Tag Archives: get ip address
C# || How To Get The Computer & User Client IP Address Using C#
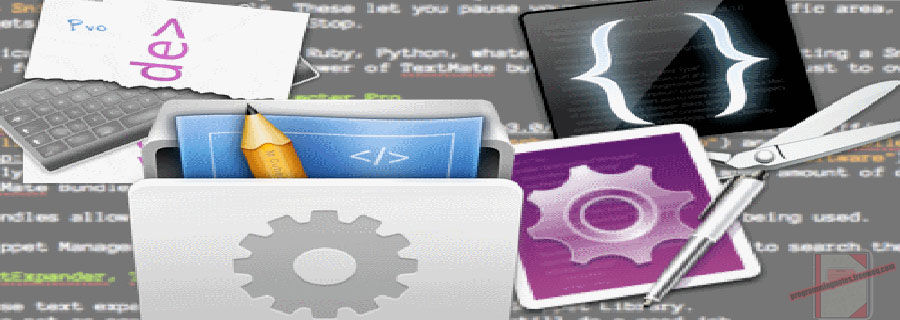
The following is a module with functions which demonstrates how to get the computers and user client request IPv4 IP address using C#.
The function demonstrated on this page returns the IPv4 address of the calling user. When under a web environment, it returns the clients System.Web.HttpContext.Current.Request IP address, otherwise it returns the IP address of the local machine (i.e: server) if there is no request.
The following function uses System.Web to determine the IP address of the client.
Note: To use the function in this module, make sure you have a reference to ‘System.Web‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add Reference‘, then type ‘System.Web‘ in the search box, and add the reference titled System.Web in the results Tab.
1. Get IP Address
The example below demonstrates the use of ‘Utils.Methods.GetIPv4Address‘ to get the IPv4 address of the calling user.
1 2 3 4 5 6 7 8 9 10 11 |
// Get IP Address // Get IP address of the current user var ipAddress = Utils.Methods.GetIPv4Address(); Console.WriteLine($"IP Address: {ipAddress}"); // example output: /* IP Address: 192.168.0.5 */ |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; namespace Utils { public static class Methods { /// <summary> /// Returns the IPv4 address of the calling user. When under a web /// environment, returns the <see cref="System.Web.HttpRequest"/> /// IP address, otherwise returns the IP address of the local machine /// </summary> /// <returns>The IPv4 address of the calling user</returns> public static string GetIPv4Address() { var ipAddress = string.Empty; // Get client ip address if (System.Web.HttpContext.Current != null && System.Web.HttpContext.Current.Request != null) { // Get client ip address using ServerVariables var request = System.Web.HttpContext.Current.Request; ipAddress = request.ServerVariables["HTTP_X_FORWARDED_FOR"]; if (!string.IsNullOrEmpty(ipAddress)) { var addresses = ipAddress.Split(",".ToCharArray(), StringSplitOptions.RemoveEmptyEntries); if (addresses.Length > 0) { ipAddress = addresses[0]; } } if (string.IsNullOrEmpty(ipAddress)) { ipAddress = request.ServerVariables["REMOTE_ADDR"]; } // Get client ip address using UserHostAddress if (string.IsNullOrEmpty(ipAddress)) { var clientIPA = GetNetworkAddress(request.UserHostAddress); if (clientIPA != null) { ipAddress = clientIPA.ToString(); } } } // Get local machine ip address if (string.IsNullOrEmpty(ipAddress)) { var loacalIPA = GetNetworkAddress(System.Net.Dns.GetHostName()); if (loacalIPA != null) { ipAddress = loacalIPA.ToString(); } } return ipAddress; } private static System.Net.IPAddress GetNetworkAddress(string addresses) { return System.Net.Dns.GetHostAddresses(addresses) .FirstOrDefault(x => x.AddressFamily == System.Net.Sockets.AddressFamily.InterNetwork); } } }// http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 13, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; public class Program { static void Main(string[] args) { try { // Get IP address of the current user var ipAddress = Utils.Methods.GetIPv4Address(); Display($"IP Address: {ipAddress}"); } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Get The Computer & User Client IP Address Using VB.NET
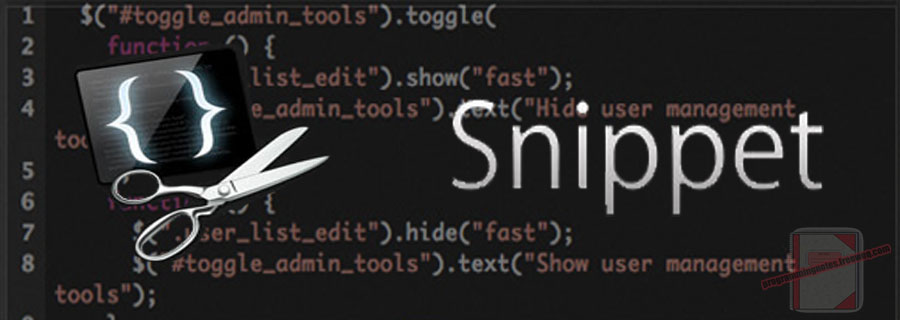
The following is a module with functions which demonstrates how to get the computers and user client request IPv4 IP address using VB.NET.
The function demonstrated on this page returns the IPv4 address of the calling user. When under a web environment, it returns the clients System.Web.HttpContext.Current.Request IP address, otherwise it returns the IP address of the local machine (i.e: server) if there is no request.
The following function uses System.Web to determine the IP address of the client.
Note: To use the function in this module, make sure you have a reference to ‘System.Web‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add Reference‘, then type ‘System.Web‘ in the search box, and add the reference titled System.Web in the results Tab.
1. Get IP Address
The example below demonstrates the use of ‘Utils.GetIPv4Address‘ to get the IPv4 address of the calling user.
1 2 3 4 5 6 7 8 9 |
' Get IP Address ' Get IP address of the current user Dim ipAddress = Utils.GetIPv4Address Debug.Print($"IP Address: {ipAddress}") ' example output: ' IP Address: 192.168.0.5 |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 29, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Returns the IPv4 address of the calling user. When under a web ''' environment, returns the <see cref="System.Web.HttpRequest"/> ''' IP address, otherwise returns the IP address of the local machine ''' </summary> ''' <returns>The IPv4 address of the calling user</returns> Public Function GetIPv4Address() As String Dim ipAddress = String.Empty ' Get client ip address If System.Web.HttpContext.Current IsNot Nothing _ AndAlso System.Web.HttpContext.Current.Request IsNot Nothing Then ' Get client ip address using ServerVariables Dim request = System.Web.HttpContext.Current.Request ipAddress = request.ServerVariables("HTTP_X_FORWARDED_FOR") If Not String.IsNullOrEmpty(ipAddress) Then Dim addresses = ipAddress.Split(",".ToCharArray, StringSplitOptions.RemoveEmptyEntries) If addresses.Length > 0 Then ipAddress = addresses(0) End If End If If String.IsNullOrEmpty(ipAddress) Then ipAddress = request.ServerVariables("REMOTE_ADDR") End If ' Get client ip address using UserHostAddress If String.IsNullOrEmpty(ipAddress) Then Dim clientIPA = GetNetworkAddress(request.UserHostAddress) If clientIPA IsNot Nothing Then ipAddress = clientIPA.ToString End If End If End If ' Get local machine ip address If String.IsNullOrEmpty(ipAddress) Then Dim loacalIPA = GetNetworkAddress(System.Net.Dns.GetHostName) If loacalIPA IsNot Nothing Then ipAddress = loacalIPA.ToString End If End If Return ipAddress End Function Private Function GetNetworkAddress(addresses As String) As System.Net.IPAddress Return System.Net.Dns.GetHostAddresses(addresses) _ .FirstOrDefault(Function(x) x.AddressFamily = System.Net.Sockets.AddressFamily.InterNetwork) End Function End Module End Namespace ' http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 29, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Sub Main(args As String()) Try ' Get IP address of the current user Dim ipAddress = Utils.GetIPv4Address Display($"IP Address: {ipAddress}") Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.