Tag Archives: string
C++ || How To Trim & Remove The Leading & Trailing Whitespace From A String Using C++
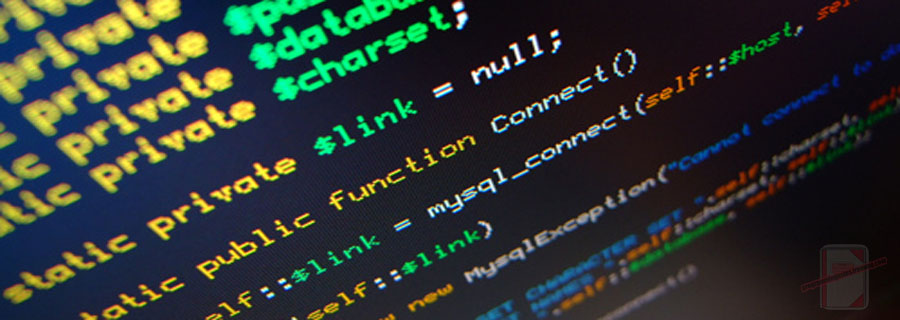
The following is a module with functions which demonstrates how to trim and remove the leading and trailing whitespace from a string using C++.
1. Trim
The example below demonstrates the use of ‘Utils::trim‘ to remove all the leading and trailing whitespace characters from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Trim // Declare string std::string original = " <-Kenneth-> "; // Trim the string auto trim = Utils::trim(original); // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Trim: '" << trim << "'" << std::endl; // expected output: /* Original: ' <-Kenneth-> ' Trim: '<-Kenneth->' */ |
2. Trim Start
The example below demonstrates the use of ‘Utils::trimStart‘ to remove all leading whitespace characters from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Trim Start // Declare string std::string original = " <-Kenneth-> "; // Trim the start of string auto ltrim = Utils::trimStart(original); // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Trim Start: '" << ltrim << "'" << std::endl; // expected output: /* Original: ' <-Kenneth-> ' Trim Start: '<-Kenneth-> ' */ |
3. Trim End
The example below demonstrates the use of ‘Utils::trimEnd‘ to remove all trailing whitespace characters from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Trim End // Declare string std::string original = " <-Kenneth-> "; // Trim the end of string auto rtrim = Utils::trimEnd(original); // Display result std::cout << "Original: '" << original << "'" << std::endl; std::cout << "Trim End: '" << rtrim << "'" << std::endl; // expected output: /* Original: ' <-Kenneth-> ' Trim End: ' <-Kenneth->' */ |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 10, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> #include <algorithm> #include <cctype> namespace Utils { /** * FUNCTION: trimEnd * USE: Returns a new string with all trailing whitespace characters removed * @param source: The source string * @return: A new string with all the trailing whitespace characters removed */ std::string trimEnd(std::string source) { source.erase(std::find_if(source.rbegin(), source.rend(), [](char c) { return !std::isspace(static_cast<unsigned char>(c)); }).base(), source.end()); return source; } /** * FUNCTION: trimStart * USE: Returns a new string with all leading whitespace characters removed * @param source: The source string * @return: A new string with all the leading whitespace characters removed */ std::string trimStart(std::string source) { source.erase(source.begin(), std::find_if(source.begin(), source.end(), [](char c) { return !std::isspace(static_cast<unsigned char>(c)); })); return source; } /** * FUNCTION: trim * USE: Returns a new string with all the leading and trailing whitespace * characters removed * @param source: The source string * @return: A new string with all the leading and trailing whitespace * characters removed */ std::string trim(std::string source) { return trimEnd(trimStart(source)); } }// http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 10, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare string std::string original = " <-Kenneth-> "; // Trim the string auto trim = Utils::trim(original); // Display result display("Original: '" + original + "'"); display("Trim: '" + trim + "'"); display(""); // Trim the start of string auto ltrim = Utils::trimStart(original); // Display result display("Original: '" + original + "'"); display("Trim Start: '" + ltrim + "'"); display(""); // Trim the end of string auto rtrim = Utils::trimEnd(original); // Display result display("Original: '" + original + "'"); display("Trim End: '" + rtrim + "'"); } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
C++ || How To Check If A String Starts & Ends With A Certain String Using C++
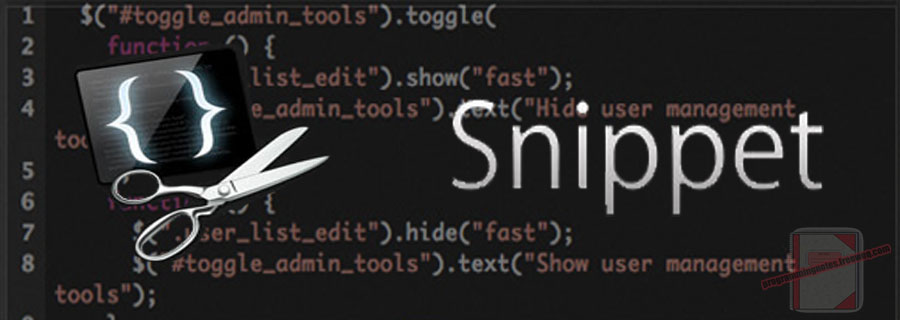
The following is a module with functions which demonstrates how to determine if a string starts and ends with a certain substring using C++.
1. Starts With
The example below demonstrates the use of ‘Utils::startsWith‘ to determine whether the beginning of a string instance matches a specified string value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Starts With // Declare values std::string values[] = { "This is a string.", "Hello!", "Nothing.", "Yes.", "randomize" }; // Declare search std::string search = "Th"; // Check if items starts with search value for (const auto& value : values) { std::cout << "'" << value << "' starts with '" << search << "': " << (Utils::startsWith(value, search) ? "True" : "False") << std::endl; } // example output: /* 'This is a string.' starts with 'Th': True 'Hello!' starts with 'Th': False 'Nothing.' starts with 'Th': False 'Yes.' starts with 'Th': False 'randomize' starts with 'Th': False */ |
2. Ends With
The example below demonstrates the use of ‘Utils::endsWith‘ to determine whether the end of a string instance matches a specified string value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Ends With // Declare values std::string values[] = { "This is a string.", "Hello!", "Nothing.", "Yes.", "randomize" }; // Declare search std::string search = "."; // Check if items ends with search value for (const auto& value : values) { std::cout << "'" << value << "' ends with '" << search << "': " << (Utils::endsWith(value, search) ? "True" : "False") << std::endl; } // example output: /* 'This is a string.' ends with '.': True 'Hello!' ends with '.': False 'Nothing.' ends with '.': True 'Yes.' ends with '.': True 'randomize' ends with '.': False */ |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 9, 2020 // Taken From: http://programmingnotes.org/ // File: Utils.h // Description: Handles general utility functions // ============================================================================ #pragma once #include <string> namespace Utils { /** * FUNCTION: startsWith * USE: Determines whether the beginning of a string instance matches * a specified string value * @param source: The source string * @param value: The string to compare * @return: True if 'value' matches the beginning of 'source', * False otherwise */ bool startsWith(const std::string& source, const std::string& value) { return source.size() >= value.size() && source.compare(0, value.size(), value) == 0; } /** * FUNCTION: endsWith * USE: Determines whether the end of a string instance matches * a specified string value * @param source: The source string * @param value: The string to compare to the substring at the end * @return: True if 'value' matches the end of 'source', * False otherwise */ bool endsWith(const std::string& source, const std::string& value) { return source.size() >= value.size() && source.compare(source.size() - value.size(), value.size(), value) == 0; } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
// ============================================================================ // Author: Kenneth Perkins // Date: Dec 9, 2020 // Taken From: http://programmingnotes.org/ // File: program.cpp // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ #include <iostream> #include <string> #include <exception> #include "Utils.h" void display(const std::string& message); int main() { try { // Declare values std::string values[] = { "This is a string.", "Hello!", "Nothing.", "Yes.", "randomize" }; // Declare search std::string search = "Th"; // Check if items starts with search value for (const auto& value : values) { std::cout << "'" << value << "' starts with '" << search << "': " << (Utils::startsWith(value, search) ? "True" : "False") << std::endl; } display(""); // Declare values std::string values2[] = { "This is a string.", "Hello!", "Nothing.", "Yes.", "randomize" }; // Declare search std::string search2 = "."; // Check if items ends with search value for (const auto& value : values2) { std::cout << "'" << value << "' ends with '" << search2 << "': " << (Utils::endsWith(value, search2) ? "True" : "False") << std::endl; } } catch (std::exception& e) { display("\nAn error occurred: " + std::string(e.what())); } std::cin.get(); return 0; } void display(const std::string& message) { std::cout << message << std::endl; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Python || Using If Statements & String Variables
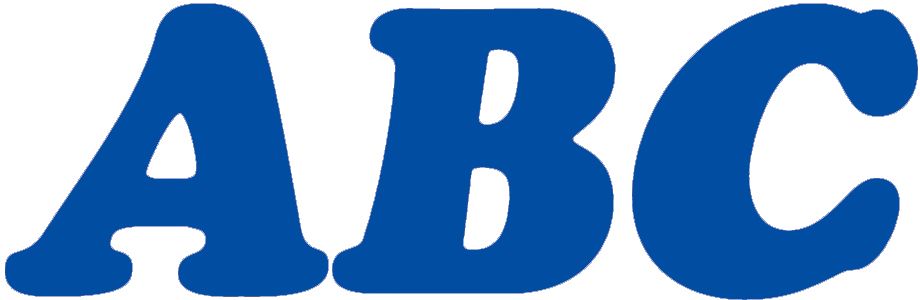
As previously mentioned, you can use “int” and “float” to represent numbers, but what if you want to store letters? Strings help you do that.
==== SINGLE CHAR ====
This example will demonstrate a simple program using strings, which checks to see if the user entered the correctly predefined letter.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: char.py # Description: Demonstrates using char variables # ============================================================================= def main(): # declare variables userInput = "" # this variable is a string letter = '' # this holds the individual character # get data from user userInput = input("Please try to guess the letter I am thinking of: ") # we only want to check the first character in the string, # so we get the letter at index zero and save it into its # own variable letter = userInput[0] # use an if statement to check equality. if ((letter == 'a') or (letter == 'A')): print("\nYou have guessed correctly!") else: print("\nSorry, that was not the correct letter I was thinking of..") if __name__ == "__main__": main() # http://programmingnotes.org/ |
Notice in line 11 I declare the string data type, naming it “userInput.” I also initialized it as an empty variable. In line 23 I used an “If/Else Statement” to determine if the user entered value matches the predefined letter within the program. I also used the “OR” operator in line 23 to determine if the letter the user entered into the program was lower or uppercase. Try compiling the program simply using this
if (letter == 'a')
as your if statement, and notice the difference.
The resulting code should give this as output
Please try to guess the letter I am thinking of: A
You have guessed correctly!
==== CHECK IF LETTER IS UPPER CASE ====
This example is similar to the previous one, and will check if a user entered letter is uppercase.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: uppercase.py # Description: Demonstrates checking if a char variable is uppercase # ============================================================================= def main(): # declare variables userInput = "" # this variable is a string letter = '' # this holds the individual character # get data from user userInput = input("Please enter an UPPERCASE letter: ") # get the 1st character in the string letter = userInput[0] # check to see if entered data falls between uppercase values if ((letter >= 'A') and (letter <= 'Z')): print("\n'%c' is an is an uppercase letter!" % (letter)) else: print("\nSorry, '%c' is not an uppercase letter.." % (letter)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
Notice in line 21, an If statement was used, which checks to see if the user entered data falls between letter A and letter Z. We did that by using the “AND” operator. So that IF statement is basically saying (in plain english)
IF ('letter' is equal to or greater than 'A') AND ('letter' is equal to or less than 'Z')
THEN it is an uppercase letter
The resulting code should give this as output
Please enter an UPPERCASE letter: g
Sorry, 'g' is not an uppercase letter..
==== CHECK IF LETTER IS A VOWEL ====
This example will utilize more if statements, checking to see if the user entered letter is a vowel or not. This will be very similar to the previous example, utilizing the OR operator once again.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: vowel.py # Description: Demonstrates checking if a char variable is a vowel # ============================================================================= def main(): # declare variables userInput = "" # this variable is a string letter = '' # this holds the individual character # get data from user userInput = input("Please enter a vowel: ") # get the 1st character in the string letter = userInput[0] # check to see if entered data is A,E,I,O,U,Y if ((letter == 'a')or(letter == 'A')or(letter == 'e')or (letter == 'E')or(letter == 'i')or(letter == 'I')or (letter == 'o')or(letter == 'O')or(letter == 'u')or (letter == 'U')or(letter == 'y')or(letter == 'Y')): print("\nCorrect, '%c' is a vowel!" % (letter)) else: print("\nSorry, '%c' is not a vowel.." % (letter)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
This program should be very straight forward, and its basically checking to see if the user entered data is the letter A, E, I, O, U or Y.
The resulting code should give the following output
Please enter a vowel: K
Sorry, 'K' is not a vowel..
==== HELLO WORLD v2 ====
This last example will demonstrate using the string data type to print the line “Hello World!” to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
# ============================================================================= # Author: Kenneth Perkins # Date: May 29, 2013 # Updated: Feb 16, 2021 # Taken From: http://programmingnotes.org/ # File: string.py # Description: Demonstrates using string variables # ============================================================================= def main(): # declare variables userInput = "" # this variable is a string # get data from user userInput = input("Please enter a sentence: ") # display string print("\nYou Entered: '%s'" % (userInput)) if __name__ == "__main__": main() # http://programmingnotes.org/ |
The following is similar to the other examples listed on this page, except we display the entire string instead of just simply the first character.
The resulting code should give following output
Please enter a sentence: Hello World!
You Entered: 'Hello World!'
C++ || Snippet – How To Convert A Decimal Number Into Binary
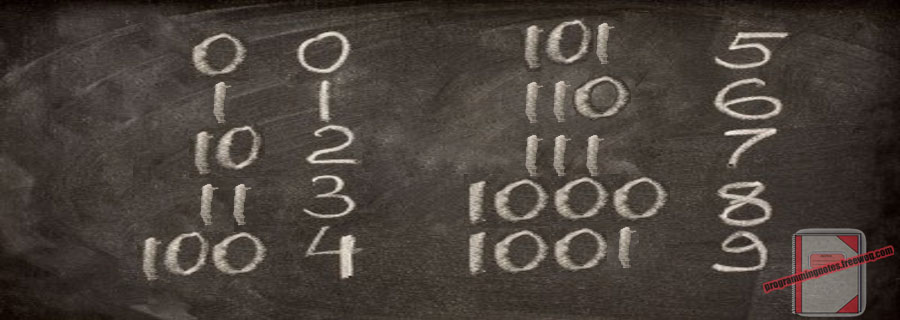
This page will demonstrate how to convert a decimal number (i.e a whole number) into its binary equivalent. So for example, if the decimal number of 26 was entered into the program, it would display the converted binary value of 11010.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
How To Count In Binary
The "Long" Datatype - What Is It?
While Loops
Online Binary to Decimal Converter - Verify For Correct Results
How To Reverse A String
If you are looking for sample code which converts binary to decimal, check back here soon!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
#include <iostream> #include <string> #include <algorithm> using namespace std; // function prototype string DecToBin(long long decNum); int main() { // declare variables long long decNum = 0; string binaryNum=""; // use a string instead of an int to avoid // overflow, because binary numbers can grow large quick cout<<"Please enter an integer value: "; cin >> decNum; if(decNum < 0) { binaryNum = "-"; } // call function to convert decimal to binary binaryNum += DecToBin(decNum); // display data to user cout<<"nThe integer value of "<<decNum<<" = "<<binaryNum<<" in binary"<<endl; return 0; } string DecToBin(long long decNum) { string binary = ""; // use this string to save the binary number if(decNum < 0) // if input is a neg number, make it positive { decNum *= -1; } // converts decimal to binary using division and modulus while(decNum > 0) { binary += (decNum % 2)+'0'; // convert int to char decNum /= 2; } // reverse the string reverse(binary.begin(), binary.end()); return binary; }// http://programmingnotes.org/ |
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Note: The code was compiled 3 separate times to display different output
====== RUN 1 ======
Please enter an integer value: 1987
The integer value of 1987 = 11111000011 in binary
====== RUN 2 ======
Please enter an integer value: -26
The integer value of -26 = -11010 in binary
====== RUN 3 ======
Please enter an integer value: 12345678910
The integer value of 12345678910 = 1011011111110111000001110000111110 in binary
Java || Snippet – How To Convert A Decimal Number Into Binary
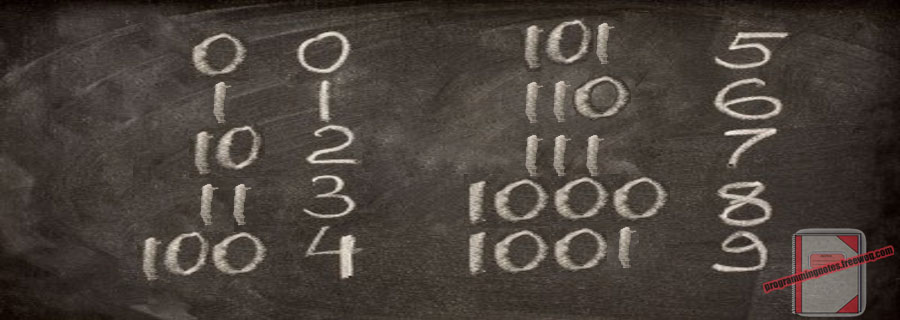
This page will demonstrate how to convert a decimal number (i.e a whole number) into its binary equivalent. So for example, if the decimal number of 25 was entered into the program, it would display the converted binary value of 11001.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
How To Count In Binary
The "Long" Datatype - What Is It?
Methods (A.K.A "Functions") - What Are They?
While Loops
Online Binary to Decimal Converter - Verify For Correct Results
If you are looking for sample code which converts binary to decimal, check back here soon!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
import java.util.Scanner; public class DecimalToBinary { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables long decNum = 0; String binaryNum = ""; // use a string instead of an int to avoid // overflow, because binary numbers can grow large quick // display message to screen System.out.println("Welcome to My Programming Notes' Java Program.n"); // get decimal number from user System.out.print("Please enter an integer value: "); decNum = cin.nextLong(); if(decNum < 0) // if user inputs a neg number, make the binary num neg too { binaryNum = "-"; } // method call to convert decimal to binary binaryNum += DecToBin(decNum); // display data to user System.out.println("nThe integer value of "+ decNum + " = " + binaryNum + " in binary"); }// end of main public static String DecToBin(long decNum) { // use this string to save the binary number String binary = ""; if(decNum < 0) // if input is a neg number, make it positive { decNum *= -1; } // converts decimal to binary using division and modulus while(decNum > 0) { binary += (decNum % 2); decNum /= 2; } // return the reversed string to main return new StringBuffer(binary).reverse().toString(); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Note: The code was compiled 3 separate times to display different output
====== RUN 1 ======
Welcome to My Programming Notes' Java Program.
Please enter an integer value: 5
The integer value of 5 = 101 in binary
====== RUN 2 ======
Welcome to My Programming Notes' Java Program.
Please enter an integer value: -25
The integer value of -25 = -11001 in binary
====== RUN 3 ======
Welcome to My Programming Notes' Java Program.
Please enter an integer value: 12345678910
The integer value of 12345678910 = 1011011111110111000001110000111110 in binary
Java || Whats My Name? – Practice Using Strings, Methods & Switch Statemens
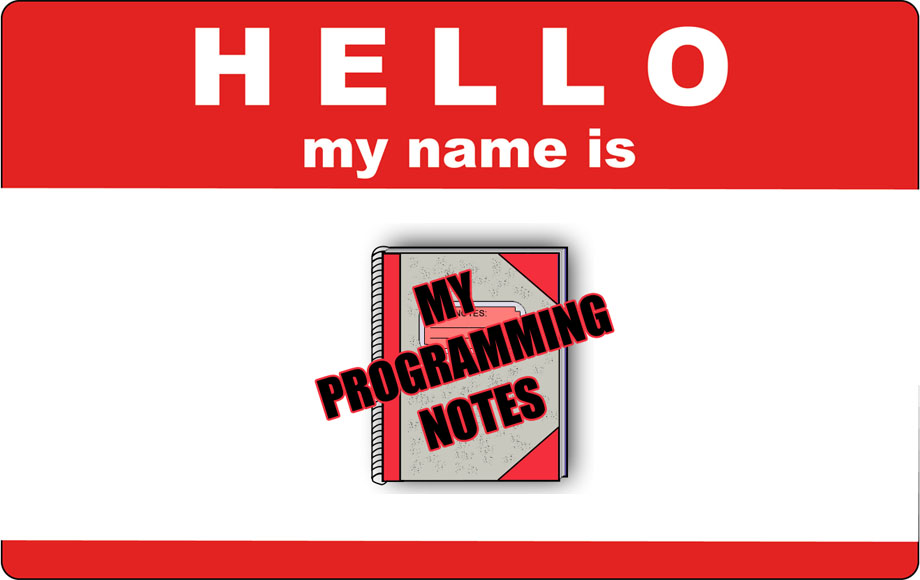
Here is another actual homework assignment which was presented in an intro to programming class. This program highlights more use using strings, modules, and switch statements.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Methods (A.K.A "Functions") - What Are They?
Switch Statements - How To Use Them
Equal - String Comparison
This program first prompts the user to enter their name. Upon receiving that information, the program saves input into a string called “firstName.” The program then asks if the user has a middle name. If they do, the user will enter a middle name. If they dont, the program proceeds to ask for a last name. Upon receiving the first, [middle], and last names, the program will append the first, [middle], and last names into a completely new string titled “fullName.” Lastly, if the users’ first, [middle], or last names are the same, the program will display that data to the screen via stdout. The program will also display to the user the number of characters their full name contains using the built in function “length.”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 |
import java.util.Scanner; public class NameString { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char response = ' '; String firstName= ""; String fullName=""; // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); // get first name from user System.out.print("Please enter your first name: "); firstName = cin.next(); System.out.print("nDo you have a middle name?(Y/N): "); String choice = cin.next(); choice = choice.toUpperCase(); response = choice.charAt(0); // use a switch statement to detrmine which function will be called switch(response) { case 'Y': fullName = FirstMiddleLast(firstName); // method declaration break; case 'N': fullName = FirstLast(firstName); // method declaration break; default: System.out.print("nPlease press either 'Y' or 'N'n" + "Program exiting...n"); System.exit(1); break; } System.out.println("And your full name is "+fullName); }// end of main // ==== FirstMiddleLast ==================================================== // // This module will take as input the first name, and prompt the user for // their middle and last name. Then it will display the total number of // characters in their name. It returns the full name back to main // // ========================================================================= private static String FirstMiddleLast(String firstName) { String middleName=""; String lastName=""; String fullName=""; System.out.print("nPlease enter your middle name: "); middleName = cin.next(); System.out.print("Please enter your last name: "); lastName = cin.next(); // copy the contents from the 3 strings into the 'fullName' string fullName = firstName+" "+middleName+" "+lastName; System.out.println(""); // check to see if the first, middle or last names are the same // if they are, display a message to the user if(firstName.equals(middleName)) { System.out.print("tYour first and middle name are the samen"); } if(middleName.equals(lastName)) { System.out.print("tYour middle and last name are the samen"); } if(firstName.equals(lastName)) { System.out.print("tYour first and last name are the samen"); } // display the total length of the string, minus the 2 white spaces System.out.println("nThe total number of characters in your " +"name is: "+(fullName.length()-2)); // return the full name string to main return fullName; }// end of FirstMiddleLast // ==== FirstLast ========================================================== // // This module will take as input the first name, and prompt the user for // their last name. Then it will display the total number of characters in // their name. It returns the full name back to main // // ============================================================================ private static String FirstLast(String firstName) { String lastName=""; String fullName=""; System.out.print("nPlease enter your last name: "); lastName = cin.next(); // copy the contents from the 3 strings into the 'fullName' string fullName = firstName+" "+lastName; System.out.println(""); // check to see if the first or last names are the same // if they are, display a message to the user if(firstName.equals(lastName)) { System.out.print("tYour first and last name are the samen"); } // display the total length of the string, minus the white space System.out.println("nThe total number of characters in your " +"name is: "+(fullName.length()-1)); // return the full name string to main return fullName; }// end of FirstLast }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Note: The code was compiled five separate times to display the different outputs its able to produce
====== RUN 1 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): y
Please enter your middle name: Programming
Please enter your last name: NotesThe total number of characters in your name is: 18
And your full name is My Programming Notes====== RUN 2 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: Programming
Do you have a middle name?(Y/N): n
Please enter your last name: NotesThe total number of characters in your name is: 16
And your full name is Programming Notes====== RUN 3 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: Notes
Do you have a middle name?(Y/N): y
Please enter your middle name: Notes
Please enter your last name: NotesYour first and middle name are the same
Your middle and last name are the same
Your first and last name are the sameThe total number of characters in your name is: 15
And your full name is Notes Notes Notes====== RUN 4 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): n
Please enter your last name: MyYour first and last name are the same
The total number of characters in your name is: 4
And your full name is My My====== RUN 5 ======
Welcome to My Programming Notes' Java Program.
Please enter your first name: My
Do you have a middle name?(Y/N): zPlease press either 'Y' or 'N'
Program exiting...
Java || Count The Total Number Of Characters, Vowels, & UPPERCASE Letters Contained In A Sentence Using A ‘For Loop’
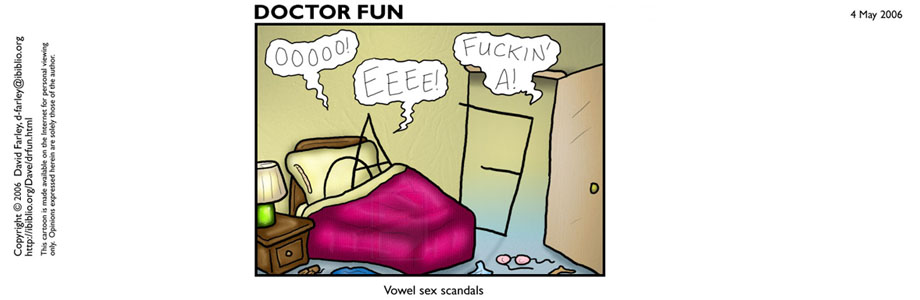
Here is a simple program, which demonstrates more practice using the input/output mechanisms which are available in Java.
This program will prompt the user to enter a sentence, then upon entering an “exit code,” will display the total number of uppercase letters, vowels and characters which are contained within that sentence. This program is very similar to an earlier project, this time, utilizing a for loop, strings, and user defined methods.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
For Loops
Methods (A.K.A "Functions") - What Are They?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
import java.util.Scanner; public class VowelCount { public static void main(String[] args) { // declare variables int numUpperCase = 0; int numVowel = 0; int numChars = 0; char character = ' '; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); System.out.print("Enter a sentence, ending with a period: "); userInput = cin.nextLine(); // loop thru the string until we reach the 'exit code' for(int index=0; userInput.charAt(index) != '.'; ++index) { character = userInput.charAt(index); // do nothing, we dont care about whitespace if(character == ' ') { continue; } // check to see if entered data is UPPERCASE if((character >= 'A')&&(character <= 'Z')) { ++numUpperCase; // Increments Total number of uppercase by one } // check to see if entered data is a vowel if((character == 'a')||(character == 'A')||(character == 'e')|| (character == 'E')||(character == 'i')||(character == 'I')|| (character == 'o')||(character == 'O')||(character == 'u')|| (character == 'U')||(character == 'y')||(character == 'Y')) { ++numVowel; // increment total number of vowels by one } ++numChars; // increment total number of chars by one } setwf("ntTotal number of upper case letters:", 45,'.'); System.out.println(numUpperCase); setwf("tTotal number of vowels:", 44,'.'); System.out.println(numVowel); setwf("tTotal number of characters:", 44,'.'); System.out.println(numChars); }// end of main public static void setwf(String str, int width, char fill) {// this mimics the C++ 'setw' function & formats string output to the screen System.out.print(str); for (int x = str.length(); x < width; ++x) { System.out.print(fill); } }// end of setwf }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted portions are areas of interest.
Notice line 22 contains the for loop declaration. The loop will continually loop thru the string, and will not stop doing so until it reaches an exit character, and the defined exit character in this program is a period (“.”). So, the program will not stop looping thru the string until it reaches a period.
Once compiling the above code, you should receive this as your output
Welcome to My Programming Notes' Java Program.
Enter a sentence, ending with a period:
My Programming Notes Is An Awesome Site.Total number of upper case letters:........7
Total number of vowels:....................14
Total number of characters:................33
Java || Compute The Sum From A String Of Integers & Display Each Number Individually
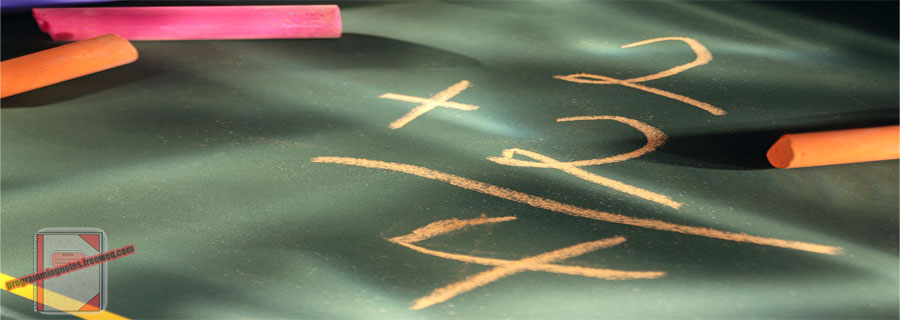
Here is a simple program, which was presented in a Java course. This program was used to introduce the input/output mechanisms which are available in Java. This assignment was modeled after Exercise 2.30, taken from the textbook “Java How to Program” (early objects) (9th Edition) (Deitel). It is the same exercise in both the 8th and 9th editions.
Our class was asked to make a program which prompts the user to enter a non-negative integer into the system. The program was supposed to then extract the digits from the inputted number, displaying them each individually, separated by a white space (” “). After the digits are displayed, the program was then supposed to display the sum of those digits to the screen. So for example, if the user inputted the number “39465,” the program would output the numbers 3 9 4 6 5 individually, and then the sum of 27.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
How To Get String Input
If/Else Statements
Do/While Loops
For Loops
Methods (A.K.A "Functions") - What Are They?
ParseInt - Convert String To Integer
Substring
Try/Catch
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
import java.util.Scanner; import java.lang.Math; public class SumFromString { public static void main(String[] args) { // declare variables char question = 'n'; String userInput= " "; Scanner cin = new Scanner(System.in); // display message to screen System.out.println("nWelcome to My Programming Notes' Java Program."); do{// this is the start of the do/while loop int sum = 0; // get data from the user System.out.print("nEnter a non negative integer: "); userInput = cin.nextLine(); System.out.println(""); // if the string isnt a number, dont compute the sum if(!IsNumeric(userInput)) { System.out.println(userInput+" is not a number..."); System.out.println("Please enter digits only!"); } // if the string is a negative number, dont compute the sum else if(IsNegative(userInput)) { System.out.println(userInput+" is a negative number..."); System.out.println("Please enter positive digits only!"); } // if the string is a decimal number (i.e 19.87), dont compute the sum else if(IsDecimal(userInput)) { System.out.println(userInput+" is a decimal number..."); System.out.println("Please enter positive whole numbers only!"); } // if everything else checks out OK, then compute the sum else { System.out.print("The digits are: "); sum = ComputeSum(userInput); System.out.println("and the sum is " + sum); } // ask user if they want to enter more data System.out.print("nDo you have more data for input? (Y/N): "); String response = cin.nextLine(); response=response.toLowerCase(); question = response.charAt(0); System.out.println("n------------------------------------------------"); }while(question == 'y'); System.out.print("nBYE!n"); }// end of main public static boolean IsNumeric(String str) {// checks to see if a string is a number try { Double.parseDouble(str); return true; } catch(NumberFormatException nfe) { return false; } }// end of IsNumeric public static boolean IsNegative(String str) {// checks to see if string is a negative number return Double.parseDouble(str) < 0; }// end of IsNegative public static boolean IsDecimal(String str) {// checks to see if string is a decimal number return (Math.floor(Double.parseDouble(str))) != Double.parseDouble(str); }// end of IsDecimal public static int ComputeSum(String userInput) {// displays each number individually & computes the sum of the string int sum = 0; String[] temp = new String[userInput.length()]; for(int counter=0; counter < userInput.length(); ++counter) { temp[counter] = userInput.substring(counter,counter+1); System.out.print(temp[counter] + " "); sum = sum + Integer.parseInt(temp[counter]); } return sum; }// end of ComputeSum }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Welcome to My Programming Notes' Java Program.
Enter a non negative integer: 0
The digits are: 0 and the sum is 0
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 39465The digits are: 3 9 4 6 5 and the sum is 27
Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: H3ll0 W0rldH3ll0 W0rld is not a number...
Please enter digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: -98-98 is a negative number...
Please enter positive digits only!Do you have more data for input? (Y/N): y
------------------------------------------------
Enter a non negative integer: 19.8719.87 is a decimal number...
Please enter positive whole numbers only!Do you have more data for input? (Y/N): n
------------------------------------------------
BYE!
C++ || Snippet – How To Read & Write Data From A User Specified Text File
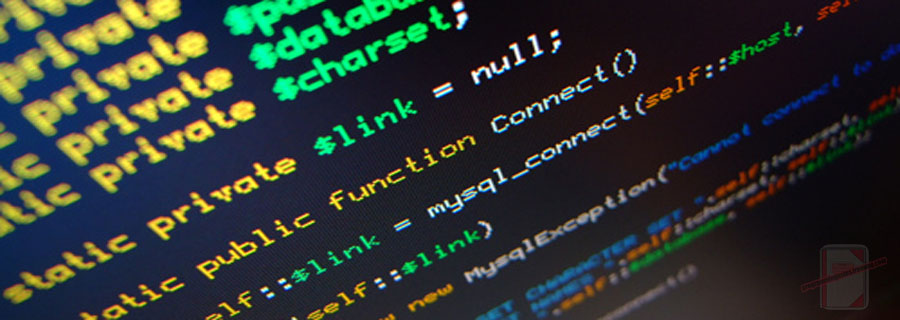
This page will consist of a demonstration of a simple quadratic formula program, which highlights the use of the input/output mechanisms of manipulating a text file. This program is very similar to an earlier snippet which was presented on this site, but in this example, the user has the option of choosing which file they want to manipulate. This program also demonstrates how to read in data from a file (numbers), manipulate that data, and output new data into a different text file.
REQUIRED KNOWLEDGE FOR THIS SNIPPET
Fstream
Ifstream
Ofstream
Working With Files
C_str() - Convert A String To Char Array Equivalent
Getline - String Version
Note: The data file that is used in this example can be downloaded here.
Also, in order to read in the data .txt file, you need to save the .txt file in the same directory (or folder) as your .cpp file is saved in. If you are using Visual C++, this directory will be located in
Documents > Visual Studio 2010 > Projects > [Your project name] > [Your project name]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
#include <iostream> #include <fstream> #include <string> #include <cmath> #include <cstdlib> using namespace std; int main() { // declare variables // char fileName[80]; string fileName; ifstream infile; ofstream outfile; double a=0,b=0,c=0; double root1=0, root2=0; // get the name of the file from the user cout << "Please enter the name of the file: "; getline(cin, fileName); // ^ you could also use a character array instead // of a string. Your getline declaration would look // like this: // --------------------------------------------- // cin.getline(fileName,80); // this opens the input file // NOTE: you need to convert the string to a // char array using the function "c_str()" infile.open(fileName.c_str()); // ^ if you used a char array as the file name instead // of a string, the declaration to open the file // would look like this: // --------------------------------------------- // infile.open(fileName); // check to see if the file even exists, & if not then EXIT if(infile.fail()) { cout<<"nError, the input file could not be found!n"; exit(1); } // this opens the output file // if the file doesnt already exist, it will be created outfile.open("OUTPUT_Quadratic_programmingnotes_freeweq_com.txt"); // this loop reads in data until there is no more // data contained in the file while(infile.peek() != EOF) { // this assigns the incoming data to the // variables 'a', 'b' and 'c' // NOTE: it is just like a cin >> statement infile>> a >> b>> c; // NOTE: if you want to read data into an array // your declaration would be like this // ------------------------------------ // infile>> a[counter] >> b[counter] >> c[counter]; // ++counter; } // this does the quadratic formula calculations root1 = ((-b) + sqrt(pow(b,2) - (4*a*c)))/(2*a); root2 = ((-b) - sqrt(pow(b,2) - (4*a*c)))/(2*a); // this displays the numbers to screen via cout cout <<"For the numbersna = "<<a<<"nb = "<<b<<"nc = "<<c<<endl; cout <<"nroot 1 = "<<root1<<"nroot 2 = "<<root2<<endl; // this saves the data to the output file // NOTE: its almost exactly the same as the cout statement outfile <<"For the numbersna = "<<a<<"nb = "<<b<<"nc = "<<c<<endl; outfile <<"nroot 1 = "<<root1<<"nroot 2 = "<<root2<<endl; // close the input/output files once you are done using them infile.close(); outfile.close(); // stops the program from automatically closing cout<<"nPress ENTER to continue..."; cin.get(); return 0; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
Please enter the name of the file: INPUT_Quadratic_programmingnotes_freeweq_com.txt
For the numbers
a = 2
b = 4
c = -16root 1 = 2
root 2 = -4Press ENTER to continue...
C++ || Class – Roman Numeral To Integer & Integer To Roman Numeral Conversion
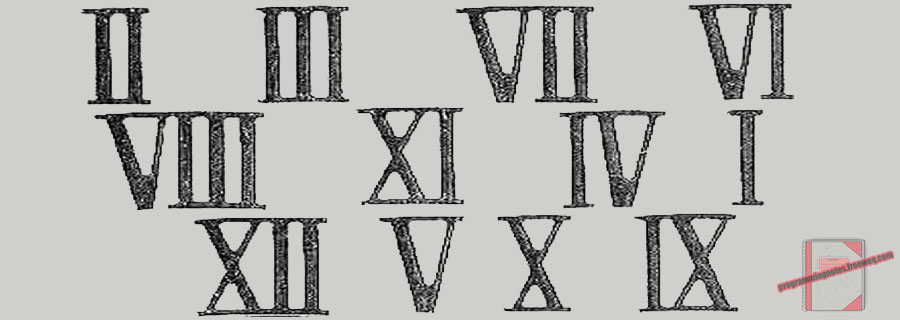
The following is another homework assignment which was presented in a C++ Data Structures course. This program was assigned in order to practice the use of the class data structure, which is very similar to the struct data structure.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Header Files - How To Use Them
Class - What Is It?
Do/While Loop
Passing a Value By Reference
Roman Numerals - How Do You Convert To Decimal?
Online Roman Numeral Converter - Check For Correct Results
This is an interactive program in which the user has the option of selecting from 7 modes of operation. Of those modes, the user has the option of entering in roman numerals for conversion, entering in decimal numbers for conversion, displaying the recently entered number, and finally converting between roman or decimal values.
A sample of the menu is as followed:
(Where the user would enter numbers 1-7 to select a choice)
1 2 3 4 5 6 7 8 9 10 11 |
From the following menu: 1. Enter a Decimal number 2. Enter a Roman Numeral 3. Convert from Decimal to Roman 4. Convert from Roman to Decimal 5. Print the current Decimal number 6. Print the current Roman Numeral 7. Quit Please enter a selection: |
This program was implemented into 3 different files (two .cpp files, and one header file .h). So the code for this program will be broken up into 3 sections, the main file (.cpp), the header file (.h), and the implementation of the functions within the header file (.cpp).
======== FILE #1 – Menu.cpp ========
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
// ============================================================================ // File: Menu.cpp // Purpose: Converts a number enetered in Decimal to Roman Numeral and a // Roman Numeral to a Decimal Number // ============================================================================ #include <iostream> #include <iomanip> #include <string> #include "ClassRomanType.h" using namespace std; // function prototypes void DisplayMenu(); int GetCommand(int command); void RunChoice(int command, ClassRomanType& romanObject); int main() { int command=7; // Holds which action the user chooses to make ClassRomanType romanObject; // Gives access to the class do{ DisplayMenu(); command = GetCommand(command); RunChoice(command, romanObject); cout<<"n--------------------------------------------------------------------n"; }while(command!=7); cout<<"nBye!n"; return 0; }// End of main void RunChoice(int command, ClassRomanType& romanObject) {// Function: Execute the choice selected from the menu // declare private variables int decimalNumber=0; // Holds decimal value char romanNumeral[150]; //Holds value of roman numeral // execute the desired choice cout <<"ntYou selected choice #"<<command<<" which will: "; switch(command) { case 1: cout << "Get Decimal Numbernn"; cout <<"Enter a Decimal Number: "; cin >> decimalNumber; romanObject.GetDecimalNumber(decimalNumber); break; case 2: cout << "Get Roman Numeralnn"; cout <<"nEnter a Roman Numeral: "; cin >> romanNumeral; romanObject.GetRomanNumeral(romanNumeral); break; case 3: cout << "Convert from Decimal to Romannn"; romanObject.ConvertDecimalToRoman(); cout<<"Running....nComplete!n"; break; case 4: cout << "Convert from Roman to Decimalnn"; romanObject.ConvertRomanToDecimal(); cout<<"Running....nComplete!n"; break; case 5: cout << "Print the current Decimal numbernn"; cout<<"n The current Roman to Decimal Value is: "; cout << romanObject.ReturnDecimalNumber()<<endl; break; case 6: cout << "Print the current Roman Numeralnn"; cout<<"n The current Decimal to Roman Value is: "; cout << romanObject.ReturnRomanNumber()<<endl; break; case 7: cout << "Quitnn"; break; default: cout << "nError, you entered an invalid command!nPlease try again..."; break; } }//End of RunChoice int GetCommand(int command) {// Function: Gets choice from menu cout <<"nPlease enter a selection: "; cin>> command; return command; }// End of GetCommand void DisplayMenu() {// Function: Displays menu cout <<"From the following menu:n"<<endl; cout <<left<<setw(2)<<"1."<<left<<setw(15)<<" Enter a Decimal number"<<endl; cout <<left<<setw(2)<<"2."<<left<<setw(15)<<" Enter a Roman Numeral"<<endl; cout <<left<<setw(2)<<"3."<<left<<setw(15)<<" Convert from Decimal to Roman"<<endl; cout <<left<<setw(2)<<"4."<<left<<setw(15)<<" Convert from Roman to Decimal"<<endl; cout <<left<<setw(2)<<"5."<<left<<setw(15)<<" Print the current Decimal number"<<endl; cout <<left<<setw(2)<<"6."<<left<<setw(15)<<" Print the current Roman Numeral"<<endl; cout <<left<<setw(2)<<"7."<<left<<setw(15)<<" Quit"<<endl; }// http://programmingnotes.org/ |
======== FILE #2 – ClassRomanType.h ========
Remember, you need to name the header file the same as the #include from the Menu.cpp file. This file contains the function declarations, but no implementation of those functions takes place here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
// ============================================================================ // File: ClassRomanType.h // Purpose: Header file for Roman Numeral conversion. It contains all // declarations and prototypes for the class members to carry out the // conversion // ============================================================================ #include <string> using namespace std; class ClassRomanType { public: ClassRomanType(); /* Function: constructor initializes class variables to "0" Precondition: none Postcondition: defines private variables */ // member functions void GetDecimalNumber(int decimalNumber); /* Function: gets decimal number Precondition: none Postcondition: Gets arabic number from user */ void GetRomanNumeral(char romanNumeral[]); /* Function: gets roman numeral Precondition: none Postcondition: Gets roman numeral from user */ void ConvertDecimalToRoman(); /* Function: converts decimal number to ronman numeral Precondition: arabic and roman numeral characters should be known Postcondition: Gets roman numeral from user */ void ConvertRomanToDecimal(); /* Function: converts roman numeral to arabic number Precondition: arabic and roman numeral characters should be known Postcondition: Gets decimal number from user */ int ReturnDecimalNumber(); /* Function: displays converted decimal number Precondition: the converted roman numeral to decimal number Postcondition: displays decimal number to user */ string ReturnRomanNumber(); /* Function: displays converted roman numeral Precondition: the converted decimal number to roman numeral Postcondition: displays roman numeral to user */ ~ClassRomanType(); /* Function: destructor used to return memory to the system Precondition: the converted decimal number to roman numeral Postcondition: none */ private: char m_romanValue[150]; // An array holding the roman value within the class int m_decimalValue; // Holds the final output answer of the roman to decimal conversion }; // http://programmingnotes.org/ |
======== FILE #3 – RomanType.cpp ========
This is the function implementation file for the ClassRomanType.h class. This file can be named anything you wish as long as you #include “ClassRomanType.h”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 |
// ============================================================================ // File: RomanType.cpp // Purpose: Contains the implementation of the class members for the // roman numeral conversion // // *NOTE*: Uncomment cout statements to visualize whats happening // ============================================================================ #include <iostream> #include <string> #include <cstring> #include "ClassRomanType.h" using namespace std; ClassRomanType::ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// end of ClassRomanType void ClassRomanType:: GetDecimalNumber(int decimalNumber) { m_decimalValue = decimalNumber; }// end of GetDecimalNumber void ClassRomanType:: GetRomanNumeral(char romanNumeral[]) { strcpy(m_romanValue,romanNumeral); }// end of GetRomanNumeral void ClassRomanType:: ConvertDecimalToRoman() { strcpy(m_romanValue,""); // erases any previous data int numericValue[]={1000,900,500,400,100,90,50,40,10,9,5,4,1,0}; char *romanNumeral[]={"M","CM","D","CD","C","XC","L" ,"XL","X","IX","V","IV","I",0}; for (int x=0; numericValue[x] > 0; ++x) { //cout<<endl<<numericValue[x]<<endl; while (m_decimalValue >= numericValue[x]) { //cout<<"current m_decimalValue = " <<m_decimalValue<<endl; //cout<<"current numericValue = "<<numericValue[x]<<endl<<endl; if(strlen(m_romanValue)==0) { strcpy(m_romanValue,romanNumeral[x]); } else { strcat(m_romanValue,romanNumeral[x]); } m_decimalValue -= numericValue[x]; } } }// end of ConvertDecimalToRoman void ClassRomanType:: ConvertRomanToDecimal() { int currentNumber = 0; // Holds value of each letter numerical value int previousNumber = 0; // Holds the numerical value being compared m_decimalValue=0; // Resets the current 'romanToDecimalValue' to zero if(strcmp(m_romanValue,"Currently Undefined")!=0) { for (int counter = strlen(m_romanValue)-1; counter >= 0; counter--) { if ((m_romanValue[counter] == 'M') || (m_romanValue[counter] == 'm')) { currentNumber = 1000; } else if ((m_romanValue[counter] == 'D') || (m_romanValue[counter] == 'd')) { currentNumber = 500; } else if ((m_romanValue[counter] == 'C') || (m_romanValue[counter] == 'c')) { currentNumber = 100; } else if ((m_romanValue[counter] == 'L') || (m_romanValue[counter] == 'l')) { currentNumber = 50; } else if ((m_romanValue[counter] == 'X') || (m_romanValue[counter] == 'x')) { currentNumber = 10; } else if ((m_romanValue[counter] == 'V') || (m_romanValue[counter] == 'v')) { currentNumber = 5; } else if ((m_romanValue[counter] == 'I') || (m_romanValue[counter] == 'i')) { currentNumber = 1; } else { currentNumber = 0; } //cout<<"previousNumber = " <<previousNumber<<endl; //cout<<"currentNumber = " <<currentNumber<<endl; if (previousNumber > currentNumber) { m_decimalValue -= currentNumber; previousNumber = currentNumber; } else { m_decimalValue += currentNumber; previousNumber = currentNumber; } //cout<<"current m_decimalValue = " <<m_decimalValue<<endl<<endl; } } }// end of ConvertRomanToDecimal int ClassRomanType::ReturnDecimalNumber() { return m_decimalValue; }// end of ReturnDecimalNumber string ClassRomanType::ReturnRomanNumber() { return m_romanValue; }// end of ReturnRomanNumber ClassRomanType::~ClassRomanType() { m_decimalValue=0; strcpy(m_romanValue,"Currently Undefined"); }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output
From the following menu:
1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 9
You selected choice #9 which will:
Error, you entered an invalid command!
Please try again...
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 0
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: Currently Undefined
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 1
You selected choice #1 which will: Get Decimal Number
Enter a Decimal Number: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 1987
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 3
You selected choice #3 which will: Convert from Decimal to Roman
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: MCMLXXXVII
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 2
You selected choice #2 which will: Get Roman Numeral
Enter a Roman Numeral: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 6
You selected choice #6 which will: Print the current Roman Numeral
The current Decimal to Roman Value is: mMxiI
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 4
You selected choice #4 which will: Convert from Roman to Decimal
Running....
Complete!
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 5
You selected choice #5 which will: Print the current Decimal number
The current Roman to Decimal Value is: 2012
--------------------------------------------------------------------
From the following menu:1. Enter a Decimal number
2. Enter a Roman Numeral
3. Convert from Decimal to Roman
4. Convert from Roman to Decimal
5. Print the current Decimal number
6. Print the current Roman Numeral
7. QuitPlease enter a selection: 7
You selected choice #7 which will: Quit
--------------------------------------------------------------------
Bye!
Java || Using If Statements, Char & String Variables
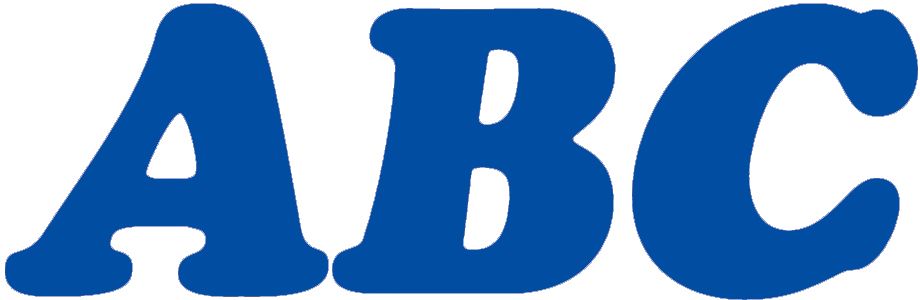
As previously mentioned, you can use the “int/float/double” data type to store numbers. But what if you want to store letters? Char and Strings help you do that.
===== SINGLE CHAR =====
This example will demonstrate a simple program using char, which checks to see if you entered the correctly predefined letter.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Mar 30, 2021 // Taken From: http://programmingnotes.org/ // File: GuessALetter.java // Description: Demonstrates using char variables // ============================================================================ import java.util.Scanner; public class GuessALetter { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char userInput = ' '; System.out.print("Please try to guess the letter I am thinking of: "); // get a single character from the user data input userInput = cin.next().charAt(0); // Use an If Statement to check equality if (userInput == 'a' || userInput == 'A') { System.out.println("You have Guessed correctly!"); } else { System.out.println("Sorry, that was not the correct letter I was thinking of"); } }// end main }// http://programmingnotes.org/ |
Notice in line 19 I declare the char data type, naming it “userInput.” I also initialized it as an empty variable. In line 26 I used an “If/Else Statement” to determine if the user inputted value matches the predefined letter within the program. I also used the “OR” operator in line 26 to determine if the letter the user inputted was lower or uppercase. Try compiling the program simply using this
if (userInput == 'a')
as your if statement, and notice the difference.
The resulting code should give this as output
Please try to guess the letter I am thinking of: A
You have Guessed correctly!
===== CHECK IF LETTER IS UPPER CASE =====
This example is similar to the previous one, and will check if a letter is uppercase
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Mar 30, 2021 // Taken From: http://programmingnotes.org/ // File: CheckIfUppercase.java // Description: Demonstrates checking if a char variable is uppercase // ============================================================================ import java.util.Scanner; public class CheckIfUppercase { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char userInput = ' '; System.out.print("Please enter an UPPERCASE letter: "); // get a single character from the user data input userInput = cin.next().charAt(0); // Checks to see if inputted data falls between uppercase values if ((userInput >= 'A') && (userInput <= 'Z')) { System.out.println(userInput + " is an uppercase letter"); } else { System.out.println(userInput + " is not an uppercase letter"); } }// end main }// http://programmingnotes.org/ |
Notice in line 26, an If statement was used, which checked to see if the user inputted data fell between letter A and letter Z. We did that by using the “AND” operator. So that IF statement is basically saying (in plain english)
IF ('userInput' is equal to or greater than 'A') AND ('userInput' is equal to or less than 'Z')
THEN it is an uppercase letter
The resulting code should give this as output
Please enter an UPPERCASE letter: p
p is not an uppercase letter
===== CHECK IF LETTER IS A VOWEL =====
This example will utilize more if statements, checking to see if the user inputted data is a vowel or not. This will be very similar to the previous example, utilizing the OR operator once again.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Mar 30, 2021 // Taken From: http://programmingnotes.org/ // File: CheckIfVowel.java // Description: Demonstrates checking if a char variable is a vowel // ============================================================================ import java.util.Scanner; public class CheckIfVowel { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables char userInput = ' '; System.out.print("Please enter a vowel: "); // get a single character from the user data input userInput = cin.next().charAt(0); // Checks to see if entered data is A,E,I,O,U,Y if ((userInput == 'a')||(userInput == 'A')||(userInput == 'e')|| (userInput == 'E')||(userInput == 'i')||(userInput == 'I')|| (userInput == 'o')||(userInput == 'O')||(userInput == 'u')|| (userInput == 'U')||(userInput == 'y')||(userInput == 'Y')) { System.out.println("Correct, " + userInput + " is a vowel!"); } else { System.out.println("Sorry, " + userInput + " is not a vowel"); } }// end main }// http://programmingnotes.org/ |
This program should be very straight forward, and its basically checking to see if the user entered data is the letter A, E, I, O, U or Y.
The resulting code should give the following output
Please enter a vowel: o
Correct, o is a vowel!
===== HELLO WORLD v2 =====
This last example will demonstrate using the string data type to print the line “Hello World!” to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Mar 30, 2021 // Taken From: http://programmingnotes.org/ // File: HelloWorldString.java // Description: Demonstrates using string variables // ============================================================================ import java.util.Scanner; public class HelloWorldString { // global variable declaration static Scanner cin = new Scanner(System.in); public static void main(String[] args) { // declare variables String userInput = ""; System.out.print("Please enter a sentence: "); // get a string from the user userInput = cin.nextLine(); // display message to user System.out.println("You entered: " + userInput); }// end main }// http://programmingnotes.org/ |
The resulting code should give following output
Please enter a sentence: Hello World!
You entered: Hello World!
===== HOW TO COMPILE CODE USING THE TERMINAL =====
*** This can be achieved by typing the following command:
(Notice the .java source file is named exactly the same as the class header)
javac YOUR_CLASS_NAME.java
*** To run the compiled program, simply type this command:
java YOUR_CLASS_NAME
C++ || Find The Day Of The Week You Were Born Using Functions, String, Modulus, If/Else, & Switch
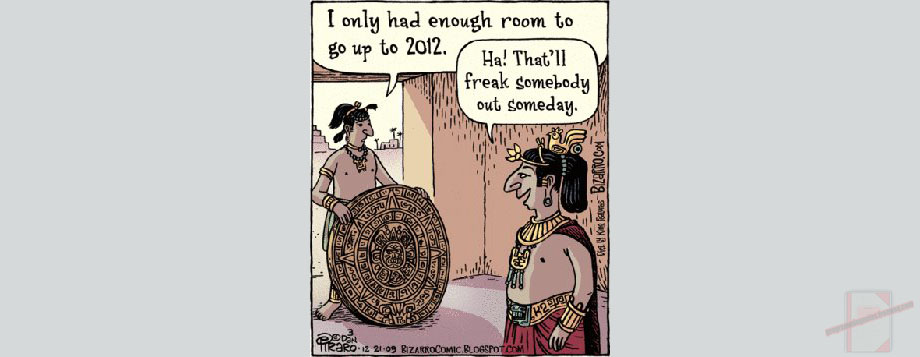
This program displays more practice using functions, modulus, if and switch statements.
REQUIRED KNOWLEDGE FOR THIS PROGRAM
Functions
Strings
Modulus
If/Else Statements
Switch Statements
Knowledge of Leap Years
A Calendar
This program prompts the user for their name, date of birth (month, day, year), and then displays information back to them via cout. Once the program obtains selected information from the user, it will use simple math to determine the day of the week in which the user was born, and determine the day of the week their current birthday will be for the current calendar year. The program will also display to the user their current age, along with re-displaying their name back to them.
NOTE: On some compilers, you may have to add #include < cstdlib> in order for the code to compile.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 |
#include <iostream> #include <string> using namespace std; // a const variable containing the current year const int CURRENT_YEAR = 2012; // function prototypes string GetNameOfMonth(int numOfMonth); int GetLastTwoDigits(int fourDigitYear); string GetDayOfWeek(int numOfMonth, int lastTwoDigits, int numOfDay, int fourDigitYear); int main () { // declare variables string nameOfMonth = " ", dayOfWeek = " ", userName = " ", dayOfWeekCurrentYear = " " ; int numOfMonth=0, numOfDay=0, fourDigitYear=0, lastTwoDigits=0, lastTwoDigitsCurrentYear; // user inputs data cout << "Please enter your name: "; cin >> userName; cout << "nPlease enter the month in which you were born (between 1 and 12): "; cin >> numOfMonth; // converts the number the user inputted from above ^ // into a literal month name (i.e month #1 = January) nameOfMonth = GetNameOfMonth(numOfMonth); // user inputs data cout << "nPlease enter the day you were born (between 1 and 31): "; cin >> numOfDay; // checks to see if user entered valid data if (numOfDay < 1 || numOfDay > 31) { cout << "nYou have entered an invalid number of day. " << "Please enter a valid number." << endl << endl; exit(1); } // user inputs data cout << "nPlease enter the year you were born (between 1900 and 2099): "; cin >> fourDigitYear; cout << endl << endl; // checks to see if user entered valid data if (fourDigitYear < 1900 || fourDigitYear > 2099) { cout << "You have entered an invalid year. " << "Please enter a valid year." << endl << endl; exit(1); } // gets the las two digits in which the user was born // (i.e 1999: last two digits = 99) lastTwoDigits = GetLastTwoDigits(fourDigitYear); // gets the las two digits of the current year // (i.e 2012: last two digits = 12) lastTwoDigitsCurrentYear = GetLastTwoDigits(CURRENT_YEAR); // finds the day of the week in which the user was born dayOfWeek = GetDayOfWeek(numOfMonth, lastTwoDigits, numOfDay, fourDigitYear); // finds the day of the week the users current birthday will be this year dayOfWeekCurrentYear = GetDayOfWeek(numOfMonth, lastTwoDigitsCurrentYear, numOfDay, CURRENT_YEAR); // display data to user cout << "Hello " << userName <<". Here are some facts about you!" <<endl; cout << "You were born "<< nameOfMonth << numOfDay << " "<< fourDigitYear << endl; cout << "Your birth took place on a " << dayOfWeek << endl; cout << "This year your birthday will take place on a " << dayOfWeekCurrentYear << endl; cout << "You currently are, or will be " << (CURRENT_YEAR - fourDigitYear) << " years old this year!"<<endl; return 0; }// end of main // converts the number the user inputted from above ^ // into a literal month name (i.e month #1 = January) string GetNameOfMonth(int numOfMonth) { string nameOfMonth = " "; // a switch determining what month the user was born switch(numOfMonth) { case 1: nameOfMonth = "January "; break; case 2: nameOfMonth = "February "; break; case 3: nameOfMonth = "March "; break; case 4: nameOfMonth = "April "; break; case 5: nameOfMonth = "May "; break; case 6: nameOfMonth = "June "; break; case 7: nameOfMonth = "July "; break; case 8: nameOfMonth = "August "; break; case 9: nameOfMonth = "September "; break; case 10: nameOfMonth = "October "; break; case 11: nameOfMonth = "November "; break; case 12: nameOfMonth = "December "; break; default: cout << "You have entered an invalid number of month. nPlease enter a valid number.n" << "Program Terminating.."; exit(1); break; }// end of switch return nameOfMonth ; }// end of GetNamOfMonth // gets the las two digits in which the user was born/current year int GetLastTwoDigits(int fourDigitYear) { if (fourDigitYear >= 1900 && fourDigitYear <= 1999) { return (fourDigitYear - 1900); } else { return (fourDigitYear - 2000); } }// end of GetLastTwoDigits // finds the day of the week in which the user was born/current year string GetDayOfWeek(int numOfMonth, int lastTwoDigits, int numOfDay, int fourDigitYear) { string dayOfWeek = " "; int Total = 0, valueOfMonth = 0; if (numOfMonth == 1) valueOfMonth = 1; else if (numOfMonth == 2) valueOfMonth = 4; else if (numOfMonth == 3) valueOfMonth = 4; else if (numOfMonth == 4) valueOfMonth = 0; else if (numOfMonth == 5) valueOfMonth = 2; else if (numOfMonth == 6) valueOfMonth = 5; else if (numOfMonth == 7) valueOfMonth = 0; else if (numOfMonth == 8) valueOfMonth = 3; else if (numOfMonth == 9) valueOfMonth = 6; else if (numOfMonth == 10) valueOfMonth = 1; else if (numOfMonth == 11) valueOfMonth = 4; else if (numOfMonth == 12) valueOfMonth = 6; else cout <<"nError"; Total = lastTwoDigits / 4; Total += lastTwoDigits; Total += numOfDay; Total += valueOfMonth; // uses the 'Total' variable from above to determine the day // of the week in which you were born if ((fourDigitYear > 2000)&&((numOfMonth != 1)&&(numOfMonth != 2))&&(fourDigitYear != 2004)) { Total = Total + 6; } else if ((fourDigitYear < 2000) && ((numOfMonth == 1) || (numOfMonth == 2))) { Total = Total + 7; } else if ((fourDigitYear == 2000) && ((numOfMonth == 1) || (numOfMonth == 2))) { Total = Total -2; } else if ((fourDigitYear == 2004||fourDigitYear == 2000)&&((numOfMonth != 1)&&(numOfMonth != 2))) { Total = Total -1; } else if ((fourDigitYear % 4 == 0) && (fourDigitYear % 100 == lastTwoDigits) && (fourDigitYear % 400 == lastTwoDigits)) { Total = Total-2; } else if ((fourDigitYear > 2000)&&((numOfMonth == 1)||(numOfMonth == 2))&&(fourDigitYear != 2004)) { Total = Total + 6; } // uses the 'Total' variable from above to determine the day // of the week in which you were born/when you birthday will be this year if (Total % 7 == 1) dayOfWeek = "Sunday"; else if (Total % 7 == 2) dayOfWeek = "Monday"; else if (Total % 7 == 3) dayOfWeek = "Tuesday"; else if (Total % 7 == 4) dayOfWeek = "Wednesday"; else if (Total % 7 == 5) dayOfWeek = "Thursday"; else if (Total % 7 == 6) dayOfWeek = "Friday"; else if (Total % 7 == 0) dayOfWeek = "Saturday"; else cout<< "nError"; return dayOfWeek; }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Once compiled, you should get this as your output:
Note: The code was compiled five separate times to display the different outputs its able to produce
Please enter your name: MyProgrammingNotes
Please enter the month in which you were born (between 1 and 12): 1
Please enter the day you were born (between 1 and 31): 1
Please enter the year you were born (between 1900 and 2099): 2012Hello MyProgrammingNotes. Here are some facts about you!
You were born January 1 2012
Your birth took place on a Sunday
This year your birthday will take place on a Sunday
You currently are, or will be 0 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 4
Please enter the day you were born (between 1 and 31): 2
Please enter the year you were born (between 1900 and 2099): 1957Hello Name. Here are some facts about you!
You were born April 2 1957
Your birth took place on a Tuesday
This year your birthday will take place on a Monday
You currently are, or will be 55 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 5
Please enter the day you were born (between 1 and 31): 7
Please enter the year you were born (between 1900 and 2099): 1999Hello Name. Here are some facts about you!
You were born May 7 1999
Your birth took place on a Friday
This year your birthday will take place on a Monday
You currently are, or will be 13 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 8
Please enter the day you were born (between 1 and 31): 4
Please enter the year you were born (between 1900 and 2099): 1983Hello Name. Here are some facts about you!
You were born August 4 1983
Your birth took place on a Thursday
This year your birthday will take place on a Saturday
You currently are, or will be 29 years old this year!
---------------------------------------------------------------------Please enter your name: Name
Please enter the month in which you were born (between 1 and 12): 6
Please enter the day you were born (between 1 and 31): 7
Please enter the year you were born (between 1900 and 2099): 2987You have entered an invalid year. Please enter a valid year.
Press any key to continue . . .
C++ || Using If Statements, Char & String Variables
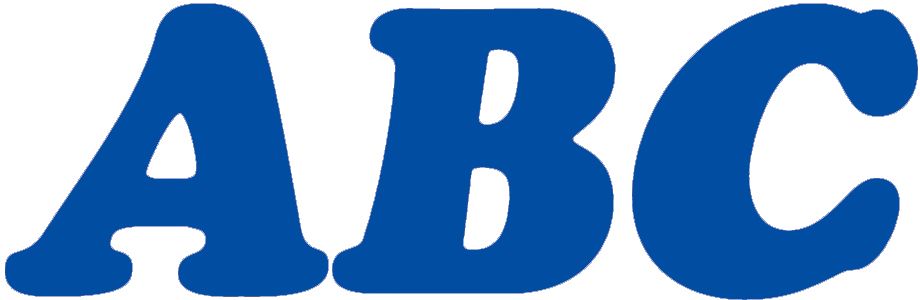
As previously mentioned, you can use the “int/float/double” data type to store numbers. But what if you want to store letters? Char and Strings help you do that.
==== SINGLE CHAR ====
This example will demonstrate a simple program using char, which checks to see if you entered the correctly predefined letter.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 8, 2021 // Taken From: http://programmingnotes.org/ // File: char.cpp // Description: Demonstrates using char variables // ============================================================================ #include <iostream> int main() { // Declare empty variable char userInput = ' '; std::cout << "Please try to guess the letter I am thinking of: "; std::cin >> userInput; // Use an If Statement to check equality if (userInput == 'a' || userInput == 'A') { std::cout <<"\nYou have Guessed correctly!"<< std::endl; } else { std::cout <<"\nSorry, that was not the correct letter I was thinking of"<< std::endl; } return 0; }// http://programmingnotes.org/ |
Notice in line 13 I declare the char data type, naming it “userInput.” I also initialized it as an empty variable. In line 19 I used an “If/Else Statement” to determine if the user inputted value matches the predefined letter within the program. I also used the “OR” operator in line 19 to determine if the letter the user inputted was lower or uppercase. Try compiling the program simply using this
if (userInput == 'a')
as your if statement, and notice the difference.
The resulting code should give this as output
Please try to guess the letter I am thinking of: K
Sorry, that was not the correct letter I was thinking of
==== CHECK IF LETTER IS UPPER CASE ====
This example is similar to the previous one, and will check if a letter is uppercase.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 8, 2021 // Taken From: http://programmingnotes.org/ // File: uppercase.cpp // Description: Demonstrates checking if a char variable is uppercase // ============================================================================ #include <iostream> int main() { // Declare empty variable char userInput = ' '; std::cout << "Please enter an UPPERCASE letter: "; std::cin >> userInput; // Checks to see if entered data falls between uppercase values if ((userInput >= 'A') && (userInput <= 'Z')) { std::cout << "\n" << userInput << " is an uppercase letter"<< std::endl; } else { std::cout <<"\nSorry, "<< userInput <<" is not an uppercase letter"<< std::endl; } return 0; }// http://programmingnotes.org/ |
Notice in line 19, an If statement was used, which checked to see if the user entered data fell between letter A and letter Z. We did that by using the “AND” operator. So that IF statement is basically saying (in plain english)
IF ('userInput' is equal to or greater than 'A') AND ('userInput' is equal to or less than 'Z')
THEN it is an uppercase letter
C++ uses ASCII codes to determine letters, so from looking at the table, the letter ‘A’ would equal ASCII code number 65, letter ‘B’ would equal ASCII code number 66 and so forth, until you reach letter Z, which would equal ASCII code number 90. So in literal terms, the program is checking to see if the user input is between ASCII code number 65 thru 90. If it is, then the number is an uppercase letter, otherwise it is not.
The resulting code should give this as output
Please enter an UPPERCASE letter: G
G is an uppercase letter
==== CHECK IF LETTER IS A VOWEL ====
This example will utilize more if statements, checking to see if the user inputted data is a vowel or not. This will be very similar to the previous example, utilizing the OR operator once again.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 8, 2021 // Taken From: http://programmingnotes.org/ // File: vowel.cpp // Description: Demonstrates checking if a char variable is a vowel // ============================================================================ #include <iostream> int main() { // Declare empty variable char userInput = ' '; std::cout << "Please enter a vowel: "; std::cin >> userInput; // Checks to see if inputted data is A,E,I,O,U,Y if ((userInput == 'a')||(userInput == 'A')||(userInput == 'e')|| (userInput == 'E')||(userInput == 'i')||(userInput == 'I')|| (userInput == 'o')||(userInput == 'O')||(userInput == 'u')|| (userInput == 'U')||(userInput == 'y')||(userInput == 'Y')) { std::cout <<"\nCorrect, "<< userInput <<" is a vowel!"<< std::endl; } else { std::cout <<"\nSorry, "<< userInput <<" is not a vowel"<< std::endl; } return 0; }// http://programmingnotes.org/ |
This program should be very straight forward, and its basically checking to see if the user inputted data is the letter A, E, I, O, U or Y.
The resulting code should give the following output
Please enter a vowel: O
Correct, O is a vowel!
==== HELLO WORLD v2 ====
This last example will demonstrate using the string data type to print the line “Hello World!” to the screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// ============================================================================ // Author: Kenneth Perkins // Date: Jan 4, 2012 // Updated: Feb 8, 2021 // Taken From: http://programmingnotes.org/ // File: string.cpp // Description: Demonstrates using string variables // ============================================================================ #include <iostream> #include <string> int main() { // Declare empty variable std::string userInput = " "; std::cout << "Please enter a sentence: "; std::getline(std::cin, userInput); std::cout << "\nYou Entered: "<<userInput << std::endl; return 0; }// http://programmingnotes.com |
Notice in line 10 we have to add “#include string” in order to use the getline function, which is used on line 17. Rather than just simply using the “cin” function, we used the getline function instead to read in data. That is because cin is unable to read entire sentences as input. So in line 17, the following code reads a line from the user input until a newline is entered.
The resulting code should give following output
Please enter a sentence: Hello World!
You Entered: Hello World!