Monthly Archives: August 2024
C# || How To Design Seat Reservation Manager Using C#
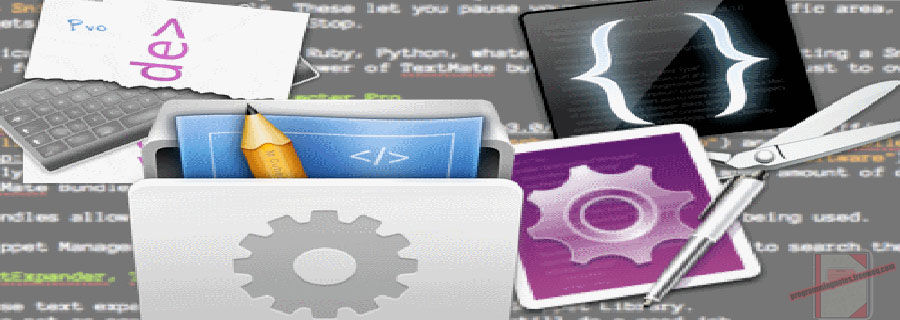
The following is a module with functions which demonstrates how to design a seat reservation manager using C#.
1. Seat Manager – Problem Statement
Design a system that manages the reservation state of n seats that are numbered from 1 to n.
Implement the SeatManager class:
- SeatManager(int n) Initializes a SeatManager object that will manage n seats numbered from 1 to n. All seats are initially available.
- int reserve() Fetches the smallest-numbered unreserved seat, reserves it, and returns its number.
- void unreserve(int seatNumber) Unreserves the seat with the given seatNumber.
Example 1:
Input
["SeatManager", "reserve", "reserve", "unreserve", "reserve", "reserve", "reserve", "reserve", "unreserve"]
[[5], [], [], [2], [], [], [], [], [5]]
Output
[null, 1, 2, null, 2, 3, 4, 5, null]Explanation
SeatManager seatManager = new SeatManager(5); // Initializes a SeatManager with 5 seats.
seatManager.reserve(); // All seats are available, so return the lowest numbered seat, which is 1.
seatManager.reserve(); // The available seats are [2,3,4,5], so return the lowest of them, which is 2.
seatManager.unreserve(2); // Unreserve seat 2, so now the available seats are [2,3,4,5].
seatManager.reserve(); // The available seats are [2,3,4,5], so return the lowest of them, which is 2.
seatManager.reserve(); // The available seats are [3,4,5], so return the lowest of them, which is 3.
seatManager.reserve(); // The available seats are [4,5], so return the lowest of them, which is 4.
seatManager.reserve(); // The only available seat is seat 5, so return 5.
seatManager.unreserve(5); // Unreserve seat 5, so now the available seats are [5].
2. Seat Manager – Solution
The following is a solution which demonstrates how to design a seat reservation manager.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// ============================================================================ // Author: Kenneth Perkins // Date: Aug 1, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to design a seat reservation manager // ============================================================================ /** * Your SeatManager object will be instantiated and called as such: * SeatManager obj = new SeatManager(n); * int param_1 = obj.Reserve(); * obj.Unreserve(seatNumber); */ public class SeatManager { // Marker to point to unreserved seats. int marker; // Sorted set to store all unreserved seats. SortedSet<int> availableSeats; public SeatManager(int n) { // Set marker to the first unreserved seat. marker = 1; // Initialize the sorted set. availableSeats = new SortedSet<int>(); } public int Reserve() { // If the sorted set has any element in it, then, // get the smallest-numbered unreserved seat from it. if (availableSeats.Count > 0) { int firstSeatNumber = availableSeats.First(); availableSeats.Remove(firstSeatNumber); return firstSeatNumber; } // Otherwise, the marker points to the smallest-numbered seat. int seatNumber = marker; marker++; return seatNumber; } public void Unreserve(int seatNumber) { // Push the unreserved seat in the sorted set. availableSeats.Add(seatNumber); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
[null,1,2,null,2,3,4,5,null]