Tag Archives: validate email
C# || How To Validate An Email Address Using C#
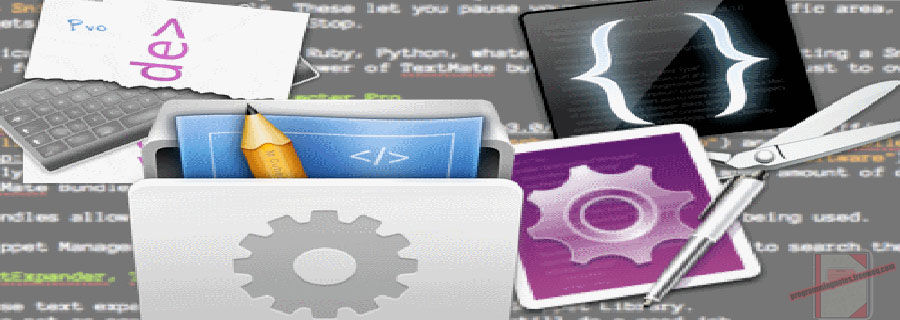
The following is a module with functions which demonstrates how to determine if an email address is valid using C#.
This function is based on RFC2821 and RFC2822 to determine the validity of an email address.
1. Validate Email – Basic Usage
The example below demonstrates the use of ‘Utils.Email.IsValid‘ to determine if an email address is valid.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
// Validate Email var addresses = new List<string>() { "a@gmail.comm", "name@yah00.com", "_name@yah00.com", "name@again@example.com", "anystring@anystring.anystring", "prettyandsimple@example.com", "very.common@example.com", "disposable.style.email.with+symbol@example.com", "other.email-with-dash@example.com", "x@example.com", "\"much.more unusual\"@example.com", "\"very.unusual.@.unusual.com\"@example.com", "<< example-indeed@strange-example.com >>", "<< admin@mailserver1 >>", @"<< #!$%&\'*+-/=?^_`{}|~@example.org >>", "example@s.solutions", "user@com", "john.doe@example..com", "john..doe@example.com", "A@b@c@example.com" }; foreach (var email in addresses) { Console.WriteLine($"Email: {email}, Is Valid: {Utils.Email.IsValid(email)}"); } // expected output: /* Email: a@gmail.comm, Is Valid: True Email: name@yah00.com, Is Valid: True Email: _name@yah00.com, Is Valid: False Email: name@again@example.com, Is Valid: False Email: anystring@anystring.anystring, Is Valid: True Email: prettyandsimple@example.com, Is Valid: True Email: very.common@example.com, Is Valid: True Email: disposable.style.email.with+symbol@example.com, Is Valid: True Email: other.email-with-dash@example.com, Is Valid: True Email: x@example.com, Is Valid: True Email: "much.more unusual"@example.com, Is Valid: False Email: "very.unusual.@.unusual.com"@example.com, Is Valid: False Email: << example-indeed@strange-example.com >>, Is Valid: False Email: << admin@mailserver1 >>, Is Valid: False Email: << #!$%&\'*+-/=?^_`{}|~@example.org >>, Is Valid: False Email: example@s.solutions, Is Valid: True Email: user@com, Is Valid: False Email: john.doe@example..com, Is Valid: False Email: john..doe@example.com, Is Valid: False Email: A@b@c@example.com, Is Valid: False */ |
2. Validate Email – Additional Options
The example below demonstrates the use of ‘Utils.Email.IsValid‘ to determine if an email address is valid with additional validation options.
Supplying options to verify an email address uses the default validity rules, in addition to the provided validation options.
This allows you to add custom rules to determine if an email is valid or not. For example, the misspelling of common email domains, or known spam domains.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
// Verify Email Using Default Rules With Additional Options var addresses = new List<string>() { "a@gmail.comm", "name@yah00.com", "prettyandsimple@bumpymail.com", "disposable@gold2world.com", "user@gold2world.biz" }; // Additional options var options = new Utils.Email.Options() { InvalidDomains = new[] { // IEnumerable of invalid domains. Example: gmail.com "aichyna.com", "gawab.com", "gold2world.biz", "front14.org", "bumpymail.com", "pleasantphoto.com", "spam.la" }, InvalidTopLevelDomains = new string[] { // IEnumerable of invalid top level domains. Example: .com "comm", "nett", "c0m" }, InvalidSecondLevelDomains = new List<string>() { // IEnumerable of invalid second level domains. Example: gmail "yah00", "ynail" } }; foreach (var email in addresses) { Console.WriteLine($"Email: {email}, Is Valid: {Utils.Email.IsValid(email, options)}"); } // expected output: /* Email: a@gmail.comm, Is Valid: False Email: name@yah00.com, Is Valid: False Email: prettyandsimple@bumpymail.com, Is Valid: False Email: disposable@gold2world.com, Is Valid: True Email: user@gold2world.biz, Is Valid: False */ |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 8, 2021 // Taken From: http://programmingnotes.org/ // File: Utils.cs // Description: Handles general utility functions // ============================================================================ using System; using System.Linq; using System.Collections.Generic; namespace Utils { public static class Email { /// <summary> /// Based on RFC2821 and RFC2822 to determine the validity of an /// email address /// </summary> /// <param name="emailAddress">The email address to verify</param> /// <param name="options">Additional email restrictions</param> /// <returns>True if the email address is valid, false otherwise</returns> public static bool IsValid(string emailAddress, Email.Options options = null) { if (string.IsNullOrWhiteSpace(emailAddress) || emailAddress.Length > 100) { return false; } var optionsPattern = options == null ? string.Empty : options.Pattern; // https://www.jochentopf.com/email/characters-in-email-addresses.pdf var pattern = "^[^a-zA-Z0-9]|[^a-zA-Z0-9]$" + // First and last characters should be between a-z, A-Z, or 0-9. @"|[\000-\052]|[\074-\077]" + // Invalid characers between oct 000-052, and oct 074-077. "|^((?!@).)*$" + // Must contain an '@' character. "|(@.*){2}" + // Must not contain more than one '@' character. "|@[^.]*$" + // Must contain at least one '.' character after the '@' character. "|~" + // Invalid '~' character. @"|\.@" + // First character before the '@' must not be '.' character. @"|@\." + // First character after the '@' must not be '.' character. @"|;|:|,|\^|\[|\]|\\|\{|\}|`|\t" + // Must not contain various invalid characters. @"|[\177-\377]" + // Invalid characers between oct 177-377. @"|\/|\.\.|^\.|\.$|@@"; // Must not contain ' / ', '..', '@@' or end with '.' character. pattern += string.IsNullOrWhiteSpace(optionsPattern) ? string.Empty : $"|{optionsPattern}"; var regex = new System.Text.RegularExpressions.Regex(pattern, System.Text.RegularExpressions.RegexOptions.IgnoreCase); var match = regex.Match(emailAddress); if (match.Success) { //System.Diagnostics.Debug.Print("Email address '" + emailAddress + "' contains invalid char/string '" + match.Value + "'."); return false; } return true; } public class Options { private Dictionary<string, object> data { get; set; } = new Dictionary<string, object>(); public Options() { var properties = new string[] { nameof(InvalidDomains), nameof(InvalidTopLevelDomains), nameof(InvalidSecondLevelDomains), nameof(Pattern) }; foreach (var prop in properties) { data.Add(prop, null); } } /// <summary> /// Invalid domains. Example: gmail.com /// </summary> public IEnumerable<string> InvalidDomains { get { return GetData<IEnumerable<string>>(nameof(InvalidDomains)); } set { SetData(nameof(InvalidDomains), value); ResetPattern(); } } /// <summary> /// Invalid top level domains. Example: .com /// </summary> public IEnumerable<string> InvalidTopLevelDomains { get { return GetData<IEnumerable<string>>(nameof(InvalidTopLevelDomains)); } set { SetData(nameof(InvalidTopLevelDomains), value); ResetPattern(); } } /// <summary> /// Invalid second level domains. Example: gmail /// </summary> public IEnumerable<string> InvalidSecondLevelDomains { get { return GetData<IEnumerable<string>>(nameof(InvalidSecondLevelDomains)); } set { SetData(nameof(InvalidSecondLevelDomains), value); ResetPattern(); } } public string Pattern { get { if (GetData<object>(nameof(Pattern)) == null) { var options = new List<string>(); if (InvalidTopLevelDomains != null) { options.AddRange(InvalidTopLevelDomains.Select(domain => FormatTld(domain))); } if (InvalidSecondLevelDomains != null) { options.AddRange(InvalidSecondLevelDomains.Select(domain => FormatSld(domain))); } if (InvalidDomains != null) { options.AddRange(InvalidDomains.Select(domain => FormatDomain(domain))); } SetData(nameof(Pattern), string.Join("|", options)); } return GetData<string>(nameof(Pattern)); } } private T GetData<T>(string key) { return (T)data[key]; } private void SetData(string key, object value) { data[key] = value; } private void ResetPattern() { SetData(nameof(Pattern), null); } private string FormatTld(string domain) { if (string.IsNullOrWhiteSpace(domain)) { return domain; } return AddPeriodSlash(AddPeriod(AddDollar(domain))); } private string FormatSld(string domain) { if (string.IsNullOrWhiteSpace(domain)) { return domain; } return AddAt(domain); } private string FormatDomain(string domain) { if (string.IsNullOrWhiteSpace(domain)) { return domain; } return AddPeriodSlash(AddAt(domain)); } private string AddDollar(string domain) { if (!domain.EndsWith("$")) { domain = $"{domain}$"; } return domain; } private string AddAt(string domain) { if (!domain.StartsWith("@")) { domain = $"@{domain}"; } return domain; } private string AddPeriod(string domain) { if (!domain.StartsWith(".")) { domain = $".{domain}"; } return domain; } private string AddPeriodSlash(string domain) { var index = domain.LastIndexOf("."); if (index > -1) { domain = domain.Insert(index, @"\"); } return domain; } } } }// http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
// ============================================================================ // Author: Kenneth Perkins // Date: May 8, 2021 // Taken From: http://programmingnotes.org/ // File: Program.cs // Description: The following demonstrates the use of the Utils Namespace // ============================================================================ using System; using System.Diagnostics; using System.Collections.Generic; public class Program { static void Main(string[] args) { try { // Verify email var addresses = new List<string>() { "a@gmail.comm", "name@yah00.com", "_name@yah00.com", "name@again@example.com", "anystring@anystring.anystring", "prettyandsimple@example.com", "very.common@example.com", "disposable.style.email.with+symbol@example.com", "other.email-with-dash@example.com", "x@example.com", "\"much.more unusual\"@example.com", "\"very.unusual.@.unusual.com\"@example.com", "<< example-indeed@strange-example.com >>", "<< admin@mailserver1 >>", @"<< #!$%&\'*+-/=?^_`{}|~@example.org >>", "example@s.solutions", "user@com", "john.doe@example..com", "john..doe@example.com", "A@b@c@example.com" }; foreach (var email in addresses) { Display($"Email: {email}, Is Valid: {Utils.Email.IsValid(email)}"); } Display(""); // Verify email using the default rules with additional options var addresses2 = new List<string>() { "a@gmail.comm", "name@yah00.com", "prettyandsimple@bumpymail.com", "disposable@gold2world.com", "user@gold2world.biz" }; // Additional options var options = new Utils.Email.Options() { InvalidDomains = new[] { // IEnumerable of invalid domains. Example: gmail.com "aichyna.com", "gawab.com", "gold2world.biz", "front14.org", "bumpymail.com", "pleasantphoto.com", "spam.la" }, InvalidTopLevelDomains = new string[] { // IEnumerable of invalid top level domains. Example: .com "comm", "nett", "c0m" }, InvalidSecondLevelDomains = new List<string>() { // IEnumerable of invalid second level domains. Example: gmail "yah00", "ynail" } }; foreach (var email in addresses2) { Display($"Email: {email}, Is Valid: {Utils.Email.IsValid(email, options)}"); } } catch (Exception ex) { Display(ex.ToString()); } finally { Console.ReadLine(); } } static void Display(string message) { Console.WriteLine(message); Debug.Print(message); } }// http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Validate An Email Address Using VB.NET
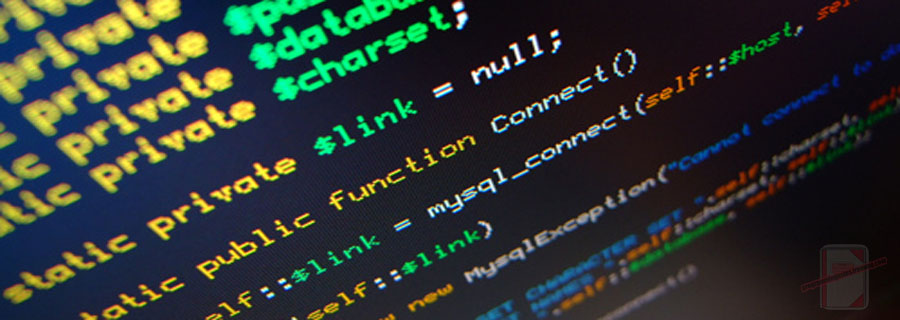
The following is a module with functions which demonstrates how to determine if an email address is valid using VB.NET.
This function is based on RFC2821 and RFC2822 to determine the validity of an email address.
1. Validate Email – Basic Usage
The example below demonstrates the use of ‘Utils.Email.IsValid‘ to determine if an email address is valid.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
' Validate Email Dim addresses = New List(Of String) From { "a@gmail.comm", "name@yah00.com", "_name@yah00.com", "name@again@example.com", "anystring@anystring.anystring", "prettyandsimple@example.com", "very.common@example.com", "disposable.style.email.with+symbol@example.com", "other.email-with-dash@example.com", "x@example.com", """much.more unusual""@example.com", """very.unusual.@.unusual.com""@example.com", "<< example-indeed@strange-example.com >>", "<< admin@mailserver1 >>", "<< #!$%&\'*+-/=?^_`{}|~@example.org >>", "example@s.solutions", "user@com", "john.doe@example..com", "john..doe@example.com", "A@b@c@example.com" } For Each email In addresses Debug.Print($"Email: {email}, Is Valid: {Utils.Email.IsValid(email)}") Next ' expected output: ' Email: a@gmail.comm, Is Valid: True ' Email: name@yah00.com, Is Valid: True ' Email: _name@yah00.com, Is Valid: False ' Email: name@again@example.com, Is Valid: False ' Email: anystring@anystring.anystring, Is Valid: True ' Email: prettyandsimple@example.com, Is Valid: True ' Email: very.common@example.com, Is Valid: True ' Email: disposable.style.email.with+symbol@example.com, Is Valid: True ' Email: other.email-with-dash@example.com, Is Valid: True ' Email: x@example.com, Is Valid: True ' Email: "much.more unusual"@example.com, Is Valid: False ' Email: "very.unusual.@.unusual.com"@example.com, Is Valid: False ' Email: << example-indeed@strange-example.com >>, Is Valid: False ' Email: << admin@mailserver1 >>, Is Valid: False ' Email: << #!$%&\'*+-/=?^_`{}|~@example.org >>, Is Valid: False ' Email: example@s.solutions, Is Valid: True ' Email: user@com, Is Valid: False ' Email: john.doe@example..com, Is Valid: False ' Email: john..doe@example.com, Is Valid: False ' Email: A@b@c@example.com, Is Valid: False |
2. Validate Email – Additional Options
The example below demonstrates the use of ‘Utils.Email.IsValid‘ to determine if an email address is valid with additional validation options.
Supplying options to verify an email address uses the default validity rules, in addition to the provided validation options.
This allows you to add custom rules to determine if an email is valid or not. For example, the misspelling of common email domains, or known spam domains.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
' Verify Email Using Default Rules With Additional Options Dim addresses = New List(Of String) From { "a@gmail.comm", "name@yah00.com", "prettyandsimple@bumpymail.com", "disposable@gold2world.com", "user@gold2world.biz" } ' Additional options Dim options = New Utils.Email.Options With { .InvalidDomains = { ' Optional: IEnumerable of invalid domains. Example: gmail.com "aichyna.com", "gawab.com", "gold2world.biz", "front14.org", "bumpymail.com", "pleasantphoto.com", "spam.la" }, .InvalidTopLevelDomains = New String() { ' Optional: IEnumerable of invalid top level domains. Example: .com "comm", "nett", "c0m" }, .InvalidSecondLevelDomains = New List(Of String) From { ' Optional: IEnumerable of invalid second level domains. Example: gmail "yah00", "ynail", "gnail" } } For Each email In addresses Debug.Print($"Email: {email}, Is Valid: {Utils.Email.IsValid(email, options)}") Next ' expected output: ' Email: a@gmail.comm, Is Valid: False ' Email: name@yah00.com, Is Valid: False ' Email: prettyandsimple@bumpymail.com, Is Valid: False ' Email: disposable@gold2world.com, Is Valid: True ' Email: user@gold2world.biz, Is Valid: False |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 4, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils Public MustInherit Class Email ''' <summary> ''' Based on RFC2821 and RFC2822 to determine the validity of an ''' email address ''' </summary> ''' <param name="emailAddress">The email address to verify</param> ''' <param name="options">Additional email restrictions</param> ''' <returns>True if the email address is valid, false otherwise</returns> Public Shared Function IsValid(emailAddress As String, Optional options As Email.Options = Nothing) As Boolean If String.IsNullOrWhiteSpace(emailAddress) OrElse emailAddress.Length > 100 Then Return False End If Dim optionsPattern = If(options Is Nothing, String.Empty, options.Pattern) 'https://www.jochentopf.com/email/characters-in-email-addresses.pdf Dim pattern = "^[^a-zA-Z0-9]|[^a-zA-Z0-9]$" & ' First and last characters should be between a-z, A-Z, or 0-9. "|[\000-\052]|[\074-\077]" & ' Invalid characers between oct 000-052, and oct 074-077. "|^((?!@).)*$" & ' Must contain an '@' character. "|(@.*){2}" & ' Must not contain more than one '@' character. "|@[^.]*$" & ' Must contain at least one '.' character after the '@' character. "|~" & ' Invalid '~' character. "|\.@" & ' First character before the '@' must not be '.' character. "|@\." & ' First character after the '@' must not be '.' character. "|;|:|,|\^|\[|\]|\\|\{|\}|`|\t" & ' Must not contain various invalid characters. "|[\177-\377]" & ' Invalid characers between oct 177-377. "|\/|\.\.|^\.|\.$|@@" & ' Must not contain '/', '..', '@@' or end with '.' character. If(String.IsNullOrWhiteSpace(optionsPattern), String.Empty, $"|{optionsPattern}") Dim regex = New Text.RegularExpressions.Regex(pattern, Text.RegularExpressions.RegexOptions.IgnoreCase) Dim match = regex.Match(emailAddress) If match.Success Then 'Debug.Print("Email address '" & emailAddress & "' contains invalid char/string '" & match.Value & "'.") Return False End If Return True End Function Public Class Options Private Property data As New Dictionary(Of String, Object) Public Sub New() Dim properties = New String() { NameOf(InvalidDomains), NameOf(InvalidTopLevelDomains), NameOf(InvalidSecondLevelDomains), NameOf(Pattern) } For Each prop In properties data.Add(prop, Nothing) Next End Sub ''' <summary> ''' Invalid domains. Example: gmail.com ''' </summary> Public Property InvalidDomains As IEnumerable(Of String) Get Return GetData(Of IEnumerable(Of String))(NameOf(InvalidDomains)) End Get Set(value As IEnumerable(Of String)) SetData(NameOf(InvalidDomains), value) ResetPattern() End Set End Property ''' <summary> ''' Invalid top level domains. Example: .com ''' </summary> Public Property InvalidTopLevelDomains As IEnumerable(Of String) Get Return GetData(Of IEnumerable(Of String))(NameOf(InvalidTopLevelDomains)) End Get Set(value As IEnumerable(Of String)) SetData(NameOf(InvalidTopLevelDomains), value) ResetPattern() End Set End Property ''' <summary> ''' Invalid second level domains. Example: gmail ''' </summary> Public Property InvalidSecondLevelDomains As IEnumerable(Of String) Get Return GetData(Of IEnumerable(Of String))(NameOf(InvalidSecondLevelDomains)) End Get Set(value As IEnumerable(Of String)) SetData(NameOf(InvalidSecondLevelDomains), value) ResetPattern() End Set End Property Public ReadOnly Property Pattern As String Get If GetData(Of Object)(NameOf(Pattern)) Is Nothing Then Dim options = New List(Of String) If InvalidTopLevelDomains IsNot Nothing Then options.AddRange(InvalidTopLevelDomains.Select(Function(domain) FormatTld(domain))) End If If InvalidSecondLevelDomains IsNot Nothing Then options.AddRange(InvalidSecondLevelDomains.Select(Function(domain) FormatSld(domain))) End If If InvalidDomains IsNot Nothing Then options.AddRange(InvalidDomains.Select(Function(domain) FormatDomain(domain))) End If SetData(NameOf(Pattern), String.Join("|", options)) End If Return GetData(Of String)(NameOf(Pattern)) End Get End Property Private Function GetData(Of T)(key As String) As T Return CType(data(key), T) End Function Private Sub SetData(key As String, value As Object) data(key) = value End Sub Private Sub ResetPattern() SetData(NameOf(Pattern), Nothing) End Sub Private Function FormatTld(domain As String) As String If String.IsNullOrWhiteSpace(domain) Then Return domain End If Return AddPeriodSlash(AddPeriod(AddDollar(domain))) End Function Private Function FormatSld(domain As String) As String If String.IsNullOrWhiteSpace(domain) Then Return domain End If Return AddAt(domain) End Function Private Function FormatDomain(domain As String) As String If String.IsNullOrWhiteSpace(domain) Then Return domain End If Return AddPeriodSlash(AddAt(domain)) End Function Private Function AddDollar(domain As String) As String If Not domain.EndsWith("$") Then domain = $"{domain}$" End If Return domain End Function Private Function AddAt(domain As String) As String If Not domain.StartsWith("@") Then domain = $"@{domain}" End If Return domain End Function Private Function AddPeriod(domain As String) As String If Not domain.StartsWith(".") Then domain = $".{domain}" End If Return domain End Function Private Function AddPeriodSlash(domain As String) As String Dim index = domain.LastIndexOf(".") If index > -1 Then domain = domain.Insert(index, "\") End If Return domain End Function End Class End Class End Module End Namespace ' http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 4, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Module Program Sub Main(args As String()) Try ' Verify email Dim addresses = New List(Of String) From { "a@gmail.comm", "name@yah00.com", "_name@yah00.com", "name@again@example.com", "anystring@anystring.anystring", "prettyandsimple@example.com", "very.common@example.com", "disposable.style.email.with+symbol@example.com", "other.email-with-dash@example.com", "x@example.com", """much.more unusual""@example.com", """very.unusual.@.unusual.com""@example.com", "<< example-indeed@strange-example.com >>", "<< admin@mailserver1 >>", "<< #!$%&\'*+-/=?^_`{}|~@example.org >>", "example@s.solutions", "user@com", "john.doe@example..com", "john..doe@example.com", "A@b@c@example.com" } For Each email In addresses Display($"Email: {email}, Is Valid: {Utils.Email.IsValid(email)}") Next Display("") ' Verify email using the default rules with additional options Dim addresses2 = New List(Of String) From { "a@gmail.comm", "name@yah00.com", "prettyandsimple@bumpymail.com", "disposable@gold2world.com", "user@gold2world.biz" } ' Additional options Dim options = New Utils.Email.Options With { .InvalidDomains = { ' IEnumerable of invalid domains. Example: gmail.com "aichyna.com", "gawab.com", "gold2world.biz", "front14.org", "bumpymail.com", "pleasantphoto.com", "spam.la" }, .InvalidTopLevelDomains = New String() { ' IEnumerable of invalid top level domains. Example: .com "comm", "nett", "c0m" }, .InvalidSecondLevelDomains = New List(Of String) From { ' IEnumerable of invalid second level domains. Example: gmail "yah00", "ynail" } } For Each email In addresses2 Display($"Email: {email}, Is Valid: {Utils.Email.IsValid(email, options)}") Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.