Tag Archives: for loop
Java || Compute The Sum From A String Of Integers & Display Each Number Individually
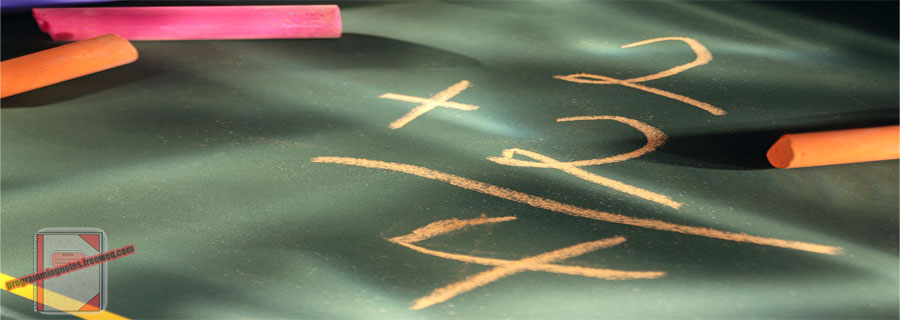
Here is a simple program, which was presented in a Java course. This program was used to introduce the input/output mechanisms which are available in Java. This assignment was modeled after Exercise 2.30, taken from the textbook “Java How to Program” (early objects) (9th Edition) (Deitel). It is the same exercise in both the 8th and 9th editions.
Our class was asked to make a program which prompts the user to enter a non-negative integer into the system. The program was supposed to then extract the digits from the inputted number, displaying them each individually, separated by a white space (” “). After the digits are displayed, the program was then supposed to display the sum of those digits to the screen. So for example, if the user inputted the number “39465,” the program would output the numbers 3 9 4 6 5 individually, and then the sum of 27.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Do/While Loops
For Loops
Methods (A.K.A "Functions") - What Are They?
ParseInt - Convert String To Integer
Substring
Try/Catch
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
import java.util.Scanner; import java.lang.Math; public class SumFromString { public static void main(String[] args) { // declare variables char question = 'n'; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); do{// this is the start of the do/while loop int sum = 0; // get data from the user System.out.print("nEnter a non negative integer: "); userInput = cin.nextLine(); System.out.println(""); // if the string isnt a number, dont compute the sum if(!IsNumeric(userInput)) { System.out.println(userInput+" is not a number..."); System.out.println("Please enter digits only!"); } // if the string is a negative number, dont compute the sum else if(IsNegative(userInput)) { System.out.println(userInput+" is a negative number..."); System.out.println("Please enter positive digits only!"); } // if the string is a decimal number (i.e 19.87), dont compute the sum else if(IsDecimal(userInput)) { System.out.println(userInput+" is a decimal number..."); System.out.println("Please enter positive whole numbers only!"); } // if everything else checks out OK, then compute the sum else { System.out.print("The digits are: "); sum = ComputeSum(userInput); System.out.println("and the sum is " + sum); } // ask user if they want to enter more data System.out.print("nDo you have more data for input? (Y/N): "); String response = cin.nextLine(); response=response.toLowerCase(); question = response.charAt(0); System.out.println("n------------------------------------------------"); }while(question == 'y'); System.out.print("nBYE!n"); }// end of main public static boolean IsNumeric(String str) {// checks to see if a string is a number try { Double.parseDouble(str); return true; } catch(NumberFormatException nfe) { return false; } }// end of IsNumeric public static boolean IsNegative(String str) {// checks to see if string is a negative number return Double.parseDouble(str) < 0; }// end of IsNegative public static boolean IsDecimal(String str) {// checks to see if string is a decimal number return (Math.floor(Double.parseDouble(str))) != Double.parseDouble(str); }// end of IsDecimal public static int ComputeSum(String userInput) {// displays each number individually & computes the sum of the string int sum = 0; String[] temp = new String[userInput.length()]; for(int counter=0; counter < userInput.length(); ++counter) { temp[counter] = userInput.substring(counter,counter+1); System.out.print(temp[counter] + " "); sum = sum + Integer.parseInt(temp[counter]); } return sum; }// end of ComputeSum }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Enter a non negative integer: 0
The digits are: 0 and the sum is 0
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 39465The digits are: 3 9 4 6 5 and the sum is 27
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: H3ll0 W0rldH3ll0 W0rld is not a number...
Please enter digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: -98-98 is a negative number...
Please enter positive digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 19.8719.87 is a decimal number...
Please enter positive whole numbers only!Do you have more data for input? (Y/N): n
------------------------------------------------
BYE!
C++ || Char Array – Determine If A String Is A Number Or Not
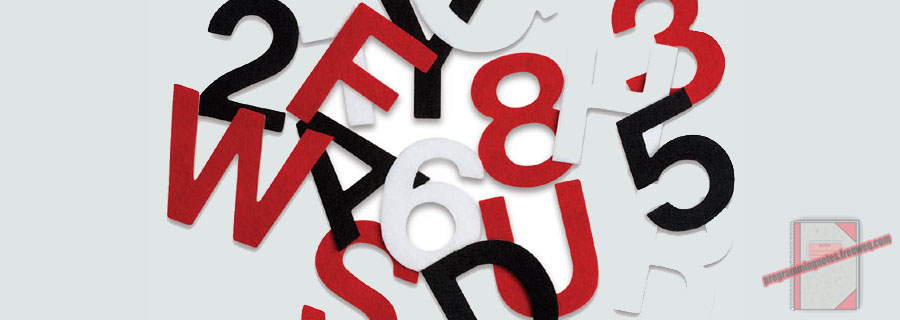
The following is another intermediate homework assignment which was presented in a C++ programming course. This program was assigned to introduce more practice using and manipulating character arrays.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Character Arrays
Cin.getline
Strlen - Get The Length Of A Char Array
Isalpha
Isspace
This program first prompts the user to input a line of text. After it obtains data from the user, using a for loop, it then displays the the string to the screen one letter (char) at a time. If the current character at that specific array index is a letter, a “flag” is set, indicating that the current word which is being displayed is not a number. If the “flag” is not set, the current word is indeed a number.
This program has the ability to intake multiple words at a time, so for example, if the user input was “Hello World 2012” the program would display the output:
Hello is NOT a number!
World is NOT a number!
2012 is a number..
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 24, 2012 // Taken From: http://programmingnotes.org/ // File: isNumber.cpp // Description: Demonstrates checking if a char array is a number // ============================================================================ #include <iostream> #include <cstring> #include <cctype> using namespace std; // function prototype void IsArryANum(char arry[]); int main() { // declare & initialize variables char arry[256]; // obtain data from user cout << "Enter some text to see if its a number or not: "; cin.getline(arry, sizeof(arry)); // getting line cout<<endl; IsArryANum(arry); return 0; }// end of main void IsArryANum(char arry[]) { int notANumber = 0; int length = strlen(arry); // get the length of the char array // and place a [space] at the end of it. Then // set the array index after the [space] to NULL arry[length] = ' '; arry[length + 1] = '\0'; // increment the length to account for the space we just added ++length; for(int x = 0; x < length; ++x) { cout <<arry[x]; // display the char at the current index // if the current char isnt a number, increment counter if(isalpha(arry[x])) { ++notANumber; } // if curerent char is a space, that indicates we are // at the end of the current word, so we // display the results to the user else if(isspace(arry[x])) { if (notANumber > 0) { cout <<"is NOT a number!" <<" There are "<<notANumber<<" letters" <<" in that word...\n"; } else { cout <<"is a number..\n"; } notANumber = 0; } } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: the code was compiled three separate times to display different output)
====== RUN 1 ======
Enter some text to see if its a number or not: My Programming Notes
My is NOT a number! There are 2 letters in that word...
Programming is NOT a number! There are 11 letters in that word...
Notes is NOT a number! There are 5 letters in that word...====== RUN 2 ======
Enter some text to see if its a number or not: May 30th 2012
May is NOT a number! There are 3 letters in that word...
30th is NOT a number! There are 2 letters in that word...
2012 is a number..====== RUN 3 ======
Enter some text to see if its a number or not: 5 31 2012
5 is a number..
31 is a number..
2012 is a number..
C++ || FizzBuzz – Tackling The Fizz Buzz Test In C++
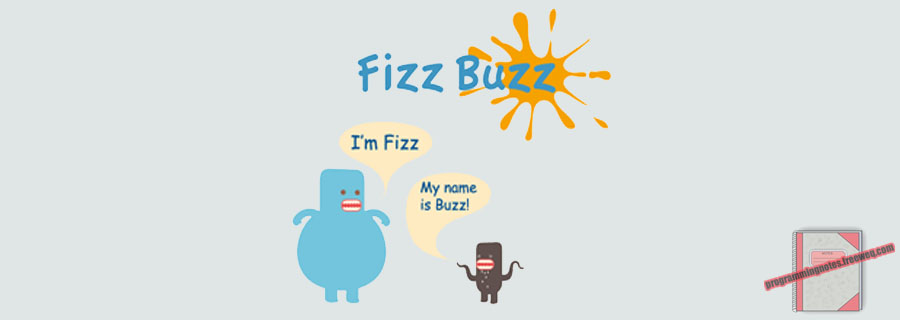
What is Fizz Buzz?
Simply put, a “Fizz-Buzz test” is a programming interview question designed to help filter out potential job prospects – those who can’t seem to program if their life depended on it.
An example of a typical Fizz-Buzz question is the following:
Write a program which prints the numbers from 1 to 100. But for multiples of three, print the word “Fizz” instead of the number, and for the multiples of five, print the word “Buzz”. For numbers which are multiples of both three and five, print the word “FizzBuzz”.
This seems easy enough, and many should be able to complete a program which carries out a solution in a few minutes. Though, after doing a little research, apparently that is not the case. This page will present one way to carry out a solution to this Fizz-Buzz problem.
There is a small “catch” that some may encounter when trying to solve this problem, and that is the fact that the conditional statement for the number divisible by 15 should come before each sequential conditional statement. Consider this pseudocode:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// A simple Fizz-Buzz algorithm using a while loop 'currentNumber' = 1 while('currentNumber' is less than or equal to 100) { if('currentNumber' is divisible by 3) AND ('currentNumber' is divisible by 5) print "FizzBuzz" else if('currentNumber' is divisible by 3) print "Fizz" else if('currentNumber' is divisible by 5) print "Buzz" else // 'currentNumber' is not divisible by 3 or 5 print 'currentNumber' increment 'currentNumber' by one }// http://programmingnotes.org/ |
The portion that may make this problem tricky for some is the fact that the conditional statement for the number divisible by 15 must be checked -before- the conditional statements which checks for numbers divisible by 3 and 5. If the conditional statements are placed in any other order, the end result will not be correct, which is what can make the problem difficult for many.
======= THE FIZZ BUZZ TEST =======
So building upon the pseudocode found from above, utilizing the modulus operator, here is a simple solution to the Fizz Buzz Test
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 10, 2012 // Taken From: http://programmingnotes.org/ // File: fizzbuzz.cpp // Description: Demonstrates the fizz buzz test // ============================================================================ #include <iostream> using namespace std; int main() { // declare variables int fizz = 3; int buzz = 5; int endNumber = 100; int fizzBuzz = fizz * buzz; // ^ numbers divisible by 3 and 5 are also divisible by 3 * 5 // start the loop, continue until the counter // reaches the 'end' for (int currentNumber = 1; currentNumber <= endNumber; ++currentNumber) { if (currentNumber % fizzBuzz == 0) // divisible by 3 and 5 { cout<<"FIZZ BUZZ!!\n"; } else if (currentNumber % fizz == 0) // divisible by 3 { cout<<"FIZZ\n"; } else if (currentNumber % buzz == 0)// divisible by 5 { cout<<"BUZZ\n"; } else // not divisible by 3 or 5 { cout<<currentNumber<<endl; } } return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
1
2
FIZZ
4
BUZZ
FIZZ
7
8
FIZZ
BUZZ
11
FIZZ
13
14
FIZZ BUZZ!!
16
17
FIZZ
19
BUZZ
FIZZ
22
23
FIZZ
BUZZ
26
FIZZ
28
29
FIZZ BUZZ!!
31
32
FIZZ
34
BUZZ
FIZZ
37
38
FIZZ
BUZZ
41
FIZZ
43
44
FIZZ BUZZ!!
46
47
FIZZ
49
BUZZ
FIZZ
52
53
FIZZ
BUZZ
56
FIZZ
58
59
FIZZ BUZZ!!
61
62
FIZZ
64
BUZZ
FIZZ
67
68
FIZZ
BUZZ
71
FIZZ
73
74
FIZZ BUZZ!!
76
77
FIZZ
79
BUZZ
FIZZ
82
83
FIZZ
BUZZ
86
FIZZ
88
89
FIZZ BUZZ!!
91
92
FIZZ
94
BUZZ
FIZZ
97
98
FIZZ
BUZZ
C++ || Class – Roman Numeral To Integer & Integer To Roman Numeral Conversion
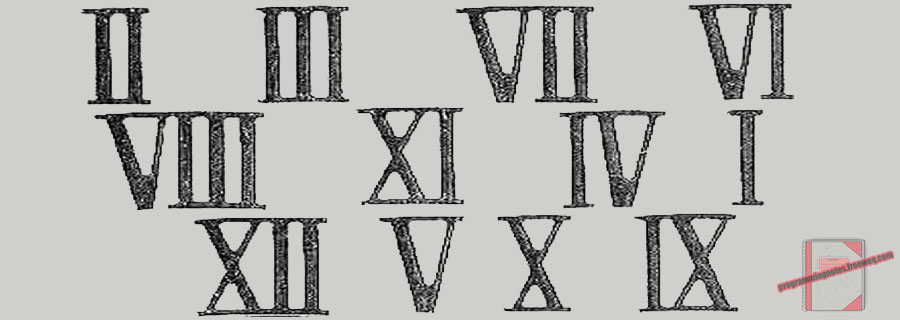
The following is another homework assignment which was presented in a C++ Data Structures course. This program was assigned in order to practice the use of the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
Do/While Loop
Passing a Value By Reference
Roman Numerals - How Do You Convert To Decimal?
Online Roman Numeral Converter - Check For Correct Results
This is an interactive program in which the user has the option of selecting from 7 modes of operation. Of those modes, the user has the option of entering in roman numerals for conversion, entering in decimal numbers for conversion, displaying the recently entered number, and finally converting between roman or decimal values.
A sample of the menu is as followed:
(Where the user would enter numbers 1-7 to select a choice)
1 2 3 4 5 6 7 8 9 10 11 |
From the following menu: 1. Enter a Decimal number 2. Enter a Roman Numeral 3. Convert from Decimal to Roman 4. Convert from Roman to Decimal 5. Print the current Decimal number 6. Print the current Roman Numeral 7. Quit Please enter a selection: |
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
======== FILE #1 – Menu.cpp ========
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
// ============================================================================ // File: Menu.cpp // Purpose: Converts a number enetered in Decimal to Roman Numeral and a // Roman Numeral to a Decimal Number // ============================================================================ #include <iostream> #include <iomanip> #include <string> #include "ClassRomanType.h" using namespace std; // function prototypes void DisplayMenu(); int GetCommand(int command); void RunChoice(int command, ClassRomanType& romanObject); int main() { int command=7; // Holds which action the user chooses to make ClassRomanType romanObject; // Gives access to the class do{ DisplayMenu(); command = GetCommand(command); RunChoice(command, romanObject); cout<<"n--------------------------------------------------------------------n"; }while(command!=7); cout<<"nBye!n"; return 0; }// End of main void RunChoice(int command, ClassRomanType& romanObject) {// Function: Execute the choice selected from the menu // declare private variables int decimalNumber=0; // Holds decimal value char romanNumeral[150]; //Holds value of roman numeral // execute the desired choice cout <<"ntYou selected choice #"<<command<<" which will: "; switch(command) { case 1: cout << "Get Decimal Numbernn"; cout <<"Enter a Decimal Number: "; cin >> decimalNumber; romanObject.GetDecimalNumber(decimalNumber); break; case 2: cout << "Get Roman Numeralnn"; cout <<"nEnter a Roman Numeral: "; cin >> romanNumeral; romanObject.GetRomanNumeral(romanNumeral); break; case 3: cout << "Convert from Decimal to Romannn"; romanObject.ConvertDecimalToRoman(); cout<<"Running....nComplete!n"; break; case 4: cout << "Convert from Roman to Decimalnn"; romanObject.ConvertRomanToDecimal(); cout<<"Running....nComplete!n"; break; case 5: cout << "Print the current Decimal numbernn"; cout<<"n The current Roman to Decimal Value is: "; cout << romanObject.ReturnDecimalNumber()<<endl; break; case 6: cout << "Print the current Roman Numeralnn"; cout<<"n The current Decimal to Roman Value is: "; cout << romanObject.ReturnRomanNumber()<<endl; break; case 7: cout << "Quitnn"; break; default: cout << "nError, you entered an invalid command!nPlease try again..."; break; } }//End of RunChoice int GetCommand(int command) {// Function: Gets choice from menu cout <<"nPlease enter a selection: "; cin>> command; return command; }// End of GetCommand void DisplayMenu() {// Function: Displays menu cout <<"From the following menu:n"<<endl; cout <<left<<setw(2)<<"1."<<left<<setw(15)<<" Enter a Decimal number"<<endl; cout <<left<<setw(2)<<"2."<<left<<setw(15)<<" Enter a Roman Numeral"<<endl; cout <<left<<setw(2)<<"3."<<left<<setw(15)<<" Convert from Decimal to Roman"<<endl; cout <<left<<setw(2)<<"4."<<left<<setw(15)<<" Convert from Roman to Decimal"<<endl; cout <<left<<setw(2)<<"5."<<left<<setw(15)<<" Print the current Decimal number"<<endl; cout <<left<<setw(2)<<"6."<<left<<setw(15)<<" Print the current Roman Numeral"<<endl; cout <<left<<setw(2)<<"7."<<left<<setw(15)<<" Quit"<<endl; }// http://programmingnotes.org/ |
======== FILE #2 – ClassRomanType.h ========
Remember, you need to name the header file the same as the #include from the Menu.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
// ============================================================================ // File: ClassRomanType.h // Purpose: Header file for Roman Numeral conversion. It contains all // declarations and prototypes for the class members to carry out the // conversion // ============================================================================ #include <string> using namespace std; class ClassRomanType { public: ClassRomanType(); /* Function: constructor initializes class variables to "0" Precondition: none Postcondition: defines private variables */ // member functions void GetDecimalNumber(int decimalNumber); /* Function: gets decimal number Precondition: none Postcondition: Gets arabic number from user */ void GetRomanNumeral(char romanNumeral[]); /* Function: gets roman numeral Precondition: none Postcondition: Gets roman numeral from user */ void ConvertDecimalToRoman(); /* Function: converts decimal number to ronman numeral Precondition: arabic and roman numeral characters should be known Postcondition: Gets roman numeral from user */ void ConvertRomanToDecimal(); /* Function: converts roman numeral to arabic number Precondition: arabic and roman numeral characters should be known Postcondition: Gets decimal number from user */ int ReturnDecimalNumber(); /* Function: displays converted decimal number Precondition: the converted roman numeral to decimal number Postcondition: displays decimal number to user */ string ReturnRomanNumber(); /* Function: displays converted roman numeral Precondition: the converted decimal number to roman numeral Postcondition: displays roman numeral to user */ ~ClassRomanType(); /* Function: destructor used to return memory to the system Precondition: the converted decimal number to roman numeral Postcondition: none */ private: char m_romanValue[150]; // An array holding the roman value within the class int m_decimalValue; // Holds the final output answer of the roman to decimal conversion }; // http://programmingnotes.org/ |
======== FILE #3 – RomanType.cpp ========
This is the function implementation file for the ClassRomanType.h class. This file can be named anything you wish as long as you #include “ClassRomanType.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 |
// ============================================================================ // File: RomanType.cpp // Purpose: Contains the implementation of the class members for the // roman numeral conversion // // *NOTE*: Uncomment cout statements to visualize whats happening // ============================================================================ #include <iostream> #include <string> #include <cstring> #include "ClassRomanType.h" using namespace std; ClassRomanType::ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// end of ClassRomanType void ClassRomanType:: GetDecimalNumber(int decimalNumber) { m_decimalValue = decimalNumber; }// end of GetDecimalNumber void ClassRomanType:: GetRomanNumeral(char romanNumeral[]) { strcpy(m_romanValue,romanNumeral); }// end of GetRomanNumeral void ClassRomanType:: ConvertDecimalToRoman() { strcpy(m_romanValue,""); // erases any previous data int numericValue[]={1000,900,500,400,100,90,50,40,10,9,5,4,1,0}; char *romanNumeral[]={"M","CM","D","CD","C","XC","L" ,"XL","X","IX","V","IV","I",0}; for (int x=0; numericValue[x] > 0; ++x) { //cout<<endl<<numericValue[x]<<endl; while (m_decimalValue >= numericValue[x]) { //cout<<"current m_decimalValue = " <<m_decimalValue<<endl; //cout<<"current numericValue = "<<numericValue[x]<<endl<<endl; if(strlen(m_romanValue)==0) { strcpy(m_romanValue,romanNumeral[x]); } else { strcat(m_romanValue,romanNumeral[x]); } m_decimalValue -= numericValue[x]; } } }// end of ConvertDecimalToRoman void ClassRomanType:: ConvertRomanToDecimal() { int currentNumber = 0; // Holds value of each letter numerical value int previousNumber = 0; // Holds the numerical value being compared m_decimalValue=0; // Resets the current 'romanToDecimalValue' to zero if(strcmp(m_romanValue,"Currently Undefined")!=0) { for (int counter = strlen(m_romanValue)-1; counter >= 0; counter--) { if ((m_romanValue[counter] == 'M') || (m_romanValue[counter] == 'm')) { currentNumber = 1000; } else if ((m_romanValue[counter] == 'D') || (m_romanValue[counter] == 'd')) { currentNumber = 500; } else if ((m_romanValue[counter] == 'C') || (m_romanValue[counter] == 'c')) { currentNumber = 100; } else if ((m_romanValue[counter] == 'L') || (m_romanValue[counter] == 'l')) { currentNumber = 50; } else if ((m_romanValue[counter] == 'X') || (m_romanValue[counter] == 'x')) { currentNumber = 10; } else if ((m_romanValue[counter] == 'V') || (m_romanValue[counter] == 'v')) { currentNumber = 5; } else if ((m_romanValue[counter] == 'I') || (m_romanValue[counter] == 'i')) { currentNumber = 1; } else { currentNumber = 0; } //cout<<"previousNumber = " <<previousNumber<<endl; //cout<<"currentNumber = " <<currentNumber<<endl; if (previousNumber > currentNumber) { m_decimalValue -= currentNumber; previousNumber = currentNumber; } else { m_decimalValue += currentNumber; previousNumber = currentNumber; } //cout<<"current m_decimalValue = " <<m_decimalValue<<endl<<endl; } } }// end of ConvertRomanToDecimal int ClassRomanType::ReturnDecimalNumber() { return m_decimalValue; }// end of ReturnDecimalNumber string ClassRomanType::ReturnRomanNumber() { return m_romanValue; }// end of ReturnRomanNumber ClassRomanType::~ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
From the following menu:
1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 9
You selected choice #9 which will:
Error, you entered an invalid command!
Please try again...
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 0
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: Currently Undefined
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 1
You selected choice #1 which will: Get Decimal Number
Enter a Decimal Number: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 3
You selected choice #3 which will: Convert from Decimal to Roman
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: MCMLXXXVII
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 2
You selected choice #2 which will: Get Roman Numeral
Enter a Roman Numeral: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 4
You selected choice #4 which will: Convert from Roman to Decimal
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 2012
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 7
You selected choice #7 which will: Quit
--------------------------------------------------------------------
Bye!
C++ || Printing Various Patterns Using Nested Loops
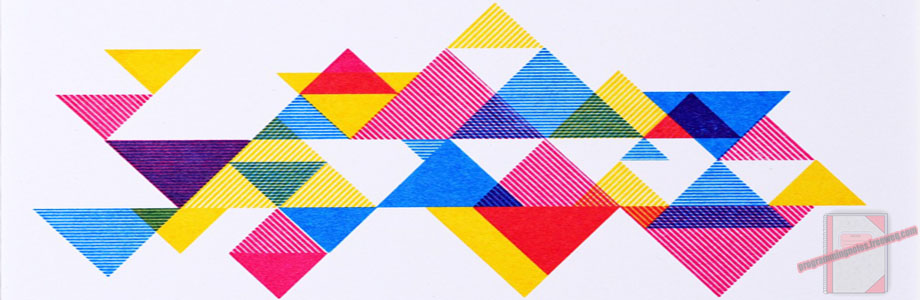
This page will demonstrate various programs which illustrates the use of nested loops to print selected patterns to the screen.
REQUIRED KNOWLEDGE FOR THIS PAGE
The following are famous homework assignments which are usually presented in an entry level programming course.
There are a total of ten (10) different patterns on this page, which is broken up into sections. This page will list:
(4) methods of printing a triangle
(4) methods of printing an upside down triangle
(1) method which prints a square
(1) method which prints a giant letter 'X'
======= PRINTING A TRIANGLE =======
This program prints a triangle shape to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; // get data from user cout<<"Enter a number: "; cin>>number; // this is the start of the nested loop. // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=0; x < counter; ++x) { cout<<"* "; } cout<<endl; ++counter; } return 0; }// http://programmingnotes.org/ |
In the above example, the user has a choice of entering the number of rows which will be displayed to the screen
SAMPLE OUTPUT:
Enter a number: 9
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
* * * * * * * * *
======= PRINTING A TRIANGLE WITH NUMBERS =======
The following program uses the same concept as above, but this time instead of using stars “*”, numbers will be printed to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this variable is just a copy // of the number that the user just entered // which will be used in the nested loop copy=number; // this is the start of the nested loop. // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=0; x < counter; ++x) { cout<<copy<<" "; } cout<<endl; --copy; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9
8 8
7 7 7
6 6 6 6
5 5 5 5 5
4 4 4 4 4 4
3 3 3 3 3 3 3
2 2 2 2 2 2 2 2
1 1 1 1 1 1 1 1 1
======= PRINTING A TRIANGLE WITH NUMBERS IN-ORDER =======
The following program uses the same concept as above, but this time instead of using stars “*”, numbers will be printed to the screen in-order.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this is the start of the nested loop. // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { copy=number; for(int x=0; x < counter; ++x) { cout<<copy-(counter-1)<<" "; ++copy; } cout<<endl; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9
8 9
7 8 9
6 7 8 9
5 6 7 8 9
4 5 6 7 8 9
3 4 5 6 7 8 9
2 3 4 5 6 7 8 9
1 2 3 4 5 6 7 8 9
======= PRINTING A TRIANGLE WITH NUMBERS USING MULTIPLICATION =======
This example demonstrates another triangle, this time printing a multiplication table.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; // get data from user cout<<"Enter a number: "; cin>>number; // this is the start of the nested loop // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=1; x <= counter; ++x) { cout<<number*x<<" "; } cout<<endl; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9
9 18
9 18 27
9 18 27 36
9 18 27 36 45
9 18 27 36 45 54
9 18 27 36 45 54 63
9 18 27 36 45 54 63 72
9 18 27 36 45 54 63 72 81
======= PRINTING AN UPSIDE-DOWN TRIANGLE =======
This program is similar to the first one, this time printing the triangle upside-down.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=0; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this variable is just a copy // of the number that the user just entered // which will be used in the nested loop copy=number; // this is the start of the nested loop // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=1; x <= copy; ++x) { cout<<"* "; } cout<<endl; --copy; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
* * * * * * * * *
* * * * * * * *
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
======= PRINTING AN UPSIDE-DOWN TRIANGLE WITH NUMBERS =======
This program is similar to the second one, this time printing the triangle upside-down.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this variable is just a copy // of the number that the user just entered // which will be used in the nested loop copy=number; // this is the start of the nested loop // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=1; x <= copy; ++x) { cout<<copy<<" "; } cout<<endl; --copy; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9 9 9 9 9 9 9 9 9
8 8 8 8 8 8 8 8
7 7 7 7 7 7 7
6 6 6 6 6 6
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1
======= PRINTING AN UPSIDE-DOWN TRIANGLE WITH NUMBERS IN-ORDER =======
The following program uses the same concept as above, but this time instead of using stars “*”, numbers will be printed to the screen in-order.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; copy=number; // this is the start of the nested loop. // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=counter; x <= copy; ++x) { cout<<x<<" "; } cout<<endl; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
1 2 3 4 5 6 7 8 9
2 3 4 5 6 7 8 9
3 4 5 6 7 8 9
4 5 6 7 8 9
5 6 7 8 9
6 7 8 9
7 8 9
8 9
9
===== PRINTING AN UPSIDE-DOWN TRIANGLE WITH NUMBERS USING MULTIPLICATION =====
This program is similar to the third one, this time printing the triangle upside-down.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include <iostream> using namespace std; int main() { // declare variables int number=0; int counter=1; int copy=0; // get data from user cout<<"Enter a number: "; cin>>number; // this variable is just a copy // of the number that the user just entered // which will be used in the nested loop copy=number; // this is the start of the nested loop // NOTE: many use two nested 'for' loops, but // sometimes its nice to switch things up while(counter <= number) { for(int x=1; x <= copy; ++x) { cout<<number*x<<" "; } cout<<endl; --copy; ++counter; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
Enter a number: 9
9 18 27 36 45 54 63 72 81
9 18 27 36 45 54 63 72
9 18 27 36 45 54 63
9 18 27 36 45 54
9 18 27 36 45
9 18 27 36
9 18 27
9 18
9
======= PRINTING A SQUARE =======
This program prints a square to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
#include <iostream> using namespace std; int main() { // declare variables int row=0; int column=0; int number=0; cout<<"Enter the number of rows to be printed: "; cin >> number; row = number; // draw top while (row > 0) { cout<<"*"; --row; } cout<<endl; row = number - 2; column = number; // draw middle while (row > 0) { while (column > 0) { if ((column == number) || (column == 1)) { cout<<"*"; } else { cout<<" "; } --column; } cout<<endl; column = number; --row; } row = number; // draw bottom while (row > 0) { cout<<"*"; --row; } cout<<endl; return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
1 2 3 4 5 6 7 8 9 10 |
Enter the number of rows to be printed: 9 ********* * * * * * * * * * * * * * * ********* |
======= PRINTING THE LETTER “X” =======
The final program for this page will print a giant letter “X” to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
#include <iostream> #include <cstdlib> using namespace std; int main() { // declare variables int number=0; int counter1=0; cout<<"Enter the size of the shape: "; cin >> number; // set 'counter1' equal to 'number' counter1=-number; // start of the nested loop while(counter1 <=number) { for (int counter2 = -number; counter2 <= number; ++counter2) { if (abs(counter1) == abs(counter2)) { cout<<"x"; } else { cout<<" "; } } ++counter1; cout<<endl; } return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
Enter the size of the shape: 9 x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x x |
And there you have it. Simple shapes made possible in C++.
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || Savings Account Balance – Calculate The Balance Of A Savings Account At The End Of A Period
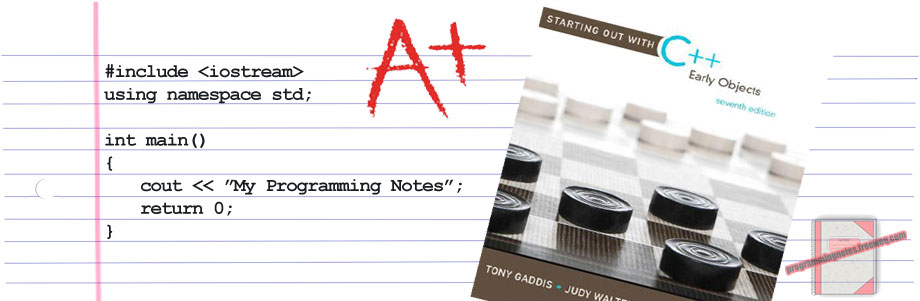
Here is another actual homework assignment which was presented in an intro to programming class. The following program was a question taken from the book “Starting Out with C++: Early Objects (7th Edition),” chapter 5, problem #16.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
For Loops - How To Use Them
Assignment Operators - What Are They?
Setprecision - What Is It?
Interest Rate
This program first prompts the user to enter the annual interest rate, starting balance, and the number of months which has passed since the account was established. Upon obtaining the information, a loop is then used to iterate through each month, performing the following:
• Ask the user for the amount deposited into the account during the month (positive values only). This amount is added to the balance.
• Ask the user for the amount withdrawn from the account during the month (positive values only). This is subtracted from the balance.
• Calculate the monthly interest. The monthly interest rate is the annual interest rate divided by 12. After each loop iteration, the monthly interest rate is multiplied by the current balance, which is then added to the total balance.
After the last iteration, the program displays the ending balance, the total amount of deposits, the total amount of withdrawals, and the total interest earned.
If a negative balance is calculated at any point, an erroneous message is displayed to the screen indicating that the account has been closed, and then the program terminates.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 |
// ============================================================================ // Author: Kenneth Perkins // Date: April 4, 2012 // Taken From: http://programmingnotes.org/ // File: SavingsAccount.cpp // Description: Demonstrates how to calculate the balance of a savings // account at the end of a period // ============================================================================ #include <iostream> #include <cstdlib> #include <iomanip> using namespace std; int main() { // declare variables double annInterestRate = 0; double startingBalance = 0; int monthsPassed = 0; double deposits = 0; double withdrawal = 0; double totDeposits = 0; double totWithdrawal = 0; double totInterest = 0; double monthlyInterestRate = 0; double totBalance = 0; // obtain data from user cout<<"Enter the annual interest rate on the account (e.g .04): "; cin >> annInterestRate; cout<<"Enter the starting balance: $"; cin >> startingBalance; cout<<"How many months have passed since the account was established? "; cin >> monthsPassed; // initialize variables with the required info totBalance = startingBalance; monthlyInterestRate = annInterestRate/12; // use a loop to obtain more data from user for(int x=1; x <= monthsPassed; ++x) { cout<<"\nMonth #"<<x<<endl; cout<<"\tTotal deposits for this month: $"; cin >> deposits; totDeposits += deposits; totBalance += deposits; cout<<"\tTotal withdrawal for this month: $"; cin >> withdrawal; totWithdrawal += withdrawal; totBalance -= withdrawal; totInterest += (totBalance*monthlyInterestRate); totBalance += (totBalance*monthlyInterestRate); // check for negative values // end loop if the values are negative if(deposits < 0 || withdrawal < 0 || totBalance < 0) { cout<< "\nPlease enter positive numbers only!"; cout<<"\nThe account has been closed..\n"; exit(1); } } // display results cout<<fixed<<setprecision(2); cout.fill('.'); cout<<"\nEnding balance:"<<left<<setw(20)<<right<<setw(20)<<" $ "<<totBalance; cout<<"\nAmount of deposits:"<<left<<setw(20)<<right<<setw(16)<<" $ "<<totDeposits; cout<<"\nAmount of withdrawals:"<<left<<setw(20)<<right<<setw(13)<<" $ "<<totWithdrawal; cout<<"\nAmount of interest earned:"<<left<<setw(20)<<right<<setw(9)<<" $ "<<totInterest<<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Enter the annual interest rate on the account (e.g .04): .07
Enter the starting balance: $5000
How many months have passed since the account was established? 3Month #1
Total deposits for this month: $500
Total withdrawal for this month: $120Month #2
Total deposits for this month: $750
Total withdrawal for this month: $200Month #3
Total deposits for this month: $500
Total withdrawal for this month: $100Ending balance:................. $ 6433.47
Amount of deposits:............. $ 1750.00
Amount of withdrawals:.......... $ 420.00
Amount of interest earned:...... $ 103.47
C++ || “One Size Fits All” – BubbleSort Which Works For Integer, Float, & Char Arrays
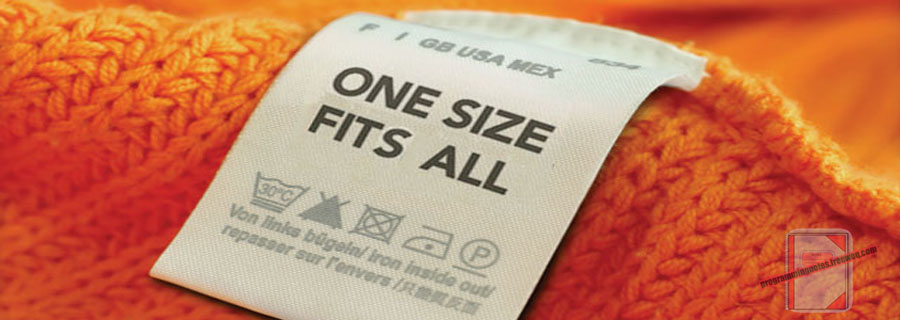
Here is another sorting algorithm, which sorts data that is contained in an array. The difference between this method, and the previous methods of sorting data is that this method works with multiple data types, all in one function. So for example, if you wanted to sort an integer and a character array within the same program, the code demonstrated on this page has the ability to sort both data types, eliminating the need to make two separate sorting functions for the two different data types.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Integer Arrays
Character Arrays
BubbleSort
Pointers
Function Pointers - What Are They?
Memcpy
Sizeof
Sizet
Malloc
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 |
#include <iostream> #include <cstdlib> #include <cctype> #include <iomanip> #include <cstring> #include <ctime> using namespace std; // function prototypes void BubbleSort(void* list, size_t nelm, size_t size, int (*cmp) (const void*, const void*)); void Swap(void* a, void* b, size_t width); int IntCompare (const void * a, const void * b); int StrCompare(const void *a, const void *b); int FloatCompare (const void * a, const void * b); // constant variable for int arrays const int NUM_INTS=14; int main() { // seed random number generator srand(time(NULL)); // =================== INT EXAMPLE =================== // int intNumbers[100]; int numInts=0; // place random numbers into the array for(int x = 0; x < NUM_INTS; ++x) { intNumbers[x] =rand()%100+1; } cout<<"Original values in the int array:n"; // show original data to the user for(int x=0; x<NUM_INTS; ++x) { cout << setw(4) << intNumbers[x]; } // this is the function call, which takes in an // integer array for sorting purposes // NOTICE: the function "IntCompare" is being used as a parameter BubbleSort(intNumbers,NUM_INTS,sizeof(int),IntCompare); cout<<"nnThe sorted values in the int array:n"; // display sorted array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(4) << intNumbers[x]; } // creates a line seperator cout << "n-----------------------------------------------" <<"----------------------------------------n"; // =================== FLOAT EXAMPLE =================== // float floatNumbers[100]; // place random numbers into the array for(int x = 0; x < NUM_INTS; ++x) { floatNumbers[x] =(rand()%100+1)+(0.5); } cout<<"Original values in the float array:n"; // show original array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(6) << floatNumbers[x]; } // this is the function call, which takes in a // float array for sorting purposes // NOTICE: the function "FloatCompare" is being used as a parameter BubbleSort(floatNumbers,NUM_INTS,sizeof(float),FloatCompare); cout<<"nnThe sorted values in the float array:n"; // show sorted array to user for(int x=0; x<NUM_INTS; ++x) { cout << setw(6) << floatNumbers[x]; } // creates a line seperator cout << "n-----------------------------------------------" <<"----------------------------------------n"; // =================== CHAR EXAMPLE =================== // char* names[100]={"This", "Is","Random","Text","Brought","To", "You","By","My","Programming","Notes"}; int numNames=11; cout<<"Original values in the char array:n"; // show original array to user for(int x=0; x<numNames; ++x) { cout << " " << names[x]; } // this is the function call, which takes in a // char array for sorting purposes // NOTICE: the function "StrCompare" is being used as a parameter BubbleSort(names,numNames,sizeof(char*),StrCompare); cout<<"nnThe sorted values in the char array:n"; // show sorted array to user for(int x=0; x<numNames; ++x) { cout << " " << names[x]; } cout<<endl; return 0; }// end of main void BubbleSort(void* arry, size_t nelm, size_t size, int (*cmp) (const void*, const void*)) {// this function sorts the received array bool sorted = false; do{ sorted = true; for(unsigned int x=0; x < nelm-1; ++x) { if(cmp((((char*)arry)+(x*size)), (((char*)arry)+((x+1)*size))) > 0) { Swap((((char*)arry)+((x)*size)), (((char*)arry)+((x+1)*size)),size); sorted=false; } } --nelm; }while(!sorted); }// end of BubbleSort void Swap(void* a, void* b, size_t width) {// this function swaps the values in the array void *tmp = malloc(width); memcpy(tmp, a, width); memcpy(a, b, width); memcpy(b, tmp, width); free(tmp); }// end of Swap int FloatCompare (const void * a, const void * b) {// this function compares two float values together return (*(float*)a - *(float*)b); }// end of FloatCompare int IntCompare (const void * a, const void * b) {// this function compares two int values together return (*(int*)a - *(int*)b); }// end of IntCompare int StrCompare(const void *a, const void *b) {// this function compares two char values together return(strcmp(*(const char **)a, *(const char **)b)); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Notice, the same function declaration is being used for all 3 different data types, with the only difference between each function call are the parameters which are being sent out.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: The function works for all three data types)
Original values in the int array:
44 91 43 22 20 100 77 80 84 60 47 91 51 81The sorted values in the int array:
20 22 43 44 47 51 60 77 80 81 84 91 91 100
---------------------------------------------------------------------------------------
Original values in the float array:
49.5 30.5 67.5 50.5 29.5 89.5 78.5 80.5 54.5 7.5 54.5 38.5 56.5 70.5The sorted values in the float array:
7.5 29.5 30.5 38.5 49.5 50.5 54.5 54.5 56.5 67.5 70.5 78.5 80.5 89.5
---------------------------------------------------------------------------------------
Original values in the char array:
This Is Random Text Brought To You By My Programming NotesThe sorted values in the char array:
Brought By Is My Notes Programming Random Text This To You
C++ || Stack Based Postfix Evaluation (Single Digit)
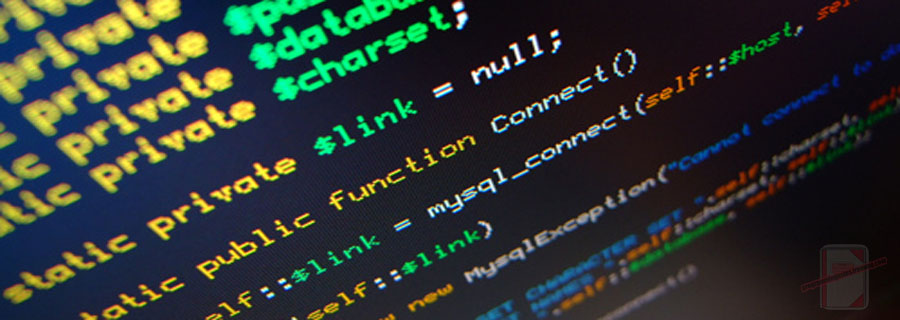
This page consists of another homework assignment which was presented in a C++ Data Structures course. While the previously discussed program dealt with converting Infix expressions to Postfix, this program will demonstrate exactly how to evaluate them.
NOTE: Want to convert & evaluate multi digit, decimal, and negative numbers? Click here!
REQUIRED KNOWLEDGE FOR THIS PROGRAM
What Is Postfix?
How To Convert Infix To Postfix Equations
Stack Data Structure
Cin.getline
How To Evaluate Postfix Expressions
The Order Of Operations
#include "ClassStackType.h"
The title of this page is called – “Stack Based Postfix Evaluation (Single Digit).” Why “single digit?” The program demonstrated on this page has the ability to evaluate a postfix equation, but it only has the ability to evaluate single digit values. What do I mean by that? Consider the infix equation: 5+2. When that expression is converted to postfix, it will come out to be: 52+, and the answer will be 7 (5+2=7). But what if we have an equation like 12+2? When that expression is converted to postfix, it will come out to be: 122+. The postfix conversion is correct, but when you try to evaluate the expression, we do not know if the math operation should be 12+2 or 1+22, it can be read either way.
Question: So why is this program being displayed if it only works for single digits?
Answer: Because it demonstrates the process of evaluating postfix equations very well.
Want to convert & evaluate multi digit, decimal, and negative numbers? Click here!
Before we get into things, here is a helpful algorithm for evaluating a postfix expression in pseudo code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// An algorithm for postfix evaluation. // For example, (1 + 2) / (5 + 6) translates to 1 2 + 5 6 + / // which equals the result of 0.272727 // Valid operands are single digits: 0-9 // Valid operators are: +, -, *, /, ^, $ // Highest precedence: ^, $ // Lowest precedence: +,- // the operators ')' and '('never goes on stack. double EvaluatePostfix(string postfix) { while there is input { if input is a number push current number on stack else if input is a math operator and stack is not empty set operand2 to the top of the operand stack pop the stack set operand1 to the top of the operand stack pop the stack apply the math operation that represents to operand1 and operand2 push the result onto the stack else error } // When the loop is finished, the operand stack will contain one item, // the result of evaluating the expression pop the stack return the answer to the caller }// http://programmingnotes.org/ |
Once you understand the process of converting from infix to postfix, adding the ability to evaluate multiple digits within this program should be doable.
======= POSTFIX EVALUATION =======
This program uses a custom template.h class. To obtain the code for that class, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 |
// ============================================================================ // Author: Kenneth Perkins // Date: Mar 24, 2012 // Taken From: http://programmingnotes.org/ // File: PostfixEvaluation.cpp // Description: Demonstrate the use of a stack based postfix evaluation. // ============================================================================ #include <iostream> #include <cstdlib> #include <cmath> #include "ClassStackType.h" using namespace std; // function prototypes void DisplayDirections(); double EvaluatePostfix(char* postfix); bool IsMathOperator(char token); double DoMath(double op1, double op2, char token); int main() { // declare variables char expression[50]; // array holding the postfix data double answer = 0; // display directions to user DisplayDirections(); // get data from user cout<<"\nPlease enter a postfix expression: "; cin.getline(expression, sizeof(expression)); cout <<"\nThe postfix expression = "<<expression<<endl; cout<<"\nCalculations:\n"; answer = EvaluatePostfix(expression); cout<<"\nFinal answer = "<<answer<<endl; return 0; }// end of main void DisplayDirections() { cout << "\n==== Postfix Evaluation ====\n" <<"\nMath Operators:\n" <<"+ || Addition\n" <<"- || Subtraction\n" <<"* || Multiplication\n" <<"/ || Division\n" <<"% || Modulus\n" <<"^ || Power\n" <<"$ || Square Rootn\n" <<"Sample Postfix Equation: 45^14*232+$2-/12%24*/* \n"; }// end of DisplayDirections double EvaluatePostfix(char* postfix) { // declare function variables int counter = 0; int currentNum = 0; char token = 'a'; double op1 = 0; double op2 = 0; double answer = 0; StackType<double> doubleStack; // loop thru array until there is no more data while(postfix[counter] != '\0') { // push numbers onto the stack if(isdigit(postfix[counter])) { currentNum = postfix[counter] - '0'; doubleStack.Push(currentNum); } else if(isspace(postfix[counter])) { // DO NOTHING } // if expression is a math operator, pop numbers from stack // & send the popped numbers to the 'DoMath' function else if((IsMathOperator(postfix[counter])) && (!doubleStack.IsEmpty())) { token = postfix[counter]; // if expression is square root operation // only pop stack once if(token == '$') { op2 = 0; op1 = doubleStack.Top(); doubleStack.Pop(); answer = DoMath(op1,op2,token); doubleStack.Push(answer); } else { op2 = doubleStack.Top(); doubleStack.Pop(); op1 = doubleStack.Top(); doubleStack.Pop(); answer = DoMath(op1,op2,token); doubleStack.Push(answer); } } else { cout<<"\nINVALID INPUT\n"; exit(1); } ++counter; } // pop the final answer from the stack, and return to main answer = doubleStack.Top(); doubleStack.Pop(); return answer; }// end of EvaluatePostfix bool IsMathOperator(char token) {// this function checks if operand is a math operator switch(token) { case '+': return true; break; case '-': return true; break; case '*': return true; break; case '/': return true; break; case '%': return true; break; case '^': return true; break; case '$': return true; break; default: return false; break; } }// end of IsMathOperator double DoMath(double op1, double op2, char token) {// this function carries out the actual math process double ans = 0; switch(token) { case '+': cout<<op1<<token<<op2<<" = "; ans = op1 + op2; break; case '-': cout<<op1<<token<<op2<<" = "; ans = op1 - op2; break; case '*': cout<<op1<<token<<op2<<" = "; ans = op1 * op2; break; case '/': cout<<op1<<token<<op2<<" = "; ans = op1 / op2; break; case '%': cout<<op1<<token<<op2<<" = "; ans = (int)op1 % (int)op2; break; case '^': cout<<op1<<token<<op2<<" = "; ans = pow(op1, op2); break; case '$': cout<<char(251)<<op1<<" = "; ans = sqrt(op1); break; default: ans = 0; break; } cout<<ans<<endl; return ans; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Want to convert & evaluate multi digit, decimal, and negative numbers? Click here!
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: the code was compile three separate times to display different output)
====== RUN 1 ======
==== Postfix Evaluation ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Postfix Equation: 45^14*232+$2-/12%24*/*
Please enter a postfix expression: 1 2 + 5 6 + /
The postfix expression = 1 2 + 5 6 + /Calculations:
1+2 = 3
5+6 = 11
3/11 = 0.272727
Final answer = 0.272727====== RUN 2 ======
==== Postfix Evaluation ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Postfix Equation: 45^14*232+$2-/12%24*/*
Please enter a postfix expression: 35*76^+
The postfix expression = 35*76^+Calculations:
3*5 = 15
7^6 = 117649
15+117649 = 117664
Final answer = 117664====== RUN 3 ======
==== Postfix Evaluation ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Postfix Equation: 45^14*232+$2-/12%24*/*
Please enter a postfix expression: 45^4*32+$2-/12%24*/*
The postfix expression = 45^4*32+$2-/12%24*/*Calculations:
4^5 = 1024
1024*4 = 4096
3+2 = 5
√5 = 2.23607
2.23607-2 = 0.236068
4096/0.236068 = 17350.9
1%2 = 1
2*4 = 8
1/8 = 0.125
17350.9*0.125 = 2168.87
Final answer = 2168.87
C++ || Stack Based Infix To Postfix Conversion (Single Digit)
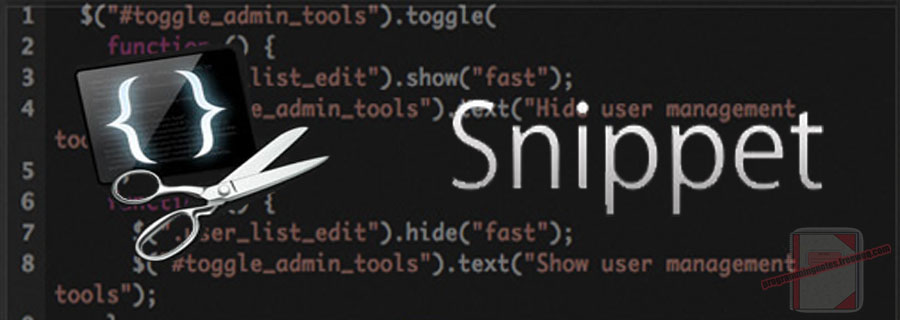
This page consists of another homework assignment which was presented in a C++ Data Structures course. No matter which institution you attend, it seems every instructor assigns a program similar to this at one time or another.
Want to evaluate a postfix expression? Click here.
Want to convert & evaluate multi digit, decimal, and negative numbers? Click here!
REQUIRED KNOWLEDGE FOR THIS PROGRAM
What Is Infix?
What Is Postfix?
Stack Data Structure
Cin.getline
How To Convert To Postfix
The Order Of Operations
#include "ClassStackType.h"
The program demonstrated on this page has the ability to convert a normal infix equation to postfix equation, so for example, if the user enters the infix equation of (1*2)+3, the program will display the postfix result of 12*3+.
Before we get into things, here is a helpful algorithm for converting from infix to postfix in pseudo code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
// An algorithm for infix to postfix expression conversion. // For example, a + b - c translates to a b + c - // a + b * c translates to a b c * + // (1 + 2) / (5 + 6) goes to 1 2 + 5 6 + / // Valid operands are single digits: 0-9, a-z, A-Z // Valid operators are: +, -, *, /, (, ), ^, $ // Highest precedence: ^, $ // Lowest precedence: +,- // ) never goes on stack. // ( has lowest precedence on the stack and highest precedence outside of stack. // Bottom of the stack has the lowest precedence than any operator. // Use a prec() function to compare the precedence of the operators based on the above rules. // Note there is little error checking in the algorithm! void ConvertInfixToPostfix(string infix) { while there is input { if input is a number or a letter place onto postfix string else if input is '(' // '(' has lowest precedence in the stack, highest outside push input on stack else if input is ')' while stack is not empty and top of stack is not '(' place item from top of stack onto postfix string pop stack if stack is not empty // pops '(' off the stack pop stack else error // no matching '(' else if input is a math operator if stack is empty push input on stack else if prec(top of stack) >= prec(current math operator) while stack is not empty and prec(top of stack) >= prec(current math operator) place item from top of stack onto postfix string pop stack push current math operator on stack else error } while stack is not empty { place item from top of stack onto postfix string pop stack } }// http://programmingnotes.org/ |
======= INFIX TO POSTFIX CONVERSION =======
This program uses a custom template.h class. To obtain the code for that class, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 |
// ============================================================================ // Author: Kenneth Perkins // Date: Mar 23, 2012 // Taken From: http://programmingnotes.org/ // File: PostfixConversion.cpp // Description: Demonstrate the use of a stack based infix to // postfix conversion. // ============================================================================ #include <iostream> #include <cctype> #include <cstdlib> #include <cstring> #include "ClassStackType.h" using namespace std; // function prototypes void DisplayDirections(); void ConvertInfixToPostfix(char* infix); int OrderOfOperations(char token); bool IsMathOperator(char token); int main() { // declare variables char expression[50]; // array holding the infix data // display directions to user DisplayDirections(); // get data from user cout<<"\nPlease enter an infix expression: "; cin.getline(expression, sizeof(expression)); cout <<"\nThe Infix expression = "<<expression<<endl; ConvertInfixToPostfix(expression); cout<<"The Postfix expression = "<<expression<<endl; return 0; }// end of main void DisplayDirections() { cout << "\n==== Infix to Postfix Conversion ====\n" <<"\nMath Operators:\n" <<"+ || Addition\n" <<"- || Subtraction\n" <<"* || Multiplication\n" <<"/ || Division\n" <<"% || Modulus\n" <<"^ || Power\n" <<"$ || Square Root\n" <<"Sample Infix Equation: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)\n"; }// end of DisplayDirections void ConvertInfixToPostfix(char* infix) { // declare function variables int infixCounter = 0; int postfixCounter = 0; char token = 'a'; char postfix[50]; StackType<char> charStack; // loop thru array until there is no more data while(infix[infixCounter] != '\0') { // push numbers/letters onto 'postfix' array if(isdigit(infix[infixCounter]) || isalpha(infix[infixCounter])) { postfix[postfixCounter] = infix[infixCounter]; ++postfixCounter; } else if(isspace(infix[infixCounter])) { // DO NOTHING } else if(IsMathOperator(infix[infixCounter])) { // if stack is empty, place first math operator onto stack token = infix[infixCounter]; if(charStack.IsEmpty()) { charStack.Push(token); } else { // get the current math operator from the top of the stack token = charStack.Top(); charStack.Pop(); // use the 'OrderOfOperations' function to check equality // of the math operators while(OrderOfOperations(token) >= OrderOfOperations(infix[infixCounter])) { // if stack is empty, do nothing if(charStack.IsEmpty()) { break; } // place the popped math operator from above ^ // onto the postfix array else { postfix[postfixCounter] = token; ++postfixCounter; // pop the next operator from the stack and // continue the process until complete token = charStack.Top(); charStack.Pop(); } } // push any remainding math operators onto the stack charStack.Push(token); charStack.Push(infix[infixCounter]); } } // push outer parentheses onto stack else if(infix[infixCounter] == '(') { charStack.Push(infix[infixCounter]); } else if(infix[infixCounter] == ')') { // pop the current math operator from the stack token = charStack.Top(); charStack.Pop(); while(token != '(' && !charStack.IsEmpty()) { // place the math operator onto the postfix array postfix[postfixCounter] = token; ++postfixCounter; // pop the next operator from the stack and // continue the process until complete token = charStack.Top(); charStack.Pop(); } } else { cout<<"\nINVALID INPUT\n"; exit(1); } ++infixCounter; } // place any remaining math operators from the stack onto // the postfix array while(!charStack.IsEmpty()) { postfix[postfixCounter] = charStack.Top(); ++postfixCounter; charStack.Pop(); } postfix[postfixCounter] = '\0'; // copy the data from the postfix array into the infix array // the data in the infix array gets sent back to main // since the array is passed by reference strcpy(infix,postfix); }// end of ConvertInfixToPostfix int OrderOfOperations(char token) {// this function checks priority of each math operator int priority = 0; if(token == '^'|| token == '$') { priority = 4; } else if(token == '*' || token == '/' || token == '%') { priority = 3; } else if(token == '-') { priority = 2; } else if(token == '+') { priority = 1; } return priority; }// end of OrderOfOperations bool IsMathOperator(char token) {// this function checks if operand is a math operator switch(token) { case '+': return true; break; case '-': return true; break; case '*': return true; break; case '/': return true; break; case '%': return true; break; case '^': return true; break; case '$': return true; break; default: return false; break; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Want to convert & evaluate multi digit, decimal, and negative numbers? Click here!
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Want to evaluate a postfix expression? Click here for sample code.
Once compiled, you should get this as your output
(Note: the code was compile three separate times to display different output)
====== RUN 1 ======
==== Infix to Postfix Conversion ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Infix Equation: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
Please enter an infix expression: ((a+b)+c)/(d^e)
The Infix expression = ((a+b)+c)/(d^e)
The Postfix expression = ab+c+de^/====== RUN 2 ======
==== Infix to Postfix Conversion ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Infix Equation: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
Please enter an infix expression: (3*5)+(7^6)
The Infix expression = (3*5)+(7^6)
The Postfix expression = 35*76^+====== RUN 3 ======
==== Infix to Postfix Conversion ====
Math Operators:
+ || Addition
- || Subtraction
* || Multiplication
/ || Division
% || Modulus
^ || Power
$ || Square RootSample Infix Equation: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
Please enter an infix expression: (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
The Infix expression = (((4^5)*14)/($(23+2)-2))*(1%2)/(2*4)
The Postfix expression = 45^14*232+$2-/12%24*/*
C++ || 8 Different Ways To Reverse A String/Character Array In C++
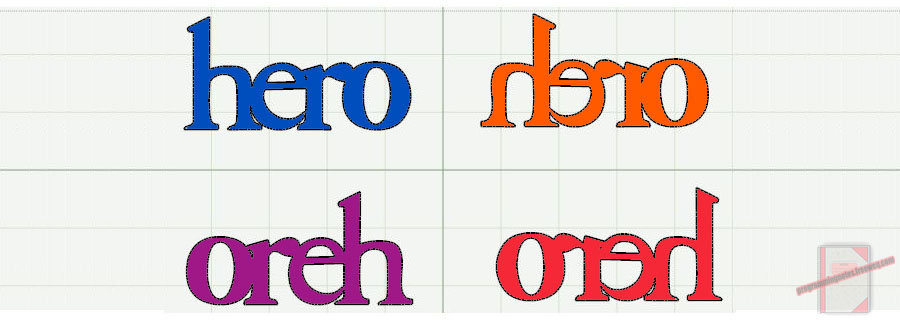
This page will consist of 8 different ways to reverse a character array, and a string literal (std::string).
REQUIRED KNOWLEDGE FOR THE PROGRAMS
Character Arrays
String Literals
Cin.getline - Use For Char Arrays
Getline - Use For std::string
Length
Strlen
Strcpy
While Loops
For Loops
Recursion - What is it?
#include < algorithm>
#include < stack>
The methods on this page will be broken up into sections. This page will list:
(3) methods which reverses string literals (std::string)
(4) methods which reverses character arrays
(1) method which utilizes the stack to "reverse" a character sequence
Some methods listed on this page demonstrates the use of reversing a character sequence without the use of strlen/length.
======= REVERSE AN STD::STRING =======
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
#include <iostream> #include <algorithm> #include <string> using namespace std; int main() { // declare variable string name=""; cout << "Enter your name: "; getline(cin,name); // built in C++ function to reverse an std::string reverse(name.begin(), name.end()); cout <<"\nYour name reversed is: " <<name << endl; return 0; }// http://programmingnotes.org/ |
SAMPLE OUTPUT
Enter your name: My Programming NotesYour name reversed is: setoN gnimmargorP yM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
#include <iostream> #include <string> using namespace std; // function prototypes void Reverse(string name); int main() { // declare variable string name=""; // get user data cout<<"Enter your name: "; getline(cin,name); cout<<"\nYour name reversed is: "; // function declaration Reverse(name); cout<<endl; return 0; }// end of main void Reverse(string name) { if(name == "") // the base case { return; } else // the recursive step { Reverse(name.substr(1)); cout<<name.at(0); } }// http://programmingnotes.org/ |
SAMPLE OUTPUT
Enter your name: My Programming NotesYour name reversed is: setoN gnimmargorP yM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
#include <iostream> #include <string> using namespace std; // function prototypes void Reverse(string name); int main() { // declare variable string name=""; // get user data cout<<"Enter your name: "; getline(cin,name); cout<<"\nYour name reversed is: "; // function declaration Reverse(name); cout<<endl; return 0; }// end of main void Reverse(string name) { // get the length of the string int nameLength = name.length()-1; while(nameLength >= 0) { cout<<name[nameLength]; --nameLength; } }// http://programmingnotes.org/ |
SAMPLE OUTPUT
Enter your name: My Programming NotesYour name reversed is: setoN gnimmargorP yM
======= REVERSE A CHARACTER ARRAY =======
The following will demonstrate (4) methods which reverses a character array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
#include <iostream> using namespace std; // function prototype void Reverse(char name[]); int main() { // declare variable char name[30]; // get user data cout<<"Enter your name: "; cin.getline(name, sizeof(name)); cout<<"\nYour name reversed is: "; // function declaration Reverse(name); cout<<endl; return 0; }// end of main void Reverse(char name[]) { if(*name=='\0') // the base case { return; } else // the recursive step { Reverse(name+1); cout<<*(name); } }// http://programmingnotes.org/ |
SAMPLE OUTPUT
Enter your name: My Programming NotesYour name reversed is: setoN gnimmargorP yM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
#include <iostream> using namespace std; // function prototypes void Reverse(char name[]); int main() { // declare variable char name[30]; // get user data cout<<"Enter your name: "; cin.getline(name, sizeof(name)); cout<<"\nYour name reversed is: "; // function declaration Reverse(name); cout<<endl; return 0; }// end of main void Reverse(char name[]) { int nameLength = 0; //get the length of array while(name[nameLength] != '\0') { ++nameLength; } //decrease the length of by 1 --nameLength; // display reversed string while(nameLength >= 0) { cout<<name[nameLength]; --nameLength; } }// http://programmingnotes.org/ |
SAMPLE OUTPUT
Enter your name: My Programming NotesYour name reversed is: setoN gnimmargorP yM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
#include <iostream> #include <cstring> using namespace std; // function prototypes void Reverse(char name[]); int main() { // declare variable char name[30]; // get user data cout<<"Enter your name: "; cin.getline(name, sizeof(name)); // function declaration Reverse(name); cout<<"\nYour name reversed is: "<< name<<endl; return 0; }// end of main void Reverse(char name[]) { // private variables int nameLength = 0; char copy[30]; strcpy(copy,name); //get lenght of array while(name[nameLength] != '\0') { ++nameLength; } //decrease the length of by 1 --nameLength; // reverse the array for(int x = 0; x <= nameLength; ++x) { // rearange the order of the two arrays name[nameLength - x] = copy[x]; } }// http://programmingnotes.org/ |
SAMPLE OUTPUT
Enter your name: My Programming NotesYour name reversed is: setoN gnimmargorP yM
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
#include <iostream> #include <cstring> using namespace std; // function prototypes void Reverse(char name[]); int main() { // declare variable char name[30]; // get user data cout<<"Enter your name: "; cin.getline(name, sizeof(name)); // function declaration Reverse(name); cout<<"\nYour name reversed is: "<< name<<endl; return 0; }// end of main void Reverse(char name[]) { // get the length of the current word in the array index int nameLength = strlen(name)-1; // increment thru each letter within the current char array index // reversing the order of the array for(int currentChar=0; currentChar < nameLength; --nameLength, ++currentChar) { // copy 1st letter in the array index into temp char temp = name[currentChar]; // copy last letter in the array index into the 1st array index name[currentChar] = name[nameLength]; // copy temp into last array index name[nameLength] = temp; } }// http://programmingnotes.org/ |
SAMPLE OUTPUT
Enter your name: My Programming NotesYour name reversed is: setoN gnimmargorP yM
======= REVERSE A CHARACTER SEQUENCE USING A STACK =======
The following will demonstrate (1) method which reverses a character sequence using the STL stack.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
#include <iostream> #include <stack> using namespace std; // function prototypes void Reverse(stack<char> &name); int main() { // using the internal system stack stack<char> name; char singleChar; cout<<"Enter your name: "; while(cin.get(singleChar) && singleChar != '\n') { name.push(singleChar); } cout<<"\nYour name reversed is: "; // function declaration Reverse(name); cout<<endl; return 0; }// end of main void Reverse(stack<char> &name) { while(!name.empty()) { cout << name.top(); name.pop(); } }// http://programmingnotes.org/ |
SAMPLE OUTPUT
Enter your name: My Programming NotesYour name reversed is: setoN gnimmargorP yM
C++ || Char Array – Palindrome Checker Using A Character Array, ToUpper, Strlen, Strcpy, & Strcmp
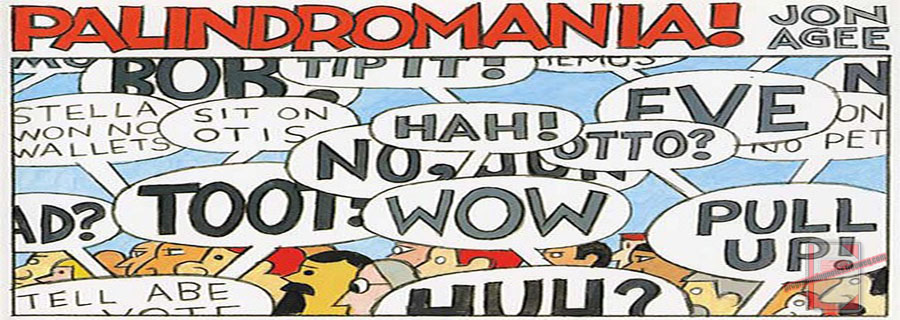
The following is a palindrome checking program, which demonstrates more use of char array’s, ToUpper, Strlen, & Strcmp.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Character Arrays
Cin.getline
How to convert text in a char array from lower to uppercase
How to reverse a character array
Palindrome - What is it?
Strlen
Strcpy
Strcmp
While Loops
For Loops
Constant Variables
Setw
Using a constant value, by default, this program first asks the user to enter 5 words and/or sentences that they want to compare for similarity. If the text which was entered into the program is a palindrome, the program will prompt a message to the user via cout. This program determines similarity by using the strcmp function, to compare two arrays together. This program also demonstrates how to reverse a character array, aswell as demonstrates how to convert all the text which was placed into the char array from lower to UPPERCASE.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 26, 2012 // Taken From: http://programmingnotes.org/ // File: palindrome.cpp // Description: Demonstrates a simple palindrome checker // ============================================================================ #include <iostream> #include <iomanip> #include <cstdlib> #include <cctype> #include <cstring> using namespace std; // constant value const int NUM_WORDS = 5; // function prototypes void ConvertAllToUpper(char wordsCopy[][30], char palindrome[][30]); void ReverseCharArray(char palindrome[][30]); void CheckIfPalindrome(char wordsCopy[][30], char palindrome[][30], char words[][30]); int main() { // declare variable // this is a 2-D char array, which by default, has // the ability to hold 5 names, each 30 characters long char words[NUM_WORDS][30]; char wordsCopy[NUM_WORDS][30]; char palindrome[NUM_WORDS][30]; // get data from user cout << "\tWelcome to the Palindrome Check System!\n"; cout << "\nPlease enter " << NUM_WORDS << " word(s) to check for similarity:\n"; for (int index = 0; index < NUM_WORDS; ++index) { cout << "\t#" << index + 1 << ": "; cin.getline(words[index], 30); } // copy the user input into the 'wordsCopy' & // 'palindrome' char array for (int index = 0; index < NUM_WORDS; ++index) { strcpy(wordsCopy[index], words[index]); strcpy(palindrome[index], words[index]); } // create a line seperator cout << endl; cout.fill('-'); cout << left << setw(30) << "" << right << setw(30) << "" << endl; // re-display the input to the screen cout << "\nThis is what you entered into the system:\n"; for (int index = 0; index < NUM_WORDS; ++index) { cout << "\tText #" << index + 1 << ": " << words[index] << endl; } // create a line seperator cout << endl; cout.fill('-'); cout << left << setw(30) << "" << right << setw(30) << "" << endl; // function declaration // convert all the text contained in the 2 arrays to // UPPERCASE ConvertAllToUpper(wordsCopy, palindrome); // function declaration // reverses all the text contained inside the char array // to determine if it is a palindrome ReverseCharArray(palindrome); // display the palindrome's to the screen cout << "\nHere are the palindrome's:\n"; // function declaration // checks to see if the text contained in the char // array is a palindrome or not CheckIfPalindrome(wordsCopy, palindrome, words); cin.get(); return 0; }// end of main void ConvertAllToUpper(char wordsCopy[][30], char palindrome[][30]) { int index = 0; // increment thru the current char array index while (index < NUM_WORDS) { // increment thru each letter within the current char array index for (unsigned currentChar = 0; currentChar < strlen(wordsCopy[index]); ++currentChar) { // checks each letter in the current array index // to see if its lower or UPPERCASE // if its lowercase, change to UPPERCASE if (islower(wordsCopy[index][currentChar])) { wordsCopy[index][currentChar] = toupper(wordsCopy[index][currentChar]); palindrome[index][currentChar] = toupper(palindrome[index][currentChar]); } } ++index; } }// end of ConvertAllToUpper void ReverseCharArray(char palindrome[][30]) { int index = 0; // increment thru the current char array index while (index < NUM_WORDS) { // get the length of the current word in the array index int wordLength = strlen(palindrome[index]) - 1; // increment thru each letter within the current char array index // reversing the order of the array for (int currentChar = 0; currentChar < wordLength; --wordLength, ++currentChar) { // copy 1st letter in the array index into temp char temp = palindrome[index][currentChar]; // copy last letter in the array index into the 1st array index palindrome[index][currentChar] = palindrome[index][wordLength]; // copy temp into last array index palindrome[index][wordLength] = temp; } ++index; } }// end of ReverseCharArray void CheckIfPalindrome(char wordsCopy[][30], char palindrome[][30], char words[][30]) { int palindromeCount = 0; // increment thru the current char array index for (int index = 0; index < NUM_WORDS; ++index) { // if the contents in the 'wordsCopy' & 'palindrome' // are the same, then the word is a palindrome if (strcmp(wordsCopy[index], palindrome[index]) == 0) { cout << "\tText #" << index + 1 << ": " << words[index] << " is a palindrome" << endl; ++palindromeCount; } } if (palindromeCount == 0) { cout << "There were no palindrome's found in the current list!\n"; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Click here to see how cin.getline works.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
(Note: The code was compiled 2 seperate times to demonstrate different output)
====== RUN 1 ======
Welcome to the Palindrome Check System!
Please enter 5 word(s) to check for similarity:
#1: SteP oN nO PEts
#2: My ProGramminG NoTeS
#3: RaTs liVE ON No eViL StaR
#4: ABLe wAs I ErE I sAw ElBa
#5: LiVE Non Evil------------------------------------------------------------
This is what you entered into the system:
Text #1: SteP oN nO PEts
Text #2: My ProGramminG NoTeS
Text #3: RaTs liVE ON No eViL StaR
Text #4: ABLe wAs I ErE I sAw ElBa
Text #5: LiVE Non Evil------------------------------------------------------------
Here are the palindrome's:
Text #1: SteP oN nO PEts is a palindrome
Text #3: RaTs liVE ON No eViL StaR is a palindrome
Text #4: ABLe wAs I ErE I sAw ElBa is a palindrome
Text #5: LiVE Non Evil is a palindrome====== RUN 2 ======
Welcome to the Palindrome Check System!
Please enter 5 word(s) to check for similarity:
#1: today Is Great
#2: Tomorrow is Foriegn
#3: Sunday Brunch
#4: Hello SkiPper
#5: Mayday Ship DowN!------------------------------------------------------------
This is what you entered into the system:
Text #1: today Is Great
Text #2: Tomorrow is Foriegn
Text #3: Sunday Brunch
Text #4: Hello SkiPper
Text #5: Mayday Ship DowN!------------------------------------------------------------
Here are the palindrome's:
There were no palindrome's found in the current list!
C++ || Char Array – Convert Text Contained In A Character Array From Lower To UPPERCASE
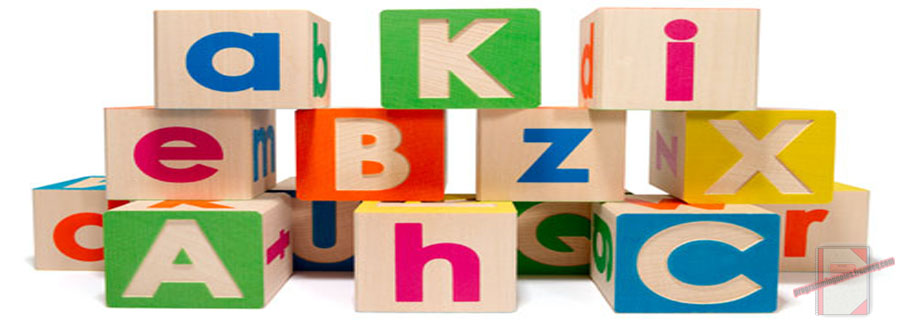
This program demonstrates how to switch text which is contained in a char array from lower to UPPERCASE. This program also demonstrates how to convert all of the text contained in a char array to lower/UPPERCASE.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Character Arrays
Cin.getline
Islower
Isupper
Tolower
Toupper
Strlen
While Loops
For Loops
Constant Variables
Setw
Using a constant integer value, this program first asks the user to enter in 3 lines of text they wish to convert from lower to UPPERCASE. Upon obtaining the information from the user, the program then converts all the text which was placed into the character array from lower to uppercase in the following order:
(1) Switches the text from lower to UPPERCASE
(2) Converts all the text to UPPERCASE
(3) Converts all the text to lowercase
After each conversion is complete, the program displays the updated information to the screen via cout.
NOTE: On some compilers, you may have to add #include < cstdlib>, #include < cctype>, and #include < cstring> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 |
#include <iostream> #include <iomanip> using namespace std; // constant value const int NUM_NAMES = 3; // function prototype void ConvertLowerToUpper(char names[][30]); void ConvertAllToUpper(char names[][30]); void ConvertAllToLower(char names[][30]); int main() { // declare variable // this is a 2-D char array, which by default, has // the ability to hold 3 names, each 30 characters long char names[NUM_NAMES][30]; // get data from user cout << "Please enter "<<NUM_NAMES<<" line(s) of text you wish to convert from lower to UPPERCASE:nt"; for(int index=0; index < NUM_NAMES; ++index) { cout<<"#"<< index+1 <<": "; cin.getline(names[index],30); cout<< "t"; } // create a line seperator cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // re-display the input to the screen cout << "nThis is what you entered into the system:nt"; for(int index=0; index < NUM_NAMES; ++index) { cout<<"Text #"<< index+1 <<": "<<names[index]<<endl; cout<< "t"; } // create a line seperator cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // display the switched lower to UPPERCASE text to the user cout << "nThis is the information switched from lower to UPPERCASE:nt"; // function declaration ConvertLowerToUpper(names); for(int index=0; index < NUM_NAMES; ++index) { cout<<"Text #"<< index+1 <<": "<<names[index]<<endl; cout<< "t"; } // create a line seperator cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // display the 'all UPPERCASE' converted text to the user cout << "nThis is the information converted to all UPPERCASE:nt"; // function declaration ConvertAllToUpper(names); for(int index=0; index < NUM_NAMES; ++index) { cout<<"Text #"<< index+1 <<": "<<names[index]<<endl; cout<< "t"; } // create a line seperator cout<<endl; cout.fill('-'); cout<<left<<setw(30)<<""<<right<<setw(30)<<""<<endl; // display the 'all lowercase' converted text to the user cout << "nThis is the information converted to all lowercase:nt"; // function declaration ConvertAllToLower(names); for(int index=0; index < NUM_NAMES; ++index) { cout<<"Text #"<< index+1 <<": "<<names[index]<<endl; cout<< "t"; } return 0; }// end of main void ConvertLowerToUpper(char names[][30]) { int index=0; // increment thru the current char array index while(index < NUM_NAMES) { // increment thru each letter within the current char array index for(int currentChar=0; currentChar < strlen(names[index]); ++currentChar) { // checks each letter in the current array index // to see if its lower or UPPERCASE // if its lowercase, change to UPPERCASE if (islower(names[index][currentChar])) { names[index][currentChar] = toupper(names[index][currentChar]); } else // if its UPPERCASE, change to lowercase { names[index][currentChar] = tolower(names[index][currentChar]); } } ++index; } }// end of ConvertLowerToUpper void ConvertAllToUpper(char names[][30]) { int index=0; // increment thru the current char array index while(index < NUM_NAMES) { // increment thru each letter within the current char array index for(int currentChar=0; currentChar < strlen(names[index]); ++currentChar) { // checks each letter in the current array index // to see if its lower or UPPERCASE // if its lowercase, change to UPPERCASE if (islower(names[index][currentChar])) { names[index][currentChar] = toupper(names[index][currentChar]); } } ++index; } }// end of ConvertAllToUpper void ConvertAllToLower(char names[][30]) { int index=0; // increment thru the current char array index while(index < NUM_NAMES) { // increment thru each letter within the current char array index for(int currentChar=0; currentChar < strlen(names[index]); ++currentChar) { // checks each letter in the current array index // to see if its lower or UPPERCASE // if its UPPERCASE, change to lowercase if (isupper(names[index][currentChar])) { names[index][currentChar] = tolower(names[index][currentChar]); } } ++index; } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
Click here to see how cin.getline works.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Please enter 3 line(s) of text you wish to convert from lower to UPPERCASE:
#1: I StriKe hiM a heAVy bloW.
#2: When cAn the neRve ShinE?
#3: My Programming Notes.------------------------------------------------------------
This is what you entered into the system:
Text #1: I StriKe hiM a heAVy bloW.
Text #2: When cAn the neRve ShinE?
Text #3: My Programming Notes.------------------------------------------------------------
This is the information switched from lower to UPPERCASE:
Text #1: i sTRIkE HIm A HEavY BLOw.
Text #2: wHEN CaN THE NErVE sHINe?
Text #3: mY pROGRAMMING nOTES.------------------------------------------------------------
This is the information converted to all UPPERCASE:
Text #1: I STRIKE HIM A HEAVY BLOW.
Text #2: WHEN CAN THE NERVE SHINE?
Text #3: MY PROGRAMMING NOTES.------------------------------------------------------------
This is the information converted to all lowercase:
Text #1: i strike him a heavy blow.
Text #2: when can the nerve shine?
Text #3: my programming notes.
C++ || Snippet – How To Do Simple Math Using Integer Arrays
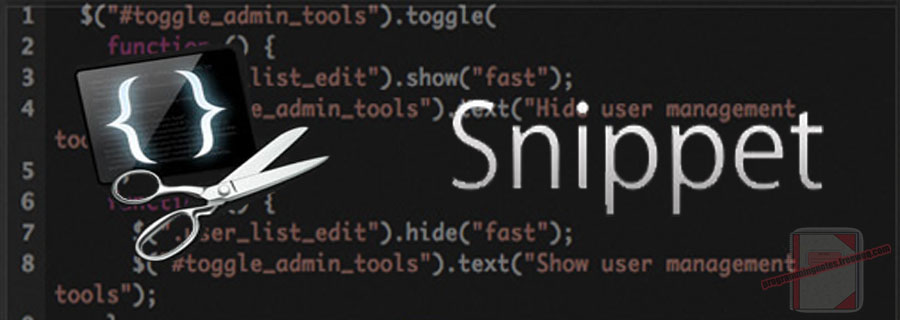
This page will consist of simple programs which demonstrate the process of doing simple math with numbers that are stored in an integer array.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
Integer Arrays
For Loops
Assignment Operators - Simple Math Operations
Setw
Note: In all of the examples on this page, a random number generator was used to place numbers into the array. If you do not know how to obtain data from the user, or if you do not know how to insert data into an array, click here for a demonstration.
===== ADDITION =====
The first code snippet will demonstrate how to add numbers together which are stored in an integer array. This example uses the “+=” assignment operator.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
#include <iostream> #include <iomanip> // used for setw #include <ctime> // used for srand & rand using namespace std; // const int allocating space for the array const int NUM_INTS = 12; int main() { // declare variables int arry[NUM_INTS]; // array is initialized using a const variable int totalSum =0; srand(time(NULL)); // place random numbers into the array for(int i = 0; i < NUM_INTS; i++) { arry[i] =rand()%100+1; } cout << "Original array values" << endl; // Display the original array values for(int i = 0; i < NUM_INTS; i++) { cout << setw(4) << arry[i]; } // creates a line seperator cout << "n--------------------------------------------------------"; cout<<"nThe sum of the items in the array is: "; // Find the sum of the values in the array for(int i = 0; i < NUM_INTS; i++) { // the code below literally means // totalSum = totalSum + arry[i] totalSum += arry[i]; } // after the calculations are complete, display the total to the user cout<< totalSum <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
SAMPLE OUTPUT
Original array values
64 85 44 31 35 2 94 67 12 80 97 10
--------------------------------------------------------
The sum of the items in the array is: 621
===== SUBTRACTION =====
The second code snippet will demonstrate how to subtract numbers which are stored in an integer array. This example uses the “-=” assignment operator.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
#include <iostream> #include <iomanip> // used for setw #include <ctime> // used for srand & rand using namespace std; // const int allocating space for the array const int NUM_INTS = 12; int main() { // declare variables int arry[NUM_INTS]; // array is initialized using a const variable int totalDiffetence =0; srand(time(NULL)); // place random numbers into the array for(int i = 0; i < NUM_INTS; i++) { arry[i] =rand()%100+1; } cout << "Original array values" << endl; // Display the original array values for(int i = 0; i < NUM_INTS; i++) { cout << setw(4) << arry[i]; } // creates a line seperator cout << "n--------------------------------------------------------"; cout<<"nThe difference of the items in the array is: "; // Find the difference of the values in the array for(int i = 0; i < NUM_INTS; i++) { // the code below literally means // totalDiffetence = totalDiffetence - arry[i] totalDiffetence -= arry[i]; } // after the calculations are complete, display the total to the user cout<< totalDiffetence <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
SAMPLE OUTPUT
Original array values
10 43 77 10 2 17 87 67 6 95 57 18
--------------------------------------------------------
The difference of the items in the array is: -489
===== MULTIPLICATION =====
The third code snippet will demonstrate how to multiply numbers which are stored in an integer array. This example uses the “*=” assignment operator.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
#include <iostream> #include <iomanip> // used for setw #include <ctime> // used for srand & rand using namespace std; // const int allocating space for the array const int NUM_INTS = 12; int main() { // declare variables int arry[NUM_INTS]; // array is initialized using a const variable int totalProduct =1; srand(time(NULL)); // place random numbers into the array for(int i = 0; i < NUM_INTS; i++) { arry[i] =rand()%100+1; } cout << "Original array values" << endl; // Display the original array values for(int i = 0; i < NUM_INTS; i++) { cout << setw(4) << arry[i]; } // creates a line seperator cout << "n--------------------------------------------------------"; cout<<"nThe product of the items in the array is: "; // Find the product of the values in the array for(int i = 0; i < NUM_INTS; i++) { // the code below literally means // totalProduct = totalProduct * arry[i]; totalProduct *= arry[i]; } // after the calculations are complete, display the total to the user cout<< totalProduct <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
SAMPLE OUTPUT
Original array values
33 36 52 28 4 99 97 17 42 81 83 33
--------------------------------------------------------
The product of the items in the array is: 1803759104
===== DIVISION =====
The fourth code snippet will demonstrate how to divide numbers which are stored in an integer array. This example uses the “/=” assignment operator.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
#include <iostream> #include <iomanip> // used for setw #include <ctime> // used for srand & rand using namespace std; // const int allocating space for the array const int NUM_INTS = 12; int main() { // declare variables int arry[NUM_INTS]; // array is initialized using a const variable float totalQuotient =1; // need to save the variable as a float, not an int srand(time(NULL)); // place random numbers into the array for(int i = 0; i < NUM_INTS; i++) { arry[i] =rand()%100+1; } cout << "Original array values" << endl; // Display the original array values for(int i = 0; i < NUM_INTS; i++) { cout << setw(4) << arry[i]; } // creates a line seperator cout << "n--------------------------------------------------------"; cout<<"nThe quotient of the items in the array is: "; // Find the quotient of the values in the array for(int i = 0; i < NUM_INTS; i++) { // the code below literally means // totalQuotient = totalQuotient / arry[i]; totalQuotient /= arry[i]; } // after the calculations are complete, display the total to the user cout<< totalQuotient <<endl; return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
SAMPLE OUTPUT
Original array values
75 38 59 14 53 42 29 88 92 27 69 16
--------------------------------------------------------
The quotient of the items in the array is: 2.72677e-020
C++ || Snippet – How To Find The Highest & Lowest Numbers Contained In An Integer Array
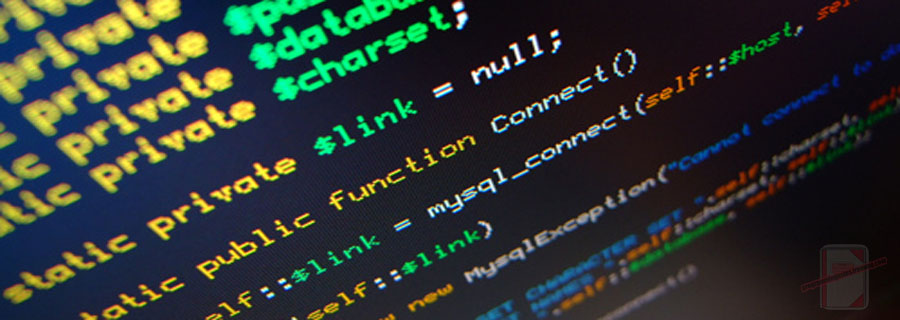
This page will consist of a simple demonstration for finding the highest and lowest numbers contained in an integer array.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
Finding the highest/lowest values in an array can be found in one or two ways. The first way would be via a sort, which would render the highest/lowest numbers contained in the array because the values would be sorted in order from highest to lowest. But a sort may not always be practical, especially when you want to keep the array values in the same order that they originally came in.
The second method of finding the highest/lowest values is by traversing through the array, checking each value it contains one by one to determine if the current number which is being compared truly is a target value or not. That method will be displayed below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
// ============================================================================ // Author: Kenneth Perkins // Date: Feb 12, 2012 // Taken From: http://programmingnotes.org/ // File: arrayMinMax.cpp // Description: Determines the highest and lowest value in an array // ============================================================================ #include <iostream> #include <iomanip> #include <ctime> using namespace std; const int NUM_INTS = 14; int main() { // declare variables int arry[NUM_INTS]; // array is initialized using a const variable srand(time(NULL)); // place random numbers into the array for (int x = 0; x < NUM_INTS; ++x) { arry[x] = rand() % 100 + 1; } cout << "Original array values" << endl; // Output the original array values for (int x = 0; x < NUM_INTS; ++x) { cout << setw(4) << arry[x]; } // creates a line separator cout << "\n--------------------------------------------------------"; // use a for loop to go thru the array checking to see the highest/lowest element cout << "\nThese are the highest and lowest array values: "; // set the lowest/highest to the first array item (if any) int lowestScore = NUM_INTS > 0 ? arry[0] : 0; int highestScore = lowestScore; for (int index = 1; index < NUM_INTS; ++index) { // if current score in the array is bigger than the current 'highestScore' // element, then set 'highestScore' equal to the current array element if (arry[index] > highestScore) { highestScore = arry[index]; } // if current score in the array is smaller than the current 'lowestScore' // element, then set 'lowestScore' equal to the current array element if (arry[index] < lowestScore) { lowestScore = arry[index]; } }// end for loop // display the results to the user cout << "\n\tHighest: " << highestScore; cout << "\n\tLowest: " << lowestScore; cout << endl; cin.get(); return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Original array values
10 14 1 94 29 25 7 95 11 17 6 71 100 59
--------------------------------------------------------
These are the highest and lowest array values:
Highest: 100
Lowest: 1