Monthly Archives: November 2020
VB.NET || How To Copy All Properties & Fields From One Object To Another Using VB.NET
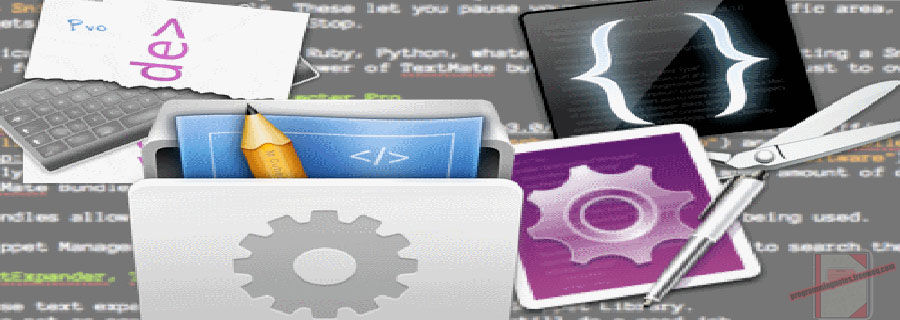
The following is a module with functions which demonstrates how to copy all properties and fields from one object to another using VB.NET.
The function demonstrated on this page is a generic extension method which uses reflection to copy all the matching properties and fields from one object to another.
This function works on both structure and class object fields and properties.
The two objects do not have to be the same type. Only the matching properties and fields are copied.
1. Copy Properties & Fields
The example below demonstrates the use of ‘Utils.Objects.CopyPropsTo‘ to copy all the matching properties and fields from the source object to the destination object.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
' Copy Properties & Fields Imports Utils.Objects Public Class Part Public Property PartName As String Public Property PartId As Integer End Class Public Structure Part2 Public Property PartName As String Public PartId As Integer End Structure ' Declare source object Dim part1 = New Part With { .PartName = "crank arm", .PartId = 1234 } ' Declare destination object Dim part2 = New Part2 ' Copy matching properties and fields to destination part1.CopyPropsTo(part2) ' Display information Debug.Print($"{part2.PartId} - {part2.PartName}") ' expected output: ' 1234 - crank arm |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 22, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace Objects Public Module modObjects ''' <summary> ''' Copies all the matching properties and fields from 'source' to 'destination' ''' </summary> ''' <param name="source">The source object to copy from</param> ''' <param name="destination">The destination object to copy to</param> <Runtime.CompilerServices.Extension()> Public Sub CopyPropsTo(Of T1, T2)(source As T1, ByRef destination As T2) Dim sourceMembers = GetMembers(source.GetType) Dim destinationMembers = GetMembers(destination.GetType) ' Copy data from source to destination For Each sourceMember In sourceMembers If Not CanRead(sourceMember) Then Continue For End If Dim destinationMember = destinationMembers.FirstOrDefault(Function(x) x.Name.ToLower = sourceMember.Name.ToLower) If destinationMember Is Nothing _ OrElse Not CanWrite(destinationMember) Then Continue For End If SetObjectValue(destination, destinationMember, GetMemberValue(source, sourceMember)) Next End Sub Private Sub SetObjectValue(Of T)(ByRef obj As T, member As System.Reflection.MemberInfo, value As Object) ' Boxing method used for modifying structures Dim boxed = If(obj.GetType.IsValueType, CType(obj, Object), obj) SetMemberValue(boxed, member, value) obj = CType(boxed, T) End Sub Private Sub SetMemberValue(Of T)(ByRef obj As T, member As System.Reflection.MemberInfo, value As Object) If IsProperty(member) Then Dim prop = CType(member, System.Reflection.PropertyInfo) If prop.SetMethod IsNot Nothing Then prop.SetValue(obj, value) End If ElseIf IsField(member) Then Dim field = CType(member, System.Reflection.FieldInfo) field.SetValue(obj, value) End If End Sub Private Function GetMemberValue(obj As Object, member As System.Reflection.MemberInfo) As Object Dim result As Object = Nothing If IsProperty(member) Then Dim prop = CType(member, System.Reflection.PropertyInfo) result = prop.GetValue(obj, If(prop.GetIndexParameters.Count = 1, New Object() {Nothing}, Nothing)) ElseIf IsField(member) Then Dim field = CType(member, System.Reflection.FieldInfo) result = field.GetValue(obj) End If Return result End Function Private Function CanWrite(member As System.Reflection.MemberInfo) As Boolean Return If(IsProperty(member), CType(member, System.Reflection.PropertyInfo).CanWrite, IsField(member)) End Function Private Function CanRead(member As System.Reflection.MemberInfo) As Boolean Return If(IsProperty(member), CType(member, System.Reflection.PropertyInfo).CanRead, IsField(member)) End Function Private Function IsProperty(member As System.Reflection.MemberInfo) As Boolean Return IsType(member.GetType, GetType(System.Reflection.PropertyInfo)) End Function Private Function IsField(member As System.Reflection.MemberInfo) As Boolean Return IsType(member.GetType, GetType(System.Reflection.FieldInfo)) End Function Private Function IsType(type As System.Type, targetType As System.Type) As Boolean Return type.Equals(targetType) OrElse type.IsSubclassOf(targetType) End Function Private Function GetMembers(type As System.Type) As List(Of System.Reflection.MemberInfo) Dim flags = System.Reflection.BindingFlags.Instance Or System.Reflection.BindingFlags.Public _ Or System.Reflection.BindingFlags.NonPublic Dim members = New List(Of System.Reflection.MemberInfo) members.AddRange(type.GetProperties(flags)) members.AddRange(type.GetFields(flags)) Return members End Function End Module End Namespace End Namespace ' http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 22, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Imports Utils.Objects Module Program Public Class Part Public Property PartName As String Public PartId As Integer? End Class Public Structure Part2 Public Property PartName As String Public PartId As Integer End Structure Sub Main(args As String()) Try ' Declare source object Dim part1 = New Part With { .PartName = "crank arm", .PartId = 1234 } ' Declare destination object Dim part2 = New Part2 ' Copy matching properties and fields to destination part1.CopyPropsTo(part2) ' Display information Display($"{part2.PartId} - {part2.PartName}") Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || Universal Object Serializer and Deserializer Using VB.NET
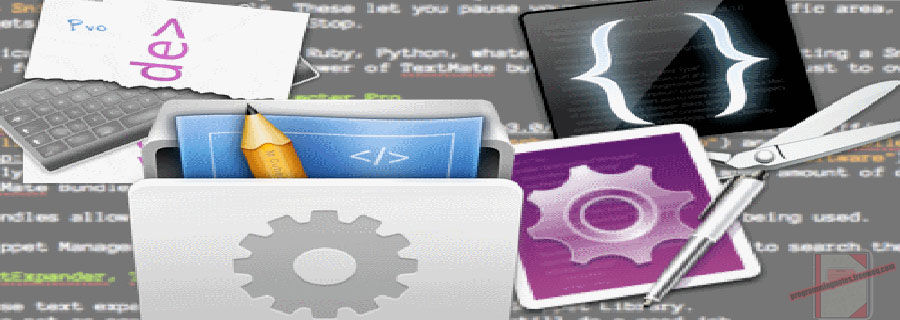
The following is a module with functions which demonstrates how to create a simple universal object serializer and deserializer using VB.NET.
The following functions take in a instance of the abstract classes ‘ObjectSerializer‘, and ‘ObjectDeserializer‘. Those classes defines the functionality that is common to all the classes derived from it. They are what carries out the actual serialization and deserialization.
Breaking things up like so makes it easy to have different serializations with only calling one function.
Note: The functions in this module uses code from previous articles which explains how to serialize and deserialize objects.
For those examples of how to serialize and deserialize objects using Json and XML, see below:
1. JsonSerializer
The example below demonstrates the use of ‘Utils.Objects.JsonSerializer‘ to serialize an object to Json.
By changing the second parameter, calling the same function will change its behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
' JsonSerializer Public Class Part Public Property PartName As String Public Property PartId As Integer End Class ' Declare list of objects Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} ' Serialize to json Dim serialized = Utils.Objects.Serialize(parts, New Utils.Objects.JsonSerializer) ' Display json Debug.Print(serialized) ' expected output: ' [ ' { ' "PartName": "crank arm", ' "PartId": 1234 ' }, ' { ' "PartName": "chain ring", ' "PartId": 1334 ' }, ' { ' "PartName": "regular seat", ' "PartId": 1434 ' }, ' { ' "PartName": "banana seat", ' "PartId": 1444 ' }, ' { ' "PartName": "cassette", ' "PartId": 1534 ' }, ' { ' "PartName": "shift lever", ' "PartId": 1634 ' } ' ] |
2. JsonDeserializer
The example below demonstrates the use of ‘Utils.Objects.JsonDeserializer‘ to deserialize an object from Json.
By changing the second parameter, calling the same function will change its behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
' JsonDeserializer ' Declare Json array of objects Dim partsJson = " [ { ""PartName"": ""crank arm"", ""PartId"": 1234 }, { ""PartName"": ""chain ring"", ""PartId"": 1334 }, { ""PartName"": ""regular seat"", ""PartId"": 1434 }, { ""PartName"": ""banana seat"", ""PartId"": 1444 }, { ""PartName"": ""cassette"", ""PartId"": 1534 }, { ""PartName"": ""shift lever"", ""PartId"": 1634 } ] " ' Deserialize to object Dim deserialized = Utils.Objects.Deserialize(Of List(Of Part))(partsJson, New Utils.Objects.JsonDeserializer) ' Display the items For Each item In deserialized Debug.Print($"{item.PartId} - {item.PartName}") Next ' expected output: ' 1234 - crank arm ' 1334 - chain ring ' 1434 - regular seat ' 1444 - banana seat ' 1534 - cassette ' 1634 - shift lever |
3. XmlSerializer
The example below demonstrates the use of ‘Utils.Objects.XmlSerializer‘ to serialize an object to Xml.
By changing the second parameter, calling the same function will change its behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
' XmlSerializer Public Class Part Public Property PartName As String Public Property PartId As Integer End Class ' Declare list of objects Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} ' Serialize to xml Dim serialized = Utils.Objects.Serialize(parts, New Utils.Objects.XmlSerializer) ' Display xml Debug.Print(serialized) ' expected output: ' <?xml version="1.0"?> ' <ArrayOfPart xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> ' <Part> ' <PartName>crank arm</PartName> ' <PartId>1234</PartId> ' </Part> ' <Part> ' <PartName>chain ring</PartName> ' <PartId>1334</PartId> ' </Part> ' <Part> ' <PartName>regular seat</PartName> ' <PartId>1434</PartId> ' </Part> ' <Part> ' <PartName>banana seat</PartName> ' <PartId>1444</PartId> ' </Part> ' <Part> ' <PartName>cassette</PartName> ' <PartId>1534</PartId> ' </Part> ' <Part> ' <PartName>shift lever</PartName> ' <PartId>1634</PartId> ' </Part> ' </ArrayOfPart> |
4. XmlDeserializer
The example below demonstrates the use of ‘Utils.Objects.XmlDeserializer‘ to deserialize an object from xml.
By changing the second parameter, calling the same function will change its behavior.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
' XmlDeserializer ' Declare Xml array of objects Dim partsXml = $"<?xml version=""1.0""?> <ArrayOfPart xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </ArrayOfPart> " ' Deserialize to object Dim deserialized = Utils.Objects.Deserialize(Of List(Of Part))(partsXml, New Utils.Objects.XmlDeserializer) ' Display the items For Each item In deserialized Debug.Print($"{item.PartId} - {item.PartName}") Next ' expected output: ' 1234 - crank arm ' 1334 - chain ring ' 1434 - regular seat ' 1444 - banana seat ' 1534 - cassette ' 1634 - shift lever |
5. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 20, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace Objects Public Module modObjects ''' <summary> ''' Serializes the specified value according to the ObjectSerializer ''' </summary> ''' <param name="value">The value to serialize</param> ''' <param name="serializer">Determines how the value should be serialized</param> ''' <returns>The value serialized according to the ObjectSerializer</returns> Public Function Serialize(Of T)(value As T, serializer As ObjectSerializer) As String Return serializer.Serialize(value) End Function ''' <summary> ''' Deserializes the specified value according to the ObjectDeserializer ''' </summary> ''' <param name="value">The value to deserialize</param> ''' <param name="deserializer">Determines how the value should be deserialized</param> ''' <returns>The value deserialized according to the ObjectSerializer</returns> Public Function Deserialize(Of T)(value As String, deserializer As ObjectDeserializer) As T Return deserializer.Deserialize(Of T)(value) End Function End Module #Region "ObjectSerializer" ''' <summary> ''' Defines the object serializer behavior ''' </summary> Public MustInherit Class ObjectSerializer Public MustOverride Function Serialize(Of T)(value As T) As String End Class Public Class JsonSerializer Inherits ObjectSerializer Public Property settings As New Newtonsoft.Json.JsonSerializerSettings Public Sub New() settings.NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore settings.Formatting = Newtonsoft.Json.Formatting.Indented End Sub Public Overrides Function Serialize(Of T)(value As T) As String Return Utils.Json.Serialize(value, settings) End Function End Class Public Class XmlSerializer Inherits ObjectSerializer Public Property root As New System.Xml.Serialization.XmlRootAttribute Public Overrides Function Serialize(Of T)(value As T) As String Return Utils.Xml.Serialize(value, root) End Function End Class #End Region #Region "ObjectDeserializer" ''' <summary> ''' Defines the object deserializer behavior ''' </summary> Public MustInherit Class ObjectDeserializer Public MustOverride Function Deserialize(Of T)(value As String) As T End Class Public Class JsonDeserializer Inherits ObjectDeserializer Public Property settings As New Newtonsoft.Json.JsonSerializerSettings Public Sub New() settings.NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore End Sub Public Overrides Function Deserialize(Of T)(value As String) As T Dim obj As T = Nothing If Not String.IsNullOrEmpty(value) Then obj = Utils.Json.Deserialize(Of T)(value, settings) End If Return obj End Function End Class Public Class XmlDeserializer Inherits ObjectDeserializer Public Property root As New System.Xml.Serialization.XmlRootAttribute Public Overrides Function Deserialize(Of T)(value As String) As T Dim obj As T = Nothing If Not String.IsNullOrEmpty(value) Then obj = Utils.Xml.Deserialize(Of T)(value, root) End If Return obj End Function End Class #End Region End Namespace End Namespace ' http://programmingnotes.org/ |
6. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 20, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Public Class Part Public Property PartName As String Public Property PartId As Integer End Class Sub Main(args As String()) Try ' Declare list of objects Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} ' Serialize to json Dim serialized = Utils.Objects.Serialize(parts, New Utils.Objects.JsonSerializer) ' Display json Display(serialized) ' Declare Json array of objects Dim partsJson = " [ { ""PartName"": ""crank arm"", ""PartId"": 1234 }, { ""PartName"": ""chain ring"", ""PartId"": 1334 }, { ""PartName"": ""regular seat"", ""PartId"": 1434 }, { ""PartName"": ""banana seat"", ""PartId"": 1444 }, { ""PartName"": ""cassette"", ""PartId"": 1534 }, { ""PartName"": ""shift lever"", ""PartId"": 1634 } ] " ' Deserialize to object Dim deserialized = Utils.Objects.Deserialize(Of List(Of Part))(partsJson, New Utils.Objects.JsonDeserializer) ' Display the items For Each item In deserialized Display($"{item.PartId} - {item.PartName}") Next ' Serialize to xml Dim serialized2 = Utils.Objects.Serialize(parts, New Utils.Objects.XmlSerializer) ' Display xml Display(serialized2) ' Declare Xml array of objects Dim partsXml = $"<?xml version=""1.0""?> <ArrayOfPart xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </ArrayOfPart> " ' Deserialize to object Dim deserialized2 = Utils.Objects.Deserialize(Of List(Of Part))(partsXml, New Utils.Objects.XmlDeserializer) ' Display the items For Each item In deserialized2 Display($"{item.PartId} - {item.PartName}") Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Send, Post & Process A REST API Web Request Using VB.NET
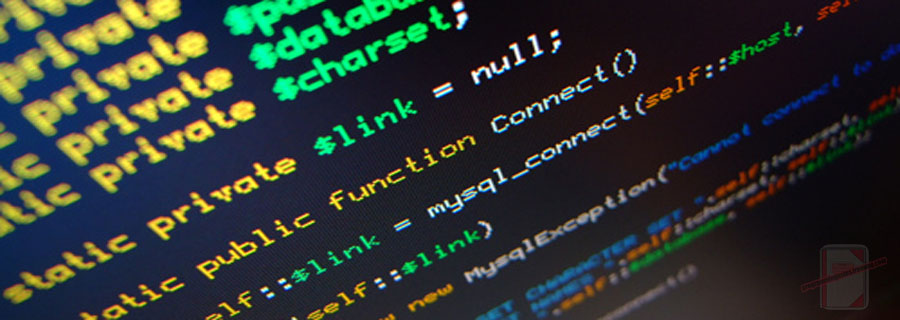
The following is a module with functions which demonstrates how to send and receive a RESTful web request using VB.NET.
Contents
1. Overview
2. WebRequest - GET
3. WebRequest - POST
4. WebRequest - PUT
5. WebRequest - PATCH
6. WebRequest - DELETE
7. Utils Namespace
8. More Examples
1. Overview
The following functions use System.Net.HttpWebRequest and System.Net.HttpWebResponse to send and process requests. They can be called synchronously or asynchronously. This page will demonstrate using the asynchronous function calls.
The examples on this page will call a test API, and the resulting calls will return Json results.
The Json objects we are sending to the API are hard coded in the examples below. In a real world application, the objects would be serialized first before they are sent over the network, and then deserialized once a response is received. For simplicity, those operations are hard coded.
For examples of how to serialize and deserialize objects using Json and XML, see below:
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
2. WebRequest – GET
The example below demonstrates the use of ‘Utils.WebRequest.Get‘ to execute a GET request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 |
' WebRequest - GET ' Declare url Dim url = "https://jsonplaceholder.typicode.com/users" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Execute a get request at the following url Dim response = Await Utils.WebRequest.GetAsync(url) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 200 - OK ' Bytes Received: 5645 ' Body: [ ' { ' "id": 1, ' "name": "Leanne Graham", ' "username": "Bret", ' "email": "Sincere@april.biz", ' "address": { ' "street": "Kulas Light", ' "suite": "Apt. 556", ' "city": "Gwenborough", ' "zipcode": "92998-3874", ' "geo": { ' "lat": "-37.3159", ' "lng": "81.1496" ' } ' }, ' "phone": "1-770-736-8031 x56442", ' "website": "hildegard.org", ' "company": { ' "name": "Romaguera-Crona", ' "catchPhrase": "Multi-layered client-server neural-net", ' "bs": "harness real-time e-markets" ' } ' }, ' { ' "id": 2, ' "name": "Ervin Howell", ' "username": "Antonette", ' "email": "Shanna@melissa.tv", ' "address": { ' "street": "Victor Plains", ' "suite": "Suite 879", ' "city": "Wisokyburgh", ' "zipcode": "90566-7771", ' "geo": { ' "lat": "-43.9509", ' "lng": "-34.4618" ' } ' }, ' "phone": "010-692-6593 x09125", ' "website": "anastasia.net", ' "company": { ' "name": "Deckow-Crist", ' "catchPhrase": "Proactive didactic contingency", ' "bs": "synergize scalable supply-chains" ' } ' }, ' { ' "id": 3, ' "name": "Clementine Bauch", ' "username": "Samantha", ' "email": "Nathan@yesenia.net", ' "address": { ' "street": "Douglas Extension", ' "suite": "Suite 847", ' "city": "McKenziehaven", ' "zipcode": "59590-4157", ' "geo": { ' "lat": "-68.6102", ' "lng": "-47.0653" ' } ' }, ' "phone": "1-463-123-4447", ' "website": "ramiro.info", ' "company": { ' "name": "Romaguera-Jacobson", ' "catchPhrase": "Face to face bifurcated interface", ' "bs": "e-enable strategic applications" ' } ' }, ' { ' "id": 4, ' "name": "Patricia Lebsack", ' "username": "Karianne", ' "email": "Julianne.OConner@kory.org", ' "address": { ' "street": "Hoeger Mall", ' "suite": "Apt. 692", ' "city": "South Elvis", ' "zipcode": "53919-4257", ' "geo": { ' "lat": "29.4572", ' "lng": "-164.2990" ' } ' }, ' "phone": "493-170-9623 x156", ' "website": "kale.biz", ' "company": { ' "name": "Robel-Corkery", ' "catchPhrase": "Multi-tiered zero tolerance productivity", ' "bs": "transition cutting-edge web services" ' } ' }, ' { ' "id": 5, ' "name": "Chelsey Dietrich", ' "username": "Kamren", ' "email": "Lucio_Hettinger@annie.ca", ' "address": { ' "street": "Skiles Walks", ' "suite": "Suite 351", ' "city": "Roscoeview", ' "zipcode": "33263", ' "geo": { ' "lat": "-31.8129", ' "lng": "62.5342" ' } ' }, ' "phone": "(254)954-1289", ' "website": "demarco.info", ' "company": { ' "name": "Keebler LLC", ' "catchPhrase": "User-centric fault-tolerant solution", ' "bs": "revolutionize end-to-end systems" ' } ' }, ' { ' "id": 6, ' "name": "Mrs. Dennis Schulist", ' "username": "Leopoldo_Corkery", ' "email": "Karley_Dach@jasper.info", ' "address": { ' "street": "Norberto Crossing", ' "suite": "Apt. 950", ' "city": "South Christy", ' "zipcode": "23505-1337", ' "geo": { ' "lat": "-71.4197", ' "lng": "71.7478" ' } ' }, ' "phone": "1-477-935-8478 x6430", ' "website": "ola.org", ' "company": { ' "name": "Considine-Lockman", ' "catchPhrase": "Synchronised bottom-line interface", ' "bs": "e-enable innovative applications" ' } ' }, ' { ' "id": 7, ' "name": "Kurtis Weissnat", ' "username": "Elwyn.Skiles", ' "email": "Telly.Hoeger@billy.biz", ' "address": { ' "street": "Rex Trail", ' "suite": "Suite 280", ' "city": "Howemouth", ' "zipcode": "58804-1099", ' "geo": { ' "lat": "24.8918", ' "lng": "21.8984" ' } ' }, ' "phone": "210.067.6132", ' "website": "elvis.io", ' "company": { ' "name": "Johns Group", ' "catchPhrase": "Configurable multimedia task-force", ' "bs": "generate enterprise e-tailers" ' } ' }, ' { ' "id": 8, ' "name": "Nicholas Runolfsdottir V", ' "username": "Maxime_Nienow", ' "email": "Sherwood@rosamond.me", ' "address": { ' "street": "Ellsworth Summit", ' "suite": "Suite 729", ' "city": "Aliyaview", ' "zipcode": "45169", ' "geo": { ' "lat": "-14.3990", ' "lng": "-120.7677" ' } ' }, ' "phone": "586.493.6943 x140", ' "website": "jacynthe.com", ' "company": { ' "name": "Abernathy Group", ' "catchPhrase": "Implemented secondary concept", ' "bs": "e-enable extensible e-tailers" ' } ' }, ' { ' "id": 9, ' "name": "Glenna Reichert", ' "username": "Delphine", ' "email": "Chaim_McDermott@dana.io", ' "address": { ' "street": "Dayna Park", ' "suite": "Suite 449", ' "city": "Bartholomebury", ' "zipcode": "76495-3109", ' "geo": { ' "lat": "24.6463", ' "lng": "-168.8889" ' } ' }, ' "phone": "(775)976-6794 x41206", ' "website": "conrad.com", ' "company": { ' "name": "Yost and Sons", ' "catchPhrase": "Switchable contextually-based project", ' "bs": "aggregate real-time technologies" ' } ' }, ' { ' "id": 10, ' "name": "Clementina DuBuque", ' "username": "Moriah.Stanton", ' "email": "Rey.Padberg@karina.biz", ' "address": { ' "street": "Kattie Turnpike", ' "suite": "Suite 198", ' "city": "Lebsackbury", ' "zipcode": "31428-2261", ' "geo": { ' "lat": "-38.2386", ' "lng": "57.2232" ' } ' }, ' "phone": "024-648-3804", ' "website": "ambrose.net", ' "company": { ' "name": "Hoeger LLC", ' "catchPhrase": "Centralized empowering task-force", ' "bs": "target end-to-end models" ' } ' } ' ] |
3. WebRequest – POST
The example below demonstrates the use of ‘Utils.WebRequest.Post‘ to execute a POST request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
' WebRequest - POST ' Declare url Dim url = $"https://jsonplaceholder.typicode.com/users" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to create a new employee Dim payload = " { ""id"": 0, ""name"": ""Kenneth Perkins"", ""username"": null, ""email"": null, ""address"": null, ""phone"": null, ""website"": null, ""company"": null } " ' Execute a post request at the following url Dim response = Await Utils.WebRequest.PostAsync(url, payload, options) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 201 - Created ' Bytes Received: 154 ' Body: { ' "id": 11, ' "name": "Kenneth Perkins", ' "username": null, ' "email": null, ' "address": null, ' "phone": null, ' "website": null, ' "company": null ' } |
4. WebRequest – PUT
The example below demonstrates the use of ‘Utils.WebRequest.Put‘ to execute a PUT request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
' WebRequest - PUT ' Declare url Dim url = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to update an existing employee Dim payload = " { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } " ' Execute a put request at the following url Dim response = Await Utils.WebRequest.PutAsync(url, payload, options) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 200 - OK ' Bytes Received: 87 ' Body: { ' "name": "Kenneth Perkins", ' "website": "https://www.programmingnotes.org/", ' "id": 1 ' } |
5. WebRequest – PATCH
The example below demonstrates the use of ‘Utils.WebRequest.Patch‘ to execute a PATCH request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
' WebRequest - PATCH ' Declare url Dim url = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to update an existing employee Dim payload = " { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } " ' Execute a patch request at the following url Dim response = Await Utils.WebRequest.PatchAsync(url, payload, options) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 200 - OK ' Bytes Received: 526 ' Body: { ' "id": 1, ' "name": "Kenneth Perkins", ' "username": "Bret", ' "email": "Sincere@april.biz", ' "address": { ' "street": "Kulas Light", ' "suite": "Apt. 556", ' "city": "Gwenborough", ' "zipcode": "92998-3874", ' "geo": { ' "lat": "-37.3159", ' "lng": "81.1496" ' } ' }, ' "phone": "1-770-736-8031 x56442", ' "website": "https://www.programmingnotes.org/", ' "company": { ' "name": "Romaguera-Crona", ' "catchPhrase": "Multi-layered client-server neural-net", ' "bs": "harness real-time e-markets" ' } ' } |
6. WebRequest – DELETE
The example below demonstrates the use of ‘Utils.WebRequest.Delete‘ to execute a DELETE request on the given url.
The optional function parameter allows you to specify System.Net.HttpWebRequest options, like the UserAgent, Headers etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
' WebRequest - DELETE ' Declare url Dim url = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Execute a delete request at the following url Dim response = Await Utils.WebRequest.DeleteAsync(url, options) ' Display the status code and response body Debug.Print($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") ' .... Do something with response.Body like deserialize it to an object ' expected output: ' Status: 200 - OK ' Bytes Received: 2 ' Body: {} |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 18, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Imports System Imports System.Linq Imports System.Threading.Tasks Namespace Global.Utils Namespace WebRequest Public Module modRequest ''' <summary> ''' Executes a GET request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function [Get](url As String _ , Optional options As Options = Nothing) As Response Return GetAsync(url, options:=options).Result End Function ''' <summary> ''' Executes a GET request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function GetAsync(url As String _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Get, url, payload:=CType(Nothing, Byte()), options:=options) End Function ''' <summary> ''' Executes a POST request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to post to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Post(url As String, payload As String _ , Optional options As Options = Nothing) As Response Return Post(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a POST request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to post to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Post(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Response Return PostAsync(url, payload:=payload, options:=options).Result End Function ''' <summary> ''' Executes a POST request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to post to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PostAsync(url As String, payload As String _ , Optional options As Options = Nothing) As Task(Of Response) Return PostAsync(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a POST request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to post to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PostAsync(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Post, url, payload:=payload, options:=options) End Function ''' <summary> ''' Executes a PUT request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to put to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Put(url As String, payload As String _ , Optional options As Options = Nothing) As Response Return Put(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a PUT request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to put to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Put(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Response Return PutAsync(url, payload:=payload, options:=options).Result End Function ''' <summary> ''' Executes a PUT request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to put to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PutAsync(url As String, payload As String _ , Optional options As Options = Nothing) As Task(Of Response) Return PutAsync(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a PUT request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to put to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PutAsync(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Put, url, payload:=payload, options:=options) End Function ''' <summary> ''' Executes a PATCH request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to patch to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Patch(url As String, payload As String _ , Optional options As Options = Nothing) As Response Return Patch(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a PATCH request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to patch to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Patch(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Response Return PatchAsync(url, payload:=payload, options:=options).Result End Function ''' <summary> ''' Executes a PATCH request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to patch to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PatchAsync(url As String, payload As String _ , Optional options As Options = Nothing) As Task(Of Response) Return PatchAsync(url, payload:=payload.GetBytes, options:=options) End Function ''' <summary> ''' Executes a PATCH request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to patch to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function PatchAsync(url As String, payload As Byte() _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Patch, url, payload:=payload, options:=options) End Function ''' <summary> ''' Executes a DELETE request on the given url ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function Delete(url As String _ , Optional options As Options = Nothing) As Response Return DeleteAsync(url, options:=options).Result End Function ''' <summary> ''' Executes a DELETE request on the given url as an asynchronous operation ''' </summary> ''' <param name="url">The url to navigate to</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function DeleteAsync(url As String _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(Method.Delete, url, payload:=CType(Nothing, Byte()), options:=options) End Function ''' <summary> ''' Executes a request method on the given url as an asynchronous operation ''' </summary> ''' <param name="type">The type of request method to execute</param> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to send to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Function ExecuteAsync(type As Method _ , url As String _ , Optional payload As String = Nothing _ , Optional options As Options = Nothing) As Task(Of Response) Return ExecuteAsync(type, url, payload:=CType(payload?.GetBytes, Byte()), options:=options) End Function ''' <summary> ''' Executes a request method on the given url as an asynchronous operation ''' </summary> ''' <param name="type">The type of request method to execute</param> ''' <param name="url">The url to navigate to</param> ''' <param name="payload">The data to send to the specified resource</param> ''' <param name="options">The options for the web request</param> ''' <returns>The result of the given request</returns> Public Async Function ExecuteAsync(type As Method _ , url As String _ , Optional payload As Byte() = Nothing _ , Optional options As Options = Nothing) As Task(Of Response) Dim request = CType(System.Net.WebRequest.Create(url), System.Net.HttpWebRequest) If options IsNot Nothing Then request.CopyProperties(options) End If request.Method = type.ToString.ToUpper If payload IsNot Nothing Then request.ContentLength = payload.Length Using requestStream = request.GetRequestStream Using payloadStream = New System.IO.MemoryStream(payload) payloadStream.CopyTo(requestStream) End Using End Using End If Dim webResponse As System.Net.HttpWebResponse = Nothing Try webResponse = CType(Await request.GetResponseAsync(), System.Net.HttpWebResponse) Catch ex As System.Net.WebException If ex.Response Is Nothing Then Throw End If webResponse = CType(ex.Response, System.Net.HttpWebResponse) Catch ex As Exception Throw End Try Dim result = New Response With { .Result = webResponse, .Bytes = GetBytes(webResponse) } Return result End Function Private Function GetBytes(response As System.Net.HttpWebResponse) As Byte() Dim bytes As Byte() Dim responseStream = response.GetResponseStream() Using memoryStream = New System.IO.MemoryStream responseStream.CopyTo(memoryStream) bytes = memoryStream.ToArray End Using Return bytes End Function <Runtime.CompilerServices.Extension()> Public Function GetBytes(str As String, Optional encode As System.Text.Encoding = Nothing) As Byte() If encode Is Nothing Then encode = GetDefaultEncoding() End If Return encode.GetBytes(str) End Function <Runtime.CompilerServices.Extension()> Public Function GetString(bytes As Byte(), Optional encode As System.Text.Encoding = Nothing) As String If encode Is Nothing Then encode = GetDefaultEncoding() End If Return encode.GetString(bytes) End Function Private Function GetDefaultEncoding() As System.Text.Encoding Static encode As New System.Text.UTF8Encoding Return encode End Function <Runtime.CompilerServices.Extension()> Private Sub CopyProperties(Of T1, T2)(dest As T1, src As T2) Dim srcProps = src.GetType().GetProperties() Dim destProps = dest.GetType().GetProperties() For Each srcProp In srcProps If srcProp.CanRead Then Dim destProp = destProps.FirstOrDefault(Function(x) x.Name = srcProp.Name) If destProp IsNot Nothing AndAlso destProp.CanWrite Then Dim value = srcProp.GetValue(src, If(srcProp.GetIndexParameters.Count = 1, New Object() {Nothing}, Nothing)) destProp.SetValue(dest, value) End If End If Next End Sub End Module ''' <summary> ''' The response result of a <see cref="System.Net.HttpWebRequest"/> ''' </summary> Public Class Response Public Property Result As System.Net.HttpWebResponse = Nothing Public Property Bytes As Byte() = Nothing Public ReadOnly Property Body As String Get If _body Is Nothing AndAlso Bytes IsNot Nothing Then _body = Bytes.GetString() End If Return _body End Get End Property Private _body As String = Nothing End Class ''' <summary> ''' Options for the given <see cref="System.Net.HttpWebRequest"/> ''' </summary> Public Class Options Public Property Headers As New System.Net.WebHeaderCollection Public Property Credentials As System.Net.ICredentials = Nothing Public Property Connection As String = Nothing Public Property KeepAlive As Boolean = True Public Property Expect As String = Nothing Public Property IfModifiedSince As Date Public Property TransferEncoding As String Public Property Accept As String = Nothing Public Property AllowAutoRedirect As Boolean = True Public Property AllowReadStreamBuffering As Boolean = False Public Property AllowWriteStreamBuffering As Boolean = True Public Property MaximumAutomaticRedirections As Integer = 50 Public Property MediaType As String = Nothing Public Property Pipelined As Boolean = True Public Property PreAuthenticate As Boolean = False Public Property Referer As String = Nothing Public Property SendChunked As Boolean = False Public Property UseDefaultCredentials As Boolean = False Public Property UserAgent As String = Nothing Public Property ContentType As String = Nothing Public Property Timeout As Integer = 100000 Public Property ReadWriteTimeout As Integer = 300000 End Class Public MustInherit Class ContentType Public Const ApplicationUrlEncoded As String = "application/x-www-form-urlencoded" Public Const ApplicationJson As String = "application/json" Public Const TextXml As String = "text/xml" End Class Public Enum Method [Get] Post Put Patch Delete End Enum End Namespace End Namespace ' http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 18, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Sub Main(args As String()) Try ProcessRequests().Wait() Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Async Function ProcessRequests() As Task ' Declare url Dim url = "https://jsonplaceholder.typicode.com/users" ' Optional: Specify request options Dim options = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Execute a get request at the following url Dim response = Await Utils.WebRequest.GetAsync(url) ' Display the status code and response body Display($" Status: {CInt(response.Result.StatusCode)} - {response.Result.StatusDescription} Bytes Received: {response.Bytes.Length} Body: {response.Body} ") Display("") ' Declare url Dim url2 = $"https://jsonplaceholder.typicode.com/users" ' Optional: Specify request options Dim options2 = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to get the Json to create a new employee Dim payload = " { ""id"": 0, ""name"": ""Kenneth Perkins"", ""username"": null, ""email"": null, ""address"": null, ""phone"": null, ""website"": null, ""company"": null } " ' Execute a post request at the following url Dim response2 = Await Utils.WebRequest.PostAsync(url2, payload, options2) ' Display the status code and response body Display($" Status: {CInt(response2.Result.StatusCode)} - {response2.Result.StatusDescription} Bytes Received: {response2.Bytes.Length} Body: {response2.Body} ") Display("") ' Declare url Dim url3 = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options3 = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to update an existing employee Dim payload3 = " { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } " ' Execute a put request at the following url Dim response3 = Await Utils.WebRequest.PutAsync(url3, payload3, options3) ' Display the status code and response body Display($" Status: {CInt(response3.Result.StatusCode)} - {response3.Result.StatusDescription} Bytes Received: {response3.Bytes.Length} Body: {response3.Body} ") Display("") ' Declare url Dim employeeId = 1 Dim url4 = $"https://jsonplaceholder.typicode.com/users/{employeeId}" ' Optional: Specify request options Dim options4 = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Execute a delete request at the following url Dim response4 = Await Utils.WebRequest.DeleteAsync(url4, options4) ' Display the status code and response body Display($" Status: {CInt(response4.Result.StatusCode)} - {response4.Result.StatusDescription} Bytes Received: {response4.Bytes.Length} Body: {response4.Body} ") Display("") ' Declare url Dim url5 = $"https://jsonplaceholder.typicode.com/users/1" ' Optional: Specify request options Dim options5 = New Utils.WebRequest.Options With { .UserAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:82.0) Gecko/20100101 Firefox/82.0", .ContentType = Utils.WebRequest.ContentType.ApplicationJson, .Headers = New System.Net.WebHeaderCollection From { {"key", "value"} } } ' Serialize object to Json to update an existing employee Dim payload5 = " { ""name"": ""Kenneth Perkins"", ""website"": ""https://www.programmingnotes.org/"" } " ' Execute a patch request at the following url Dim response5 = Await Utils.WebRequest.PatchAsync(url5, payload5, options5) ' Display the status code and response body Display($" Status: {CInt(response5.Result.StatusCode)} - {response5.Result.StatusDescription} Bytes Received: {response5.Bytes.Length} Body: {response5.Body} ") End Function Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Check If A String Is A Valid HTTP URL Using VB.NET
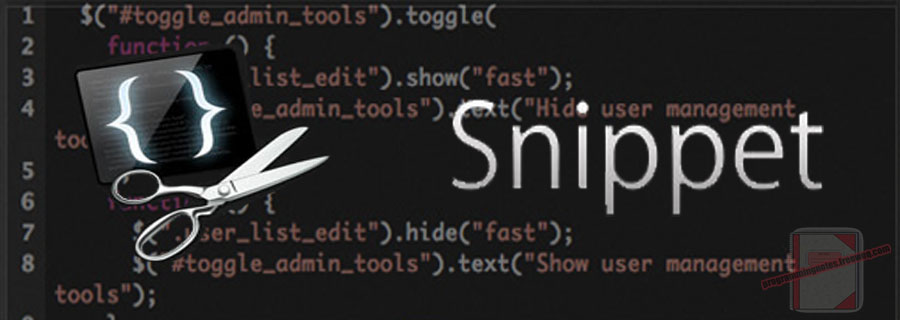
The following is a module with functions which demonstrates how to check whether a string is a valid HTTP URL using VB.NET.
Note: The following function only checks for valid url formatting. It does not determine if the address behind the url is valid for navigation.
1. Is Valid URL
The example below demonstrates the use of ‘Utils.Http.IsValidURL‘ to check whether a string is a valid HTTP URL.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
' Is Valid URL ' Declare urls Dim urls = New String() { "https://www.programmingnotes.org", "http://www.programmingnotes.org", "www.programmingnotes.org", "programmingnotes.org", "javascript:alert('programmingnotes.org')", "programmingnotes", "programmingnotes.org/path?queryparam=123", "t:/www.programmingnotes.org", ":programmingnotes.org", "http/programmingnotes.org", "h91ps://programmingnotes.org/", "//programmingnotes.org/", "www@.programmingnotes.org", "protocol://programmingnotes.org/path?queryparam=123", ":programmingnotes.org/", ":programmingnotes.org:80/", "programmingnotes.org:80/", "mailto:test@email.com", "test@email.com", "file:///path/to/file/text.doc", "http://192.168.1.1/", "192.168.1.1" } ' Check if valid For Each url In urls Debug.Print($"URL: {url}, Is Valid: {Utils.Http.IsValidURL(url)}") Next ' expected output: ' URL: https://www.programmingnotes.org, Is Valid: True ' URL: http://www.programmingnotes.org, Is Valid: True ' URL: www.programmingnotes.org, Is Valid: True ' URL: programmingnotes.org, Is Valid: True ' URL: javascript:alert('programmingnotes.org'), Is Valid: False ' URL: programmingnotes, Is Valid: False ' URL: programmingnotes.org/path?queryparam=123, Is Valid: True ' URL: t:/www.programmingnotes.org, Is Valid: False ' URL: :programmingnotes.org, Is Valid: False ' URL: http/programmingnotes.org, Is Valid: False ' URL: h91ps://programmingnotes.org/, Is Valid: False ' URL: //programmingnotes.org/, Is Valid: False ' URL: www@.programmingnotes.org, Is Valid: False ' URL: protocol://programmingnotes.org/path?queryparam=123, Is Valid: False ' URL: :programmingnotes.org/, Is Valid: False ' URL: :programmingnotes.org:80/, Is Valid: False ' URL: programmingnotes.org:80/, Is Valid: True ' URL: mailto:test@email.com, Is Valid: False ' URL: test@email.com, Is Valid: False ' URL: file:///path/to/file/text.doc, Is Valid: False ' URL: http://192.168.1.1/, Is Valid: True ' URL: 192.168.1.1, Is Valid: True |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 18, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace Http Public Module modHttp ''' <summary> ''' Determines if a url is valid or not ''' </summary> ''' <param name="url">The url to check</param> ''' <returns>True if a url is valid, false otherwise</returns> Public Function IsValidURL(url As String) As Boolean Dim period = url.IndexOf(".") If period > -1 AndAlso Not url.Contains("@") Then ' Check if there are remnants where the url scheme should be. ' Dont modify string if so Dim colon = url.IndexOf(":") Dim slash = url.IndexOf("/") If (colon = -1 OrElse period < colon) AndAlso (slash = -1 OrElse period < slash) Then url = $"http://{url}" End If End If Dim uriResult As System.Uri = Nothing Dim result = System.Uri.TryCreate(url, System.UriKind.Absolute, uriResult) _ AndAlso (uriResult.Scheme = System.Uri.UriSchemeHttp OrElse uriResult.Scheme = System.Uri.UriSchemeHttps) Return result End Function End Module End Namespace End Namespace ' http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 18, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Sub Main(args As String()) Try ' Declare urls Dim urls = New String() { "https://www.programmingnotes.org", "http://www.programmingnotes.org", "www.programmingnotes.org", "programmingnotes.org", "javascript:alert('programmingnotes.org')", "programmingnotes", "programmingnotes.org/path?queryparam=123", "t:/www.programmingnotes.org", ":programmingnotes.org", "http/programmingnotes.org", "h91ps://programmingnotes.org/", "//programmingnotes.org/", "www@.programmingnotes.org", "protocol://programmingnotes.org/path?queryparam=123", ":programmingnotes.org/", ":programmingnotes.org:80/", "programmingnotes.org:80/", "mailto:test@email.com", "test@email.com", "file:///path/to/file/text.doc", "http://192.168.1.1/", "192.168.1.1" } ' Check if valid For Each url In urls Display($"URL: {url}, Is Valid: {Utils.Http.IsValidURL(url)}") Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Get, Add, Update & Remove Values From A URL Query String Using VB.NET
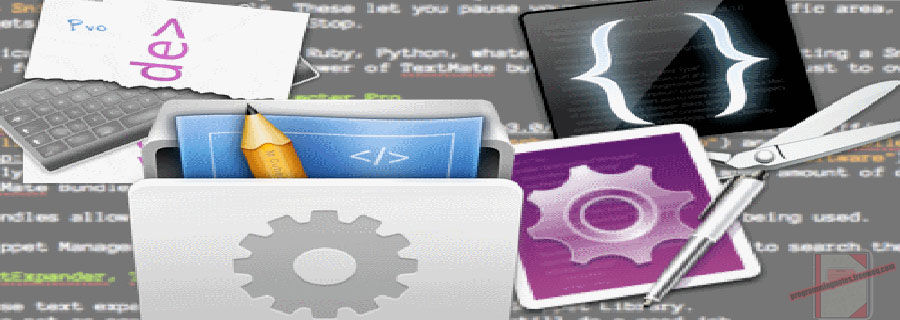
The following is a module with functions which demonstrates how to get, add, update and remove parameters from a query string using C#.
The following functions use System.Web to modify the url.
To use the functions in this module, make sure you have a reference to ‘System.Web‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add Reference‘, then type ‘System.Web‘ in the search box, and add the reference titled System.Web in the results Tab.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
1. Add – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Add‘ to add a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Add - Single Parameter ' Declare url Dim url = "http://programmingnotes.org/" ' Add single key value parameter to the url Dim result = Utils.HttpParams.Add(url, New KeyValuePair(Of String, String)("ids", "1987")) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=1987 |
2. Add – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Add‘ to add a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
' Add - Multiple Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Add multiple key value parameters to the url Dim addition = New System.Collections.Specialized.NameValueCollection() From { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result = Utils.HttpParams.Add(url, addition) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=1987%2c1991%2c2010%2c2019&names=Kenneth%2cJennifer%2cLynn%2cSole |
3. Update – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Update‘ to update a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Update - Single Parameter ' Declare url Dim url = "http://programmingnotes.org/?ids=1987" ' Update single key value parameter to the url Dim result = Utils.HttpParams.Update(url7, New KeyValuePair(Of String, String)("ids", "1991")) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=1991 |
4. Update – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Update‘ to update a query string parameter to a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
' Update - Multiple Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Update multiple key value parameters to the url Dim addition = New System.Collections.Specialized.NameValueCollection() From { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result = Utils.HttpParams.Update(url, addition) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=2010%2c2019&names=Lynn%2cSole |
5. Remove – Single Parameter
The example below demonstrates the use of ‘Utils.HttpParams.Remove‘ to remove a query string parameter from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Remove - Single Parameter ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove single parameter from the url Dim result = Utils.HttpParams.Remove(url, "names") ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/?ids=1987%2c1991 |
6. Remove – Multiple Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Remove‘ to remove multiple query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Remove - Multiple Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove multiple parameters from the url Dim result = Utils.HttpParams.Remove(url, New String() {"ids", "names"}) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/ |
7. Clear – Remove All Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Clear‘ to remove all query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
' Clear - Remove All Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove multiple parameters from the url Dim result = Utils.HttpParams.Clear(url) ' Display result Debug.Print(result) ' expected output: ' http://programmingnotes.org/ |
8. Get – Get All Parameters
The example below demonstrates the use of ‘Utils.HttpParams.Get‘ to get all query string parameters from a url.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
' Get - Get All Parameters ' Declare url Dim url = "http://programmingnotes.org/?ids=1987,1991,2010,2019&names=Kenneth,Jennifer,Lynn,Sole" ' Get parameters from the url Dim result = Utils.HttpParams.Get(url) ' Display result For Each key As String In result Debug.Print($"Parameter: {key}, Value: {result(key)}") Next ' expected output: ' Parameter: ids, Value: 1987,1991,2010,2019 ' Parameter: names, Value: Kenneth,Jennifer,Lynn,Sole |
9. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 17, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace HttpParams Public Module modHttpParams ''' <summary> ''' Adds the query string parameters to a url ''' </summary> ''' <param name="url">The url to append parameters</param> ''' <param name="query">The parameters to add to the url</param> ''' <returns>The url appended with the specified query</returns> Function Add(url As String, query As System.Collections.Specialized.NameValueCollection) As String Return UpdateQuery(url, Sub(queryString) Dim merged = New System.Collections.Specialized.NameValueCollection From { queryString, query } CopyProps(merged, queryString) End Sub) End Function ''' <summary> ''' Adds the query string parameters to a url ''' </summary> ''' <param name="url">The url to append parameters</param> ''' <param name="param">The parameters to add to the url</param> ''' <returns>The url appended with the specified query</returns> Function Add(url As String, param As KeyValuePair(Of String, String)) As String Return Add(url, New System.Collections.Specialized.NameValueCollection() From { {param.Key, param.Value} }) End Function ''' <summary> ''' Updates the query string parameters to a url ''' </summary> ''' <param name="url">The url to update parameters</param> ''' <param name="query">The parameters to update to the url</param> ''' <returns>The url updated with the specified query</returns> Function Update(url As String, query As System.Collections.Specialized.NameValueCollection) As String Return UpdateQuery(url, Sub(queryString) CopyProps(query, queryString) End Sub) End Function ''' <summary> ''' Updates the query string parameters to a url ''' </summary> ''' <param name="url">The url to update parameters</param> ''' <param name="param">The parameters to update to the url</param> ''' <returns>The url updated with the specified query</returns> Function Update(url As String, param As KeyValuePair(Of String, String)) As String Return Update(url, New System.Collections.Specialized.NameValueCollection() From { {param.Key, param.Value} }) End Function ''' <summary> ''' Removes the parameters with the matching keys from the query string of a url ''' </summary> ''' <param name="url">The url to remove parameters</param> ''' <param name="keys">The parameter keys to remove from the url</param> ''' <returns>The url with the parameters removed</returns> Function Remove(url As String, keys As IEnumerable(Of String)) As String Return UpdateQuery(url, Sub(queryString) For Each key In keys If queryString.AllKeys.Contains(key) Then queryString.Remove(key) End If Next End Sub) End Function ''' <summary> ''' Removes the parameter with the matching key from the query string of a url ''' </summary> ''' <param name="url">The url to remove a parameter</param> ''' <param name="key">The parameter key to remove from the url</param> ''' <returns>The url with the parameter removed</returns> Function Remove(url As String, key As String) As String Return Remove(url, New String() {key}) End Function ''' <summary> ''' Removes all parameters from the query string of a url ''' </summary> ''' <param name="url">The url to clear parameters</param> ''' <returns>The url with the parameters removed</returns> Function Clear(url As String) As String Return UpdateQuery(url, Sub(queryString) queryString.Clear() End Sub) End Function ''' <summary> ''' Returns all parameters from the query string of a url ''' </summary> ''' <param name="url">The url to get parameters</param> ''' <returns>The url parameters</returns> Function [Get](url As String) As System.Collections.Specialized.NameValueCollection Dim result As System.Collections.Specialized.NameValueCollection = Nothing UpdateQuery(url, Sub(queryString) result = queryString End Sub) Return result End Function ''' <summary> ''' Sets the query string parameters to a url ''' </summary> ''' <param name="url">The url to set parameters</param> ''' <param name="query">The parameters to set to the url</param> ''' <returns>The url set with the specified query</returns> Function [Set](url As String, query As System.Collections.Specialized.NameValueCollection) As String url = Clear(url) Return Add(url, query) End Function ''' <summary> ''' Sets the query string parameters to a url ''' </summary> ''' <param name="url">The url to set parameters</param> ''' <param name="param">The parameters to set to the url</param> ''' <returns>The url set with the specified query</returns> Function [Set](url As String, param As KeyValuePair(Of String, String)) As String Return [Set](url, New System.Collections.Specialized.NameValueCollection() From { {param.Key, param.Value} }) End Function Private Sub CopyProps(source As System.Collections.Specialized.NameValueCollection, destination As System.Collections.Specialized.NameValueCollection) For Each key As String In source destination(key) = source(key) Next End Sub Private Function UpdateQuery(url As String, modifyQuery As Action(Of System.Collections.Specialized.NameValueCollection)) As String Dim uriBuilder = New System.UriBuilder(url) Dim query = System.Web.HttpUtility.ParseQueryString(uriBuilder.Query) modifyQuery(query) uriBuilder.Query = query.ToString() Return uriBuilder.Uri.ToString() End Function End Module End Namespace End Namespace ' http://programmingnotes.org/ |
10. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 17, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Sub Main(args As String()) Try ' Declare url Dim url = "http://programmingnotes.org/" ' Add single key value parameter to the url Dim result = Utils.HttpParams.Add(url, New KeyValuePair(Of String, String)("ids", "1987")) ' Display result Display(result) Display("") ' Declare url Dim url2 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Add multiple key value parameters to the url Dim addition = New System.Collections.Specialized.NameValueCollection() From { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result2 = Utils.HttpParams.Add(url2, addition) ' Display result Display(result2) Display("") ' Declare url Dim url3 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove single parameter from the url Dim result3 = Utils.HttpParams.Remove(url3, "names") ' Display result Display(result3) Display("") ' Declare url Dim url4 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove multiple parameters from the url Dim result4 = Utils.HttpParams.Remove(url4, New String() {"ids", "names"}) ' Display result Display(result4) Display("") ' Declare url Dim url5 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Remove multiple parameters from the url Dim result5 = Utils.HttpParams.Clear(url5) ' Display result Display(result5) Display("") ' Declare url Dim url6 = "http://programmingnotes.org/?ids=1987,1991,2010,2019&names=Kenneth,Jennifer,Lynn,Sole" ' Get parameters from the url Dim result6 = Utils.HttpParams.Get(url6) ' Display result For Each key As String In result6 Display($"Parameter: {key}, Value: {result6(key)}") Next Display("") ' Declare url Dim url7 = "http://programmingnotes.org/?ids=1987" ' Update single key value parameter to the url Dim result7 = Utils.HttpParams.Update(url7, New KeyValuePair(Of String, String)("ids", "1991")) ' Display result Display(result7) Display("") ' Declare url Dim url8 = "http://programmingnotes.org/?ids=1987,1991&names=Kenneth,Jennifer" ' Update multiple key value parameters to the url Dim addition8 = New System.Collections.Specialized.NameValueCollection() From { {"ids", "2010"}, {"ids", "2019"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result8 = Utils.HttpParams.Update(url8, addition8) ' Display result Display(result8) Display("") ' Declare url Dim url9 = "http://programmingnotes.org/?ids=1987&names=Kenneth" ' Set multiple key value parameters to the url Dim addition9 = New System.Collections.Specialized.NameValueCollection() From { {"ids", "1991"}, {"ids", "2010"}, {"ids", "2019"}, {"names", "Jennifer"}, {"names", "Lynn"}, {"names", "Sole"} } ' Update the url Dim result9 = Utils.HttpParams.Set(url9, addition9) ' Display result Display(result9) Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Serialize & Deserialize JSON Using VB.NET
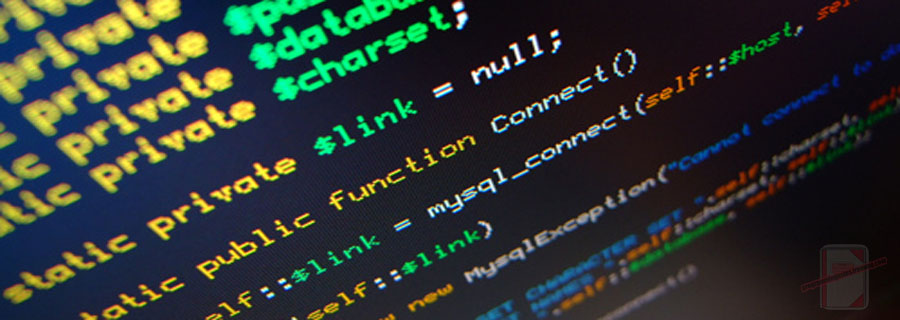
The following is a module with functions which demonstrates how to serialize and deserialize Json using VB.NET.
The following generic functions use Newtonsoft.Json to serialize and deserialize an object.
Note: To use the functions in this module, make sure you have the ‘Newtonsoft.Json‘ package installed in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Manage NuGet Packages….‘, then type ‘Newtonsoft.Json‘ in the search box, and install the package titled Newtonsoft.Json in the results Tab.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
1. Serialize – Integer Array
The example below demonstrates the use of ‘Utils.Json.Serialize‘ to serialize an integer array to Json.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
' Serialize - Integer Array ' Declare array of integers Dim numbers = New Integer() {1987, 19, 22, 2009, 2019, 1991, 28, 31} ' Optional: Create the JsonSerializerSettings with optional settings. Dim settings = New Newtonsoft.Json.JsonSerializerSettings With { .NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore } ' Serialize to Json Dim numbersJson = Utils.Json.Serialize(numbers) ' Display Json Debug.Print(numbersJson) ' expected output: ' [ ' 1987, ' 19, ' 22, ' 2009, ' 2019, ' 1991, ' 28, ' 31 ' ] |
2. Serialize – String List
The example below demonstrates the use of ‘Utils.Json.Serialize‘ to serialize a list of strings to Json.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
' Serialize - String List ' Declare list of strings Dim names = New List(Of String) From { "Kenneth", "Jennifer", "Lynn", "Sole" } ' Serialize to Json Dim namesJson = Utils.Json.Serialize(names) ' Display Json Debug.Print(namesJson) ' expected output: ' [ ' "Kenneth", ' "Jennifer", ' "Lynn", ' "Sole" ' ] |
3. Serialize – Custom Object List
The example below demonstrates the use of ‘Utils.Json.Serialize‘ to serialize a list of custom objects to Json.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
' Serialize - Custom Object List Public Class Part Public Property PartName As String Public Property PartId As Integer End Class ' Declare list of objects Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} ' Serialize to Json Dim partsJson = Utils.Json.Serialize(parts) ' Display Json Debug.Print(partsJson) ' expected output: ' [ ' { ' "PartName": "crank arm", ' "PartId": 1234 ' }, ' { ' "PartName": "chain ring", ' "PartId": 1334 ' }, ' { ' "PartName": "regular seat", ' "PartId": 1434 ' }, ' { ' "PartName": "banana seat", ' "PartId": 1444 ' }, ' { ' "PartName": "cassette", ' "PartId": 1534 ' }, ' { ' "PartName": "shift lever", ' "PartId": 1634 ' } ' ] |
4. Deserialize – Integer Array
The example below demonstrates the use of ‘Utils.Json.Deserialize‘ to deserialize Json to an integer array.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
' Deserialize - Integer Array ' Declare Json array of integers Dim numbersJson = " [ 1987, 19, 22, 2009, 2019, 1991, 28, 31 ] " ' Optional: Create the JsonSerializerSettings with optional settings. Dim settings = New Newtonsoft.Json.JsonSerializerSettings With { .NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore } ' Deserialize from Json to specified type Dim numbers = Utils.Json.Deserialize(Of Integer())(numbersJson) ' Display the items For Each item In numbers Debug.Print($"{item}") Next ' expected output: ' 1987 ' 19 ' 22 ' 2009 ' 2019 ' 1991 ' 28 ' 31 |
5. Deserialize – String List
The example below demonstrates the use of ‘Utils.Json.Deserialize‘ to deserialize Json to a list of strings.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
' Deserialize - String List ' Declare Json array of strings Dim namesJson = " [ ""Kenneth"", ""Jennifer"", ""Lynn"", ""Sole"" ] " ' Deserialize from Json to specified type Dim names = Utils.Json.Deserialize(Of List(Of String))(namesJson) ' Display the items For Each item In names Debug.Print($"{item}") Next ' expected output: ' Kenneth ' Jennifer ' Lynn ' Sole |
6. Deserialize – Custom Object List
The example below demonstrates the use of ‘Utils.Json.Deserialize‘ to deserialize Json to a list of objects.
The optional function parameter allows you to specify the Newtonsoft.Json.JsonSerializerSettings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
' Deserialize - Custom Object List Public Class Part Public Property PartName As String Public Property PartId As Integer End Class ' Declare Json array of objects Dim partsJson = " [ { ""PartName"": ""crank arm"", ""PartId"": 1234 }, { ""PartName"": ""chain ring"", ""PartId"": 1334 }, { ""PartName"": ""regular seat"", ""PartId"": 1434 }, { ""PartName"": ""banana seat"", ""PartId"": 1444 }, { ""PartName"": ""cassette"", ""PartId"": 1534 }, { ""PartName"": ""shift lever"", ""PartId"": 1634 } ] " ' Deserialize from Json to specified type Dim parts = Utils.Json.Deserialize(Of List(Of Part))(partsJson) ' Display the items For Each item In parts Debug.Print($"{item.PartId} - {item.PartName}") Next ' expected output: ' 1234 - crank arm ' 1334 - chain ring ' 1434 - regular seat ' 1444 - banana seat ' 1534 - cassette ' 1634 - shift lever |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 17, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace Json Public Module modJson ''' <summary> ''' Serializes the specified value to Json ''' </summary> ''' <param name="value">The value to serialize</param> ''' <param name="settings">The Newtonsoft.Json.JsonSerializerSettings used to serialize the object</param> ''' <returns>The value serialized to Json</returns> Public Function Serialize(value As Object _ , Optional settings As Newtonsoft.Json.JsonSerializerSettings = Nothing) As String If settings Is Nothing Then settings = GetDefaultSettings() End If Return Newtonsoft.Json.JsonConvert.SerializeObject(value, settings) End Function ''' <summary> ''' Deserializes the specified value from Json to Object T ''' </summary> ''' <param name="value">The value to deserialize</param> ''' <param name="settings">The Newtonsoft.Json.JsonSerializerSettings used to deserialize the object</param> ''' <returns>The value deserialized to Object T</returns> Public Function Deserialize(Of T)(value As String _ , Optional settings As Newtonsoft.Json.JsonSerializerSettings = Nothing) As T If settings Is Nothing Then settings = GetDefaultSettings() End If Return Newtonsoft.Json.JsonConvert.DeserializeObject(Of T)(value, settings) End Function Private Function GetDefaultSettings() As Newtonsoft.Json.JsonSerializerSettings Static settings As New Newtonsoft.Json.JsonSerializerSettings With { .Formatting = Newtonsoft.Json.Formatting.Indented } Return settings End Function End Module End Namespace End Namespace ' http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 17, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program Public Class Part Public Property PartName As String Public Property PartId As Integer End Class Sub Main(args As String()) Try ' Declare array of integers Dim numbers = New Integer() {1987, 19, 22, 2009, 2019, 1991, 28, 31} ' Optional: Create the JsonSerializerSettings with optional settings. Dim settings = New Newtonsoft.Json.JsonSerializerSettings With { .NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore } ' Serialize to Json Dim numbersJson = Utils.Json.Serialize(numbers) ' Display Json Display(numbersJson) Display("") ' Declare list of strings Dim names = New List(Of String) From { "Kenneth", "Jennifer", "Lynn", "Sole" } ' Serialize to Json Dim namesJson = Utils.Json.Serialize(names) ' Display Json Display(namesJson) Display("") ' Declare list of objects Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} ' Serialize to Json Dim partsJson = Utils.Json.Serialize(parts) ' Display Json Display(partsJson) Display("") ' Declare Json array of integers Dim numbersJsonTest = " [ 1987, 19, 22, 2009, 2019, 1991, 28, 31 ] " ' Deserialize from Json to specified type Dim numbersTest = Utils.Json.Deserialize(Of Integer())(numbersJsonTest) ' Display the items For Each item In numbersTest Display($"{item}") Next Display("") ' Declare Json array of strings Dim namesJsonTest = " [ ""Kenneth"", ""Jennifer"", ""Lynn"", ""Sole"" ] " ' Deserialize from Json to specified type Dim namesTest = Utils.Json.Deserialize(Of List(Of String))(namesJsonTest) ' Display the items For Each item In namesTest Display($"{item}") Next Display("") ' Declare Json array of objects Dim partsJsonTest = " [ { ""PartName"": ""crank arm"", ""PartId"": 1234 }, { ""PartName"": ""chain ring"", ""PartId"": 1334 }, { ""PartName"": ""regular seat"", ""PartId"": 1434 }, { ""PartName"": ""banana seat"", ""PartId"": 1444 }, { ""PartName"": ""cassette"", ""PartId"": 1534 }, { ""PartName"": ""shift lever"", ""PartId"": 1634 } ] " ' Deserialize from Json to specified type Dim partsTest = Utils.Json.Deserialize(Of List(Of Part))(partsJsonTest) ' Display the items For Each item In partsTest Display($"{item.PartId} - {item.PartName}") Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Serialize & Deserialize XML Using VB.NET
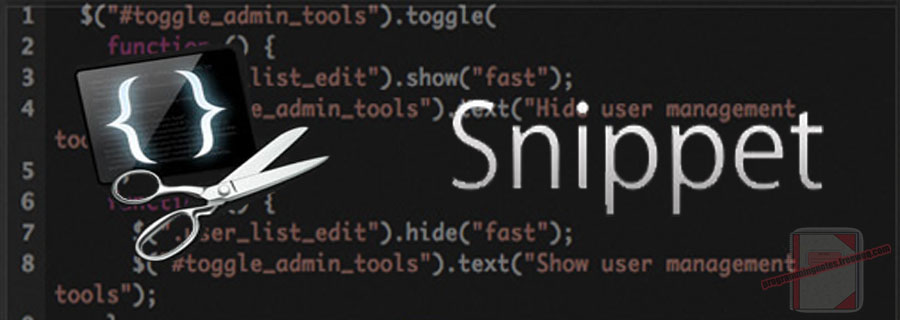
The following is a module with functions which demonstrates how to serialize and deserialize XML using VB.NET.
The following generic functions use System.Xml.Serialization to serialize and deserialize an object.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
1. Serialize – Integer Array
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize an integer array to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
' Serialize - Integer Array ' Declare array of integers Dim numbers = New Integer() {1987, 19, 22, 2009, 2019, 1991, 28, 31} ' Optional: Create an XmlRootAttribute overloaded constructor and set its namespace. Dim myXmlRootAttribute = New System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") With { .Namespace = "http://www.microsoft.com" } ' Serialize to XML Dim numbersXml = Utils.Xml.Serialize(numbers) ' Display XML Debug.Print(numbersXml) ' expected output: ' <?xml version="1.0"?> ' <ArrayOfInt xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> ' <int>1987</int> ' <int>19</int> ' <int>22</int> ' <int>2009</int> ' <int>2019</int> ' <int>1991</int> ' <int>28</int> ' <int>31</int> ' </ArrayOfInt> |
2. Serialize – String List
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize a list of strings to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
' Serialize - String List ' Declare list of strings Dim names = New List(Of String) From { "Kenneth", "Jennifer", "Lynn", "Sole" } ' Serialize to XML Dim namesXml = Utils.Xml.Serialize(names) ' Display XML Debug.Print(namesXml) ' expected output: ' <?xml version="1.0"?> ' <ArrayOfString xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> ' <string>Kenneth</string> ' <string>Jennifer</string> ' <string>Lynn</string> ' <string>Sole</string> ' </ArrayOfString> |
3. Serialize – Custom Object List
The example below demonstrates the use of ‘Utils.Xml.Serialize‘ to serialize a list of custom objects to xml.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 |
' Serialize - Custom Object List <System.Xml.Serialization.XmlRoot(ElementName:="Inventory")> ' Optional Public Class Inventory <System.Xml.Serialization.XmlArray(ElementName:="Parts")> ' Optional Public Property Parts As List(Of Part) End Class Public Class Part <System.Xml.Serialization.XmlElement(ElementName:="PartName")> ' Optional Public Property PartName As String <System.Xml.Serialization.XmlElement(ElementName:="PartId")> ' Optional Public Property PartId As Integer End Class ' Declare list of objects Dim inventory = New Inventory With { .Parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} } ' Serialize to XML Dim inventoryXml = Utils.Xml.Serialize(inventory) ' Display XML Debug.Print(inventoryXml) ' expected output: ' <?xml version="1.0"?> ' <Inventory xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"> ' <Parts> ' <Part> ' <PartName>crank arm</PartName> ' <PartId>1234</PartId> ' </Part> ' <Part> ' <PartName>chain ring</PartName> ' <PartId>1334</PartId> ' </Part> ' <Part> ' <PartName>regular seat</PartName> ' <PartId>1434</PartId> ' </Part> ' <Part> ' <PartName>banana seat</PartName> ' <PartId>1444</PartId> ' </Part> ' <Part> ' <PartName>cassette</PartName> ' <PartId>1534</PartId> ' </Part> ' <Part> ' <PartName>shift lever</PartName> ' <PartId>1634</PartId> ' </Part> ' </Parts> ' </Inventory> |
4. Deserialize – Integer Array
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to an integer array.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
' Deserialize - Integer Array ' Declare Xml array of integers Dim numbersXml = $"<?xml version=""1.0""?> <ArrayOfInt xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <int>1987</int> <int>19</int> <int>22</int> <int>2009</int> <int>2019</int> <int>1991</int> <int>28</int> <int>31</int> </ArrayOfInt> " ' Optional: Create an XmlRootAttribute overloaded constructor and set its namespace. Dim myXmlRootAttribute = New System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") With { .Namespace = "http://www.microsoft.com" } ' Deserialize from XML to specified type Dim numbers = Utils.Xml.Deserialize(Of Integer())(numbersXml) ' Display the items For Each item In numbers Debug.Print($"{item}") Next ' expected output: ' 1987 ' 19 ' 22 ' 2009 ' 2019 ' 1991 ' 28 ' 31 |
5. Deserialize – String List
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to a list of strings.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
' Deserialize - String List ' Declare Xml array of strings Dim namesXml = $"<?xml version=""1.0""?> <ArrayOfString xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <string>Kenneth</string> <string>Jennifer</string> <string>Lynn</string> <string>Sole</string> </ArrayOfString> " ' Deserialize from XML to specified type Dim names = Utils.Xml.Deserialize(Of List(Of String))(namesXml) ' Display the items For Each item In names Debug.Print($"{item}") Next ' expected output: ' Kenneth ' Jennifer ' Lynn ' Sole |
6. Deserialize – Custom Object List
The example below demonstrates the use of ‘Utils.Xml.Deserialize‘ to deserialize xml to a list of objects.
The optional function parameter allows you to specify the System.Xml.Serialization.XmlRootAttribute.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
' Deserialize - Custom Object List <System.Xml.Serialization.XmlRoot(ElementName:="Inventory")> ' Optional Public Class Inventory <System.Xml.Serialization.XmlArray(ElementName:="Parts")> ' Optional Public Property Parts As List(Of Part) End Class Public Class Part <System.Xml.Serialization.XmlElement(ElementName:="PartName")> ' Optional Public Property PartName As String <System.Xml.Serialization.XmlElement(ElementName:="PartId")> ' Optional Public Property PartId As Integer End Class ' Declare Xml array of objects Dim inventoryXml = $"<?xml version=""1.0""?> <Inventory xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance""> <Parts> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </Parts> </Inventory> " ' Deserialize from XML to specified type Dim inventory = Utils.Xml.Deserialize(Of Inventory)(inventoryXml) ' Display the items For Each item In inventory.Parts Debug.Print($"{item.PartId} - {item.PartName}") Next ' expected output: ' 1234 - crank arm ' 1334 - chain ring ' 1434 - regular seat ' 1444 - banana seat ' 1534 - cassette ' 1634 - shift lever |
7. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 16, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace Xml Public Module modXml ''' <summary> ''' Serializes the specified value to XML ''' </summary> ''' <param name="value">The value to serialize</param> ''' <param name="root">The XmlRootAttribute that represents the XML root element</param> ''' <returns>The value serialized to XML</returns> Public Function Serialize(Of T)(value As T _ , Optional root As System.Xml.Serialization.XmlRootAttribute = Nothing) As String Dim xml = String.Empty Dim type = If(value IsNot Nothing, value.GetType, GetType(T)) Dim serializer = New System.Xml.Serialization.XmlSerializer(type:=type, root:=root) Using stream = New System.IO.MemoryStream serializer.Serialize(stream, value) xml = GetStreamText(stream) End Using Return xml End Function ''' <summary> ''' Deserializes the specified value from XML to Object T ''' </summary> ''' <param name="value">The value to deserialize</param> ''' <param name="root">The XmlRootAttribute that represents the XML root element</param> ''' <returns>The value deserialized to Object T</returns> Public Function Deserialize(Of T)(value As String _ , Optional root As System.Xml.Serialization.XmlRootAttribute = Nothing) As T Dim obj As T = Nothing Dim serializer = New System.Xml.Serialization.XmlSerializer(type:=GetType(T), root:=root) Using stringReader = New System.IO.StringReader(value) Using xmlReader = System.Xml.XmlReader.Create(stringReader) obj = CType(serializer.Deserialize(xmlReader), T) End Using End Using Return obj End Function Public Function GetStreamText(stream As System.IO.Stream) As String Try TryResetPosition(stream) Dim stmReader = New System.IO.StreamReader(stream) Dim text = stmReader.ReadToEnd Return text Catch ex As Exception Throw Finally TryResetPosition(stream) End Try End Function Private Sub TryResetPosition(stream As System.IO.Stream) If stream.CanSeek Then stream.Position = 0 End If End Sub End Module End Namespace End Namespace ' http://programmingnotes.org/ |
8. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 16, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Public Module Program <System.Xml.Serialization.XmlRoot(ElementName:="Inventory")> ' Optional Public Class Inventory <System.Xml.Serialization.XmlArray(ElementName:="Parts")> ' Optional Public Property Parts As List(Of Part) End Class Public Class Part Public Property PartName As String Public Property PartId As Integer End Class Sub Main(args As String()) Try ' Declare array of integers Dim numbers = New Integer() {1987, 19, 22, 2009, 2019, 1991, 28, 31} ' Create an XmlRootAttribute overloaded constructor and set its namespace. Dim myXmlRootAttribute = New System.Xml.Serialization.XmlRootAttribute("OverriddenRootElementName") With { .Namespace = "http://www.microsoft.com" } ' Serialize to XML Dim numbersXml = Utils.Xml.Serialize(numbers) ' Display XML Display(numbersXml) Display("") ' Declare list of strings Dim names = New List(Of String) From { "Kenneth", "Jennifer", "Lynn", "Sole" } ' Serialize to XML Dim namesXml = Utils.Xml.Serialize(names) ' Display XML Display(namesXml) Display("") ' Declare list of objects Dim inventory = New Inventory With { .Parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} } ' Serialize to XML Dim inventoryXml = Utils.Xml.Serialize(inventory) ' Display XML Display(inventoryXml) Display("") ' Declare Xml array of integers Dim numbersXmlTest = $"<?xml version=""1.0""?> <ArrayOfInt xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <int>1987</int> <int>19</int> <int>22</int> <int>2009</int> <int>2019</int> <int>1991</int> <int>28</int> <int>31</int> </ArrayOfInt> " ' Deserialize from XML to specified type Dim numbersTest = Utils.Xml.Deserialize(Of Integer())(numbersXmlTest) ' Display the items For Each item In numbersTest Display($"{item}") Next Display("") ' Declare Xml array of strings Dim namesXmlTest = $"<?xml version=""1.0""?> <ArrayOfString xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema""> <string>Kenneth</string> <string>Jennifer</string> <string>Lynn</string> <string>Sole</string> </ArrayOfString> " ' Deserialize from XML to specified type Dim namesTest = Utils.Xml.Deserialize(Of List(Of String))(namesXmlTest) ' Display the items For Each item In namesTest Display($"{item}") Next Display("") ' Declare Xml array of objects Dim inventoryXmlTest = $"<?xml version=""1.0""?> <Inventory xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance""> <Parts> <Part> <PartName>crank arm</PartName> <PartId>1234</PartId> </Part> <Part> <PartName>chain ring</PartName> <PartId>1334</PartId> </Part> <Part> <PartName>regular seat</PartName> <PartId>1434</PartId> </Part> <Part> <PartName>banana seat</PartName> <PartId>1444</PartId> </Part> <Part> <PartName>cassette</PartName> <PartId>1534</PartId> </Part> <Part> <PartName>shift lever</PartName> <PartId>1634</PartId> </Part> </Parts> </Inventory> " ' Deserialize from XML to specified type Dim inventoryTest = Utils.Xml.Deserialize(Of Inventory)(inventoryXmlTest) ' Display the items For Each item In inventoryTest.Parts Display($"{item.PartId} - {item.PartName}") Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Split & Batch An Array/List/IEnumerable Into Smaller Sub-Lists Of N Size Using VB.NET
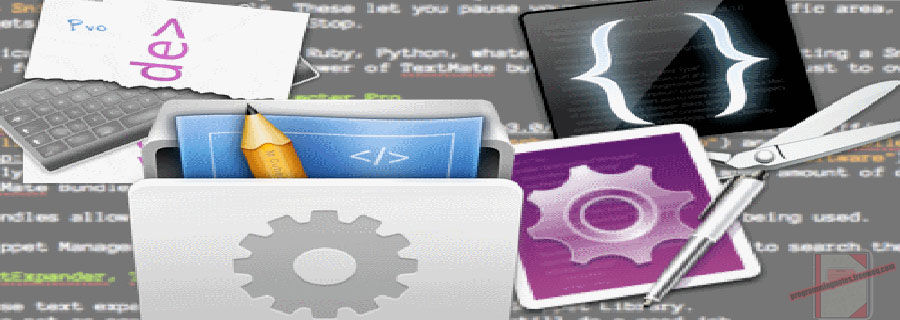
The following is a module with functions which demonstrates how to split/batch an Array/List/IEnumerable into smaller sublists of n size using VB.NET.
This generic extension function uses a simple for loop to group items into batches.
1. Partition – Integer Array
The example below demonstrates the use of ‘Utils.Partition‘ to group an integer array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
' Partition - Integer Array Imports Utils ' Declare array of integers Dim numbers = New Integer() {1987, 19, 22, 2009, 2019, 1991, 28, 31} ' Split array into sub groups Dim numbersPartition = numbers.Partition(3) ' Display grouped batches For batchCount = 0 To numbersPartition.Count - 1 Dim batch = numbersPartition(batchCount) Debug.Print($"Batch #{batchCount + 1}") For Each item In batch Debug.Print($" Item: {item}") Next Next ' expected output: ' Batch #1 ' Item: 1987 ' Item: 19 ' Item: 22 ' Batch #2 ' Item: 2009 ' Item: 2019 ' Item: 1991 ' Batch #3 ' Item: 28 ' Item: 31 |
2. Partition – String List
The example below demonstrates the use of ‘Utils.Partition‘ to group a list of strings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
' Partition - String List Imports Utils ' Declare list of strings Dim names = New List(Of String) From { "Kenneth", "Jennifer", "Lynn", "Sole" } ' Split array into sub groups Dim namesPartition = names.Partition(2) ' Display grouped batches For batchCount = 0 To namesPartition.Count - 1 Dim batch = namesPartition(batchCount) Debug.Print($"Batch #{batchCount + 1}") For Each item In batch Debug.Print($" Item: {item}") Next Next ' expected output: ' Batch #1 ' Item: Kenneth ' Item: Jennifer ' Batch #2 ' Item: Lynn ' Item: Sole |
3. Partition – Custom Object List
The example below demonstrates the use of ‘Utils.Partition‘ to group a list of custom objects.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
' Partition - Custom Object List Imports Utils Public Class Part Public Property PartName As String Public Property PartId As Integer End Class ' Declare list of objects Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} ' Split array into sub groups Dim partsPartition = parts.Partition(4) ' Display grouped batches For batchCount = 0 To partsPartition.Count - 1 Dim batch = partsPartition(batchCount) Debug.Print($"Batch #{batchCount + 1}") For Each item In batch Debug.Print($" Item: {item.PartId} - {item.PartName}") Next Next ' expected output: ' Batch #1 ' Item: 1234 - crank arm ' Item: 1334 - chain ring ' Item: 1434 - regular seat ' Item: 1444 - banana seat ' Batch #2 ' Item: 1534 - cassette ' Item: 1634 - shift lever |
4. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 15, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Breaks a list into smaller sub-lists of a specified size ''' </summary> ''' <param name="source">An IEnumerable to split into smaller sub-lists</param> ''' <param name="size">The maximum size of each sub-list</param> ''' <returns>The smaller sub-lists of the specified size</returns> <Runtime.CompilerServices.Extension()> Public Function Partition(Of T)(source As IEnumerable(Of T), size As Integer) As List(Of List(Of T)) Dim result = New List(Of List(Of T)) Dim batch As List(Of T) = Nothing For index = 0 To source.Count - 1 If index Mod size = 0 Then batch = New List(Of T) result.Add(batch) End If batch.Add(source(index)) Next Return result End Function End Module End Namespace ' http://programmingnotes.org/ |
5. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 15, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Imports Utils Public Module Program Public Class Part Public Property PartName As String Public Property PartId As Integer End Class Sub Main(args As String()) Try ' Declare array of integers Dim numbers = New Integer() {1987, 19, 22, 2009, 2019, 1991, 28, 31} ' Split array into sub groups Dim numbersPartition = numbers.Partition(3) ' Display grouped batches For batchCount = 0 To numbersPartition.Count - 1 Dim batch = numbersPartition(batchCount) Display($"Batch #{batchCount + 1}") For Each item In batch Display($" Item: {item}") Next Next Display("") ' Declare list of strings Dim names = New List(Of String) From { "Kenneth", "Jennifer", "Lynn", "Sole" } ' Split array into sub groups Dim namesPartition = names.Partition(2) ' Display grouped batches For batchCount = 0 To namesPartition.Count - 1 Dim batch = namesPartition(batchCount) Display($"Batch #{batchCount + 1}") For Each item In batch Display($" Item: {item}") Next Next Display("") ' Declare list of objects Dim parts = New List(Of Part) From { New Part With { .PartName = "crank arm", .PartId = 1234 }, New Part With { .PartName = "chain ring", .PartId = 1334 }, New Part With { .PartName = "regular seat", .PartId = 1434 }, New Part With { .PartName = "banana seat", .PartId = 1444 }, New Part With { .PartName = "cassette", .PartId = 1534 }, New Part With { .PartName = "shift lever", .PartId = 1634 }} ' Split array into sub groups Dim partsPartition = parts.Partition(4) ' Display grouped batches For batchCount = 0 To partsPartition.Count - 1 Dim batch = partsPartition(batchCount) Display($"Batch #{batchCount + 1}") For Each item In batch Display($" Item: {item.PartId} - {item.PartName}") Next Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Set & Get The Description Of An Enum Using VB.NET
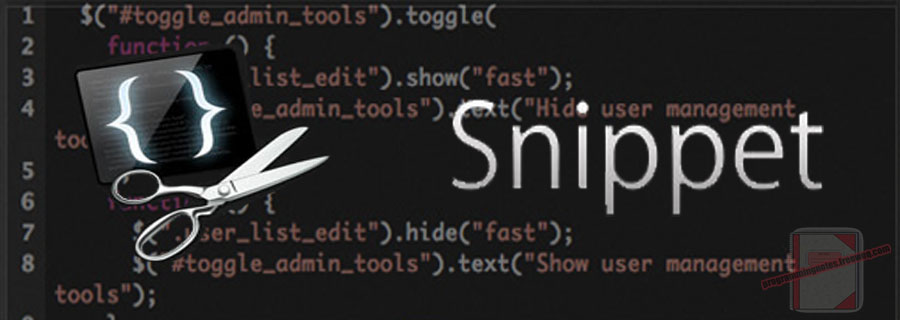
The following is a module with functions which demonstrates how to set and get the description of an enum using VB.NET.
This function uses the DescriptionAttribute to get the description of an Enum element. If a description attribute is not specified, the string value of the Enum element is returned.
1. Get Description
The example below demonstrates the use of ‘Utils.GetDescription‘ to get the description of enum elements.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
' Get Description Imports Utils Public Enum MimeTypes <System.ComponentModel.Description("application/pdf")> ApplicationPDF = 1 <System.ComponentModel.Description("application/octet-stream")> ApplicationOctect = 2 <System.ComponentModel.Description("application/zip")> ApplicationZip = 3 <System.ComponentModel.Description("application/msword")> ApplicationMSWordDOC = 4 <System.ComponentModel.Description("application/vnd.openxmlformats-officedocument.wordprocessingml.document")> ApplicationMSWordDOCX = 5 <System.ComponentModel.Description("application/vnd.ms-excel")> ApplicationMSExcelXLS = 6 <System.ComponentModel.Description("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet")> ApplicationMSExcelXLSX = 7 End Enum For Each value As MimeTypes In System.[Enum].GetValues(GetType(MimeTypes)) Debug.Print($"Numeric Value: {CInt(value)}, Name: {value.ToString}, Description: {value.GetDescription}") Next ' expected output: ' Numeric Value: 1, Name: ApplicationPDF, Description: application/pdf ' Numeric Value: 2, Name: ApplicationOctect, Description: application/octet-stream ' Numeric Value: 3, Name: ApplicationZip, Description: application/zip ' Numeric Value: 4, Name: ApplicationMSWordDOC, Description: application/msword ' Numeric Value: 5, Name: ApplicationMSWordDOCX, Description: application/vnd.openxmlformats-officedocument.wordprocessingml.document" ' Numeric Value: 6, Name: ApplicationMSExcelXLS, Description: application/vnd.ms-excel ' Numeric Value: 7, Name: ApplicationMSExcelXLSX, Description: application/vnd.openxmlformats-officedocument.spreadsheetml.sheet |
2. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 11, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Returns the description of an enum decorated with a DescriptionAttribute ''' </summary> ''' <param name="value">An Enumeration value</param> ''' <returns>The description of an enumeration value</returns> <Runtime.CompilerServices.Extension()> Public Function GetDescription(value As System.[Enum]) As String Dim description = value.ToString Dim attribute = GetAttribute(Of System.ComponentModel.DescriptionAttribute)(value) If attribute IsNot Nothing Then description = attribute.Description End If Return description End Function Public Function GetAttribute(Of T As {System.Attribute})(value As System.[Enum]) As T Dim field = value.GetType().GetField(value.ToString()) Dim attribute = CType(field.GetCustomAttributes(GetType(T), False), T()).FirstOrDefault Return attribute End Function End Module End Namespace ' http://programmingnotes.org/ |
3. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 11, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Imports Utils Module Program Public Enum MimeTypes <System.ComponentModel.Description("application/pdf")> ApplicationPDF = 1 <System.ComponentModel.Description("application/octet-stream")> ApplicationOctect = 2 <System.ComponentModel.Description("application/zip")> ApplicationZip = 3 <System.ComponentModel.Description("application/msword")> ApplicationMSWordDOC = 4 <System.ComponentModel.Description("application/vnd.openxmlformats-officedocument.wordprocessingml.document")> ApplicationMSWordDOCX = 5 <System.ComponentModel.Description("application/vnd.ms-excel")> ApplicationMSExcelXLS = 6 <System.ComponentModel.Description("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet")> ApplicationMSExcelXLSX = 7 <System.ComponentModel.Description("application/vnd.ms-powerpoint")> ApplicationMSPowerPointPPT = 8 <System.ComponentModel.Description("application/vnd.openxmlformats-officedocument.presentationml.presentation")> ApplicationMSPowerPointPPTX = 9 <System.ComponentModel.Description("audio/basic")> AudioBasic = 10 <System.ComponentModel.Description("audio/mpeg")> AudioMPEG = 11 <System.ComponentModel.Description("audio/x-wav")> AudioWAV = 12 <System.ComponentModel.Description("image/gif")> ImageGIF = 13 <System.ComponentModel.Description("image/jpeg")> ImageJPEG = 14 <System.ComponentModel.Description("image/png")> ImagePNG = 15 <System.ComponentModel.Description("text/html")> TextHTML = 16 <System.ComponentModel.Description("text/plain")> TextPlain = 17 <System.ComponentModel.Description("video/mpeg")> VideoMPEG = 18 <System.ComponentModel.Description("video/x-msvideo")> VideoAVI = 19 End Enum Sub Main(args As String()) Try For Each value As MimeTypes In System.[Enum].GetValues(GetType(MimeTypes)) Display($"Numeric Value: {CInt(value)}, Name: {value.ToString}, Description: {value.GetDescription}") Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Iterate & Get The Values Of An Enum Using VB.NET
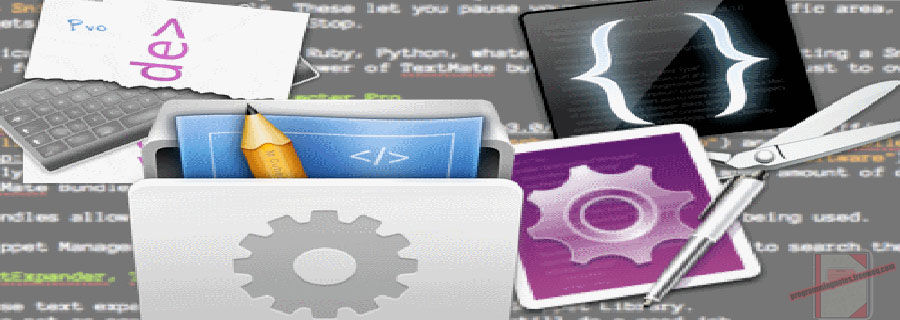
The following is a module with functions which demonstrates how to iterate and get the values of an enum using VB.NET.
This function is a generic wrapper for the Enum.GetValues function.
1. Get Values – Basic Enum
The example below demonstrates the use of ‘Utils.GetEnumValues‘ to get the values of a simple Enum.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
' Get Values - Basic Enum Enum Colors Red Green Blue Yellow End Enum Dim colors = Utils.GetEnumValues(Of Colors) For Each color In colors Debug.Print($"Numeric Value: {CInt(color)}, Name: {color.ToString}") Next ' expected output: ' Numeric Value: 0, Name: Red ' Numeric Value: 1, Name: Green ' Numeric Value: 2, Name: Blue ' Numeric Value: 3, Name: Yellow |
2. Get Values – Numbered Enum
The example below demonstrates the use of ‘Utils.GetEnumValues‘ to get the values of a numbered Enum.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
' Get Values - Numbered Enum Public Enum Pets None = 0 Dog = 1 Cat = 2 Rodent = 4 Bird = 8 Fish = 16 Reptile = 32 Other = 64 End Enum Dim pets = Utils.GetEnumValues(Of Pets) For Each pet In pets Debug.Print($"Numeric Value: {CInt(pet)}, Name: {pet.ToString}") Next ' expected output: ' Numeric Value: 0, Name: None ' Numeric Value: 1, Name: Dog ' Numeric Value: 2, Name: Cat ' Numeric Value: 4, Name: Rodent ' Numeric Value: 8, Name: Bird ' Numeric Value: 16, Name: Fish ' Numeric Value: 32, Name: Reptile ' Numeric Value: 64, Name: Other |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 11, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Returns a List of the values of the constants in a specified enumeration ''' </summary> ''' <returns>The values contained in the enumeration</returns> Public Function GetEnumValues(Of T)() As List(Of T) Dim type = GetType(T) If Not type.IsSubclassOf(GetType(System.[Enum])) Then Throw New InvalidCastException($"Unable to cast '{type.FullName}' to System.Enum") End If Dim result = New List(Of T) For Each value As T In System.[Enum].GetValues(type) result.Add(value) Next Return result End Function End Module End Namespace ' http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 11, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Module Program Enum Colors Red Green Blue Yellow End Enum Public Enum Pets None = 0 Dog = 1 Cat = 2 Rodent = 4 Bird = 8 Fish = 16 Reptile = 32 Other = 64 End Enum Sub Main(args As String()) Try Dim colors = Utils.GetEnumValues(Of Colors) For Each color In colors Display($"Numeric Value: {CInt(color)}, Name: {color.ToString}") Next Display("") Dim pets = Utils.GetEnumValues(Of Pets) For Each pet In pets Display($"Numeric Value: {CInt(pet)}, Name: {pet.ToString}") Next Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Resize & Rotate Image, Convert Image To Byte Array, Change Image Format, & Fix Image Orientation Using VB.NET
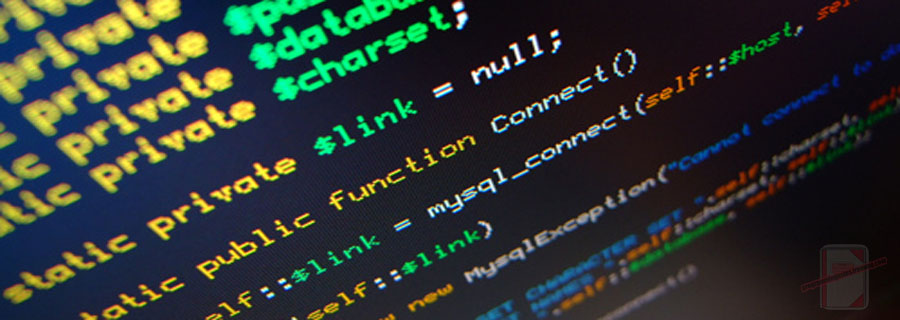
The following is a module with functions which demonstrates how to resize an image, rotate an image to a specific angle, convert an image to a byte array, change an image format, and fix an image orientation Using VB.NET.
Contents
1. Overview
2. Resize & Rotate - Image
3. Resize & Rotate - Byte Array
4. Resize & Rotate - Memory Stream
5. Convert Image To Byte Array
6. Change Image Format
7. Fix Image Orientation
8. Utils Namespace
9. More Examples
1. Overview
To use the functions in this module, make sure you have a reference to ‘System.Drawing‘ in your project.
One way to do this is, in your Solution Explorer (where all the files are shown with your project), right click the ‘References‘ folder, click ‘Add References‘, then type ‘System.Drawing‘ in the search box, and add a reference to System.Drawing in the results Tab.
Note: Don’t forget to include the ‘Utils Namespace‘ before running the examples!
2. Resize & Rotate – Image
The example below demonstrates the use of ‘Utils.Image.Resize‘ and ‘Utils.Image.Rotate‘ to resize and rotate an image object.
Resizing and rotating an image returns a newly created image object.
1 2 3 4 5 6 7 8 9 10 11 12 |
' Resize & Rotate - Image Imports Utils.Image Dim path = $"path-to-file\file.extension" Dim image = System.Drawing.Image.FromFile(path) ' Resize image 500x500 Dim resized = image.Resize(New System.Drawing.Size(500, 500)) ' Rotate 90 degrees counter clockwise Dim rotated = image.Rotate(-90) |
3. Resize & Rotate – Byte Array
The example below demonstrates the use of ‘Utils.Image.Resize‘ and ‘Utils.Image.Rotate‘ to resize and rotate a byte array.
Resizing and rotating an image returns a newly created image object.
1 2 3 4 5 6 7 8 9 10 |
' Resize & Rotate - Byte Array ' Read file to byte array Dim bytes As Byte() ' Resize image 800x800 Dim resized = Utils.Image.Resize(bytes, New System.Drawing.Size(800, 800)) ' Rotate 180 degrees clockwise Dim rotated = Utils.Image.Rotate(bytes, 180) |
4. Resize & Rotate – Memory Stream
The example below demonstrates the use of ‘Utils.Image.Resize‘ and ‘Utils.Image.Rotate‘ to resize and rotate a memory stream.
Resizing and rotating an image returns a newly created image object.
1 2 3 4 5 6 7 8 9 10 |
' Resize & Rotate - Memory Stream ' Read file to memory stream Dim stream As New System.IO.MemoryStream() ' Resize image 2000x2000 Dim resized = Utils.Image.Resize(stream, New System.Drawing.Size(2000, 2000)) ' Rotate 360 degrees Dim rotated = Utils.Image.Rotate(stream, 360) |
5. Convert Image To Byte Array
The example below demonstrates the use of ‘Utils.Image.ToArray‘ to convert an image object to a byte array.
The optional function parameter allows you to specify the image format.
1 2 3 4 5 6 7 8 9 |
' Convert Image To Byte Array Imports Utils.Image Dim path = $"path-to-file\file.extension" Dim image = System.Drawing.Image.FromFile(path) ' Convert image to byte array Dim bytes = image.ToArray() |
6. Change Image Format
The example below demonstrates the use of ‘Utils.Image.ConvertFormat‘ to convert the image object raw format to another format.
Converting an images format returns a newly created image object.
1 2 3 4 5 6 7 8 9 |
' Change Image Format Imports Utils.Image Dim path = $"path-to-file\file.extension" Dim image = System.Drawing.Image.FromFile(path) ' Convert image to another format Dim newImage = image.ConvertFormat(System.Drawing.Imaging.ImageFormat.Png) |
7. Fix Image Orientation
The example below demonstrates the use of ‘Utils.Image.FixOrientation‘ to update the image object to reflect its Exif orientation.
When you view an image in a Photo Viewer, it automatically corrects image orientation if it has Exif orientation data.
‘Utils.Image.FixOrientation‘ makes it so the loaded image object reflects the correct orientation when rendered according to its Exif orientation data.
1 2 3 4 5 6 7 8 9 |
' Fix Image Orientation Imports Utils.Image Dim path = $"path-to-file\file.extension" Dim image = System.Drawing.Image.FromFile(path) ' Correct the image object to reflect its Exif orientation image.FixOrientation |
8. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 9, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Namespace Image Public Module modImage ''' <summary> ''' Resizes an image to a specified size ''' </summary> ''' <param name="source">The image to resize</param> ''' <param name="desiredSize">The size to resize the image to</param> ''' <param name="preserveAspectRatio">Determines if the aspect ratio should be preserved</param> ''' <returns>A new image resized to the specified size</returns> <Runtime.CompilerServices.Extension()> Public Function Resize(source As System.Drawing.Image, desiredSize As System.Drawing.Size, Optional preserveAspectRatio As Boolean = True) As System.Drawing.Image If desiredSize.IsEmpty Then Throw New ArgumentException("Image size cannot be empty", NameOf(desiredSize)) End If Dim resizedWidth = desiredSize.Width Dim resizedHeight = desiredSize.Height Dim sourceFormat = source.RawFormat source.FixOrientation If preserveAspectRatio Then Dim sourceWidth = Math.Max(source.Width, 1) Dim sourceHeight = Math.Max(source.Height, 1) Dim resizedPercentWidth = desiredSize.Width / sourceWidth Dim resizedPercentHeight = desiredSize.Height / sourceHeight Dim resizedPercentRatio = Math.Min(resizedPercentHeight, resizedPercentWidth) resizedWidth = CInt(sourceWidth * resizedPercentRatio) resizedHeight = CInt(sourceHeight * resizedPercentRatio) End If Dim resizedImage = New System.Drawing.Bitmap(resizedWidth, resizedHeight) resizedImage.SetResolution(source.HorizontalResolution, source.VerticalResolution) Using graphicsHandle = System.Drawing.Graphics.FromImage(resizedImage) graphicsHandle.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic graphicsHandle.CompositingQuality = System.Drawing.Drawing2D.CompositingQuality.HighQuality graphicsHandle.PixelOffsetMode = System.Drawing.Drawing2D.PixelOffsetMode.HighQuality graphicsHandle.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality graphicsHandle.DrawImage(source, 0, 0, resizedWidth, resizedHeight) End Using If Not sourceFormat.Equals(resizedImage.RawFormat) Then resizedImage = CType(resizedImage.ConvertFormat(sourceFormat), System.Drawing.Bitmap) End If Return resizedImage End Function ''' <summary> ''' Resizes an image to a specified size ''' </summary> ''' <param name="stream">The stream of the image to resize</param> ''' <param name="desiredSize">The size to resize the image to</param> ''' <param name="preserveAspectRatio">Determines if the aspect ratio should be preserved</param> ''' <returns>A new image resized to the specified size</returns> Public Function Resize(stream As System.IO.Stream, desiredSize As System.Drawing.Size, Optional preserveAspectRatio As Boolean = True) As System.Drawing.Image Dim image As System.Drawing.Image Using original = StreamToImage(stream) image = Resize(original, desiredSize, preserveAspectRatio:=preserveAspectRatio) End Using Return image End Function ''' <summary> ''' Resizes an image to a specified size ''' </summary> ''' <param name="bytes">The byte array of the image to resize</param> ''' <param name="desiredSize">The size to resize the image to</param> ''' <param name="preserveAspectRatio">Determines if the aspect ratio should be preserved</param> ''' <returns>A new image resized to the specified size</returns> Public Function Resize(bytes As Byte(), desiredSize As System.Drawing.Size, Optional preserveAspectRatio As Boolean = True) As System.Drawing.Image Dim image As System.Drawing.Image Using original = BytesToImage(bytes) image = Resize(original, desiredSize, preserveAspectRatio:=preserveAspectRatio) End Using Return image End Function ''' <summary> ''' Rotates an image to a specified angle ''' </summary> ''' <param name="source">The image to resize</param> ''' <param name="angle">The angle to rotate the image</param> ''' <returns>A new image rotated to the specified angle</returns> <Runtime.CompilerServices.Extension()> Public Function Rotate(source As System.Drawing.Image, angle As Decimal) As System.Drawing.Image Dim sourceFormat = source.RawFormat source.FixOrientation Dim alpha = angle While alpha < 0 alpha += 360 End While Dim gamma = 90D Dim beta = 180 - angle - gamma Dim c1 = source.Height Dim a1 = Math.Abs((c1 * Math.Sin(alpha * Math.PI / 180))) Dim b1 = Math.Abs((c1 * Math.Sin(beta * Math.PI / 180))) Dim c2 = source.Width Dim a2 = Math.Abs((c2 * Math.Sin(alpha * Math.PI / 180))) Dim b2 = Math.Abs((c2 * Math.Sin(beta * Math.PI / 180))) Dim width = CInt(b2 + a1) Dim height = CInt(b1 + a2) Dim rotatedImage = New System.Drawing.Bitmap(width, height) rotatedImage.SetResolution(source.HorizontalResolution, source.VerticalResolution) Using graphicsHandle = System.Drawing.Graphics.FromImage(rotatedImage) graphicsHandle.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic graphicsHandle.CompositingQuality = System.Drawing.Drawing2D.CompositingQuality.HighQuality graphicsHandle.PixelOffsetMode = System.Drawing.Drawing2D.PixelOffsetMode.HighQuality graphicsHandle.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality graphicsHandle.TranslateTransform(rotatedImage.Width \ 2, rotatedImage.Height \ 2) graphicsHandle.RotateTransform(angle) graphicsHandle.TranslateTransform(-rotatedImage.Width \ 2, -rotatedImage.Height \ 2) graphicsHandle.DrawImage(source, New System.Drawing.Point((width - source.Width) \ 2, (height - source.Height) \ 2)) End Using If Not sourceFormat.Equals(rotatedImage.RawFormat) Then rotatedImage = CType(rotatedImage.ConvertFormat(sourceFormat), System.Drawing.Bitmap) End If Return rotatedImage End Function ''' <summary> ''' Rotates an image to a specified angle ''' </summary> ''' <param name="stream">The stream of the image to resize</param> ''' <param name="angle">The angle to rotate the image</param> ''' <returns>A new image rotated to the specified angle</returns> Public Function Rotate(stream As System.IO.Stream, angle As Decimal) As System.Drawing.Image Dim image As System.Drawing.Image Using original = StreamToImage(stream) image = Rotate(original, angle) End Using Return image End Function ''' <summary> ''' Rotates an image to a specified angle ''' </summary> ''' <param name="bytes">The byte array of the image to rotate</param> ''' <param name="angle">The angle to rotate the image</param> ''' <returns>A new image rotated to the specified angle</returns> Public Function Rotate(bytes As Byte(), angle As Decimal) As System.Drawing.Image Dim image As System.Drawing.Image Using original = BytesToImage(bytes) image = Rotate(original, angle) End Using Return image End Function ''' <summary> ''' Converts an image to a byte array ''' </summary> ''' <param name="source">The image to convert</param> ''' <param name="format">The format the byte array image should be converted to</param> ''' <returns>The image as a byte array</returns> <Runtime.CompilerServices.Extension()> Public Function ToArray(source As System.Drawing.Image, Optional format As System.Drawing.Imaging.ImageFormat = Nothing) As Byte() If format Is Nothing Then format = source.ResolvedFormat If format.Equals(System.Drawing.Imaging.ImageFormat.MemoryBmp) Then format = System.Drawing.Imaging.ImageFormat.Png End If End If Dim bytes As Byte() Using stream = New System.IO.MemoryStream source.Save(stream, format) bytes = stream.ToArray End Using Return bytes End Function ''' <summary> ''' Converts an image to a different ImageFormat ''' </summary> ''' <param name="source">The image to convert</param> ''' <param name="format">The format the image should be changed to</param> ''' <returns>The new image converted to the specified format</returns> <Runtime.CompilerServices.Extension()> Public Function ConvertFormat(source As System.Drawing.Image, format As System.Drawing.Imaging.ImageFormat) As System.Drawing.Image Dim bytes = source.ToArray(format) Dim image = BytesToImage(bytes) Return image End Function ''' <summary> ''' Returns the resolved static image format from an images RawFormat ''' </summary> ''' <param name="source">The image to get the format from</param> ''' <returns>The resolved image format</returns> <Runtime.CompilerServices.Extension()> Public Function ResolvedFormat(source As System.Drawing.Image) As System.Drawing.Imaging.ImageFormat Dim format = FindImageFormat(source.RawFormat) Return format End Function ''' <summary> ''' Returns all the ImageFormats as an IEnumerable ''' </summary> ''' <returns>All the ImageFormats as an IEnumerable</returns> Public Function GetImageFormats() As IEnumerable(Of System.Drawing.Imaging.ImageFormat) Static formats As IEnumerable(Of System.Drawing.Imaging.ImageFormat) = GetType(System.Drawing.Imaging.ImageFormat) _ .GetProperties(Reflection.BindingFlags.Static Or Reflection.BindingFlags.Public) _ .Select(Function(p) CType(p.GetValue(Nothing, Nothing), System.Drawing.Imaging.ImageFormat)) Return formats End Function ''' <summary> ''' Returns the static image format from a RawFormat ''' </summary> ''' <param name="raw">The ImageFormat to get info from</param> ''' <returns>The resolved image format</returns> Public Function FindImageFormat(raw As System.Drawing.Imaging.ImageFormat) As System.Drawing.Imaging.ImageFormat Dim format = GetImageFormats().FirstOrDefault(Function(x) raw.Equals(x)) If format Is Nothing Then format = System.Drawing.Imaging.ImageFormat.Png End If Return format End Function ''' <summary> ''' Update the image to reflect its exif orientation ''' </summary> ''' <param name="image">The image to update</param> <Runtime.CompilerServices.Extension()> Public Sub FixOrientation(image As System.Drawing.Image) Dim orientationId = 274 If Not image.PropertyIdList.Contains(orientationId) Then Return End If Dim orientation = BitConverter.ToInt16(image.GetPropertyItem(orientationId).Value, 0) Dim rotateFlip = System.Drawing.RotateFlipType.RotateNoneFlipNone Select Case orientation Case 1 ' Image is correctly oriented rotateFlip = System.Drawing.RotateFlipType.RotateNoneFlipNone Case 2 ' Image has been mirrored horizontally rotateFlip = System.Drawing.RotateFlipType.RotateNoneFlipX Case 3 ' Image has been rotated 180 degrees rotateFlip = System.Drawing.RotateFlipType.Rotate180FlipNone Case 4 ' Image has been mirrored horizontally and rotated 180 degrees rotateFlip = System.Drawing.RotateFlipType.Rotate180FlipX Case 5 ' Image has been mirrored horizontally and rotated 90 degrees clockwise rotateFlip = System.Drawing.RotateFlipType.Rotate90FlipX Case 6 ' Image has been rotated 90 degrees clockwise rotateFlip = System.Drawing.RotateFlipType.Rotate90FlipNone Case 7 ' Image has been mirrored horizontally and rotated 270 degrees clockwise rotateFlip = System.Drawing.RotateFlipType.Rotate270FlipX Case 8 ' Image has been rotated 270 degrees clockwise rotateFlip = System.Drawing.RotateFlipType.Rotate270FlipNone End Select If rotateFlip <> System.Drawing.RotateFlipType.RotateNoneFlipNone Then image.RotateFlip(rotateFlip) End If End Sub ''' <summary> ''' Returns an image from a byte array ''' </summary> ''' <param name="bytes">The bytes representing the image</param> ''' <returns>The image from the byte array</returns> Public Function BytesToImage(bytes As Byte()) As System.Drawing.Image Return StreamToImage(New System.IO.MemoryStream(bytes)) End Function ''' <summary> ''' Returns an image from a stream ''' </summary> ''' <param name="stream">The stream representing the image</param> ''' <returns>The image from the stream</returns> Public Function StreamToImage(stream As System.IO.Stream) As System.Drawing.Image Return System.Drawing.Image.FromStream(stream) End Function End Module End Namespace End Namespace ' http://programmingnotes.org/ |
9. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 9, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Imports Utils.Image Module Program Sub Main(args As String()) Try Dim path = $"" ' Image Dim image = System.Drawing.Image.FromFile(path) 'Dim newImage = image.Resize(New System.Drawing.Size(500, 500)) Dim newImage = image.Rotate(-90) ' Byte 'Dim bytes As Byte() 'Dim newImage = Utils.Image.Resize(bytes, New System.Drawing.Size(800, 800)) 'Dim newImage = Utils.Image.Rotate(bytes, 180) ' Stream 'Dim stream As New System.IO.MemoryStream 'Dim newImage = Utils.Image.Resize(stream, New System.Drawing.Size(2000, 2000)) 'Dim newImage = Utils.Image.Rotate(stream, 360) Dim newPath = $"" newImage.Save(newPath) Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Convert A String To Byte Array & Byte Array To String Using VB.NET
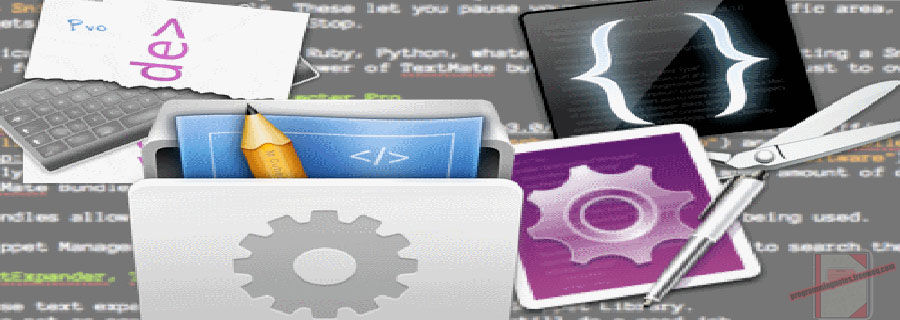
The following is a module with functions which demonstrates how to convert a string to a byte array and a byte array to a string using VB.NET.
1. String To Byte Array
The example below demonstrates the use of ‘Utils.GetBytes‘ to convert a string to a byte array. The optional parameter allows to specify the encoding.
1 2 3 4 5 6 7 8 |
' String To Byte Array Imports Utils Dim text = $"My Programming Notes!" Dim bytes = text.GetBytes() ' Do something with the byte array |
2. Byte Array To String
The example below demonstrates the use of ‘Utils.GetString‘ to convert a byte array to a string. The optional parameter allows to specify the encoding.
1 2 3 4 5 6 7 8 9 |
' Byte Array To String Imports Utils Dim text = $"My Programming Notes!" Dim bytes = text.GetBytes() Dim str = bytes.GetString() ' Do something with the string |
3. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 5, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Decodes all bytes in the specified byte array into a string ''' </summary> ''' <param name="bytes">The byte array containing the sequence of bytes to decode</param> ''' <param name="encode">The type of encoding to be used. Default is UTF8Encoding</param> ''' <returns> A byte array containing the results of encoding</returns> <Runtime.CompilerServices.Extension()> Public Function GetString(bytes As Byte(), Optional encode As System.Text.Encoding = Nothing) As String If encode Is Nothing Then encode = New System.Text.UTF8Encoding End If Return encode.GetString(bytes) End Function ''' <summary> ''' Encodes the characters in the specified string into a sequence of bytes ''' </summary> ''' <param name="str">The string containing the characters to encode</param> ''' <param name="encode">The type of encoding to be used. Default is UTF8Encoding</param> ''' <returns> A byte array containing the results of encoding</returns> <Runtime.CompilerServices.Extension()> Public Function GetBytes(str As String, Optional encode As System.Text.Encoding = Nothing) As Byte() If encode Is Nothing Then encode = New System.Text.UTF8Encoding End If Return encode.GetBytes(str) End Function End Module End Namespace ' http://programmingnotes.org/ |
4. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 5, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Module Program Sub Main(args As String()) Try Dim text = $"My Programming Notes!" Dim bytes = Utils.GetBytes(text) Dim str = Utils.GetString(bytes) Display(str) Display($"{str = text}") Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.
VB.NET || How To Save, Open & Read File As A Byte Array & Memory Stream Using VB.NET
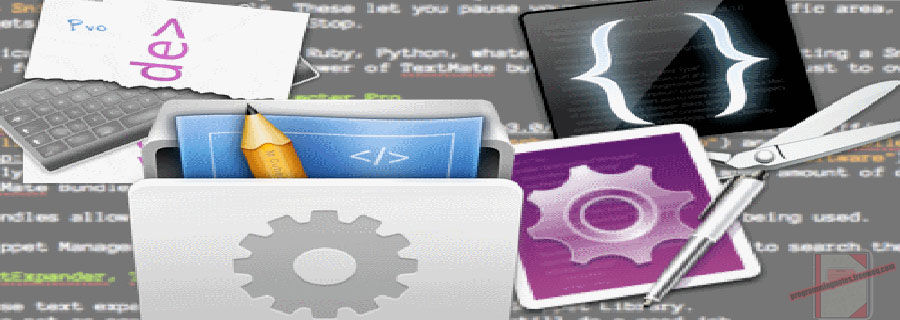
The following is a module with functions which demonstrates how to save, open and read a file as a byte array and memory stream using VB.NET.
1. Read File – Byte Array
The example below demonstrates the use of ‘Utils.ReadFile‘ to read a file as a byte array.
1 2 3 4 5 6 |
' Byte Array Dim path = $"path-to-file\file.extension" Dim fileByteArray = Utils.ReadFile(path) ' Do something with the byte array |
2. Read File – Memory Stream
The example below demonstrates the use of ‘Utils.ReadFile‘ to read a file as a memory stream.
1 2 3 4 5 6 |
' Memory Stream Dim path = $"path-to-file\file.extension" Using fileMS = New System.IO.MemoryStream(Utils.ReadFile(path)) ' Do something with the stream End Using |
3. Save File – Byte Array
The example below demonstrates the use of ‘Utils.SaveFile‘ to save the contents of a byte array to a file.
1 2 3 4 5 6 |
' Byte Array Dim path = $"path-to-file\file.extension" Dim bytes As Byte() Utils.SaveFile(path, bytes) |
4. Save File – Memory Stream
The example below demonstrates the use of ‘Utils.SaveFile‘ to save the contents of a memory stream to a file.
1 2 3 4 5 6 |
' Memory Stream Dim path = $"path-to-file\file.extension" Dim fileMS As System.IO.MemoryStream Utils.SaveFile(path, fileMS.ToArray) |
5. Utils Namespace
The following is the Utils Namespace. Include this in your project to start using!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 5, 2020 ' Taken From: http://programmingnotes.org/ ' File: Utils.vb ' Description: Handles general utility functions ' ============================================================================ Option Strict On Option Explicit On Namespace Global.Utils Public Module modUtils ''' <summary> ''' Reads a file at the given path into a byte array ''' </summary> ''' <param name="filePath">The path of the file to be read</param> ''' <param name="shareOption">Determines how the file will be shared by processes</param> ''' <returns>The contents of the file as a byte array</returns> Public Function ReadFile(filePath As String, Optional shareOption As System.IO.FileShare = System.IO.FileShare.None) As Byte() Dim fileBytes() As Byte Using fs = New System.IO.FileStream(filePath, System.IO.FileMode.Open, System.IO.FileAccess.Read, shareOption) ReDim fileBytes(CInt(fs.Length - 1)) fs.Read(fileBytes, 0, fileBytes.Length) End Using Return fileBytes End Function ''' <summary> ''' Saves a file at the given path with the contents of the byte array ''' </summary> ''' <param name="filePath">The path of the file to be saved</param> ''' <param name="fileBytes">The byte array containing the file contents</param> ''' <param name="shareOption">Determines how the file will be shared by processes</param> Public Sub SaveFile(filePath As String, fileBytes As Byte(), Optional shareOption As System.IO.FileShare = System.IO.FileShare.None) Using fs = New System.IO.FileStream(filePath, System.IO.FileMode.Create, System.IO.FileAccess.Write, shareOption) fs.Write(fileBytes, 0, fileBytes.Length) End Using End Sub End Module End Namespace ' http://programmingnotes.org/ |
6. More Examples
Below are more examples demonstrating the use of the ‘Utils‘ Namespace. Don’t forget to include the module when running the examples!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
' ============================================================================ ' Author: Kenneth Perkins ' Date: Nov 5, 2020 ' Taken From: http://programmingnotes.org/ ' File: Program.vb ' Description: The following demonstrates the use of the Utils Namespace ' ============================================================================ Option Strict On Option Explicit On Imports System Module Program Sub Main(args As String()) Try Dim path = $"" Dim fileByteArray = Utils.ReadFile(path, System.IO.FileShare.None) Display("") Using fileMS = New System.IO.MemoryStream(Utils.ReadFile(path, System.IO.FileShare.None)) ' Do something with the stream End Using Catch ex As Exception Display(ex.ToString) Finally Console.ReadLine() End Try End Sub Public Sub Display(message As String) Console.WriteLine(message) Debug.Print(message) End Sub End Module ' http://programmingnotes.org/ |
QUICK NOTES:
The highlighted lines are sections of interest to look out for.
The code is heavily commented, so no further insight is necessary. If you have any questions, feel free to leave a comment below.