Tag Archives: array
C++ || Struct – Add One Day To Today’s Date Using A Struct
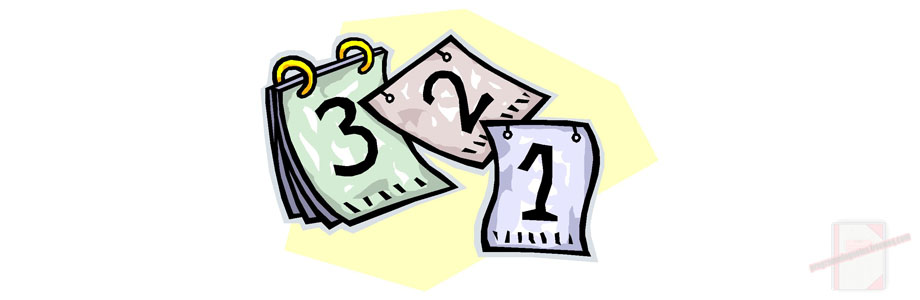
This program displays more practice using the structure data type, and is very similar to another program which was previously discussed here.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Functions
Passing a Value By Reference
Integer Arrays
Structures
Constant Variables
Boolean Expressions
This program utilizes a struct, which is very similar to the class concept. This program first prompts the user to enter the current date in mm/dd/yyyy format. Upon obtaining the date from the user, the program then uses a struct implementation to simply add one day to the date which was entered by the user. If the day that was entered into the program by the user falls on the end of the month, the program will”roll over” the incremented date into the next month. If the user enters 12/31/2012, the program will “roll over” the incremented date into the next calendar year.
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 |
#include <iostream> using namespace std; // struct declaration struct Date { int month; int day; int year; }; // constants which do not change // specifies the max values for each variable const int MAX_DAYS = 31; const int MAX_MONTHS = 12; // function prototypes bool IsDateValid(Date dateVal); void CalcNextDay(Date &dateVal); int main() { // initialize the structure: month, day, year Date dateVal = {1,18,2012}; char backSlash = '/'; // this is a 'placeholder' variable // obtain information from user cout << "Please enter today's date in mm/dd/yyyy format: "; cin >> dateVal.month >> backSlash >> dateVal.day >> backSlash >> dateVal.year; // Checks to see if user entered valid data if (IsDateValid(dateVal) == false) { cout << "Invalid input...nProgram exiting...." << endl; exit(EXIT_FAILURE); } // function declaration which calculates the next day CalcNextDay(dateVal); // display updated data to user cout << "The next day is " << dateVal.month << "/" << dateVal.day << "/" << dateVal.year <<endl; return 0; }// end of main // this function checks to see if the user inputted valid data bool IsDateValid(Date dateVal) { // checks to see if user inputted data falls between the specified // const variables as declared above if ((dateVal.day >= 1 && dateVal.day <= MAX_DAYS) &&(dateVal.month >= 1 && dateVal.month <= MAX_MONTHS)) { return true; } else { return false; } }// end of IsDateValid // this calculates the next day & updates values via reference void CalcNextDay(Date &dateVal) { // int array which holds the max date each month has // from January to December int maxDays[12]={31,28,31,30,31,30,31,31,30,31,30,31}; // bool which lets the program know if the month the // user entered is a max month for the specific month // the user inputted bool maxDay = false; // checks to see if user inputted day is a max date from the // above array for the currently selected month which // was entered by the user if(dateVal.day == maxDays[dateVal.month-1]) { dateVal.day = 1; ++dateVal.month; maxDay = true; } // checks to see if user inputted a valid date // for the currently selected month else if (dateVal.day > maxDays[dateVal.month-1]) { cout << "Invalid day input - There is no such date for the selected month.nProgram exiting...." << endl; exit(EXIT_FAILURE); } // if user didnt enter a max date, and the date is valid, increment the day else { ++dateVal.day; } // if the date is 12/31/yyyy // increment to the next year if ((dateVal.month > 12) && maxDay == true) { dateVal.month = 1; ++dateVal.year; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled 6 separate times to display the different outputs its able to produce
Please enter today's date in mm/dd/yyyy format: 1/18/2012
The next day is 1/19/2012
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 7/31/2012
The next day is 8/1/2012
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 2/28/2012
The next day is 3/1/2012
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 13/5/2012
Invalid input...
Program exiting....
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 2/31/2012
Invalid day input - There is no such date for the selected month.
Program exiting....
-------------------------------------------------------------------Please enter today's date in mm/dd/yyyy format: 12/31/2012
The next day is 1/1/2013
C++ || Searching An Integer Array For A Target Value
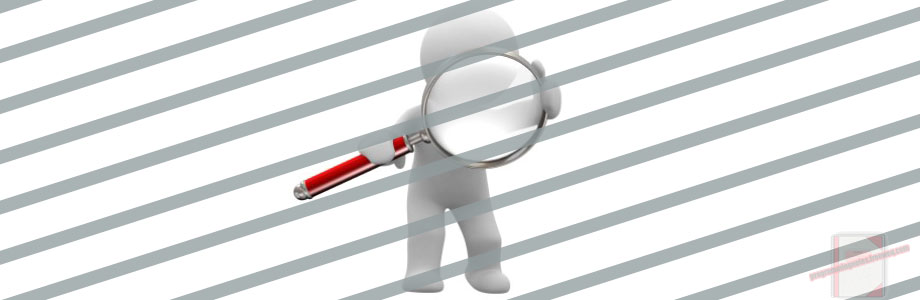
Here is another actual homework assignment which was presented in an intro to programming class.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
This is a small and simple program which demonstrates how to search for a target value that is stored in an integer array. This program prompts the user to enter five values into an int array. After the user has entered all the values, it displays a prompt asking the user for a search value. Once it has the search value, the program will search through the array looking for the target; wherever the value is found, it will display the index location. After it displays all the locations where the value is found, it will display the total number of occurrences the search value was found within the array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
// ============================================================================ // File: ArraySearch.cpp // ============================================================================ // // Description: // This program allows the user to populate an array of integers with five // values. After the user has entered all the values, it displays a prompt // asking the user for a search value. Once it has the search value, the // program will search through the array looking for the target; wherever the // value is found, it will display the index location. After it displays all // the locations where the value is found, it will display the total number // of occurrences the search value was found within the array. // // ============================================================================ #include <iostream> using namespace std; // constant variable const int NUM_INTS = 5; // function prototypes int SearchArray(int numValues[], int searchValue); // ==== main ================================================================== // // ============================================================================ int main() { // declare variables int searchValue; int numOccurences = 0; int numValues[NUM_INTS]; // int array size is initialized with a const value // get data from user via a 'for loop' cout << "Please enter "<< NUM_INTS <<" integer values:nn"; for (int index=0; index < NUM_INTS; ++index) { cout << "#" << index + 1 << ": "; cin >> numValues[index]; cout <<endl; } // get a search value from the user cout << "Please enter a search value: "; cin >> searchValue; cout <<endl; // finds the number of occurences the search value was found in the array numOccurences = SearchArray(numValues, searchValue); // display data to user cout << "nThe total occurrences of value " << searchValue << " within the array is: " << numOccurences; cout << endl << endl; return 0; }// end of main // ==== SearchArray =========================================================== // // This function will take as input the array, the number of array // elements, and the target value to search for. The function will traverse // the array looking for the target value, and when it finds it, display the // index location within the array. // // Input: // limit [IN] -- the array, the number of array // elements, and the target value // // Output: // The total number of occurrences of the target in the array // // ============================================================================ int SearchArray(int numValues[], int searchValue) { int numFound=0; for (int index=0; index < NUM_INTS; ++index) { if (numValues[index] == searchValue) { cout << "t" << searchValue << " was found at array index #" << index << endl; ++numFound; // if the search values was found, // increment the variable by 1 } } return numFound; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled 3 separate times to display the different outputs its able to produce
Please enter 5 integer values:
#1: 12
#2: 12
#3: 12
#4: 12
#5: 12
Please enter a search value: 1212 was found at array index #0
12 was found at array index #1
12 was found at array index #2
12 was found at array index #3
12 was found at array index #4The total occurrences of value 12 within the array is: 5
-------------------------------------------------------------------------Please enter 5 integer values:
#1: 12
#2: 87
#3: 45
#4: 87
#5: 33
Please enter a search value: 8787 was found at array index #1
87 was found at array index #3The total occurrences of value 87 within the array is: 2
-------------------------------------------------------------------------Please enter 5 interger values:
#1: 54
#2: 67
#3: 98
#4: 45
#5: 98
Please enter a search value: 123The total occurrences of value 123 within the array is: 0
C++ || Find The Prime, Perfect & All Known Divisors Of A Number Using A For, While & Do/While Loop
This program was designed to better understand how to use different loops which are available in C++.
This program first asks the user to enter a non negative number. After it obtains a non negative integer from the user, the program will determine if the inputted number is a prime number or not, aswell as determine if the user inputted number is a perfect number or not. After it obtains its results, the program will display to the screen if the user inputted number is prime/perfect number or not. The program will also display a list of all the possible divisors of the user inputted number via cout.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Do/While Loop
While Loop
For Loop
Modulus
Basic Math - Prime Numbers
Basic Math - Perfect Numbers
Basic Math - Divisors
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 |
#include <iostream> #include <iomanip> using namespace std; int main () { int userInput = 0; int divisor = 0; int sumOfDivisors = 0; char response ='n'; do{ // this is the start of the do/while loop cout<<"Enter a number: "; cin >> userInput; // if the user inputs a negative number, do this code while(userInput < 0) { cout<<"ntaSorry, but the number entered is less than the allowable limit. Please try again"; cout<<"nnEnter an number: "; cin >> userInput; } cout << "nInput number: " << userInput; // for loop adds sum of all possible divisors for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0) { // this will repeatedly add the found divisors together sumOfDivisors += counter; } } // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is prime if(userInput == (sumOfDivisors - 1)) { cout<<endl; cout << userInput << " is a prime number."; } else { cout<<endl; cout << userInput << " is not a prime number."; } // uses the 'sumOfDivisors' variable from ^ above for loop to // check if 'userInput' is a perfect number if (userInput == (sumOfDivisors - userInput)) { cout<<endl; cout << userInput << " is a perfect number."; } else { cout<<endl; cout << userInput << " is not a perfect number."; } cout << "nDivisors of " << userInput << " are: "; // for loop lists all the possible divisors for the // 'userInput' variable by using the modulus operator for(int counter=1; counter <= userInput; ++counter) { divisor = (userInput % counter); if(divisor == 0 && counter !=userInput) { cout << counter << ", "; } } cout << "and "<< userInput; // asks user if they want to enter new data cout << "nntDo you want to input another number?(Y/N): "; cin >> response; // creates a line seperator if user wants to enter new data cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // resets variable back to zero if the user wants to enter new data sumOfDivisors=0; }while(response =='y' || response =='Y'); // ^ End of the do/while loop. As long as the user chooses // 'Y' the loop will keep going. // It stops when the user chooses the letter 'N' return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Enter a number: 8128
Input number: 8128
8128 is not a prime number.
8128 is a perfect number.
Divisors of 8128 are: 1, 2, 4, 8, 16, 32, 64, 127, 254, 508, 1016, 2032, 4064, and 8128Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 6Input number: 6
6 is not a prime number.
6 is a perfect number.
Divisors of 6 are: 1, 2, 3, and 6Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 241Input number: 241
241 is a prime number.
241 is not a perfect number.
Divisors of 241 are: 1, and 241Do you want to input another number?(Y/N): y
------------------------------------------------------------
Enter a number: 2012Input number: 2012
2012 is not a prime number.
2012 is not a perfect number.
Divisors of 2012 are: 1, 2, 4, 503, 1006, and 2012Do you want to input another number?(Y/N): n
------------------------------------------------------------
Press any key to continue . . .
C++ || Whats My Name? – Using a Char Array, Strcpy, Strcat, Strcmp, & Strlen
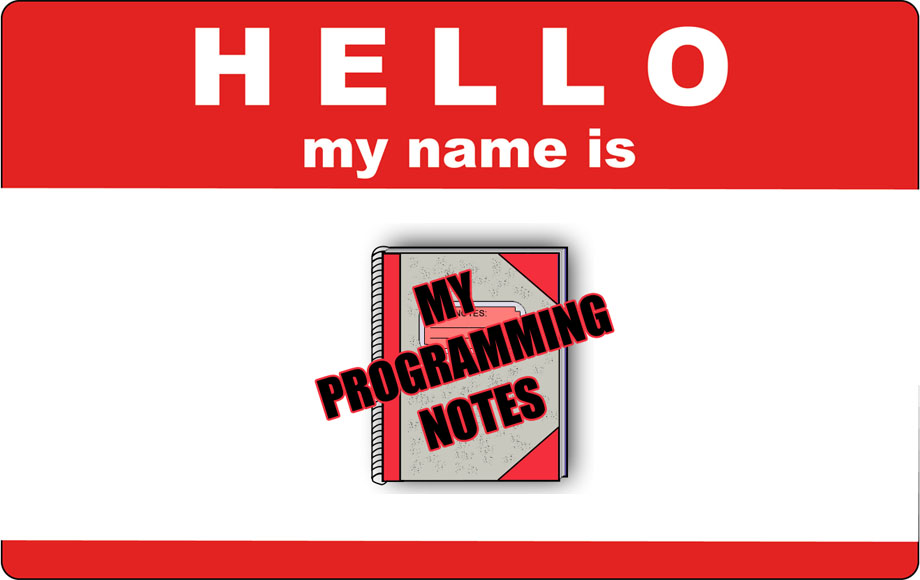
Here is another actual homework assignment which was presented in an intro to programming class.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
This program first prompts the user to enter their name. Upon receiving that information, the program saves input into a character array of size 15. The program then asks if the user has a middle name. If they do, the user will enter a middle name. If they dont, the program proceeds to ask for a last name. Upon receiving the first, [middle], and last names, the program will use strcpy and strcat to copy/append the first, [middle], and last names into a completely new char array titled “fullname.” Lastly, if the users’ first, [middle], or last names are the same, the program will display that data to the screen via cout. The program will also display to the user the number of characters their full name contains using strlen.
NOTE: On some compilers, you may have to add #include < cstring> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 |
// ============================================================================ // File: NameString.cpp // ============================================================================ // // Description: // This program prompts the user for a first name, middle name and last name. // Each name string is to be saved in its own character array. Then a // "full name" string is created by copying the first name to a fourth array, // and concatenating the middle and last names. Once the full name is // in its own buffer, the total number of characters in the full name will // be displayed. // // ============================================================================ #include <iostream> using namespace std; //function prototypes void FirstMiddleLast(char firstname[]); void FirstLast(char firstname[]); // ==== main ================================================================== // // ============================================================================ int main() { char firstname[15]; // char array with ability to hold 15 characters char response = ' '; // get data from user. Notice, you dont need a loop to input data // into the char array cout << "Please enter your first name: "; cin >> firstname; // check to see if user has a middle name cout << "nDo you have a middle name?(Y/N): "; cin >> response; // you could also use a switch statement here if ((response=='y')||(response=='Y')) { FirstMiddleLast(firstname); // function declaration } else if ((response=='n')||(response=='N')) { FirstLast(firstname); // function declaration } else { cout << "nPlease press either 'Y' or 'N'nna" << "Program exiting...n"; } return 0; }// end of main // ==== FirstMiddleLast ======================================================= // // This function will take as input the first name, and prompt the user for // their middle and last name. Then it will display the total number of // characters in their name // // Input: // limit [IN] -- The users first name // // Output: // The users full name, and the total number of characters in their name // // ============================================================================ void FirstMiddleLast(char firstname[]) { char middlename[15]; char lastname[15]; char fullname[30]; cout << "nPlease enter your middle name: "; cin >> middlename; cout <<"nPlease enter your last name: "; cin >> lastname; strcpy(fullname, firstname); // copies data from 'firstname' into 'fullname' array strcat(fullname, " "); // appends 'fullname' with a space strcat(fullname, middlename); // appends 'fullname' to contain the data from 'middlename' array strcat(fullname, " "); // appends 'fullname' with a space strcat(fullname, lastname); // appends 'fullname' to contain the data from 'lastname' array cout <<"ntYour full name is " << fullname << endl; cout << "tThe total number of characters in your name is: "; cout << strlen(fullname)-2 << endl; // gets total num of chars contained in the 'fullname' array, minus the 2 spaces // if strcmp returns 0, the two strings are the same if(strcmp(firstname, middlename) == 0) { cout << "tYour first and middle name are the samen"; } if(strcmp(middlename, lastname) == 0) { cout << "tYour middle and last name are the samen"; } if(strcmp(firstname, lastname) == 0) { cout << "tYour first and last name are the samen"; } }// end of FirstMiddleLast // ==== FirstLast ============================================================= // // This function will take as input the first name, and prompt the user for // their last name. Then it will display the total number of characters in // their name // // Input: // limit [IN] -- The users first name // // Output: // The users full name, and the total number of characters in their name // // ============================================================================ void FirstLast(char firstname[]) { char lastname[15]; char fullname[30]; cout <<"nPlease enter your last name: "; cin >> lastname; strcpy(fullname, firstname); // copies data from 'firstname' into 'fullname' array strcat(fullname, " "); // appends 'fullname' with a space strcat(fullname, lastname); // appends 'fullname' to contain the data from 'lastname' array cout <<"ntYour full name is " << fullname << endl; cout << "tThe total number of characters in your name is: "; cout << strlen(fullname)-1 << endl; // gets total num of chars contained in the 'fullname' array, minus the single space // if strcmp returns 0, the two strings are the same if(strcmp(firstname, lastname) == 0) { cout << "tYour first and last name are the samenn"; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
CHAR ARRAY
Unlike integer arrays, a loop is not necessary in order to input data into a char array. This is highlighted on lines 32, 76, 79, and 125.
FUNCTIONS
Notice lines 18, 19, 69 and 119. When dealing with arrays, in order to pass variables to different functions, you need to include brackets[] letting the compiler know that you are passing an array variable. If you do not declare the functions as so, you will get a compiler error.
STRCPY/STRCAT
These functions are highlighted on lines 81-85, and 127-129. Read the comments within the code on those selected lines, they are helpful.
STRCMP
This compares two strings together to determine if they are the same. This is displayed on lines 92, 96, 100, and 136 when comparing the first, [middle], and last names together for sameness.
STRLEN
This finds the length of the current string, as noted on lines 89 and 133.
Once compiled, you should get this as your output:
Note: The code was compiled five separate times to display the different outputs its able to produce
Please enter your first name: My
Do you have a middle name?(Y/N): y
Please enter your middle name: Programming
Please enter your last name: NotesYour full name is My Programming Notes
The total number of characters in your name is: 18
-----------------------------------------------------------------------Please enter your first name: Programming
Do you have a middle name?(Y/N): N
Please enter your last name: NotesYour full name is Programming Notes
The total number of characters in your name is: 16
-----------------------------------------------------------------------Please enter your first name: Notes
Do you have a middle name?(Y/N): y
Please enter your middle name: Notes
Please enter your last name: NotesYour full name is Notes Notes Notes
The total number of characters in your name is: 15
Your first and middle name are the same
Your middle and last name are the same
Your first and last name are the same
-----------------------------------------------------------------------Please enter your first name: My
Do you have a middle name?(Y/N): N
Please enter your last name: MyYour full name is My My
The total number of characters in your name is: 4
Your first and last name are the same
-----------------------------------------------------------------------Please enter your first name: My
Do you have a middle name?(Y/N): zPlease press either 'Y' or 'N'
Program exiting...