on the given url /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to patch to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Response Patch(string url, byte[] payload, Options options = null) { return PatchAsync(url, payload: payload, options: options).Result; } /// <summary> /// Executes a PATCH request on the given url as an asynchronous operation /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to patch to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> PatchAsync(string url, string payload, Options options = null) { return PatchAsync(url, payload: payload.GetBytes(), options: options); } /// <summary> /// Executes a PATCH request on the given url as an asynchronous operation /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to patch to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> PatchAsync(string url, byte[] payload, Options options = null) { return ExecuteAsync(Method.Patch, url, payload: payload, options: options); } /// <summary> /// Executes a DELETE request on the given url /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Response Delete(string url, Options options = null) { return DeleteAsync(url, options: options).Result; } /// <summary> /// Executes a DELETE request on the given url as an asynchronous operation /// </summary> /// <param name="url">The url to navigate to</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> DeleteAsync(string url, Options options = null) { return ExecuteAsync(Method.Delete, url, payload: (byte[])null, options: options); } /// <summary> /// Executes a request method on the given url as an asynchronous operation /// </summary> /// <param name="type">The type of request method to execute</param> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to send to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public static Task<Response> ExecuteAsync(Method type , string url , string payload = null, Options options = null) { return ExecuteAsync(type, url, payload: (byte[])payload?.GetBytes(), options: options); } /// <summary> /// Executes a request method on the given url as an asynchronous operation /// </summary> /// <param name="type">The type of request method to execute</param> /// <param name="url">The url to navigate to</param> /// <param name="payload">The data to send to the specified resource</param> /// <param name="options">The options for the web request</param> /// <returns>The result of the given request</returns> public async static Task<Response> ExecuteAsync(Method type, string url , byte[] payload = null, Options options = null) { var request = (System.Net.HttpWebRequest)System.Net.WebRequest.Create(url); if (options != null) { request.CopyProperties(options); } request.Method = type.ToString().ToUpper(); if (payload != null) { request.ContentLength = payload.Length; using (var requestStream = request.GetRequestStream()) { using (var payloadStream = new System.IO.MemoryStream(payload)) { payloadStream.CopyTo(requestStream); } } } System.Net.HttpWebResponse webResponse = null; try { webResponse = (System.Net.HttpWebResponse)await request.GetResponseAsync(); } catch (System.Net.WebException ex) { if (ex.Response == null) { throw; } webResponse = (System.Net.HttpWebResponse)ex.Response; } catch { throw; } var result = new Response() { Result = webResponse, Bytes = GetBytes(webResponse) }; return result; } private static byte[] GetBytes(System.Net.HttpWebResponse response) { byte[] bytes; var responseStream = response.GetResponseStream(); using (var memoryStream = new System.IO.MemoryStream()) { responseStream.CopyTo(memoryStream); bytes = memoryStream.ToArray(); } return bytes; } public static byte[] GetBytes(this string str, System.Text.Encoding encode = null) { if (encode == null) { encode = GetDefaultEncoding(); } return encode.GetBytes(str); } public static string GetString(this byte[] bytes, System.Text.Encoding encode = null) { if (encode == null) { encode = GetDefaultEncoding(); } return encode.GetString(bytes); } private static System.Text.Encoding GetDefaultEncoding() { var encode = new System.Text.UTF8Encoding(); return encode; } private static void CopyProperties<T1, T2>(this T1 dest, T2 src) { var srcProps = src.GetType().GetProperties(); var destProps = dest.GetType().GetProperties(); foreach (var srcProp in srcProps) { if (srcProp.CanRead) { var destProp = destProps.FirstOrDefault(x => x.Name == srcProp.Name); if (destProp != null && destProp.CanWrite) { var value = srcProp.GetValue(src, srcProp.GetIndexParameters().Count() == 1 ? new object[] { null } : null); destProp.SetValue(dest, value); } } } } /// <summary> /// The response result of a <see cref="System.Net.HttpWebRequest"/> /// </summary> public class Response { public System.Net.HttpWebResponse Result { get; set; } = null; public byte[] Bytes { get; set; } = null; public string Body { get { if (_body == null && Bytes != null) { _body = Bytes.GetString(); } return _body; } } private string _body = null; } /// <summary> /// Options for the given <see cref="System.Net.HttpWebRequest"/> /// </summary> public class Options { public System.Net.WebHeaderCollection Headers { get; set; } = new System.Net.WebHeaderCollection(); public System.Net.ICredentials Credentials { get; set; } = null; public string Connection { get; set; } = null; public bool KeepAlive { get; set; } = true; public string Expect { get; set; } = null; public DateTime IfModifiedSince { get; set; } public string TransferEncoding { get; set; } public string Accept { get; set; } = null; public bool AllowAutoRedirect { get; set; } = true; public bool AllowReadStreamBuffering { get; set; } = false; public bool AllowWriteStreamBuffering { get; set; } = true; public int MaximumAutomaticRedirections { get; set; } = 50; public string MediaType { get; set; } = null; public bool Pipelined { get; set; } = true; public bool PreAuthenticate { get; set; } = false; public string Referer { get; set; } = null; public bool SendChunked { get; set; } = false; public bool UseDefaultCredentials { get; set; } = false; public string UserAgent { get; set; } = null; public string ContentType { get; set; } = null; public int Timeout { get; set; } = 100000; public int ReadWriteTimeout { get; set; } = 300000; } public abstract class ContentType { public const string ApplicationUrlEncoded = "application/x-www-form-urlencoded"; public const string ApplicationJson = "application/json"; public const string TextXml = "text/xml"; } public enum Method { Get, Post, Put, Patch, Delete } } }// http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 11, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; using System.Threading.Tasks; public class Program { static void Main(string[] args) { try { ProcessRequests().Wait(); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } public static async Task ProcessRequests() { // Declare url var url = "https://jsonplaceholder.typicode.com/users"; // Optional: Specify request options var options = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Execute a get request at the following url var response = await Utils.WebRequest.GetAsync(url); // Display the status code and response body Display($@" Status: {(int)response.Result.StatusCode} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} "); Display(""); // Declare url var url2 = $"https://jsonplaceholder.typicode.com/users"; // Optional: Specify request options var options2 = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", ContentType = Utils.WebRequest.ContentType.ApplicationJson, Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Serialize object to get the Json to create a new employee var payload = @" { ""id"": 0, ""name"": ""Kenneth Perkins"", ""username"": null, ""email"": null, ""address"": null, ""phone"": null, ""website"": null, ""company"": null } "; // Execute a post request at the following url var response2 = await Utils.WebRequest.PostAsync(url2, payload, options2); // Display the status code and response body Display($@" Status: {(int)response2.Result.StatusCode} - {response2.Result.StatusDescription} Bytes Received: {response2.Bytes.Length} Body: {response2.Body} "); Display(""); // Declare url var url3 = $"https://jsonplaceholder.typicode.com/users/1"; // Optional: Specify request options var options3 = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", ContentType = Utils.WebRequest.ContentType.ApplicationJson, Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Serialize object to get the Json to update an existing employee var payload3 = @" { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } "; // Execute a put request at the following url var response3 = await Utils.WebRequest.PutAsync(url3, payload3, options3); // Display the status code and response body Display($@" Status: {(int)response3.Result.StatusCode} - {response3.Result.StatusDescription} Bytes Received: {response3.Bytes.Length} Body: {response3.Body} "); Display(""); // Declare url var url4 = $"https://jsonplaceholder.typicode.com/users/1"; // Optional: Specify request options var options4 = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Execute a delete request at the following url var response4 = await Utils.WebRequest.DeleteAsync(url4, options4); // Display the status code and response body Display($@" Status: {(int)response4.Result.StatusCode} - {response4.Result.StatusDescription} Bytes Received: {response4.Bytes.Length} Body: {response4.Body} "); Display(""); // Declare url var url5 = $"https://jsonplaceholder.typicode.com/users/1"; // Optional: Specify request options var options5 = new Utils.WebRequest.Options() { UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:88.0) Gecko/20100101 Firefox/88.0", ContentType = Utils.WebRequest.ContentType.ApplicationJson, Headers = new System.Net.WebHeaderCollection() { {"key", "value"} } }; // Serialize object to get the Json to update an existing employee var payload5 = @" { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } "; // Execute a put request at the following url var response5 = await Utils.WebRequest.PatchAsync(url5, payload5, options5); // Display the status code and response body Display($@" Status: {(int)response5.Result.StatusCode} - {response5.Result.StatusDescription} Bytes Received: {response5.Bytes.Length} Body: {response5.Body} "); } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Check If A String Is A Valid HTTP URL Using C#
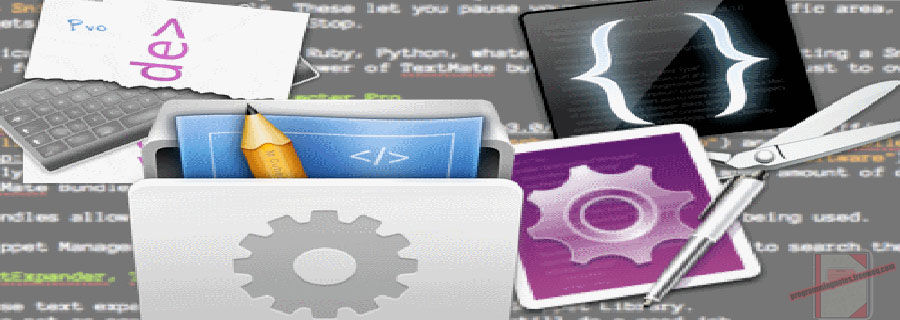
The following is a module with functions which demonstrates how to check whether a string is a valid HTTP URL using C#.
Note: The following function only checks for valid url formatting. It does not determine if the address behind the url is valid for navigation.
1. Is Valid URL
The example below demonstrates the use of ‘Utils.Http.IsValidURL‘ to check whether a string is a valid HTTP URL.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
// Is Valid URL // Declare urls var urls = new string[] { "https://www.programmingnotes.org", "http://www.programmingnotes.org", "www.programmingnotes.org", "programmingnotes.org", "javascript:alert('programmingnotes.org')", "programmingnotes", "programmingnotes.org/path?queryparam=123", "t:/www.programmingnotes.org", ":programmingnotes.org", "http/programmingnotes.org", "h91ps://programmingnotes.org/", "//programmingnotes.org/", "www@.programmingnotes.org", "protocol://programmingnotes.org/path?queryparam=123", ":programmingnotes.org/", ":programmingnotes.org:80/", "programmingnotes.org:80/", "mailto:test@email.com", "test@email.com", "file:///path/to/file/text.doc", "http://192.168.1.1/", "192.168.1.1" }; // Check if valid foreach (var url in urls) { Console.WriteLine($"URL: {url}, Is Valid: {Utils.Http.IsValidURL(url)}"); } // expected output: /* URL: https://www.programmingnotes.org, Is Valid: True URL: http://www.programmingnotes.org, Is Valid: True URL: www.programmingnotes.org, Is Valid: True URL: programmingnotes.org, Is Valid: True URL: javascript:alert('programmingnotes.org'), Is Valid: False URL: programmingnotes, Is Valid: False URL: programmingnotes.org/path?queryparam=123, Is Valid: True URL: t:/www.programmingnotes.org, Is Valid: False URL: :programmingnotes.org, Is Valid: False URL: http/programmingnotes.org, Is Valid: False URL: h91ps://programmingnotes.org/, Is Valid: False URL: //programmingnotes.org/, Is Valid: False URL: www@.programmingnotes.org, Is Valid: False URL: protocol://programmingnotes.org/path?queryparam=123, Is Valid: False URL: :programmingnotes.org/, Is Valid: False URL: :programmingnotes.org:80/, Is Valid: False URL: programmingnotes.org:80/, Is Valid: True URL: mailto:test@email.com, Is Valid: False URL: test@email.com, Is Valid: False URL: file:///path/to/file/text.doc, Is Valid: False URL: http://192.168.1.1/, Is Valid: True URL: 192.168.1.1, Is Valid: True */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 11, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Http { /// <summary> /// Determines if a url is valid or not /// </summary> /// <param name="url">The url to check</param> /// <returns>True if a url is valid, false otherwise</returns> public static bool IsValidURL(string url) { var period = url.IndexOf("."); if (period > -1 && !url.Contains("@")) { // Check if there are remnants where the url scheme should be. // Dont modify string if so var colon = url.IndexOf(":"); var slash = url.IndexOf("/"); if ((colon == -1 || period < colon) && (slash == -1 || period < slash)) { url = $"http://{url}"; } } System.Uri uriResult = null; var result = System.Uri.TryCreate(url, System.UriKind.Absolute, out uriResult) && (uriResult.Scheme == System.Uri.UriSchemeHttp || uriResult.Scheme == System.Uri.UriSchemeHttps); return result; } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 11, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; public class Program { static void Main(string[] args) { try { // Declare urls var urls = new string[] { "https://www.programmingnotes.org", "http://www.programmingnotes.org", "www.programmingnotes.org", "programmingnotes.org", "javascript:alert('programmingnotes.org')", "programmingnotes", "programmingnotes.org/path?queryparam=123", "t:/www.programmingnotes.org", ":programmingnotes.org", "http/programmingnotes.org", "h91ps://programmingnotes.org/", "//programmingnotes.org/", "www@.programmingnotes.org", "protocol://programmingnotes.org/path?queryparam=123", ":programmingnotes.org/", ":programmingnotes.org:80/", "programmingnotes.org:80/", "mailto:test@email.com", "test@email.com", "file:///path/to/file/text.doc", "http://192.168.1.1/", "192.168.1.1" }; // Check if valid foreach (var url in urls) { Display($"URL: {url}, Is Valid: {Utils.Http.IsValidURL(url)}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Get, Add, Update & Remove Values From A URL Query String Using C#
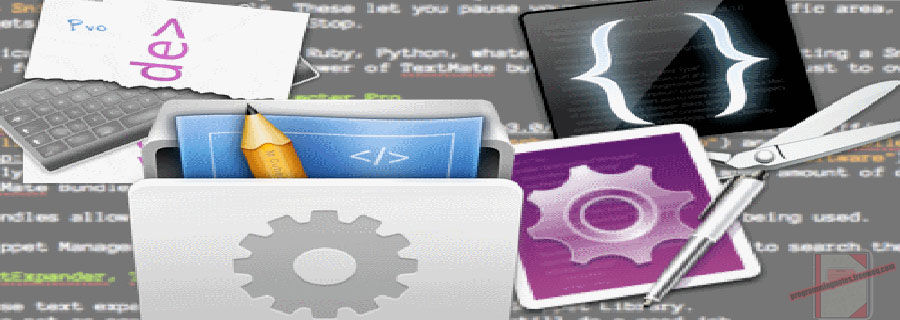
The following is a module with functions which demonstrates how to get, add, update and remove parameters from a query string using C#.
The following functions use System.Web to modify the url.
To use the functions in this module, make sure you have a reference to ‘System.Web‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add Reference‘, then type ‘System.Web‘ in the search box, and add the reference titled System.Web in the results Tab.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
1. Add – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Add‘ to add a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Add - Single Parameter // Declare url var url = "http://programmingnotes.org/"; // Add single key value parameter to the url var result = Utils.HttpParams.Add(url, new KeyValuePair<string, string>("ids", "1987")); // Display result Console.WriteLine(result); // expected output: /* http://programmingnotes.org/?ids=1987 */ |
2. Add – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Add‘ to add a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Add - Multiple Parameters // Declare url var url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Add multiple key value parameters to the url var addition = new System.Collections.Specialized.NameValueCollection() { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} }; // Update the url var result = Utils.HttpParams.Add(url, addition); // Display result Console.WriteLine(result); // expected output: /* http://programmingnotes.org/?ids=1987%2c1991%2c2010%2c2019&names=Kenneth%2cJennifer%2cLynn%2cSole */ |
3. Update – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Update‘ to update a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Update - Single Parameter // Declare url var url = "http://programmingnotes.org/?ids=1987"; // Update single key value parameter to the url var result = Utils.HttpParams.Update(url, new KeyValuePair<string, string>("ids", "1991")); // Display result Console.WriteLine(result); // expected output: /* http://programmingnotes.org/?ids=1991 */ |
4. Update – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Update‘ to update a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Update - Multiple Parameters // Declare url var url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Update multiple key value parameters to the url var addition = new System.Collections.Specialized.NameValueCollection() { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} }; // Update the url var result = Utils.HttpParams.Update(url, addition); // Display result Console.WriteLine(result); // expected output: /* http://programmingnotes.org/?ids=2010%2c2019&names=Lynn%2cSole */ |
5. Remove – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Remove‘ to remove a query string parameter from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Remove - Single Parameter // Declare url var url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Remove single parameter from the url var result = Utils.HttpParams.Remove(url, "names"); // Display result Console.WriteLine(result); // expected output: /* http://programmingnotes.org/?ids=1987%2c1991 */ |
6. Remove – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Remove‘ to remove multiple query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Remove - Multiple Parameters // Declare url var url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Remove multiple parameters from the url var result = Utils.HttpParams.Remove(url, new string[] { "ids", "names" }); // Display result Console.WriteLine(result); // expected output: /* http://programmingnotes.org/ */ |
7. Clear – Remove All Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Clear‘ to remove all query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Clear - Remove All Parameters // Declare url var url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Remove multiple parameters from the url var result = Utils.HttpParams.Clear(url); // Display result Console.WriteLine(result); // expected output: /* http://programmingnotes.org/ */ |
8. Get – Get All Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Get‘ to get all query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// Get - Get All Parameters // Declare url var url = "http://programmingnotes.org/?ids=1987,1991,2010,2019&names=Kenneth,Jennifer,Lynn,Sole"; // Get parameters from the url var result = Utils.HttpParams.Get(url); // Display result foreach (string key in result) { Console.WriteLine($"Parameter: {key}, Value: {result[key]}"); } // expected output: /* Parameter: ids, Value: 1987,1991,2010,2019 Parameter: names, Value: Kenneth,Jennifer,Lynn,Sole */ |
9. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Collections.Generic; using System.Linq; namespace Utils { public static class HttpParams { /// <summary> /// Adds the query string parameters to a url /// </summary> /// <param name="url">The url to append parameters</param> /// <param name="query">The parameters to add to the url</param> /// <returns>The url appended with the specified query</returns> public static string Add(string url, System.Collections.Specialized.NameValueCollection query) { return UpdateQuery(url, queryString => { var merged = new System.Collections.Specialized.NameValueCollection { queryString, query }; CopyProps(merged, queryString); }); } /// <summary> /// Adds the query string parameters to a url /// </summary> /// <param name="url">The url to append parameters</param> /// <param name="param">The parameters to add to the url</param> /// <returns>The url appended with the specified query</returns> public static string Add(string url, KeyValuePair<string, string> param) { return Add(url, new System.Collections.Specialized.NameValueCollection() { { param.Key, param.Value } }); } /// <summary> /// Updates the query string parameters to a url /// </summary> /// <param name="url">The url to update parameters</param> /// <param name="query">The parameters to update to the url</param> /// <returns>The url updated with the specified query</returns> public static string Update(string url, System.Collections.Specialized.NameValueCollection query) { return UpdateQuery(url, queryString => { CopyProps(query, queryString); }); } /// <summary> /// Updates the query string parameters to a url /// </summary> /// <param name="url">The url to update parameters</param> /// <param name="param">The parameters to update to the url</param> /// <returns>The url updated with the specified query</returns> public static string Update(string url, KeyValuePair<string, string> param) { return Update(url, new System.Collections.Specialized.NameValueCollection() { { param.Key, param.Value } }); } /// <summary> /// Removes the parameters with the matching keys from the query string of a url /// </summary> /// <param name="url">The url to remove parameters</param> /// <param name="keys">The parameter keys to remove from the url</param> /// <returns>The url with the parameters removed</returns> public static string Remove(string url, IEnumerable<string> keys) { return UpdateQuery(url, queryString => { foreach (var key in keys) { if (queryString.AllKeys.Contains(key)) { queryString.Remove(key); } } }); } /// <summary> /// Removes the parameter with the matching key from the query string of a url /// </summary> /// <param name="url">The url to remove a parameter</param> /// <param name="key">The parameter key to remove from the url</param> /// <returns>The url with the parameter removed</returns> public static string Remove(string url, string key) { return Remove(url, new string[]{ key }); } /// <summary> /// Removes all parameters from the query string of a url /// </summary> /// <param name="url">The url to clear parameters</param> /// <returns>The url with the parameters removed</returns> public static string Clear(string url) { return UpdateQuery(url, queryString => { queryString.Clear(); }); } /// <summary> /// Returns all parameters from the query string of a url /// </summary> /// <param name="url">The url to get parameters</param> /// <returns>The url parameters</returns> public static System.Collections.Specialized.NameValueCollection Get(string url) { System.Collections.Specialized.NameValueCollection result = null; UpdateQuery(url, queryString => { result = queryString; }); return result; } /// <summary> /// Sets the query string parameters to a url /// </summary> /// <param name="url">The url to set parameters</param> /// <param name="query">The parameters to set to the url</param> /// <returns>The url set with the specified query</returns> public static string Set(string url, System.Collections.Specialized.NameValueCollection query) { url = Clear(url); return Add(url, query); } /// <summary> /// Sets the query string parameters to a url /// </summary> /// <param name="url">The url to set parameters</param> /// <param name="param">The parameters to set to the url</param> /// <returns>The url set with the specified query</returns> public static string Set(string url, KeyValuePair<string, string> param) { return Set(url, new System.Collections.Specialized.NameValueCollection() { { param.Key, param.Value } }); } private static void CopyProps(System.Collections.Specialized.NameValueCollection source, System.Collections.Specialized.NameValueCollection destination) { foreach (string key in source) { destination[key] = source[key]; } } private static string UpdateQuery(string url, Action<System.Collections.Specialized.NameValueCollection> modifyQuery) { var uriBuilder = new System.UriBuilder(url); var query = System.Web.HttpUtility.ParseQueryString(uriBuilder.Query); modifyQuery(query); uriBuilder.Query = query.ToString(); return uriBuilder.Uri.ToString(); } } }// http://programmingnotes.org/ |
10. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { static void Main(string[] args) { try { // Declare url var url = "http://programmingnotes.org/"; // Add single key value parameter to the url var result = Utils.HttpParams.Add(url, new KeyValuePair<string, string>("ids", "1987")); // Display result Display(result); Display(""); // Declare url var url2 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Add multiple key value parameters to the url var addition = new System.Collections.Specialized.NameValueCollection() { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} }; // Update the url var result2 = Utils.HttpParams.Add(url2, addition); // Display result Display(result2); Display(""); // Declare url var url3 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Remove single parameter from the url var result3 = Utils.HttpParams.Remove(url3, "names"); // Display result Display(result3); Display(""); // Declare url var url4 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Remove multiple parameters from the url var result4 = Utils.HttpParams.Remove(url4, new string[] { "ids", "names" }); // Display result Display(result4); Display(""); // Declare url var url5 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Remove multiple parameters from the url var result5 = Utils.HttpParams.Clear(url5); // Display result Display(result5); Display(""); // Declare url var url6 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer"; // Get parameters from the url var result6 = Utils.HttpParams.Get(url6); // Display result foreach (string key in result6) { Display($"Parameter: {key}, Value: {result6[key]}"); } Display(""); // Declare url var url7 = "http://programmingnotes.org/?ids=1987"; // Update single key value parameter to the url var result7 = Utils.HttpParams.Update(url7, new KeyValuePair<string, string>("ids", "1991")); // Display result Display(result7); Display(""); // Declare url var url8 = "http://programmingnotes.org/?ids=1987&names=Kenneth"; // Update multiple key value parameters to the url var addition8 = new System.Collections.Specialized.NameValueCollection() { {"ids", "1991"}, {"ids", "2019"}, {"names", "Jennifer"}, {"names", "Sole"} }; // Update the url var result8 = Utils.HttpParams.Update(url8, addition8); // Display result Display(result8); Display(""); // Declare url var url9 = "http://programmingnotes.org/?ids=1987&names=Kenneth"; // Set multiple key value parameters to the url var addition9 = new System.Collections.Specialized.NameValueCollection() { {"ids", "1991"}, {"ids", "2010"}, {"ids", "2019"}, {"names", "Jennifer"}, {"names", "Lynn"}, {"names", "Sole"} }; // Update the url var result9 = Utils.HttpParams.Set(url9, addition9); // Display result Display(result9); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Serialize & Deserialize JSON Using C#
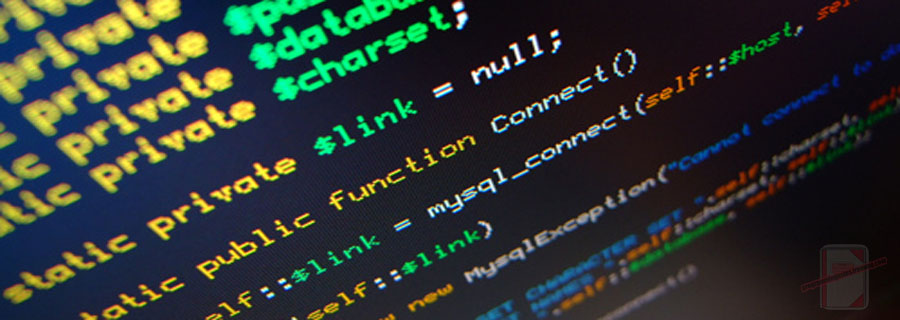
The following is a module with functions which demonstrates how to serialize and deserialize Json using C#.
The following generic functions use Newtonsoft.Json to serialize and deserialize an object.
Note: To use the functions in this module, make sure you have the ‘Newtonsoft.Json‘ package installed in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Manage NuGet Packages….‘, then type ‘Newtonsoft.Json‘ in the search box, and install the package titled Newtonsoft.Json in the results Tab.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
1. Serialize – Integer Array
The example below demonstrates the use of ‘Utils.Json.Serialize‘ to serialize an integer array to Json.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// Serialize - Integer Array // Declare array of integers var numbers = new int[] { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Optional: Create the JsonSerializerSettings with optional settings. var settings = new Newtonsoft.Json.JsonSerializerSettings() { NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore }; // Serialize to Json var numbersJson = Utils.Json.Serialize(numbers); // Display Json Console.WriteLine(numbersJson); // expected output: /* [ 1987, 19, 22, 2009, 2019, 1991, 28, 31 ] */ |
2. Serialize – String List
The example below demonstrates the use of ‘Utils.Json.Serialize‘ to serialize a list of strings to Json.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
// Serialize - String List // Declare list of strings var names = new List<string>() { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Serialize to Json var namesJson = Utils.Json.Serialize(names); // Display Json Console.WriteLine(namesJson); // expected output: /* [ "Kenneth", "Jennifer", "Lynn", "Sole" ] */ |
3. Serialize – Custom Object List
The example below demonstrates the use of ‘Utils.Json.Serialize‘ to serialize a list of custom objects to Json.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
// Serialize - Custom Object List public class Part { public string PartName { get; set; } public int PartId { get; set; } } // Declare list of objects var parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } }; // Serialize to Json var partsJson = Utils.Json.Serialize(parts); // Display Json Console.WriteLine(partsJson); // expected output: /* [ { "PartName": "crank arm", "PartId": 1234 }, { "PartName": "chain ring", "PartId": 1334 }, { "PartName": "regular seat", "PartId": 1434 }, { "PartName": "banana seat", "PartId": 1444 }, { "PartName": "cassette", "PartId": 1534 }, { "PartName": "shift lever", "PartId": 1634 } ] */ |
4. Deserialize – Integer Array
The example below demonstrates the use of ‘Utils.Json.Deserialize‘ to deserialize Json to an integer array.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// Deserialize - Integer Array // Declare Json array of integers var numbersJson = @" [ 1987, 19, 22, 2009, 2019, 1991, 28, 31 ] "; // Optional: Create the JsonSerializerSettings with optional settings. var settings = new Newtonsoft.Json.JsonSerializerSettings() { NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore }; // Deserialize from Json to specified type var numbers = Utils.Json.Deserialize<int[]>(numbersJson); // Display the items foreach (var item in numbers) { Console.WriteLine($"{item}"); } // expected output: /* 1987 19 22 2009 2019 1991 28 31 */ |
5. Deserialize – String List
The example below demonstrates the use of ‘Utils.Json.Deserialize‘ to deserialize Json to a list of strings.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Deserialize - String List // Declare Json array of strings var namesJson = @" [ ""Kenneth"", ""Jennifer"", ""Lynn"", ""Sole"" ] "; // Deserialize from Json to specified type var names = Utils.Json.Deserialize<List<string>>(namesJson); // Display the items foreach (var item in names) { Console.WriteLine($"{item}"); } // expected output: /* Kenneth Jennifer Lynn Sole */ |
6. Deserialize – Custom Object List
The example below demonstrates the use of ‘Utils.Json.Deserialize‘ to deserialize Json to a list of objects.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
// Deserialize - Custom Object List public class Part { public string PartName { get; set; } public int PartId { get; set; } } // Declare Json array of objects var partsJson = @" [ { ""PartName"": ""crank arm"", ""PartId"": 1234 }, { ""PartName"": ""chain ring"", ""PartId"": 1334 }, { ""PartName"": ""regular seat"", ""PartId"": 1434 }, { ""PartName"": ""banana seat"", ""PartId"": 1444 }, { ""PartName"": ""cassette"", ""PartId"": 1534 }, { ""PartName"": ""shift lever"", ""PartId"": 1634 } ] "; // Deserialize from Json to specified type var parts = Utils.Json.Deserialize<List<Part>>(partsJson); // Display the items foreach (var item in parts) { Console.WriteLine($"{item.PartId} - {item.PartName}"); } // expected output: /* 1234 - crank arm 1334 - chain ring 1434 - regular seat 1444 - banana seat 1534 - cassette 1634 - shift lever */ |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Json { /// <summary> /// Serializes the specified value to Json /// </summary> /// <param name="value">The value to serialize</param> /// <param name="settings">The Newtonsoft.Json.JsonSerializerSettings used to serialize the object</param> /// <returns>The value serialized to Json</returns> public static string Serialize(object value , Newtonsoft.Json.JsonSerializerSettings settings = null) { if (settings == null) { settings = GetDefaultSettings(); } return Newtonsoft.Json.JsonConvert.SerializeObject(value, settings); } /// <summary> /// Deserializes the specified value from Json to Object T /// </summary> /// <param name="value">The value to deserialize</param> /// <param name="settings">The Newtonsoft.Json.JsonSerializerSettings used to deserialize the object</param> /// <returns>The value deserialized to Object T</returns> public static T Deserialize<T>(string value , Newtonsoft.Json.JsonSerializerSettings settings = null) { if (settings == null) { settings = GetDefaultSettings(); } return Newtonsoft.Json.JsonConvert.DeserializeObject<T>(value, settings); } private static Newtonsoft.Json.JsonSerializerSettings GetDefaultSettings() { var settings = new Newtonsoft.Json.JsonSerializerSettings() { Formatting = Newtonsoft.Json.Formatting.Indented }; return settings; } } }// http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { public class Part { public string PartName { get; set; } public int PartId { get; set; } } static void Main(string[] args) { try { // Declare array of integers var numbers = new int[] { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Optional: Create the JsonSerializerSettings with optional settings. var settings = new Newtonsoft.Json.JsonSerializerSettings() { NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore }; // Serialize to Json var numbersJson = Utils.Json.Serialize(numbers); // Display Json Display(numbersJson); Display(""); // Declare list of strings var names = new List<string>() { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Serialize to Json var namesJson = Utils.Json.Serialize(names); // Display Json Display(namesJson); Display(""); // Declare list of objects var parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } }; // Serialize to Json var partsJson = Utils.Json.Serialize(parts); // Display Json Display(partsJson); Display(""); // Declare Json array of integers var numbersJsonTest = @" [ 1987, 19, 22, 2009, 2019, 1991, 28, 31 ] "; // Deserialize from Json to specified type var numbersTest = Utils.Json.Deserialize<int[]>(numbersJsonTest); // Display the items foreach (var item in numbersTest) { Display($"{item}"); } Display(""); // Declare Json array of strings var namesJsonTest = @" [ ""Kenneth"", ""Jennifer"", ""Lynn"", ""Sole"" ] "; // Deserialize from Json to specified type var namesTest = Utils.Json.Deserialize<List<string>>(namesJsonTest); // Display the items foreach (var item in namesTest) { Display($"{item}"); } Display(""); // Declare Json array of objects var partsJsonTest = @" [ { ""PartName"": ""crank arm"", ""PartId"": 1234 }, { ""PartName"": ""chain ring"", ""PartId"": 1334 }, { ""PartName"": ""regular seat"", ""PartId"": 1434 }, { ""PartName"": ""banana seat"", ""PartId"": 1444 }, { ""PartName"": ""cassette"", ""PartId"": 1534 }, { ""PartName"": ""shift lever"", ""PartId"": 1634 } ] "; // Deserialize from Json to specified type var partsTest = Utils.Json.Deserialize<List<Part>>(partsJsonTest); // Display the items foreach (var item in partsTest) { Display($"{item.PartId} - {item.PartName}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Serialize & Deserialize XML Using C#
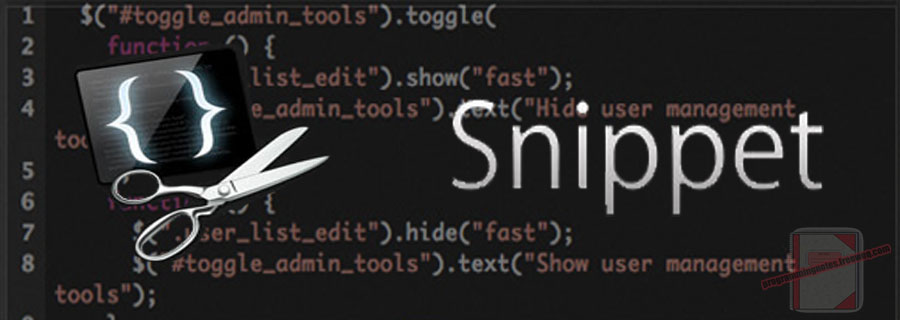
The following is a module with functions which demonstrates how to serialize and deserialize XML using C#.
The following generic functions use System.Xml.Serialization to serialize and deserialize an object.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
1. Serialize – Integer Array
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize an integer array to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// Serialize - Integer Array // Declare array of integers var numbers = new int[] { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Optional: Create an XmlRootAttribute overloaded constructor and set its namespace. var myXmlRootAttribute = new System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") { Namespace = "http://www.microsoft.com" }; // Serialize to XML var numbersXml = Utils.Xml.Serialize(numbers); // Display XML Console.WriteLine(numbersXml); // expected output: /* <?xml version="1.0"?> <ArrayOfInt xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <int>1987</int> <int>19</int> <int>22</int> <int>2009</int> <int>2019</int> <int>1991</int> <int>28</int> <int>31</int> </ArrayOfInt> */ |
2. Serialize – String List
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize a list of strings to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// Serialize - String List // Declare list of strings var names = new List<string>() { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Serialize to XML var namesXml = Utils.Xml.Serialize(names); // Display XML Console.WriteLine(namesXml); // expected output: /* <?xml version="1.0"?> <ArrayOfString xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <string>Kenneth</string> <string>Jennifer</string> <string>Lynn</string> <string>Sole</string> </ArrayOfString> */ |
3. Serialize – Custom Object List
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize a list of custom objects to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 |
// Serialize - Custom Object List [System.Xml.Serialization.XmlRoot(ElementName = "Inventory")] // Optional public class Inventory { [System.Xml.Serialization.XmlArray(ElementName = "Parts")] // Optional public List<Part> Parts { get; set; } } public class Part { [System.Xml.Serialization.XmlElement(ElementName = "PartName")] // Optional public string PartName { get; set; } [System.Xml.Serialization.XmlElement(ElementName = "PartId")] // Optional public int PartId { get; set; } } // Declare list of objects var inventory = new Inventory() { Parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } } }; // Serialize to XML var inventoryXml = Utils.Xml.Serialize(inventory); // Display XML Console.WriteLine(inventoryXml); // expected output: /* <?xml version="1.0"?> <Inventory xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <Parts> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </Parts> </Inventory> */ |
4. Deserialize – Integer Array
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to an integer array.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
// Deserialize - Integer Array // Declare Xml array of integers var numbersXml = $@"<?xml version=""1.0""?> <ArrayOfInt xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <int>1987</int> <int>19</int> <int>22</int> <int>2009</int> <int>2019</int> <int>1991</int> <int>28</int> <int>31</int> </ArrayOfInt> "; // Optional: Create an XmlRootAttribute overloaded constructor and set its namespace. var myXmlRootAttribute = new System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") { Namespace = "http://www.microsoft.com" }; // Deserialize from XML to specified type var numbers = Utils.Xml.Deserialize<int[]>(numbersXml); // Display the items foreach (var item in numbers) { Console.WriteLine($"{item}"); } // expected output: /* 1987 19 22 2009 2019 1991 28 31 */ |
5. Deserialize – String List
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to a list of strings.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Deserialize - String List // Declare Xml array of strings var namesXml = $@"<?xml version=""1.0""?> <ArrayOfString xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <string>Kenneth</string> <string>Jennifer</string> <string>Lynn</string> <string>Sole</string> </ArrayOfString> "; // Deserialize from XML to specified type var names = Utils.Xml.Deserialize<List<string>>(namesXml); // Display the items foreach (var item in names) { Console.WriteLine($"{item}"); } // expected output: /* Kenneth Jennifer Lynn Sole */ |
6. Deserialize – Custom Object List
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to a list of objects.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
// Deserialize - Custom Object List [System.Xml.Serialization.XmlRoot(ElementName = "Inventory")] // Optional public class Inventory { [System.Xml.Serialization.XmlArray(ElementName = "Parts")] // Optional public List<Part> Parts { get; set; } } public class Part { [System.Xml.Serialization.XmlElement(ElementName = "PartName")] // Optional public string PartName { get; set; } [System.Xml.Serialization.XmlElement(ElementName = "PartId")] // Optional public int PartId { get; set; } } // Declare Xml array of objects var inventoryXml = $@"<?xml version=""1.0""?> <Inventory xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance""> <Parts> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </Parts> </Inventory> "; // Deserialize from XML to specified type var inventory = Utils.Xml.Deserialize<Inventory>(inventoryXml); // Display the items foreach (var item in inventory.Parts) { Console.WriteLine($"{item.PartId} - {item.PartName}"); } // expected output: /* 1234 - crank arm 1334 - chain ring 1434 - regular seat 1444 - banana seat 1534 - cassette 1634 - shift lever */ |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; namespace Utils { public static class Xml { /// <summary> /// Serializes the specified value to XML /// </summary> /// <param name="value">The value to serialize</param> /// <param name="root">The XmlRootAttribute that represents the XML root element</param> /// <returns>The value serialized to XML</returns> public static string Serialize<T>(T value , System.Xml.Serialization.XmlRootAttribute root = null) { var xml = string.Empty; var type = value != null ? value.GetType() : typeof(T); var serializer = new System.Xml.Serialization.XmlSerializer(type: type, root: root); using (var stream = new System.IO.MemoryStream()) { serializer.Serialize(stream, value); xml = GetStreamText(stream); } return xml; } /// <summary> /// Deserializes the specified value from XML to Object T /// </summary> /// <param name="value">The value to deserialize</param> /// <param name="root">The XmlRootAttribute that represents the XML root element</param> /// <returns>The value deserialized to Object T</returns> public static T Deserialize<T>(string value , System.Xml.Serialization.XmlRootAttribute root = null) { T obj = default; var serializer = new System.Xml.Serialization.XmlSerializer(type: typeof(T), root: root); using (var stringReader = new System.IO.StringReader(value)) { using (var xmlReader = System.Xml.XmlReader.Create(stringReader)) { obj = (T)serializer.Deserialize(xmlReader); } } return obj; } public static string GetStreamText(System.IO.Stream stream) { try { TryResetPosition(stream); var stmReader = new System.IO.StreamReader(stream); var text = stmReader.ReadToEnd(); return text; } catch { throw; } finally { TryResetPosition(stream); } } private static void TryResetPosition(System.IO.Stream stream) { if (stream.CanSeek) { stream.Position = 0; } } } }// http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { [System.Xml.Serialization.XmlRoot(ElementName = "Inventory")] // Optional public class Inventory { [System.Xml.Serialization.XmlArray(ElementName = "Parts")] // Optional public List<Part> Parts { get; set; } } public class Part { [System.Xml.Serialization.XmlElement(ElementName = "PartName")] // Optional public string PartName { get; set; } [System.Xml.Serialization.XmlElement(ElementName = "PartId")] // Optional public int PartId { get; set; } } static void Main(string[] args) { try { // Declare array of integers var numbers = new int[] { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Create an XmlRootAttribute overloaded constructor and set its namespace. var myXmlRootAttribute = new System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") { Namespace = "http://www.microsoft.com" }; // Serialize to XML var numbersXml = Utils.Xml.Serialize(numbers); // Display XML Display(numbersXml); Display(""); // Declare list of strings var names = new List<string>() { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Serialize to XML var namesXml = Utils.Xml.Serialize(names); // Display XML Display(namesXml); Display(""); // Declare list of objects var inventory = new Inventory() { Parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } } }; // Serialize to XML var inventoryXml = Utils.Xml.Serialize(inventory); // Display XML Display(inventoryXml); Display(""); // Declare Xml array of integers var numbersXmlTest = $@"<?xml version=""1.0""?> <ArrayOfInt xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <int>1987</int> <int>19</int> <int>22</int> <int>2009</int> <int>2019</int> <int>1991</int> <int>28</int> <int>31</int> </ArrayOfInt> "; // Deserialize from XML to specified type var numbersTest = Utils.Xml.Deserialize<int[]>(numbersXmlTest); // Display the items foreach (var item in numbersTest) { Display($"{item}"); } Display(""); // Declare Xml array of strings var namesXmlTest = $@"<?xml version=""1.0""?> <ArrayOfString xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <string>Kenneth</string> <string>Jennifer</string> <string>Lynn</string> <string>Sole</string> </ArrayOfString> "; // Deserialize from XML to specified type var namesTest = Utils.Xml.Deserialize<List<string>>(namesXmlTest); // Display the items foreach (var item in namesTest) { Display($"{item}"); } Display(""); // Declare Xml array of objects var inventoryXmlTest = $@"<?xml version=""1.0""?> <Inventory xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance""> <Parts> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </Parts> </Inventory> "; // Deserialize from XML to specified type var inventoryTest = Utils.Xml.Deserialize<Inventory>(inventoryXmlTest); // Display the items foreach (var item in inventoryTest.Parts) { Display($"{item.PartId} - {item.PartName}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Split & Batch An Array/List/IEnumerable Into Smaller Sub-Lists Of N Size Using C#
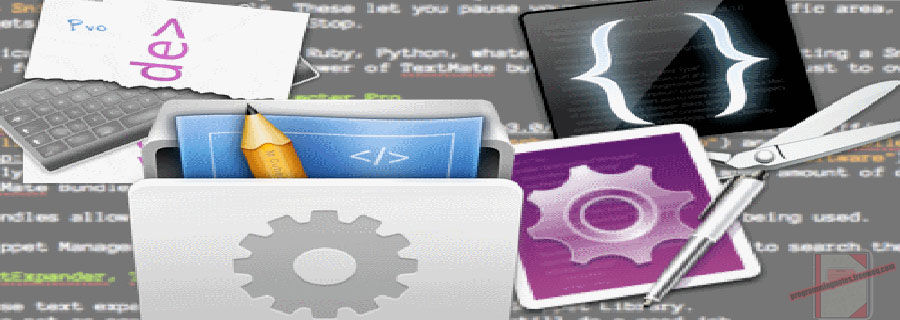
The following is a module with functions which demonstrates how to split/batch an Array/List/IEnumerable into smaller sublists of n size using C#.
This generic extension function uses a simple for loop to group items into batches.
1. Partition – Integer Array
The example below demonstrates the use of ‘Utils.Extensions.Partition‘ to group an integer array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
// Partition - Integer Array using Utils; // Declare array of integers var numbers = new int[] { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Split array into sub groups var numbersPartition = numbers.Partition(3); // Display grouped batches for (var batchCount = 0; batchCount < numbersPartition.Count; ++batchCount) { var batch = numbersPartition[batchCount]; Console.WriteLine($"Batch #{batchCount + 1}"); foreach (var item in batch) { Console.WriteLine($" Item: {item}"); } } // expected output: /* Batch #1 Item: 1987 Item: 19 Item: 22 Batch #2 Item: 2009 Item: 2019 Item: 1991 Batch #3 Item: 28 Item: 31 */ |
2. Partition – String List
The example below demonstrates the use of ‘Utils.Extensions.Partition‘ to group a list of strings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
// Partition - String List using Utils; // Declare list of strings var names = new List<string>() { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Split array into sub groups var namesPartition = names.Partition(2); // Display grouped batches for (var batchCount = 0; batchCount < namesPartition.Count; ++batchCount) { var batch = namesPartition[batchCount]; Console.WriteLine($"Batch #{batchCount + 1}"); foreach (var item in batch) { Console.WriteLine($" Item: {item}"); } } // expected output: /* Batch #1 Item: Kenneth Item: Jennifer Batch #2 Item: Lynn Item: Sole */ |
3. Partition – Custom Object List
The example below demonstrates the use of ‘Utils.Extensions.Partition‘ to group a list of custom objects.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
// Partition - Custom Object List using Utils; public class Part { public string PartName { get; set; } public int PartId { get; set; } } // Declare list of objects var parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } }; // Split array into sub groups var partsPartition = parts.Partition(4); // Display grouped batches for (var batchCount = 0; batchCount < partsPartition.Count; ++batchCount) { var batch = partsPartition[batchCount]; Console.WriteLine($"Batch #{batchCount + 1}"); foreach (var item in batch) { Console.WriteLine($" Item: {item.PartId} - {item.PartName}"); } } // expected output: /* Batch #1 Item: 1234 - crank arm Item: 1334 - chain ring Item: 1434 - regular seat Item: 1444 - banana seat Batch #2 Item: 1534 - cassette Item: 1634 - shift lever */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 9, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Collections.Generic; namespace Utils { public static class Extensions { /// <summary> /// Breaks a list into smaller sub-lists of a specified size /// </summary> /// <param name="source">An IEnumerable to split into smaller sub-lists</param> /// <param name="size">The maximum size of each sub-list</param> /// <returns>The smaller sub-lists of the specified size</returns> public static List<List<T>> Partition<T>(this IEnumerable<T> source, int size) { var result = new List<List<T>>(); List<T> batch = null; int index = 0; foreach (var item in source) { if (index % size == 0) { batch = new List<T>(); result.Add(batch); } batch.Add(item); ++index; } return result; } } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 9, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; using Utils; public class Program { public class Part { public string PartName { get; set; } public int PartId { get; set; } } static void Main(string[] args) { try { // Declare array of integers var numbers = new int[] { 1987, 19, 22, 2009, 2019, 1991, 28, 31 }; // Split array into sub groups var numbersPartition = numbers.Partition(3); // Display grouped batches for (var batchCount = 0; batchCount < numbersPartition.Count; ++batchCount) { var batch = numbersPartition[batchCount]; Display($"Batch #{batchCount + 1}"); foreach (var item in batch) { Display($" Item: {item}"); } } Display(""); // Declare list of strings var names = new List<string>() { "Kenneth", "Jennifer", "Lynn", "Sole" }; // Split array into sub groups var namesPartition = names.Partition(2); // Display grouped batches for (var batchCount = 0; batchCount < namesPartition.Count; ++batchCount) { var batch = namesPartition[batchCount]; Display($"Batch #{batchCount + 1}"); foreach (var item in batch) { Display($" Item: {item}"); } } Display(""); // Declare list of objects var parts = new List<Part>() { new Part() { PartName = "crank arm", PartId = 1234 }, new Part() { PartName = "chain ring", PartId = 1334 }, new Part() { PartName = "regular seat", PartId = 1434 }, new Part() { PartName = "banana seat", PartId = 1444 }, new Part() { PartName = "cassette", PartId = 1534 }, new Part() { PartName = "shift lever", PartId = 1634 } }; // Split array into sub groups var partsPartition = parts.Partition(4); // Display grouped batches for (var batchCount = 0; batchCount < partsPartition.Count; ++batchCount) { var batch = partsPartition[batchCount]; Display($"Batch #{batchCount + 1}"); foreach (var item in batch) { Display($" Item: {item.PartId} - {item.PartName}"); } } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.