Monthly Archives: April 2022
VB.NET || How To Add Simple Object Change Tracking To Track Changes Using VB.NET
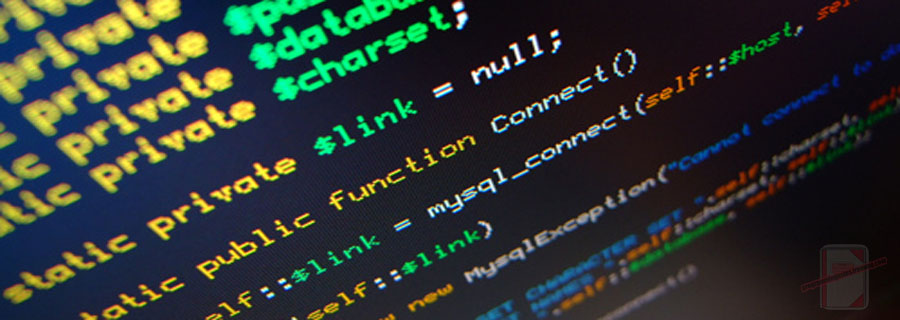
The following is a module with functions which demonstrates how to add simple object change tracking to track changes made to an object using VB.NET.
Contents
1. Overview
2. Basic Usage
3. Accept Changes
4. Reject Changes
5. Ignore Tracking For Property
6. Notify Property Changed
7. Utils Namespace
8. More Examples
1. Overview
The following is a simple abstract change tracker class which implements INotifyPropertyChanged and IRevertibleChangeTracking which provides functionality to track object changes, and to ‘accept’ or ‘revert’ changes.
To use this class, simply inherit the abstract class, and your all set!
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
2. Basic Usage
The example below demonstrates the use of ChangeTracker.BeginChanges to start tracking object changes, as well as ChangeTracker.GetChanges to get the changes made to an object.
The following example demonstrates the basic usage of adding change tracking to an object, and making and getting changes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
' Basic Usage Imports Utils ' Declare class and inherit ChangeTracker Public Class Person : Inherits ChangeTracker Public Property FirstName As String Public Property LastName As String Public Property Age As Integer? End Class ' Declare data Dim person = New Person With { .FirstName = "Kenneth", .LastName = "Perkins", .Age = 31 } ' Required: Changes made to this object will start being ' tracked the moment this function is called person.BeginChanges() ' Make changes to the object person.FirstName = "Jennifer" person.LastName = "Nguyen" person.Age = 28 ' Get changes made to the object Dim changes = person.GetChanges() ' Display the changes Debug.Print($"Changes: {changes.Count}") For Each change In changes Debug.Print($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}") Next ' expected output: ' Changes: 3 ' PropertyName: FirstName, OriginalValue: Kenneth, CurrentValue: Jennifer ' PropertyName: LastName, OriginalValue: Perkins, CurrentValue: Nguyen ' PropertyName: Age, OriginalValue: 31, CurrentValue: 28 |
3. Accept Changes
The example below demonstrates the use of ChangeTracker.AcceptChanges to accept modification changes made to an object.
This function commits all the changes made to the object since either ChangeTracker.BeginChanges was called, or since ChangeTracker.AcceptChanges was last called.
When the accept function is called, all the changes made to the object up to that point will be marked as the current ‘source of truth’ for change tracking.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
' Accept Changes Imports Utils ' Declare class and inherit ChangeTracker Public Class Person : Inherits ChangeTracker Public Property FirstName As String Public Property LastName As String Public Property Age As Integer? End Class ' Declare data Dim person = New Person With { .FirstName = "Kenneth", .LastName = "Perkins", .Age = 31 } ' Required: Changes made to this object will start being ' tracked the moment this function is called person.BeginChanges() ' Make changes to the object person.FirstName = "Lynn" person.LastName = "P" person.Age = 10 ' Accept all the changes made to the object up to ' this point as the source of truth for change tracking person.AcceptChanges() ' Get changes made to the object Dim changes = person.GetChanges() ' Display the changes ' Note: No changes will display because the most recent modifications has been accepted Debug.Print($"Changes: {changes.Count}") For Each change In changes Debug.Print($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}") Next ' expected output: ' Changes: 0 |
4. Reject Changes
The example below demonstrates the use of ChangeTracker.RejectChanges to reject modification changes made to an object.
This function rejects all the changes made to the object since either ChangeTracker.BeginChanges was called, or since ChangeTracker.AcceptChanges was last called.
When the reject function is called, all the changes made to the object up to that point reverts back to the objects state before modifications initially began or modifications was last accepted.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
' Reject Changes Imports Utils ' Declare class and inherit ChangeTracker Public Class Person : Inherits ChangeTracker Public Property FirstName As String Public Property LastName As String Public Property Age As Integer? End Class ' Declare data Dim person = New Person With { .FirstName = "Kenneth", .LastName = "Perkins", .Age = 31 } ' Required: Changes made to this object will start being ' tracked the moment this function is called person.BeginChanges() ' Make changes to the object person.FirstName = "Sole" person.LastName = "P" person.Age = 19 ' Rejects all the changes made to the object up to ' this point and reverts back to the objects state ' before modifications were made person.RejectChanges() ' Get changes made to the object Dim changes = person.GetChanges() ' Display the changes ' Note: No changes will display because the most recent modifications has been rejected Debug.Print($"Changes: {changes.Count}") For Each change In changes Debug.Print($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}") Next ' expected output: ' Changes: 0 |
5. Ignore Tracking For Property
The example below demonstrates the use of ChangeTracker.ChangeTrackerIgnore attribute to mark a specific property to be ignored from change tracking.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
' Ignore Tracking For Property Imports Utils ' Declare class and inherit ChangeTracker Public Class Person : Inherits ChangeTracker Public Property FirstName As String Public Property LastName As String <ChangeTrackerIgnore> Public Property Age As Integer? End Class ' Declare data Dim person = New Person With { .FirstName = "Kenneth", .LastName = "Perkins", .Age = 31 } ' Required: Changes made to this object will start being ' tracked the moment this function is called person.BeginChanges() ' Make changes to the object person.FirstName = "Jennifer" person.LastName = "Nguyen" person.Age = 28 ' Get changes made to the object Dim changes = person.GetChanges() ' Display the changes Debug.Print($"Changes: {changes.Count}") For Each change In changes Debug.Print($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}") Next ' expected output: ' Changes: 2 ' PropertyName: FirstName, OriginalValue: Kenneth, CurrentValue: Jennifer ' PropertyName: LastName, OriginalValue: Perkins, CurrentValue: Nguyen |
6. Notify Property Changed
The example below demonstrates the use of ChangeTracker.NotifyPropertyChanged to fire the PropertyChanged event notifying that the specified property value has changed.
In the class declaration, simply add ChangeTracker.NotifyPropertyChanged to the properties you wish to notify changes, and add a PropertyChanged event handler function to receive the notifications.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
' Notify Property Changed Imports Utils ' Declare class and inherit ChangeTracker ' Modify the properties you wish to notify changes Public Class Person : Inherits ChangeTracker Private _firstName As String Public Property FirstName As String Get Return _firstName End Get Set(value As String) _firstName = value NotifyPropertyChanged(value) End Set End Property Private _lastName As String Public Property LastName As String Get Return _lastName End Get Set(value As String) _lastName = value NotifyPropertyChanged(value) End Set End Property Private _age As Integer? Public Property Age As Integer? Get Return _age End Get Set(value As Integer?) _age = value NotifyPropertyChanged(value) End Set End Property End Class ' Declare data Dim person = New Person With { .FirstName = "Kenneth", .LastName = "Perkins", .Age = 31 } ' Set custom event handler function for property changed event AddHandler person.PropertyChanged, AddressOf Person_PropertyChanged ' Required: Changes made to this object will start being ' tracked the moment this function is called person.BeginChanges() ' Make changes to the object person.FirstName = "Jennifer" person.LastName = "Nguyen" person.Age = 28 ' Declare Custom event handler function for the PropertyChanged event Public Sub Person_PropertyChanged(sender As Object, e As System.ComponentModel.PropertyChangedEventArgs) Dim eventArgs = CType(e, ChangeTracker.PropertyChangedTrackedEventArgs) Debug.Print($"Event Handler Changes:") Debug.Print($" PropertyName: {eventArgs.PropertyName}, OriginalValue: {eventArgs.OriginalValue}, CurrentValue: {eventArgs.CurrentValue}") End Sub ' expected output: ' Event Handler Changes: ' PropertyName: FirstName, OriginalValue: Kenneth, CurrentValue: Jennifer ' Event Handler Changes: ' PropertyName: LastName, OriginalValue: Perkins, CurrentValue: Nguyen ' Event Handler Changes: ' PropertyName: Age, OriginalValue: 31, CurrentValue: 28 |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Apr 23, 2022 ' Taken From: http://programmingnotes.org/ ' File: Utils.cs ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Imports System Imports System.Linq Imports System.Reflection Imports System.Collections.Generic Imports System.Runtime.CompilerServices Imports System.ComponentModel Namespace Global.Utils Public MustInherit Class ChangeTracker : Implements System.ComponentModel.INotifyPropertyChanged, System.ComponentModel.IRevertibleChangeTracking Protected Property Tracked As Dictionary(Of String, ITrackedChange) Public Event PropertyChanged As System.ComponentModel.PropertyChangedEventHandler Implements INotifyPropertyChanged.PropertyChanged Public ReadOnly Property IsTracking As Boolean Get Return Tracked.Count > 0 End Get End Property ''' <summary> ''' Gets the objects changed status ''' </summary> ''' <returns><see langword="true"/> if the objects content has changed since either <see cref="BeginChanges"/> ''' was called, or since <see cref="AcceptChanges"/> was last called; <see langword="false"/> otherwise</returns> Public Property IsChanged As Boolean Implements IRevertibleChangeTracking.IsChanged Get If Not _isChanged AndAlso IsTracking Then Dim trackedChanges = GetTrackedChanges() If trackedChanges.Any(Function(x) x.DetectChange(GetValue(x.Property))) Then _isChanged = True End If End If Return _isChanged End Get Protected Set(value As Boolean) _isChanged = value End Set End Property Private _isChanged As Boolean = False Protected Sub New() Tracked = New Dictionary(Of String, ITrackedChange)() End Sub ''' <summary> ''' Fires the PropertyChanged event notifying that the specified property value changed ''' </summary> ''' <param name="currentValue">The current value</param> ''' <param name="originalValue">The original value</param> ''' <param name="propertyName">The corresponding property name</param> Protected Sub NotifyPropertyChanged(currentValue As Object, originalValue As Object, <CallerMemberName> Optional propertyName As String = "") RaiseEvent PropertyChanged(Me, New PropertyChangedTrackedEventArgs(propertyName, originalValue, currentValue)) End Sub ''' <summary> ''' Fires the PropertyChanged event notifying that the specified property value changed ''' </summary> ''' <param name="currentValue">The current value</param> ''' <param name="propertyName">The corresponding property name</param> Protected Overridable Sub NotifyPropertyChanged(currentValue As Object, <CallerMemberName> Optional propertyName As String = "") NotifyPropertyChanged(currentValue, GetTrackedChange(propertyName)?.OriginalValue, propertyName) End Sub ''' <summary> ''' Starts the tracking operation on the object. Changes made to the ''' object will start being tracked the moment this function is called ''' </summary> ''' <exception cref="InvalidOperationException">Changes have already begun</exception> Public Overridable Sub BeginChanges() ThrowIfTrackingStarted() Track(GetTrackableProperties()) IsChanged = False End Sub ''' <summary> ''' Ends the tracking operation on the object. Any pending tracked changes made to the ''' object since either <see cref="BeginChanges"/> was called, or since <see cref="AcceptChanges"/> ''' was last called will be lost the moment this function is called ''' </summary> ''' <exception cref="InvalidOperationException">Change tracking has not started</exception> Public Overridable Sub EndChanges() ThrowIfTrackingNotStarted() Tracked.Clear() IsChanged = False End Sub ''' <summary> ''' Sets the current object state as its default state by accepting the modifications. ''' Commits all the changes made to this object since either <see cref="BeginChanges"/> ''' was called, or since <see cref="AcceptChanges"/> was last called ''' </summary> ''' <exception cref="InvalidOperationException">Change tracking has not started</exception> Public Overridable Sub AcceptChanges() Implements IChangeTracking.AcceptChanges ThrowIfTrackingNotStarted() Dim trackedChanges = UpdateTracked() For Each change In trackedChanges change.AcceptChange() Next IsChanged = False End Sub ''' <summary> ''' Resets the current objects state by rejecting the modifications. Rejects ''' all changes made to the object since either <see cref="BeginChanges"/> ''' was called, or since <see cref="AcceptChanges"/> was last called ''' </summary> ''' <exception cref="InvalidOperationException">Change tracking has not started</exception> Public Overridable Sub RejectChanges() Implements IRevertibleChangeTracking.RejectChanges ThrowIfTrackingNotStarted() Dim trackedChanges = GetTrackedChanges() For Each change In trackedChanges SetValue(change.[Property], change.OriginalValue) change.RejectChange() Next IsChanged = False End Sub ''' <summary> ''' Returns a list containing information of all the properties with changes ''' applied to it since either <see cref="BeginChanges"/> was called, or ''' since <see cref="AcceptChanges"/> was last called ''' </summary> ''' <returns>A list containing the changes made to the object</returns> ''' <exception cref="InvalidOperationException">Change tracking has not started</exception> Public Overridable Function GetChanges() As List(Of ITrackedChange) ThrowIfTrackingNotStarted() Dim result = New List(Of ITrackedChange)() Dim trackedChanges = UpdateTracked() For Each change In trackedChanges If change.HasChanges Then result.Add(change) End If Next Return result End Function ''' <summary> ''' Returns a list containing information of all the properties being tracked ''' </summary> ''' <returns>A list containing property tracking information</returns> ''' <exception cref="InvalidOperationException">Change tracking has not started</exception> Public Function GetTracked() As List(Of ITrackedChange) ThrowIfTrackingNotStarted() Return Tracked.Values.ToList() End Function ''' <summary> ''' Updates the tracking status of all the properties being tracked ''' </summary> ''' <returns>A list containing properties being tracked</returns> Protected Function UpdateTracked() As IEnumerable(Of TrackedChange) Dim trackedChanges = GetTrackedChanges() Track(trackedChanges.Select(Function(x) x.Property)) Return trackedChanges End Function ''' <summary> ''' Keeps track of the original and current values of the provided properties ''' </summary> ''' <param name="properties">The properties to track</param> Protected Sub Track(properties As IEnumerable(Of PropertyInfo)) If properties IsNot Nothing Then For Each prop In properties Track(prop, GetValue(prop)) Next End If End Sub ''' <summary> ''' Keeps track of the original and current value of the provided property ''' </summary> ''' <param name="property">The property to track</param> ''' <param name="currentValue">The current value of the property</param> Protected Sub Track([property] As PropertyInfo, currentValue As Object) Dim currentChange As TrackedChange = Nothing If Not IsTrackedChange([property].Name) Then Dim hasChangedFunc As Func(Of Object, Object, Boolean) = Function(original, current) Return HasValuesChanged(original, current) End Function currentChange = New TrackedChange With { .Property = [property], .OriginalValue = currentValue, .HasChangedFunc = hasChangedFunc } Tracked.Add([property].Name, currentChange) Else currentChange = GetTrackedChange([property].Name) currentChange.CurrentValue = currentValue currentChange.Status = TrackedChange.TrackingStatus.Checked currentChange.LastChecked = Date.Now End If If currentChange.HasChanges Then IsChanged = True End If End Sub ''' <summary> ''' Class which contains property tracking information ''' </summary> Protected Class TrackedChange : Implements ITrackedChange Public Enum TrackingStatus Unchecked Checked End Enum Public Property [Property] As PropertyInfo Public ReadOnly Property PropertyName As String Implements ITrackedChange.PropertyName Get Return [Property]?.Name End Get End Property Public Property OriginalValue As Object Implements ITrackedChange.OriginalValue Public Property CurrentValue As Object Implements ITrackedChange.CurrentValue Public Property Status As TrackingStatus = TrackingStatus.Unchecked Public ReadOnly Property HasChanges As Boolean Get Return Status = TrackingStatus.Checked AndAlso DetectChange(CurrentValue) End Get End Property Public Property LastChecked As Date? = Nothing Public Property HasChangedFunc As Func(Of Object, Object, Boolean) Public Function DetectChange(currentValue As Object) As Boolean Return HasChangedFunc(OriginalValue, currentValue) End Function Public Sub AcceptChange() OriginalValue = CurrentValue RejectChange() End Sub Public Sub RejectChange() CurrentValue = Nothing Status = TrackingStatus.Unchecked LastChecked = Nothing End Sub End Class ''' <summary> ''' Provides support for object change tracking ''' </summary> Interface ITrackedChange ReadOnly Property PropertyName As String ReadOnly Property OriginalValue As Object ReadOnly Property CurrentValue As Object End Interface ''' <summary> ''' Provides data for the <see cref=" System.ComponentModel.PropertyChangedEventArgs"/> event ''' </summary> Public Class PropertyChangedTrackedEventArgs : Inherits System.ComponentModel.PropertyChangedEventArgs Public Property OriginalValue As Object Public Property CurrentValue As Object Public Sub New(propertyName As String, originalValue As Object, currentValue As Object) MyBase.New(propertyName) Me.OriginalValue = originalValue Me.CurrentValue = currentValue End Sub End Class ''' <summary> ''' Attribute which allows a property to be ignored from being tracked ''' </summary> Public Class ChangeTrackerIgnoreAttribute : Inherits System.Attribute End Class #Region "Helpers" Protected Function IsTrackedChange(propertyName As String) As Boolean Return Tracked.ContainsKey(propertyName) End Function Protected Function GetTrackedChange(propertyName As String) As TrackedChange Return If(IsTrackedChange(propertyName), CType(Tracked(propertyName), TrackedChange), Nothing) End Function Protected Function GetTrackedChanges() As IEnumerable(Of TrackedChange) Return Tracked.Select(Function(pair) CType(pair.Value, TrackedChange)) End Function Protected Overridable Function GetTrackableProperties() As IEnumerable(Of PropertyInfo) Return Me.GetType().GetProperties() _ .Where(Function(p) Return Not p.DeclaringType.Equals(GetType(ChangeTracker)) _ AndAlso p.CanRead _ AndAlso p.CanWrite _ AndAlso Not p.GetCustomAttributes(Of ChangeTrackerIgnoreAttribute)(False).Any() End Function) End Function Protected Overridable Function GetValue([property] As PropertyInfo) As Object Return [property]?.GetValue(Me, If([property].GetIndexParameters().Count() = 1, New Object() {Nothing}, Nothing)) End Function Protected Overridable Sub SetValue([property] As PropertyInfo, value As Object) If [property] IsNot Nothing AndAlso [property].CanWrite Then [property].SetValue(Me, value) End If End Sub Protected Overridable Function HasValuesChanged(Of T)(originalValue As T, currentValue As T) As Boolean Return Not EqualityComparer(Of T).Default.Equals(originalValue, currentValue) End Function Protected Sub ThrowIfTrackingNotStarted() If Not IsTracking Then Throw New InvalidOperationException("Change tracking has not started. Call 'BeginChanges' to start change tracking") End If End Sub Protected Sub ThrowIfTrackingStarted() If IsTracking Then Throw New InvalidOperationException("Change tracking has already started") End If End Sub #End Region End Class End Namespace ' http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Apr 23, 2022 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Imports Utils Public Module Program ' Declare class and inherit ChangeTracker ' Modify the properties you wish to notify changes Public Class Person : Inherits ChangeTracker Private _firstName As String Public Property FirstName As String Get Return _firstName End Get Set(value As String) _firstName = value NotifyPropertyChanged(value) End Set End Property Private _lastName As String Public Property LastName As String Get Return _lastName End Get Set(value As String) _lastName = value NotifyPropertyChanged(value) End Set End Property Private _age As Integer? '<ChangeTrackerIgnore> Public Property Age As Integer? Get Return _age End Get Set(value As Integer?) _age = value NotifyPropertyChanged(value) End Set End Property End Class Sub Main(args As String()) Try ' Declare data Dim person = New Person With { .FirstName = "Kenneth", .LastName = "Perkins", .Age = 31 } ' Set custom event handler function for property changed event AddHandler person.PropertyChanged, AddressOf Person_PropertyChanged ' Required: Changes made to this object will start being ' tracked the moment this function is called person.BeginChanges() Dim changes1 = person.GetChanges() ' Make changes to the object person.FirstName = "Jennifer" person.LastName = "Nguyen" person.Age = 28 ' Get changes made to the object Dim changes2 = person.GetChanges() ' Display the changes DisplayChanges(changes2) ' --- Accept changes --- ' Make changes to the object person.FirstName = "Lynn" person.LastName = "P" person.Age = 10 Dim changes3 = person.GetChanges() ' Accept all the changes made to the object up to ' this point as the source of truth for change tracking person.AcceptChanges() 'Get changes made to the object Dim changes4 = person.GetChanges() ' Display the changes ' Note: No changes will display because the most recent modifications has been accepted DisplayChanges(changes4) ' --- Reject changes --- ' Make changes to the object person.FirstName = "Sole" person.LastName = "P" person.Age = 19 ' Rejects all the changes made to the object up to ' this point and reverts back to the objects state ' before modifications were made person.RejectChanges() ' Get changes made to the object Dim changes5 = person.GetChanges() ' Display the changes ' Note: No changes will display because the most recent modifications has been rejected DisplayChanges(changes5) Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub ' Declare Custom event handler function for the PropertyChanged event Public Sub Person_PropertyChanged(sender As Object, e As System.ComponentModel.PropertyChangedEventArgs) Dim eventArgs = CType(e, ChangeTracker.PropertyChangedTrackedEventArgs) Display($"Event Handler Changes:") Display($" PropertyName: {eventArgs.PropertyName}, OriginalValue: {eventArgs.OriginalValue}, CurrentValue: {eventArgs.CurrentValue}") End Sub Public Sub DisplayChanges(changes As List(Of ChangeTracker.ITrackedChange)) Display($"Changes: {changes.Count}") For Each change In changes Display($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}") Next Display("============") End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || How To Add Simple Object Change Tracking To Track Changes Using C#
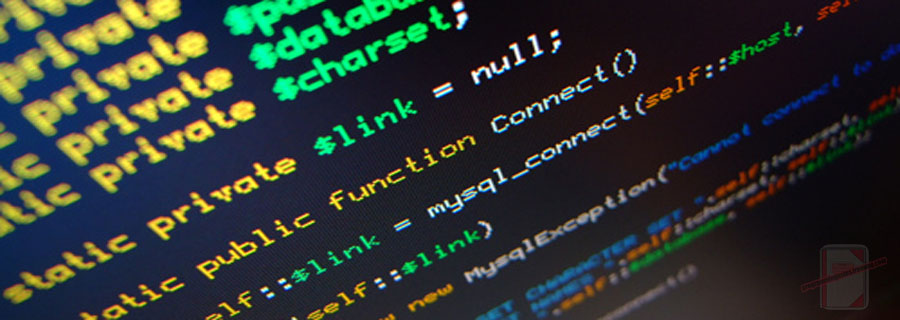
The following is a module with functions which demonstrates how to add simple object change tracking to track changes made to an object using C#.
Contents
1. Overview
2. Basic Usage
3. Accept Changes
4. Reject Changes
5. Ignore Tracking For Property
6. Notify Property Changed
7. Utils Namespace
8. More Examples
1. Overview
The following is a simple abstract change tracker class which implements INotifyPropertyChanged and IRevertibleChangeTracking which provides functionality to track object changes, and to ‘accept’ or ‘revert’ changes.
To use this class, simply inherit the abstract class, and your all set!
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
2. Basic Usage
The example below demonstrates the use of ChangeTracker.BeginChanges to start tracking object changes, as well as ChangeTracker.GetChanges to get the changes made to an object.
The following example demonstrates the basic usage of adding change tracking to an object, and making and getting changes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// Basic Usage using Utils; // Declare class and inherit ChangeTracker public class Person : ChangeTracker { public string FirstName { get; set; } public string LastName { get; set; } public int? Age { get; set; } } // Declare data var person = new Person { FirstName = "Kenneth", LastName = "Perkins", Age = 31 }; // Required: Changes made to this object will start being // tracked the moment this function is called person.BeginChanges(); // Make changes to the object person.FirstName = "Jennifer"; person.LastName = "Nguyen"; person.Age = 28; // Get changes made to the object var changes = person.GetChanges(); // Display the changes Console.WriteLine($"Changes: {changes.Count}"); foreach (var change in changes) { Console.WriteLine($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}"); } // expected output: /* Changes: 3 PropertyName: FirstName, OriginalValue: Kenneth, CurrentValue: Jennifer PropertyName: LastName, OriginalValue: Perkins, CurrentValue: Nguyen PropertyName: Age, OriginalValue: 31, CurrentValue: 28 */ |
3. Accept Changes
The example below demonstrates the use of ChangeTracker.AcceptChanges to accept modification changes made to an object.
This function commits all the changes made to the object since either ChangeTracker.BeginChanges was called, or since ChangeTracker.AcceptChanges was last called.
When the accept function is called, all the changes made to the object up to that point will be marked as the current ‘source of truth’ for change tracking.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
// Accept Changes using Utils; // Declare class and inherit ChangeTracker public class Person : ChangeTracker { public string FirstName { get; set; } public string LastName { get; set; } public int? Age { get; set; } } // Declare data var person = new Person { FirstName = "Kenneth", LastName = "Perkins", Age = 31 }; // Required: Changes made to this object will start being // tracked the moment this function is called person.BeginChanges(); // Make changes to the object person.FirstName = "Lynn"; person.LastName = "P"; person.Age = 10; // Accept all the changes made to the object up to // this point as the source of truth for change tracking person.AcceptChanges(); // Get changes made to the object var changes = person.GetChanges(); // Display the changes // Note: No changes will display because the most recent modifications has been accepted Console.WriteLine($"Changes: {changes.Count}"); foreach (var change in changes) { Console.WriteLine($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}"); } // expected output: /* Changes: 0 */ |
4. Reject Changes
The example below demonstrates the use of ChangeTracker.RejectChanges to reject modification changes made to an object.
This function rejects all the changes made to the object since either ChangeTracker.BeginChanges was called, or since ChangeTracker.AcceptChanges was last called.
When the reject function is called, all the changes made to the object up to that point reverts back to the objects state before modifications initially began or modifications was last accepted.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
// Reject Changes using Utils; // Declare class and inherit ChangeTracker public class Person : ChangeTracker { public string FirstName { get; set; } public string LastName { get; set; } public int? Age { get; set; } } // Declare data var person = new Person { FirstName = "Kenneth", LastName = "Perkins", Age = 31 }; // Required: Changes made to this object will start being // tracked the moment this function is called person.BeginChanges(); // Make changes to the object person.FirstName = "Sole"; person.LastName = "P"; person.Age = 19; // Rejects all the changes made to the object up to // this point and reverts back to the objects state // before modifications were made person.RejectChanges(); // Get changes made to the object var changes = person.GetChanges(); // Display the changes // Note: No changes will display because the most recent modifications has been rejected Console.WriteLine($"Changes: {changes.Count}"); foreach (var change in changes) { Console.WriteLine($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}"); } // expected output: /* Changes: 0 */ |
5. Ignore Tracking For Property
The example below demonstrates the use of ChangeTracker.ChangeTrackerIgnore attribute to mark a specific property to be ignored from change tracking.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// Ignore Tracking For Property using Utils; // Declare class and inherit ChangeTracker public class Person : ChangeTracker { public string FirstName { get; set; } public string LastName { get; set; } [ChangeTrackerIgnore] public int? Age { get; set; } } // Declare data var person = new Person { FirstName = "Kenneth", LastName = "Perkins", Age = 31 }; // Required: Changes made to this object will start being // tracked the moment this function is called person.BeginChanges(); // Make changes to the object person.FirstName = "Jennifer"; person.LastName = "Nguyen"; person.Age = 28; // Get changes made to the object var changes = person.GetChanges(); // Display the changes Console.WriteLine($"Changes: {changes.Count}"); foreach (var change in changes) { Console.WriteLine($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}"); } // expected output: /* Changes: 2 PropertyName: FirstName, OriginalValue: Kenneth, CurrentValue: Jennifer PropertyName: LastName, OriginalValue: Perkins, CurrentValue: Nguyen */ |
6. Notify Property Changed
The example below demonstrates the use of ChangeTracker.NotifyPropertyChanged to fire the PropertyChanged event notifying that the specified property value has changed.
In the class declaration, simply add ChangeTracker.NotifyPropertyChanged to the properties you wish to notify changes, and add a PropertyChanged event handler function to receive the notifications.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
// Notify Property Changed using Utils; // Declare class and inherit ChangeTracker // Modify the properties you wish to notify changes public class Person : ChangeTracker { string _firstName; public string FirstName { get { return _firstName; } set { _firstName = value; NotifyPropertyChanged(value); } } string _lastName; public string LastName { get { return _lastName; } set { _lastName = value; NotifyPropertyChanged(value); } } int? _age; public int? Age { get { return _age; } set { _age = value; NotifyPropertyChanged(value); } } } // Declare data var person = new Person { FirstName = "Kenneth", LastName = "Perkins", Age = 31 }; // Set custom event handler function for property changed event person.PropertyChanged += Person_PropertyChanged; // Required: Changes made to this object will start being // tracked the moment this function is called person.BeginChanges(); // Make changes to the object person.FirstName = "Jennifer"; person.LastName = "Nguyen"; person.Age = 28; // Declare Custom event handler function for the PropertyChanged event public static void Person_PropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e) { var eventArgs = (ChangeTracker.PropertyChangedTrackedEventArgs)e; Console.WriteLine($"Event Handler Changes:"); Console.WriteLine($" PropertyName: {eventArgs.PropertyName}, OriginalValue: {eventArgs.OriginalValue}, CurrentValue: {eventArgs.CurrentValue}"); } // expected output: /* Event Handler Changes: PropertyName: FirstName, OriginalValue: Kenneth, CurrentValue: Jennifer Event Handler Changes: PropertyName: LastName, OriginalValue: Perkins, CurrentValue: Nguyen Event Handler Changes: PropertyName: Age, OriginalValue: 31, CurrentValue: 28 */ |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 |
// ============================================================================ // Author: Kenneth Perkins // Date: Apr 10, 2022 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; using System.Reflection; using System.Collections.Generic; using System.Runtime.CompilerServices; namespace Utils { public abstract class ChangeTracker : System.ComponentModel.INotifyPropertyChanged, System.ComponentModel.IRevertibleChangeTracking { protected Dictionary<string, ITrackedChange> Tracked { get; set; } public event System.ComponentModel.PropertyChangedEventHandler PropertyChanged; public bool IsTracking { get { return Tracked.Count > 0; } } /// <summary> /// Gets the objects changed status /// </summary> /// <returns><see langword="true"/> if the objects content has changed since either <see cref="BeginChanges"/> /// was called, or since <see cref="AcceptChanges"/> was last called; <see langword="false"/> otherwise</returns> public bool IsChanged { get { if (!_isChanged && IsTracking) { var trackedChanges = GetTrackedChanges(); if (trackedChanges.Any(x => x.DetectChange(GetValue(x.Property)))) { _isChanged = true; } } return _isChanged; } protected set { _isChanged = value; } } private bool _isChanged = false; protected ChangeTracker() { Tracked = new Dictionary<string, ITrackedChange>(); } /// <summary> /// Fires the PropertyChanged event notifying that the specified property value changed /// </summary> /// <param name="currentValue">The current value</param> /// <param name="originalValue">The original value</param> /// <param name="propertyName">The corresponding property name</param> protected void NotifyPropertyChanged(object currentValue, object originalValue, [CallerMemberName] string propertyName = "") { this.PropertyChanged?.Invoke(this, new PropertyChangedTrackedEventArgs(propertyName, originalValue, currentValue)); } /// <summary> /// Fires the PropertyChanged event notifying that the specified property value changed /// </summary> /// <param name="currentValue">The current value</param> /// <param name="propertyName">The corresponding property name</param> protected virtual void NotifyPropertyChanged(object currentValue, [CallerMemberName] string propertyName = "") { NotifyPropertyChanged(currentValue, GetTrackedChange(propertyName)?.OriginalValue, propertyName); } /// <summary> /// Starts the tracking operation on the object. Changes made to the /// object will start being tracked the moment this function is called /// </summary> /// <exception cref="InvalidOperationException">Changes have already begun</exception> public virtual void BeginChanges() { ThrowIfTrackingStarted(); Track(GetTrackableProperties()); IsChanged = false; } /// <summary> /// Ends the tracking operation on the object. Any pending tracked changes made to the /// object since either <see cref="BeginChanges"/> was called, or since <see cref="AcceptChanges"/> /// was last called will be lost the moment this function is called /// </summary> /// <exception cref="InvalidOperationException">Change tracking has not started</exception> public virtual void EndChanges() { ThrowIfTrackingNotStarted(); Tracked.Clear(); IsChanged = false; } /// <summary> /// Sets the current object state as its default state by accepting the modifications. /// Commits all the changes made to this object since either <see cref="BeginChanges"/> /// was called, or since <see cref="AcceptChanges"/> was last called /// </summary> /// <exception cref="InvalidOperationException">Change tracking has not started</exception> public virtual void AcceptChanges() { ThrowIfTrackingNotStarted(); var trackedChanges = UpdateTracked(); foreach (var change in trackedChanges) { change.AcceptChange(); } IsChanged = false; } /// <summary> /// Resets the current objects state by rejecting the modifications. Rejects /// all changes made to the object since either <see cref="BeginChanges"/> /// was called, or since <see cref="AcceptChanges"/> was last called /// </summary> /// <exception cref="InvalidOperationException">Change tracking has not started</exception> public virtual void RejectChanges() { ThrowIfTrackingNotStarted(); var trackedChanges = GetTrackedChanges(); foreach (var change in trackedChanges) { SetValue(change.Property, change.OriginalValue); change.RejectChange(); } IsChanged = false; } /// <summary> /// Returns a list containing information of all the properties with changes /// applied to it since either <see cref="BeginChanges"/> was called, or /// since <see cref="AcceptChanges"/> was last called /// </summary> /// <returns>A list containing the changes made to the object</returns> /// <exception cref="InvalidOperationException">Change tracking has not started</exception> public virtual List<ITrackedChange> GetChanges() { ThrowIfTrackingNotStarted(); var result = new List<ITrackedChange>(); var trackedChanges = UpdateTracked(); foreach (var change in trackedChanges) { if (change.HasChanges) { result.Add(change); } } return result; } /// <summary> /// Returns a list containing information of all the properties being tracked /// </summary> /// <returns>A list containing property tracking information</returns> /// <exception cref="InvalidOperationException">Change tracking has not started</exception> public List<ITrackedChange> GetTracked() { ThrowIfTrackingNotStarted(); return Tracked.Values.ToList(); } /// <summary> /// Updates the tracking status of all the properties being tracked /// </summary> /// <returns>A list containing properties being tracked</returns> protected IEnumerable<TrackedChange> UpdateTracked() { var trackedChanges = GetTrackedChanges(); Track(trackedChanges.Select(x => x.Property)); return trackedChanges; } /// <summary> /// Keeps track of the original and current values of the provided properties /// </summary> /// <param name="properties">The properties to track</param> protected void Track(IEnumerable<PropertyInfo> properties) { if (properties != null) { foreach (var prop in properties) { Track(prop, GetValue(prop)); } } } /// <summary> /// Keeps track of the original and current value of the provided property /// </summary> /// <param name="property">The property to track</param> /// <param name="currentValue">The current value of the property</param> protected void Track(PropertyInfo property, object currentValue) { TrackedChange currentChange = null; if (!IsTrackedChange(property.Name)) { Func<object, object, bool> hasChangedFunc = (original, current) => { return HasValuesChanged(original, current); }; currentChange = new TrackedChange { Property = property, OriginalValue = currentValue, HasChangedFunc = hasChangedFunc }; Tracked.Add(property.Name, currentChange); } else { currentChange = GetTrackedChange(property.Name); currentChange.CurrentValue = currentValue; currentChange.Status = TrackedChange.TrackingStatus.Checked; currentChange.LastChecked = DateTime.Now; } if (currentChange.HasChanges) { IsChanged = true; } } /// <summary> /// Class which contains property tracking information /// </summary> protected class TrackedChange : ITrackedChange { public enum TrackingStatus { Unchecked, Checked } public PropertyInfo Property { get; set; } public string PropertyName { get { return Property?.Name; } } public object OriginalValue { get; set; } public object CurrentValue { get; set; } public TrackingStatus Status { get; set; } = TrackingStatus.Unchecked; public bool HasChanges { get { return Status == TrackingStatus.Checked && DetectChange(CurrentValue); } } public DateTime? LastChecked { get; set; } = null; public Func<object, object, bool> HasChangedFunc { get; set; } public bool DetectChange(object currentValue) { return HasChangedFunc(OriginalValue, currentValue); } public void AcceptChange() { OriginalValue = CurrentValue; RejectChange(); } public void RejectChange() { CurrentValue = null; Status = TrackingStatus.Unchecked; LastChecked = null; } } /// <summary> /// Provides support for object change tracking /// </summary> public interface ITrackedChange { string PropertyName { get; } object OriginalValue { get; } object CurrentValue { get; } } /// <summary> /// Provides data for the <see cref=" System.ComponentModel.PropertyChangedEventArgs"/> event /// </summary> public class PropertyChangedTrackedEventArgs : System.ComponentModel.PropertyChangedEventArgs { public object OriginalValue{ get; private set; } public object CurrentValue { get; private set; } public PropertyChangedTrackedEventArgs(string propertyName, object originalValue, object currentValue) : base(propertyName) { OriginalValue = originalValue; CurrentValue = currentValue; } } /// <summary> /// Attribute which allows a property to be ignored from being tracked /// </summary> public class ChangeTrackerIgnoreAttribute : System.Attribute {} #region "Helpers" protected bool IsTrackedChange(string propertyName) { return Tracked.ContainsKey(propertyName); } protected TrackedChange GetTrackedChange(string propertyName) { return IsTrackedChange(propertyName) ? (TrackedChange)Tracked[propertyName] : null; } protected IEnumerable<TrackedChange> GetTrackedChanges() { return Tracked.Select(pair => (TrackedChange)pair.Value); } protected virtual IEnumerable<PropertyInfo> GetTrackableProperties() { return this.GetType().GetProperties() .Where(p => !p.DeclaringType.Equals(typeof(ChangeTracker)) && p.CanRead && p.CanWrite && !p.GetCustomAttributes<ChangeTrackerIgnoreAttribute>(false).Any() ); } protected virtual object GetValue(PropertyInfo property) { return property?.GetValue(this, property.GetIndexParameters() .Count() == 1 ? new object[] { null } : null); } protected virtual void SetValue(PropertyInfo property, object value) { if (property != null && property.CanWrite) { property.SetValue(this, value); } } protected virtual bool HasValuesChanged<T>(T originalValue, T currentValue) { return !EqualityComparer<T>.Default.Equals(originalValue, currentValue); } protected void ThrowIfTrackingNotStarted() { if (!IsTracking) { throw new InvalidOperationException("Change tracking has not started. Call 'BeginChanges' to start change tracking"); } } protected void ThrowIfTrackingStarted() { if (IsTracking) { throw new InvalidOperationException("Change tracking has already started"); } } #endregion } }// http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 |
// ============================================================================ // Author: Kenneth Perkins // Date: Apr 10, 2022 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; using System.Threading.Tasks; using Utils; public class Program { // Declare class and inherit ChangeTracker // Modify the properties you wish to notify changes public class Person : ChangeTracker { string _firstName; public string FirstName { get { return _firstName; } set { _firstName = value; NotifyPropertyChanged(value); } } string _lastName; public string LastName { get { return _lastName; } set { _lastName = value; NotifyPropertyChanged(value); } } int? _age; //[ChangeTrackerIgnore] public int? Age { get { return _age; } set { _age = value; NotifyPropertyChanged(value); } } } static void Main(string[] args) { try { // Declare data var person = new Person { FirstName = "Kenneth", LastName = "Perkins", Age = 31 }; // Set custom event handler function for property changed event person.PropertyChanged += Person_PropertyChanged; // Required: Changes made to this object will start being // tracked the moment this function is called person.BeginChanges(); var changes1 = person.GetChanges(); // Make changes to the object person.FirstName = "Jennifer"; person.LastName = "Nguyen"; person.Age = 28; // Get changes made to the object var changes2 = person.GetChanges(); // Display the changes DisplayChanges(changes2); // --- Accept changes --- // Make changes to the object person.FirstName = "Lynn"; person.LastName = "P"; person.Age = 10; var changes3 = person.GetChanges(); // Accept all the changes made to the object up to // this point as the source of truth for change tracking person.AcceptChanges(); // Get changes made to the object var changes4 = person.GetChanges(); // Display the changes // Note: No changes will display because the most recent modifications has been accepted DisplayChanges(changes4); // --- Reject changes --- // Make changes to the object person.FirstName = "Sole"; person.LastName = "P"; person.Age = 19; // Rejects all the changes made to the object up to // this point and reverts back to the objects state // before modifications were made person.RejectChanges(); // Get changes made to the object var changes5 = person.GetChanges(); // Display the changes // Note: No changes will display because the most recent modifications has been rejected DisplayChanges(changes5); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } // Declare Custom event handler function for the PropertyChanged event public static void Person_PropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e) { var eventArgs = (ChangeTracker.PropertyChangedTrackedEventArgs)e; Display($"Event Handler Changes:"); Display($" PropertyName: {eventArgs.PropertyName}, OriginalValue: {eventArgs.OriginalValue}, CurrentValue: {eventArgs.CurrentValue}"); } public static void DisplayChanges(List<ChangeTracker.ITrackedChange> changes) { Display($"Changes: {changes.Count}"); foreach (var change in changes) { Display($" PropertyName: {change.PropertyName}, OriginalValue: {change.OriginalValue}, CurrentValue: {change.CurrentValue}"); } Display("============"); } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C# || Remove Linked List Elements – How To Remove All Target Linked List Elements Using C#
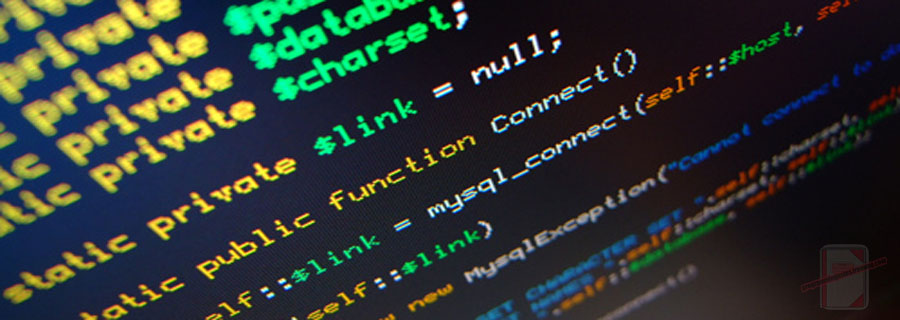
The following is a module with functions which demonstrates how to remove all target linked list elements using C#.
1. Remove Elements – Problem Statement
Given the head of a linked list and an integer val, remove all the nodes of the linked list that has Node.val == val, and return the new head.
Example 1:
Input: head = [1,2,6,3,4,5,6], val = 6
Output: [1,2,3,4,5]
Example 2:
Input: head = [], val = 1
Output: []
Example 3:
Input: head = [7,7,7,7], val = 7
Output: []
2. Remove Elements – Solution
The following is a solution which demonstrates how to remove all target linked list elements.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// ============================================================================ // Author: Kenneth Perkins // Date: Apr 2, 2022 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to remove all target linked list elements // ============================================================================ /** * Definition for singly-linked list. * public class ListNode { * public int val; * public ListNode next; * public ListNode(int val=0, ListNode next=null) { * this.val = val; * this.next = next; * } * } */ public class Solution { public ListNode RemoveElements(ListNode head, int val) { var ptr = head; // Loop through data checking to see if the next node equals the target value while (ptr != null && ptr.next != null) { // If the next node value is the target value, set the next node // to point to the one after it if (ptr.next.val == val) { ptr.next = ptr.next.next; } else { // Advance to the next node ptr = ptr.next; } } // If the head equals the target value, advance to the next if (head != null && head.val == val) { head = head.next; } return head; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[1,2,3,4,5]
[]
[]