Monthly Archives: November 2024
C# || How To Convert Integer To English Words Using C#
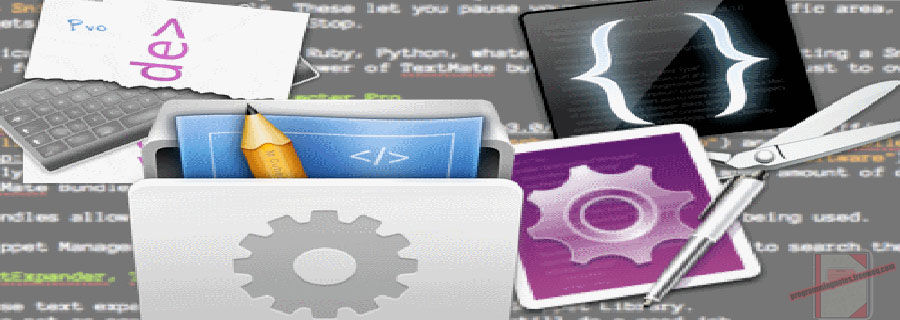
The following is a module with functions which demonstrates how to convert integer to english words using C#.
1. Integer to English Words – Problem Statement
Convert a non-negative integer num to its English words representation.
Example 1:
Input: num = 123
Output: "One Hundred Twenty Three"
Example 2:
Input: num = 12345
Output: "Twelve Thousand Three Hundred Forty Five"
Example 3:
Input: num = 1234567
Output: "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven"
2. Integer to English Words – Solution
The following is a solution which demonstrates how to convert integer to english words.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
// ============================================================================ // Author: Kenneth Perkins // Date: Nov 1, 2024 // Taken From: http://programmingnotes.org/ // File: Solution.cs // Description: Demonstrates how to convert integer to english word // ============================================================================ public class NumberWord { public int Value { get; } public string Word { get; } public NumberWord(int value, string word) { Value = value; Word = word; } } public class Solution { // List to store words for numbers private static readonly List<NumberWord> numberToWordsList = new List<NumberWord> { new NumberWord(1000000000, "Billion"), new NumberWord(1000000, "Million"), new NumberWord(1000, "Thousand"), new NumberWord(100, "Hundred"), new NumberWord(90, "Ninety"), new NumberWord(80, "Eighty"), new NumberWord(70, "Seventy"), new NumberWord(60, "Sixty"), new NumberWord(50, "Fifty"), new NumberWord(40, "Forty"), new NumberWord(30, "Thirty"), new NumberWord(20, "Twenty"), new NumberWord(19, "Nineteen"), new NumberWord(18, "Eighteen"), new NumberWord(17, "Seventeen"), new NumberWord(16, "Sixteen"), new NumberWord(15, "Fifteen"), new NumberWord(14, "Fourteen"), new NumberWord(13, "Thirteen"), new NumberWord(12, "Twelve"), new NumberWord(11, "Eleven"), new NumberWord(10, "Ten"), new NumberWord(9, "Nine"), new NumberWord(8, "Eight"), new NumberWord(7, "Seven"), new NumberWord(6, "Six"), new NumberWord(5, "Five"), new NumberWord(4, "Four"), new NumberWord(3, "Three"), new NumberWord(2, "Two"), new NumberWord(1, "One") }; public string NumberToWords(int num) { if (num == 0) { return "Zero"; } foreach (var nw in numberToWordsList) { // Check if the number is greater than or equal to the current unit if (num >= nw.Value) { // Convert the quotient to words if the current unit is 100 or greater string prefix = (num >= 100) ? NumberToWords(num / nw.Value) + " " : ""; // Get the word for the current unit string unit = nw.Word; // Convert the remainder to words if it's not zero string suffix = (num % nw.Value == 0) ? "" : " " + NumberToWords(num % nw.Value); return prefix + unit + suffix; } } return ""; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output for the example cases:
"One Hundred Twenty Three"
"Twelve Thousand Three Hundred Forty Five"
"One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven"