VB.NET || How To Get, Add, Update & Remove Values From A URL Query String Using VB.NET
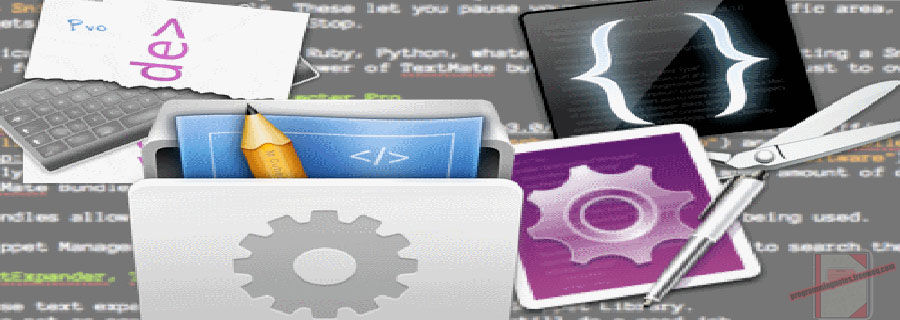
The following is a module with functions which demonstrates how to get, add, update and remove parameters from a query string using C#.
The following functions use System.Web to modify the url.
To use the functions in this module, make sure you have a reference to ‘System.Web‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add Reference‘, then type ‘System.Web‘ in the search box, and add the reference titled System.Web in the results Tab.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
1. Add – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Add‘ to add a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Add - Single Parameter ' Declare url Dim url = "http://programmingnotes.org/" ' Add single key value parameter to the url Dim result = Utils.HttpParams.Add(url, New KeyValuePair(Of String, String)("ids", "1987")) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=1987 |
2. Add – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Add‘ to add a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
' Add - Multiple Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Add multiple key value parameters to the url Dim addition = New System.Collections.Specialized.NameValueCollection() From { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result = Utils.HttpParams.Add(url, addition) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=1987%2c1991%2c2010%2c2019&names=Kenneth%2cJennifer%2cLynn%2cSole |
3. Update – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Update‘ to update a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Update - Single Parameter ' Declare url Dim url = "http://programmingnotes.org/?ids=1987" ' Update single key value parameter to the url Dim result = Utils.HttpParams.Update(url7, New KeyValuePair(Of String, String)("ids", "1991")) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=1991 |
4. Update – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Update‘ to update a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
' Update - Multiple Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Update multiple key value parameters to the url Dim addition = New System.Collections.Specialized.NameValueCollection() From { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result = Utils.HttpParams.Update(url, addition) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=2010%2c2019&names=Lynn%2cSole |
5. Remove – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Remove‘ to remove a query string parameter from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Remove - Single Parameter ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove single parameter from the url Dim result = Utils.HttpParams.Remove(url, "names") ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=1987%2c1991 |
6. Remove – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Remove‘ to remove multiple query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Remove - Multiple Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove multiple parameters from the url Dim result = Utils.HttpParams.Remove(url, New String() {"ids", "names"}) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/ |
7. Clear – Remove All Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Clear‘ to remove all query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Clear - Remove All Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove multiple parameters from the url Dim result = Utils.HttpParams.Clear(url) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/ |
8. Get – Get All Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Get‘ to get all query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
' Get - Get All Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991,2010,2019&names=Kenneth,Jennifer,Lynn,Sole" ' Get parameters from the url Dim result = Utils.HttpParams.Get(url) ' Display result For Each key As String In result Debug.Print($"Parameter: {key}, Value: {result(key)}") Next ' expected output: ' Parameter: ids, Value: 1987,1991,2010,2019 ' Parameter: names, Value: Kenneth,Jennifer,Lynn,Sole |
9. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 17, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace HttpParams Public Module modHttpParams ''' <summary> ''' Adds the query string parameters to a url ''' </summary> ''' <param name="url">The url to append parameters</param> ''' <param name="query">The parameters to add to the url</param> ''' <returns>The url appended with the specified query</returns> Function Add(url As String, query As System.Collections.Specialized.NameValueCollection) As String Return UpdateQuery(url, Sub(queryString) Dim merged = New System.Collections.Specialized.NameValueCollection From { queryString, query } CopyProps(merged, queryString) End Sub) End Function ''' <summary> ''' Adds the query string parameters to a url ''' </summary> ''' <param name="url">The url to append parameters</param> ''' <param name="param">The parameters to add to the url</param> ''' <returns>The url appended with the specified query</returns> Function Add(url As String, param As KeyValuePair(Of String, String)) As String Return Add(url, New System.Collections.Specialized.NameValueCollection() From { {param.Key, param.Value} }) End Function ''' <summary> ''' Updates the query string parameters to a url ''' </summary> ''' <param name="url">The url to update parameters</param> ''' <param name="query">The parameters to update to the url</param> ''' <returns>The url updated with the specified query</returns> Function Update(url As String, query As System.Collections.Specialized.NameValueCollection) As String Return UpdateQuery(url, Sub(queryString) CopyProps(query, queryString) End Sub) End Function ''' <summary> ''' Updates the query string parameters to a url ''' </summary> ''' <param name="url">The url to update parameters</param> ''' <param name="param">The parameters to update to the url</param> ''' <returns>The url updated with the specified query</returns> Function Update(url As String, param As KeyValuePair(Of String, String)) As String Return Update(url, New System.Collections.Specialized.NameValueCollection() From { {param.Key, param.Value} }) End Function ''' <summary> ''' Removes the parameters with the matching keys from the query string of a url ''' </summary> ''' <param name="url">The url to remove parameters</param> ''' <param name="keys">The parameter keys to remove from the url</param> ''' <returns>The url with the parameters removed</returns> Function Remove(url As String, keys As IEnumerable(Of String)) As String Return UpdateQuery(url, Sub(queryString) For Each key In keys If queryString.AllKeys.Contains(key) Then queryString.Remove(key) End If Next End Sub) End Function ''' <summary> ''' Removes the parameter with the matching key from the query string of a url ''' </summary> ''' <param name="url">The url to remove a parameter</param> ''' <param name="key">The parameter key to remove from the url</param> ''' <returns>The url with the parameter removed</returns> Function Remove(url As String, key As String) As String Return Remove(url, New String() {key}) End Function ''' <summary> ''' Removes all parameters from the query string of a url ''' </summary> ''' <param name="url">The url to clear parameters</param> ''' <returns>The url with the parameters removed</returns> Function Clear(url As String) As String Return UpdateQuery(url, Sub(queryString) queryString.Clear() End Sub) End Function ''' <summary> ''' Returns all parameters from the query string of a url ''' </summary> ''' <param name="url">The url to get parameters</param> ''' <returns>The url parameters</returns> Function [Get](url As String) As System.Collections.Specialized.NameValueCollection Dim result As System.Collections.Specialized.NameValueCollection = Nothing UpdateQuery(url, Sub(queryString) result = queryString End Sub) Return result End Function ''' <summary> ''' Sets the query string parameters to a url ''' </summary> ''' <param name="url">The url to set parameters</param> ''' <param name="query">The parameters to set to the url</param> ''' <returns>The url set with the specified query</returns> Function [Set](url As String, query As System.Collections.Specialized.NameValueCollection) As String url = Clear(url) Return Add(url, query) End Function ''' <summary> ''' Sets the query string parameters to a url ''' </summary> ''' <param name="url">The url to set parameters</param> ''' <param name="param">The parameters to set to the url</param> ''' <returns>The url set with the specified query</returns> Function [Set](url As String, param As KeyValuePair(Of String, String)) As String Return [Set](url, New System.Collections.Specialized.NameValueCollection() From { {param.Key, param.Value} }) End Function Private Sub CopyProps(source As System.Collections.Specialized.NameValueCollection, destination As System.Collections.Specialized.NameValueCollection) For Each key As String In source destination(key) = source(key) Next End Sub Private Function UpdateQuery(url As String, modifyQuery As Action(Of System.Collections.Specialized.NameValueCollection)) As String Dim uriBuilder = New System.UriBuilder(url) Dim query = System.Web.HttpUtility.ParseQueryString(uriBuilder.Query) modifyQuery(query) uriBuilder.Query = query.ToString() Return uriBuilder.Uri.ToString() End Function End Module End Namespace End Namespace ' http://programmingnotes.org/ |
10. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 17, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Sub Main(args As String()) Try ' Declare url Dim url = "http://programmingnotes.org/" ' Add single key value parameter to the url Dim result = Utils.HttpParams.Add(url, New KeyValuePair(Of String, String)("ids", "1987")) ' Display result Display(result) Display("") ' Declare url Dim url2 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Add multiple key value parameters to the url Dim addition = New System.Collections.Specialized.NameValueCollection() From { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result2 = Utils.HttpParams.Add(url2, addition) ' Display result Display(result2) Display("") ' Declare url Dim url3 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove single parameter from the url Dim result3 = Utils.HttpParams.Remove(url3, "names") ' Display result Display(result3) Display("") ' Declare url Dim url4 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove multiple parameters from the url Dim result4 = Utils.HttpParams.Remove(url4, New String() {"ids", "names"}) ' Display result Display(result4) Display("") ' Declare url Dim url5 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove multiple parameters from the url Dim result5 = Utils.HttpParams.Clear(url5) ' Display result Display(result5) Display("") ' Declare url Dim url6 = "http://programmingnotes.org/?ids=1987,1991,2010,2019&names=Kenneth,Jennifer,Lynn,Sole" ' Get parameters from the url Dim result6 = Utils.HttpParams.Get(url6) ' Display result For Each key As String In result6 Display($"Parameter: {key}, Value: {result6(key)}") Next Display("") ' Declare url Dim url7 = "http://programmingnotes.org/?ids=1987" ' Update single key value parameter to the url Dim result7 = Utils.HttpParams.Update(url7, New KeyValuePair(Of String, String)("ids", "1991")) ' Display result Display(result7) Display("") ' Declare url Dim url8 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Update multiple key value parameters to the url Dim addition8 = New System.Collections.Specialized.NameValueCollection() From { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result8 = Utils.HttpParams.Update(url8, addition8) ' Display result Display(result8) Display("") ' Declare url Dim url9 = "http://programmingnotes.org/?ids=1987&names=Kenneth" ' Set multiple key value parameters to the url Dim addition9 = New System.Collections.Specialized.NameValueCollection() From { {"ids", "1991"}, {"ids", "2010"}, {"ids", "2019"}, {"names", "Jennifer"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result9 = Utils.HttpParams.Set(url9, addition9) ' Display result Display(result9) Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
Leave a Reply